- JAVA经典算法40例
- 【程序1】 题目:古典问题:有一对兔子,从出生后第3个月起每个月都生一对兔子,小兔子长到第四个月后每个月又生一对兔子,假如兔子都不死,问每个月的兔子总数为多少?
- 1.程序分析: 兔子的规律为数列1,1,2,3,5,8,13,21....
- public class exp2{
- public static void main(String args[]){
- int i=0;
- for(i=1;i<=20;i++)
- System.out.println(f(i));
- }
- public static int f(int x)
- {
- if(x==1 || x==2)
- return 1;
- else
- return f(x-1)+f(x-2);
- }
- }
- 或
- public class exp2{
- public static void main(String args[]){
- int i=0;
- math mymath = new math();
- for(i=1;i<=20;i++)
- System.out.println(mymath.f(i));
- }
- }
- class math
- {
- public int f(int x)
- {
- if(x==1 || x==2)
- return 1;
- else
- return f(x-1)+f(x-2);
- }
- }
- 【程序2】 题目:判断101-200之间有多少个素数,并输出所有素数。
- 1.程序分析:判断素数的方法:用一个数分别去除2到sqrt(这个数),如果能被整除,
- 则表明此数不是素数,反之是素数。
- public class exp2{
- public static void main(String args[]){
- int i=0;
- math mymath = new math();
- for(i=2;i<=200;i++)
- if(mymath.iszhishu(i)==true)
- System.out.println(i);
- }
- }
- class math
- {
- public int f(int x)
- {
- if(x==1 || x==2)
- return 1;
- else
- return f(x-1)+f(x-2);
- }
- public boolean iszhishu(int x)
- {
- for(int i=2;i<=x/2;i++)
- if (x % 2==0 )
- return false;
- return true;
- }
- }
- 【程序3】 题目:打印出所有的 "水仙花数 ",所谓 "水仙花数 "是指一个三位数,其各位数字立方和等于该数本身。例如:153是一个 "水仙花数 ",因为153=1的三次方+5的三次方+3的三次方。
- 1.程序分析:利用for循环控制100-999个数,每个数分解出个位,十位,百位。
- public class exp2{
- public static void main(String args[]){
- int i=0;
- math mymath = new math();
- for(i=100;i<=999;i++)
- if(mymath.shuixianhua(i)==true)
- System.out.println(i);
- }
- }
- class math
- {
- public int f(int x)
- {
- if(x==1 || x==2)
- return 1;
- else
- return f(x-1)+f(x-2);
- }
- public boolean iszhishu(int x)
- {
- for(int i=2;i<=x/2;i++)
- if (x % i==0 )
- return false;
- return true;
- }
- public boolean shuixianhua(int x)
- {
- int i=0,j=0,k=0;
- i=x / 100;
- j=(x % 100) /10;
- k=x % 10;
- if(x==i*i*i+j*j*j+k*k*k)
- return true;
- else
- return false;
- }
- }
- 【程序4】 题目:将一个正整数分解质因数。例如:输入90,打印出90=2*3*3*5。
- 程序分析:对n进行分解质因数,应先找到一个最小的质数k,然后按下述步骤完成:
- (1)如果这个质数恰等于n,则说明分解质因数的过程已经结束,打印出即可。
- (2)如果n <> k,但n能被k整除,则应打印出k的值,并用n除以k的商,作为新的正整数你,重复执行第一步。
- (3)如果n不能被k整除,则用k+1作为k的值,重复执行第一步。
- public class exp2{
- public exp2(){}
- public void fengjie(int n){
- for(int i=2;i<=n/2;i++){
- if(n%i==0){
- System.out.print(i+"*");
- fengjie(n/i);
- }
- }
- System.out.print(n);
- System.exit(0);///不能少这句,否则结果会出错
- }
- public static void main(String[] args){
- String str="";
- exp2 c=new exp2();
- str=javax.swing.JOptionPane.showInputDialog("请输入N的值(输入exit退出):");
- int N;
- N=0;
- try{
- N=Integer.parseInt(str);
- }catch(NumberFormatException e){
- e.printStackTrace();
- }
- System.out.print(N+"分解质因数:"+N+"=");
- c.fengjie(N);
- }
- }
