三消游戏FSM状态机设计图
1) 设计FSM图
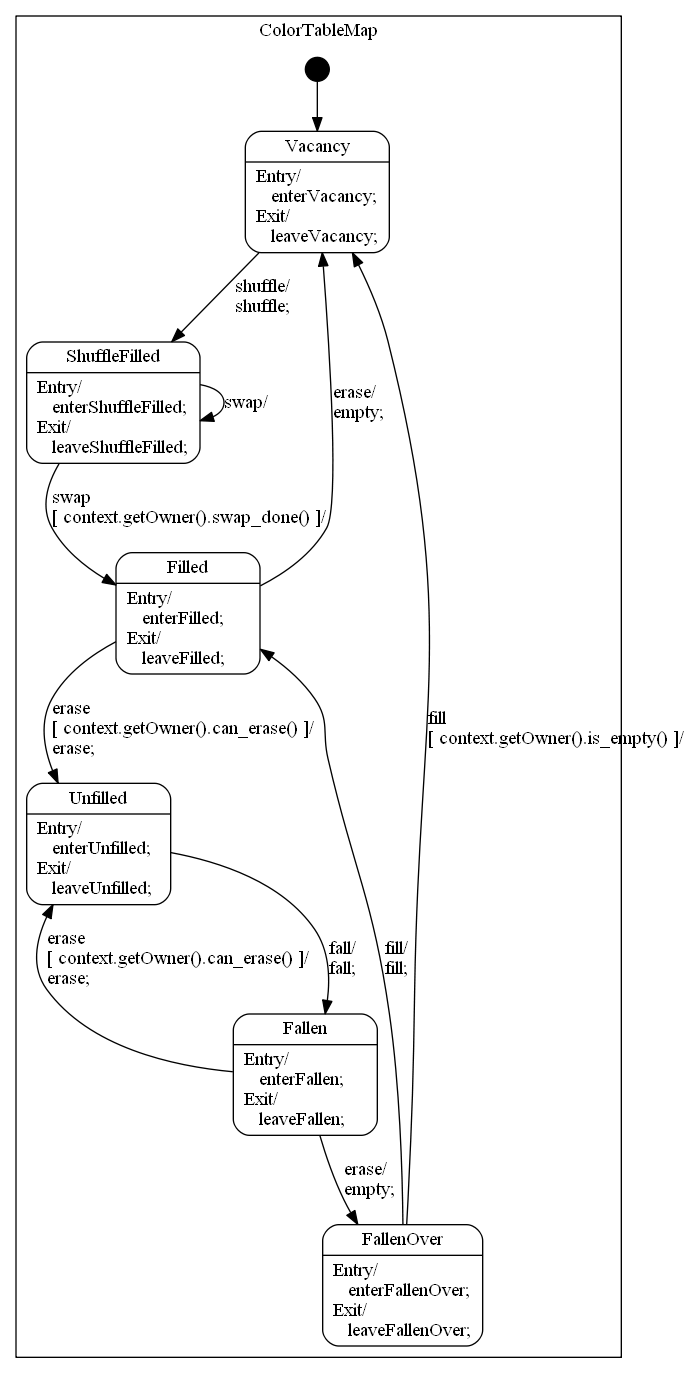
2) smc配置文件
///
// ColorTable.sm
// -- ColorTable State Map for C++ classes auto-generation
//
// 1) generate c++ classes:
// $ java -jar Smc.jar -c++ ColorTable.sm
//
// 2) generate graphviz dot graph:
// $ java -jar Smc.jar -graph -glevel 1 ColorTable.sm
//
// see also:
// http://graphviz.org/
//
// Author: cheungmine
// Copyright 2015-?, All rights reserved.
//
///
%class ColorTable
%header ColorTable.h
%start ColorTableMap::Vacancy
%map ColorTableMap
%%
/**
* State {
* Transition [Guard Condition]
* EndState {
* Action(s)
* }
*
* Transition [context.getOwner().is_valid()]
* EndState {
* Action(s)
* }
* ...
