uCrop使用
github地址
https://github.com/Yalantis/uCrop
然后clone或下载到本地,运行之。
效果预览
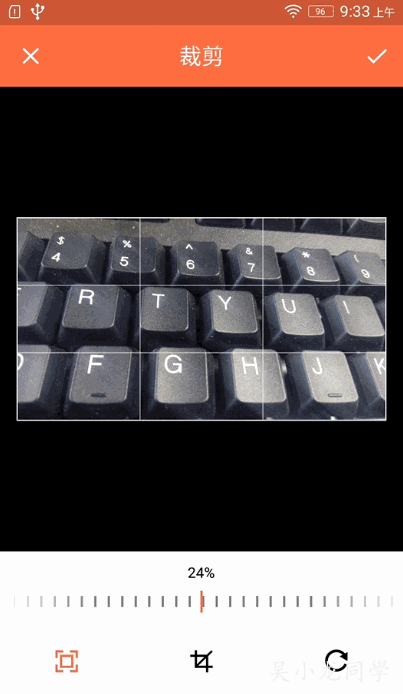
app/build.gradle
1
|
compile 'com.yalantis:ucrop:1.5.0'
|
AndroidManifest.xml
1
2
3
4
|
<activity
android:name="com.yalantis.ucrop.UCropActivity"
android:screenOrientation="portrait"
android:theme="@style/Theme.AppCompat.Light.NoActionBar" />
|
这里theme可以改成自己的
配置uCrop
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
* 启动裁剪
* @param activity 上下文
* @param sourceFilePath 需要裁剪图片的绝对路径
* @param requestCode 比如:UCrop.REQUEST_CROP
* @param aspectRatioX 裁剪图片宽高比
* @param aspectRatioY 裁剪图片宽高比
* @return
*/
public static String startUCrop(Activity activity, String sourceFilePath,
int requestCode, float aspectRatioX, float aspectRatioY) {
Uri sourceUri = Uri.fromFile(new File(sourceFilePath));
File outDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES);
if (!outDir.exists()) {
outDir.mkdirs();
}
File outFile = new File(outDir, System.currentTimeMillis() + ".jpg");
String cameraScalePath = outFile.getAbsolutePath();
Uri destinationUri = Uri.fromFile(outFile);
UCrop uCrop = UCrop.of(sourceUri, destinationUri);
UCrop.Options options = new UCrop.Options();
options.setAllowedGestures(UCropActivity.SCALE, UCropActivity.ROTATE, UCropActivity.ALL);
options.setHideBottomControls(true);
options.setToolbarColor(ActivityCompat.getColor(activity, R.color.colorPrimary));
options.setStatusBarColor(ActivityCompat.getColor(activity, R.color.colorPrimary));
options.setFreeStyleCropEnabled(true);
uCrop.withOptions(options);
uCrop.withAspectRatio(aspectRatioX, aspectRatioY);
uCrop.start(activity, requestCode);
return cameraScalePath;
}
|
其他配置
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
void setToolbarTitle(@Nullable String text)
void setCompressionFormat(@NonNull Bitmap.CompressFormat format)
void setCompressionQuality(@IntRange(from = 0) int compressQuality)
void setMaxScaleMultiplier(@FloatRange(from = 1.0, fromInclusive = false) float maxScaleMultiplier)
void setImageToCropBoundsAnimDuration(@IntRange(from = 100) |