- 【程序5】 题目:利用条件运算符的嵌套来完成此题:学习成绩> =90分的同学用A表示,60-89分之间的用B表示,60分以下的用C表示。
- 1.程序分析:(a> b)?a:b这是条件运算符的基本例子。
- import javax.swing.*;
- public class ex5 {
- public static void main(String[] args){
- String str="";
- str=JOptionPane.showInputDialog("请输入N的值(输入exit退出):");
- int N;
- N=0;
- try{
- N=Integer.parseInt(str);
- }
- catch(NumberFormatException e){
- e.printStackTrace();
- }
- str=(N>90?"A":(N>60?"B":"C"));
- System.out.println(str);
- }
- }
- 【程序6】 题目:输入两个正整数m和n,求其最大公约数和最小公倍数。
- 1.程序分析:利用辗除法。
- 最大公约数:
- public class CommonDivisor{
- public static void main(String args[])
- {
- commonDivisor(24,32);
- }
- static int commonDivisor(int M, int N)
- {
- if(N<0||M<0)
- {
- System.out.println("ERROR!");
- return -1;
- }
- if(N==0)
- {
- System.out.println("the biggest common divisor is :"+M);
- return M;
- }
- return commonDivisor(N,M%N);
- }
- }
- 最小公倍数和最大公约数:
- import java.util.Scanner;
- public class CandC
- {
- //下面的方法是求出最大公约数
- public static int gcd(int m, int n)
- {
- while (true)
- {
- if ((m = m % n) == 0)
- return n;
- if ((n = n % m) == 0)
- return m;
- }
- }
- public static void main(String args[]) throws Exception
- {
- //取得输入值
- //Scanner chin = new Scanner(System.in);
- //int a = chin.nextInt(), b = chin.nextInt();
- int a=23; int b=32;
- int c = gcd(a, b);
- System.out.println("最小公倍数:" + a * b / c + "\n最大公约数:" + c);
- }
- }
- 【程序7】 题目:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。
- 1.程序分析:利用while语句,条件为输入的字符不为 '\n '.
- import java.util.Scanner;
- public class ex7 {
- public static void main(String args[])
- {
- System.out.println("请输入字符串:");
- Scanner scan=new Scanner(System.in);
- String str=scan.next();
- String E1="[\u4e00-\u9fa5]";
- String E2="[a-zA-Z]";
- int countH=0;
- int countE=0;
- char[] arrChar=str.toCharArray();
- String[] arrStr=new String[arrChar.length];
- for (int i=0;i<arrChar.length ;i++ )
- {
- arrStr[i]=String.valueOf(arrChar[i]);
- }
- for (String i: arrStr )
- {
- if (i.matches(E1))
- {
- countH++;
- }
- if (i.matches(E2))
- {
- countE++;
- }
- }
- System.out.println("汉字的个数"+countH);
- System.out.println("字母的个数"+countE);
- }
- }
- 【程序8】 题目:求s=a+aa+aaa+aaaa+aa...a的值,其中a是一个数字。例如2+22+222+2222+22222(此时共有5个数相加),几个数相加有键盘控制。
- 1.程序分析:关键是计算出每一项的值。
- import java.io.*;
- public class Sumloop {
- public static void main(String[] args) throws IOException
- {
- int s=0;
- String output="";
- BufferedReader stadin = new BufferedReader(new InputStreamReader(System.in));
- System.out.println("请输入a的值");
- String input =stadin.readLine();
- for(int i =1;i<=Integer.parseInt(input);i++)
- {
- output+=input;
- int a=Integer.parseInt(output);
- s+=a;
- }
Java经典算法四十例编程详解+程序实例
最新推荐文章于 2024-08-20 00:54:19 发布
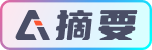