* }
*/
//==> ColorTableMap::Vacancy
Vacancy
Entry {
enterVacancy();
}
Exit {
leaveVacancy();
}
{
// Expose Transitions to other parts if necessary
shuffle
ShuffleFilled {
shuffle();
}
}
//==> ColorTableMap::ShuffleFilled
// only swappable state
ShuffleFilled
Entry {
enterShuffleFilled();
}
Exit {
leaveShuffleFilled();
}
{
// !!can swap here!!
swap [ context.getOwner().swap_done() ]
Filled {
}
swap
ShuffleFilled {
// set stack top sub sm: SwapMap
}
}
//==> ColorTableMap::FallenOver
FallenOver
Entry {
enterFallenOver();
}
Exit {
leaveFallenOver();
}
{
// Expose Transitions to other parts if necessary
fill [ context.getOwner().is_empty() ]
Vacancy {
}
fill
Filled {
fill();
}
}
//==> ColorTableMap::Filled
Filled
Entry {
enterFilled();
}
Exit {
leaveFilled();
}
{
erase [ context.getOwner().can_erase() ]
Unfilled {
erase();
}
erase
Vacancy {
empty();
}
}
//==> ColorTableMap::Unfilled
Unfilled
Entry {
enterUnfilled();
}
Exit {
leaveUnfilled();
}
{
fall
Fallen {
fall();
}
}
//==> ColorTableMap::Fallen
Fallen
Entry {
enterFallen();
}
Exit {
leaveFallen();
}
{
erase [ context.getOwner().can_erase() ]
Unfilled {
erase();
}
erase
FallenOver {
empty();
}
}
%%
3) ColorTable.h和ColorTable.cpp
/**
* ColorTable.h
* 2015-02-01
* cheungmine, all rights reserved.
*
* col->
* +-------+-------+-------+-------+-------+-------+-------+
* row |00 |01 |02 |03 |04 |05 |06 |
* | | | | | | | | |
* v | | | | | | | |
* +-------+-------+-------+-------+-------+-------+-------+
* |10 |11 |12 |13 |14 |15 |16 |
* | | | | | | | |
* | | | | | | | |
* +-------+-------+-------+-------+-------+-------+-------+
* |20 |21 |22 |23 |24 |25 |26 |
* | |(xp,yp)| | | | | | height
* | | . | | | | | |
* +-------+-------+-------+-------+-------+-------+-------+
* |30 |31 |32 |33 |34 |35 |36 |
* | | | | | | | |
* | | | | | | | |
* +-------+-------+-------+-------+-------+-------+-------+
* y |40 |41 |42 |43 |44 |45 |46 |
* ^ | | | | | | | |
* | | | | | | | | |
* | o-------+-------+-------+-------+-------+-------+-------+
* | origin width
* +------------>x
* (0,0)
*
*/
#ifndef __COLORTABLE_H__
#define __COLORTABLE_H__
#include "cocos2d.h"
USING_NS_CC;
#include "ColorTable_sm.h"
class ColorTable : public Node
{
public:
// Global Settings:
static const int ColorTable_NumRows = 5;
static const int ColorTable_NumCols = 7;
static const int ColorTable_NumCells = ColorTable_NumRows*ColorTable_NumCols;
static const int ColorTable_NumColors = 4;
static const int ColorTable_ColorsPal[ColorTable_NumColors+1]; // colors palette
// Construct and Deconstruct
ColorTable() :
swap_done_(false),
_fsm(0),
_DeltaTime(0.1f),
_NumRows(ColorTable_NumCols),
_NumCols(ColorTable_NumRows),
_NumCells(ColorTable_NumCells)
{
}
// Public Types:
enum EnumColor
{
INVALID = 0,
RED = 1,
GREEN = 2,
BLUE = 3,
YELLOW = 4
};
// Public Inline Methods:
inline int index(int row, int col) const
{
return (col*_NumCols + row);
}
inline int color(int row, int col) const
{
return table_[index(row, col)];
}
inline int result(int row, int col) const
{
return results_[index(row, col)];
}
public:
// Public Methods:
CREATE_FUNC(ColorTable);
virtual bool init();
bool is_empty();
bool can_erase();
bool swap_done();
void shuffle();
void empty();
void erase();
void fall();
void fill();
void print();
public:
// Public Callbacks:
void enterVacancy();
void leaveVacancy();
void enterShuffleFilled();
void leaveShuffleFilled();
void enterFallenOver();
void leaveFallenOver();
void enterFilled();
void leaveFilled();
void enterUnfilled();
void leaveUnfilled();
void enterFallen();
void leaveFallen();
// Public Events:
void onIdleVacancy(float dt);
void onIdleShuffleFilled(float dt);
void onIdleFallenOver(float dt);
void onIdleFilled(float dt);
void onIdleUnfilled(float dt);
void onIdleFallen(float dt);
private:
// Private Methods:
void init_table();
void exit();
private:
// Private Members:
ColorTableContext * _fsm;
const int _NumRows;
const int _NumCols;
const int _NumCells;
const float _DeltaTime;
bool swap_done_;
int table_[ColorTable_NumCells];
int results_[ColorTable_NumCells];
};
#endif // __COLORTABLE_H__
/**
* ColorTable.cpp
* 2015-02-01
* cheungmine, all rights reserved.
