根据不同条件或组合条件检索信息是很常用的需求,下面将在struts2+easyui的datagrid显示列表信息的前提下实现单条件或组合条件检索信息。
一、编辑控制器
二、编辑模型层
1.service
2.emplDaoImpl
伦理片 http://www.dotdy.com/
三、编辑jsp
四、编辑js
大功告成,效果如下:
一、编辑控制器
- //显示员工信息列表
- public void listEmpl() throws IOException{
- //设置响应格式
- resp.setCharacterEncoding("UTF-8");
- //获取当前用户的id
- Empl currEmpl = (Empl) session.getAttribute("emp1");
- int currId = currEmpl.getEid();
- //当前页
- int offset=!"".equals(req.getParameter("page"))&&null!=(req.getParameter("page"))?Integer.parseInt(req.getParameter("page")):1;
- //查询条件:员工名称、职位、部门名称、状态
- Pager<Object[]> pager=empService.search(empl,ajob,adept,ajobStatus,currId,offset);
- //将数据存入list中,它存入的是map,这样在easyui的datagrid才能以键值对的形式获取到具体的数据
- List<Map<String,String>> list = new ArrayList<>();
- Map<String,String> map0 = null;
- for (Object[] e : pager.getList()) {
- map0=new HashMap<String, String>();
- //在datagrid中显示的数据
- map0.put("eid", String.valueOf(e[0]));
- map0.put("ename", String.valueOf(e[1]));
- map0.put("birthday", String.valueOf(e[3]));
- map0.put("hiredate", String.valueOf(e[6]));
- map0.put("jname", String.valueOf(e[5]));//职位
- map0.put("dname", String.valueOf(e[4]));
- map0.put("status", String.valueOf(e[7]));
- //在datagrid中隐藏的数据(部门编号、职位编号、在职状态编号)
- map0.put("did", String.valueOf(e[8]));
- map0.put("jid", String.valueOf(e[9]));
- map0.put("jsid", String.valueOf(e[10]));
- list.add(map0);
- }
- //设置总行数,行数据。
- Map<String,Object> map = new HashMap<String, Object>();
- map.put("total", pager.getSumRows());
- map.put("rows", list);
- //将map转换为json格式
- String s = JSON.toJSONString(map);
- PrintWriter out = resp.getWriter();
- out.println(s);
- }
二、编辑模型层
1.service
- public Pager<Object[]> search(Empl empl,Job job,Dept dept,JobStatus jobStatus,int currId,int offset){
- return emplDaoImpl.search(empl,job,dept,jobStatus,currId,offset);
- }
2.emplDaoImpl
- //分页查询员工信息
- public Pager<Object[]> search(Empl empl,Job job,Dept dept,JobStatus jobStatus,int currId,int offset) {
- List<Object> params=new ArrayList<>();
- StringBuffer sql=new StringBuffer();
- sql.append("SELECT A.*, ROWNUM RN from (select e.eid,e.ename,e.sex,to_char(e.birthday,'yyyy-mm-dd'),d.dname,j.jname,to_char(e.hiredate,'yyyy-mm-dd'),js.status, d.did,j.jid,js.jsid "
- + "from t_empl e,t_dept d,t_job j,t_jobstatus js "
- + "where e.deptno=d.did and e.job=j.jid and e.status=js.jsid and e.eid!="+currId+" and j.jname!='董事长'");
- if(empl!=null){
- if(empl.getEname()!=null&&!"".equals(empl.getEname().trim())){
- sql.append(" and e.ename like ?");
- params.add("%"+empl.getEname()+"%");
- }
- }
- if(job!=null){
- if(job.getJname()!=null&&!"请选择该用户所属职位".equals(job.getJname().trim())){
- sql.append(" and j.jname like ?");
- params.add("%"+job.getJname()+"%");
- }
- }
- if(dept!=null){
- if(dept.getDname()!=null&&!"请选择该用户所属部门".equals(dept.getDname().trim())){
- sql.append(" and d.dname like ?");
- params.add("%"+dept.getDname()+"%");
- }
- }
- if(jobStatus!=null){
- if(jobStatus.getStatus()!=null&&!"请选择该用户所属状态".equals(jobStatus.getStatus().trim())){
- sql.append(" and js.status like ?");
- params.add("%"+jobStatus.getStatus()+"%");
- }
- }
- sql.append(" order by eid) A ");
