Masha has recently bought a cleaner robot, it can clean a floor without anybody's assistance.
Schematically Masha's room is a rectangle, consisting of w × h square cells of size 1 × 1. Each cell of the room is either empty (represented by character '.'), or occupied by furniture (represented by character '*').
A cleaner robot fully occupies one free cell. Also the robot has a current direction (one of four options), we will say that it looks in this direction.
The algorithm for the robot to move and clean the floor in the room is as follows:
- clean the current cell which a cleaner robot is in;
- if the side-adjacent cell in the direction where the robot is looking exists and is empty, move to it and go to step 1;
- otherwise turn 90 degrees clockwise (to the right relative to its current direction) and move to step 2.
The cleaner robot will follow this algorithm until Masha switches it off.
You know the position of furniture in Masha's room, the initial position and the direction of the cleaner robot. Can you calculate the total area of the room that the robot will clean if it works infinitely?
The first line of the input contains two integers, w and h (1 ≤ w, h ≤ 10) — the sizes of Masha's room.
Next w lines contain h characters each — the description of the room. If a cell of a room is empty, then the corresponding character equals '.'. If a cell of a room is occupied by furniture, then the corresponding character equals '*'. If a cell has the robot, then it is empty, and the corresponding character in the input equals 'U', 'R', 'D' or 'L', where the letter represents the direction of the cleaner robot. Letter 'U' shows that the robot is looking up according to the scheme of the room, letter 'R' means it is looking to the right, letter 'D' means it is looking down and letter 'L' means it is looking to the left.
It is guaranteed that in the given w lines letter 'U', 'R', 'D' or 'L' occurs exactly once. The cell where the robot initially stands is empty (doesn't have any furniture).
In the first line of the output print a single integer — the total area of the room that the robot will clean if it works infinitely.
2 3 U.. .*.
4
4 4 R... .**. .**. ....
12
3 4 ***D ..*. *...
6
In the first sample the robot first tries to move upwards, it can't do it, so it turns right. Then it makes two steps to the right, meets a wall and turns downwards. It moves down, unfortunately tries moving left and locks itself moving from cell (1, 3) to cell (2, 3) and back. The cells visited by the robot are marked gray on the picture.
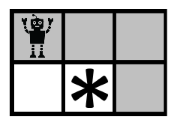
本题目的解题思路很明确,就是dfs,但是这个题目我还是错了好几遍,第一遍在第15个实例上,第二遍在第90个实例上。原因就是没有弄清机器人的行走规则。
提示:
1 、行走过的路径可以
2、在原方向的基础上找到下一条可行路径时候只走这一条(即找到一条就return);
3、因为此题并没要求探求路径,所以不需要修改visited[]状态。
4、当重复在某一区间徘徊时,说明机器人已经走完了能走的地方。
代码与实例如下:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<math.h>
#include<queue>
#include<stack>
#include<iostream>
#include<algorithm>
using namespace std;
typedef int Type;
#define MAX 100
#define RMAX 10
char map[15][15];
int vi[15][15];
int dir[][2]={-1,0,0,1,1,0,0,-1};
int sum;
int w,h;
int judge(int x,int y)
{
if(x>=0 && x<w && y>=0 && y<h && map[x][y]!='*')
return 1;
return 0;
}
void dfs(int x,int y,int di)
{
int count=0;
if(!judge(x,y))
return ;
else
{
if(!vi[x][y])
{
sum++;
vi[x][y]=1;
}
else
{
vi[x][y]++;
if(vi[x][y]>10) //这里是3的时候90实例卡住了,一不做二不休改成了10,就AC了,实例见下图。
return ;
}
for(int i=di;count<4;i++,count++)
{
if(i>=4)
i=i%4;
int x1,y1;
x1=x+dir[i][0]; y1=y+dir[i][1];
if(judge(x1,y1))
{
dfs(x1,y1,i);
return ;
}
}
}
}
int main()
{
while(~scanf("%d%d",&w,&h))
{
sum=0;
memset(vi,0,sizeof(vi));
memset(map,0,sizeof(map));
getchar();
for(int i=0;i<w;i++)
{
scanf("%s",&map[i]);
}
for(int i=0;i<w;i++)
{
for(int j=0;j<h;j++)
{
if(map[i][j]=='U')
{
dfs(i,j,0);
}
else if(map[i][j]=='R')
{
dfs(i,j,1);
}
else if(map[i][j]=='D')
{
dfs(i,j,2);
}
else if(map[i][j]=='L')
{
dfs(i,j,3);
}
}
}
printf("%d\n",sum);
}
return 0;
}
/*实例90
10 10
....*...*.
*.........
..........
.......*..
..........
..*.......
.*....L*..
..*.......
.....*.*.*
......*...
*/
//正确答案为50
/*实例15
10 10
.*********
.*.......*
.*.*****.*
D*.*...*.*
.*.*.*.*.*
.*.***.*.*
.*.....*.*
.*******.*
.*******.*
.........*
*/
//正确答案为10