*
*/
#include "ColorTable.h"
const int ColorTable::ColorTable_ColorsPal[ColorTable_NumColors+1] = {'O', 'R', 'G', 'B', 'Y'};
/// Private Methods
void ColorTable::init_table()
{
int fixed[] = {
1,1,3,1,2,
3,2,1,1,4,
2,2,4,3,3,
1,3,3,4,1,
3,4,4,2,4,
2,2,4,1,1,
2,1,1,2,4
};
int at = 0;
int * p = table_;
while (p - table_ < _NumCells) {
*p++ = fixed[at++];
}
}
/// Public Callbacks ///
void ColorTable::enterVacancy()
{
empty();
this->schedule(schedule_selector(ColorTable::onIdleVacancy), _DeltaTime);
}
void ColorTable::leaveVacancy()
{
exit();
}
void ColorTable::enterShuffleFilled()
{
this->schedule(schedule_selector(ColorTable::onIdleShuffleFilled), _DeltaTime);
}
void ColorTable::leaveShuffleFilled()
{
exit();
}
void ColorTable::enterFallenOver()
{
this->schedule(schedule_selector(ColorTable::onIdleFallenOver), _DeltaTime);
}
void ColorTable::leaveFallenOver()
{
exit();
}
void ColorTable::enterFilled()
{
this->schedule(schedule_selector(ColorTable::onIdleFilled), _DeltaTime);
}
void ColorTable::leaveFilled()
{
exit();
}
void ColorTable::enterUnfilled()
{
this->schedule(schedule_selector(ColorTable::onIdleUnfilled), _DeltaTime);
}
void ColorTable::leaveUnfilled()
{
exit();
}
void ColorTable::enterFallen()
{
this->schedule(schedule_selector(ColorTable::onIdleFallen), _DeltaTime);
}
void ColorTable::leaveFallen()
{
exit();
}
Public Events
void ColorTable::onIdleVacancy(float dt)
{
_fsm->shuffle();
}
void ColorTable::onIdleShuffleFilled(float dt)
{
}
void ColorTable::onIdleFallenOver(float dt)
{
}
void ColorTable::onIdleFilled(float dt)
{
}
void ColorTable::onIdleUnfilled(float dt)
{
}
void ColorTable::onIdleFallen(float dt)
{
}
/// Public Methods
bool ColorTable::init()
{
_fsm = new ColorTableContext(*this);
_fsm->setDebugFlag(true);
_fsm->enterStartState();
return true;
}
void ColorTable::exit()
{
this->unscheduleAllCallbacks();
}
bool ColorTable::is_empty()
{
for (int index = 0; index < _NumCells; ++index) {
if (table_[index] != INVALID) {
return false;
}
}
return true;
}
bool ColorTable::can_erase()
{
return false;
}
bool ColorTable::swap_done()
{
return swap_done_;
}
void ColorTable::shuffle()
{
init_table();
}
void ColorTable::empty()
{
for (int cell = 0; cell < _NumCells; ++cell) {
table_[cell] = INVALID;
results_[cell] = 0;
}
}
void ColorTable::erase()
{
}
void ColorTable::fall()
{
}
void ColorTable::fill()
{
}
void ColorTable::print()
{
#if defined(COCOS2D_DEBUG) && defined(WIN32)
FILE * fp = fopen("c:/temp/color-table.prt", "a+");
if (fp) {
fprintf(fp, "\n>>>> %s {", _fsm->getState().getName());
fprintf(fp, "\n---- TABLE ----\n");
for (int row = 0; row < ColorTable_NumRows; ++row) {
for (int col = 0; col < ColorTable_NumCols; ++col) {
int v = color(row, col);
fprintf(fp, " %c", ColorTable_ColorsPal[v]);
}
fprintf(fp, "\n");
}
fprintf(fp, "\n---- RESULTS ----\n");
for (int row = 0; row < ColorTable_NumRows; ++row) {
for (int col = 0; col < ColorTable_NumCols; ++col) {
int v = result(row, col);
fprintf(fp, " %d", v);
}
fprintf(fp, "\n");
}
fprintf(fp, "}\n");
fclose(fp);
}
#endif
}