- return listAsObjectArrayHaveParam(sql.toString(), offset, 10, params.toArray());
- }
伦理片 http://www.dotdy.com/
三、编辑jsp
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
- <!-- 1.页面引入样式 -->
- <link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath }/jquery-easyui-1.4.2/themes/metro/easyui.css">
- <link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath }/jquery-easyui-1.4.2/themes/icon.css">
- <!-- 2.页面引入脚本 -->
- <script type="text/javascript"src="${pageContext.request.contextPath }/jquery-easyui-1.4.2/jquery-1.8.0.min.js"></script>
- <script type="text/javascript"src="${pageContext.request.contextPath }/jquery-easyui-1.4.2/jquery.easyui.min.js"></script>
- <script type="text/javascript"src="${pageContext.request.contextPath }/jquery-easyui-1.4.2/datagrid-detailview.js"></script>
- <script type="text/javascript" src="${pageContext.request.contextPath }/jquery-easyui-1.4.2/locale/easyui-lang-zh_CN.js"></script>
- <!-- 3.页面引入自定义脚本 -->
- <script type="text/javascript" src="${pageContext.request.contextPath }/js/userManager.js" ></script>
- <script type="text/javascript" src="${pageContext.request.contextPath }/js/validate.js" ></script>
- <!-- 4.页面引入其他脚本 -->
- <link href="${pageContext.request.contextPath }/myDatePicker_2.2/datePicker.css" type="text/css" rel="stylesheet" />
- <script type="text/javascript" src="${pageContext.request.contextPath }/myDatePicker_2.2/jquery.datePicker-min.js"></script>
- <title>用户管理</title>
- <style type="text/css">
- .dv-label{
- font-weight: bolder;
- color:#15428B;
- }
- /*datagrid行高设置*/
- .datagrid-btable tr{
- height:30px;
- }
- </style>
- ...
- <form id="searchForm">
- 员工姓名:<input type="text" id="ename" name="empl.ename"/>
- 职位:<select id="job" name="ajob.eid" class="easyui-combobox" panelHeight="auto" >
- <option value="-1">请选择该用户所属职位</option>
- <c:forEach items="${job }" var="v">
- <option value="${v.jid}">${v.jname }</option>
- </c:forEach>
- </select>
- 所属部门:<select id="dept" name="adept.did" class="easyui-combobox" panelHeight="auto" >
- <option value="-1">请选择该用户所属部门</option>
- <c:forEach items="${dept }" var="v">
- <option value="${v.did }">${v.dname }</option>
- </c:forEach>
- </select>
- 员工状态:<select id="jobStatus" name="ajobStatus.jsid" class="easyui-combobox" panelHeight="auto" >
- <option selected value="-1">请选择该用户所属状态</option>
- <c:forEach items="${jobStatus }" var="v">
- <option value="${v.jsid }">${v.status }</option>
- </c:forEach>
- </select>
- <a id="search" href="#" class="easyui-linkbutton" iconCls="icon-ok">筛选</a>
- </form>
- ...
四、编辑js
- //搜索的点击事件
- $('#search').bind('click',function(){
- //重载datagrid.注:1.会向控制器发送请求,请求地址为在datagrid指定的url。2.参数:向控制器发送请求时包含的数据,struts2会将它们封装成相应bean对象。
- $('#dg').datagrid('load',
- {
- 'empl.ename':$('#ename').val(),
- 'ajob.jname':$('#job').combobox('getText'),
- 'adept.dname':$('#dept').combobox('getText'),
- 'ajobStatus.status':$('#jobStatus').combobox('getText')
- }
- );
- //清空、还原搜索表单中的默认选项
- $('#ename').val('')
- $('#job').combobox('setValue','-1')
- $('#dept').combobox('setValue','-1')
- $('#jobStatus').combobox('setValue','-1')
- })
大功告成,效果如下:
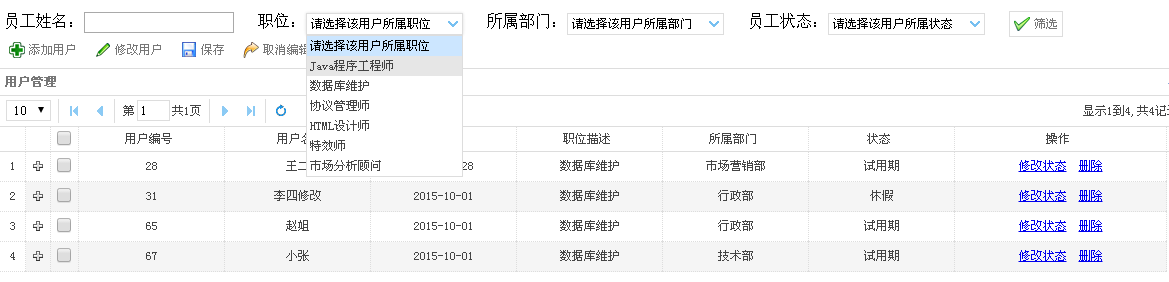