本案例代码下载,在我github上面:https://github.com/hemin1003/dubbo-spring-study
大致上实现代码一样,但有小些不同
要注意一个地方是:
1. 两个项目都要集成spring-boot-starter-web依赖,不然启动不了
2. 暴露的接口服务package路径都要一致,不然会失败
以下为转载内容:
一、使用 spring Initializr 构建 Dubbo 服务消费者 dubbo-consumer 项目
1. 登录 http://start.spring.io/ 填写如下信息后点击 “Generate Project” 按钮,得到 dubbo-consumer 项目骨架
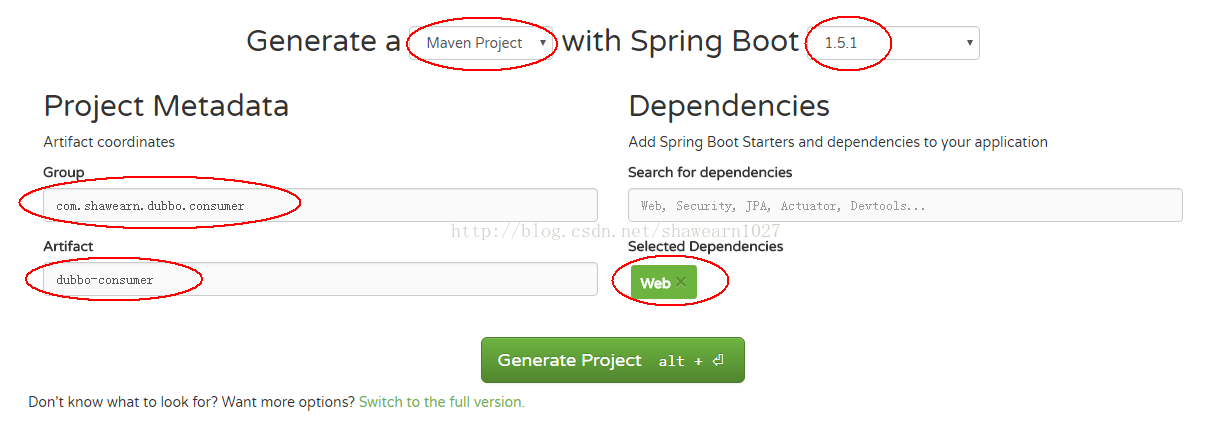
初始 dubbo-provider 项目结构如下:
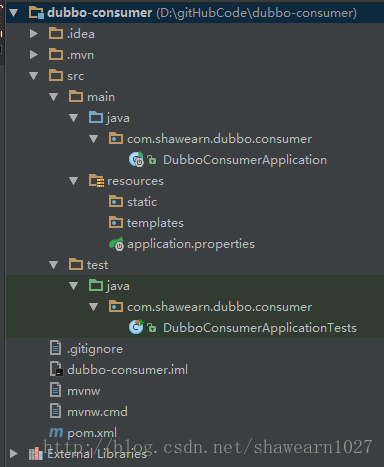
2. 为 dubbo-provider 项目添加依赖:
-
- <dependency>
- <groupId>com.alibaba</groupId>
- <artifactId>fastjson</artifactId>
- <version>1.1.41</version>
- </dependency>
- <dependency>
- <groupId>com.alibaba</groupId>
- <artifactId>dubbo</artifactId>
- <version>2.4.10</version>
- <exclusions>
- <exclusion>
- <artifactId>spring</artifactId>
- <groupId>org.springframework</groupId>
- </exclusion>
- </exclusions>
- </dependency>
- <dependency>
- <groupId>org.apache.zookeeper</groupId>
- <artifactId>zookeeper</artifactId>
- <version>3.4.6</version>
- <exclusions>
- <exclusion>
- <artifactId>slf4j-log4j12</artifactId>
- <groupId>org.slf4j</groupId>
- </exclusion>
- </exclusions>
- </dependency>
- <dependency>
- <groupId>com.github.sgroschupf</groupId>
- <artifactId>zkclient</artifactId>
- <version>0.1</version>
- </dependency>
3. 引入接口(此处偷懒了,没有将接口打成 jar 包后导入,而是直接把接口文件添加到项目下,强烈不建议此种做法)
- package com.shawearn.dubbo.remote;
-
-
-
-
-
- public interface TestService {
- String sayHello(String name);
- }
4. 定义测试用的 Controller 类
- package com.shawearn.dubbo.consumer.controller;
- import com.alibaba.fastjson.JSONObject;
- import com.shawearn.dubbo.remote.TestService;
- import org.springframework.beans.factory.annotation.Autowired;
- import org.springframework.stereotype.Controller;
- import org.springframework.web.bind.annotation.PathVariable;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.ResponseBody;
-
-
-
-
-
- @Controller
- public class TestController {
- @Autowired
- TestService testService;
-
-
-
-
-
-
- @ResponseBody
- @RequestMapping("/test/{name}")
- public JSONObject testJson(@PathVariable("name") String name) {
- JSONObject jsonObject = new JSONObject();
- String testStr = testService.sayHello(name);
- jsonObject.put("str", testStr);
- return jsonObject;
- }
- }
5. 在 resource/ 下添加 consumers
.xml 配置文件,用于向 zookeeper 注册中心注册服务
- <?xml version="1.0" encoding="UTF-8"?>
- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
- xsi:schemaLocation="http://www.springframework.org/schema/beans
- http://www.springframework.org/schema/beans/spring-beans.xsd
- http://code.alibabatech.com/schema/dubbo
- http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
-
-
- <dubbo:application name="dubbo-consumer" owner="dubbo-consumer"/>
-
- <dubbo:registry protocol="zookeeper" address="192.168.10.41:4183" client="zkclient" />
-
- <dubbo:reference id="testService" interface="com.shawearn.dubbo.remote.TestService"/>
- </beans>
6. DubboConsumerApplication 中使用 consumers.xml 配置文件;
- package com.shawearn.dubbo.consumer;
- import org.springframework.boot.SpringApplication;
- import org.springframework.boot.autoconfigure.SpringBootApplication;
- import org.springframework.context.annotation.ImportResource;
- @SpringBootApplication
- @ImportResource(value = {"classpath:consumers.xml"})
- public class DubboConsumerApplication {
- public static void main(String[] args) {
- SpringApplication.run(DubboConsumerApplication.class, args);
- }
- }
7. application.properties 中指定项目启动时占用的端口号:
8. 此时 dubbo-consumer 项目结构如下:
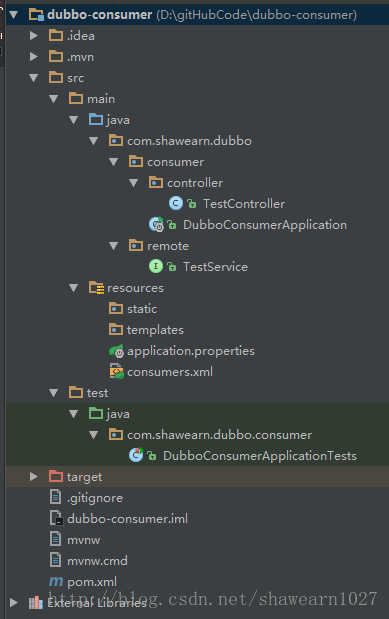
9. 启动 dubbo-consumer 项目,可以通过 Dubbo 服务控制台看到当前项目已经在消费 Dubbo 服务:
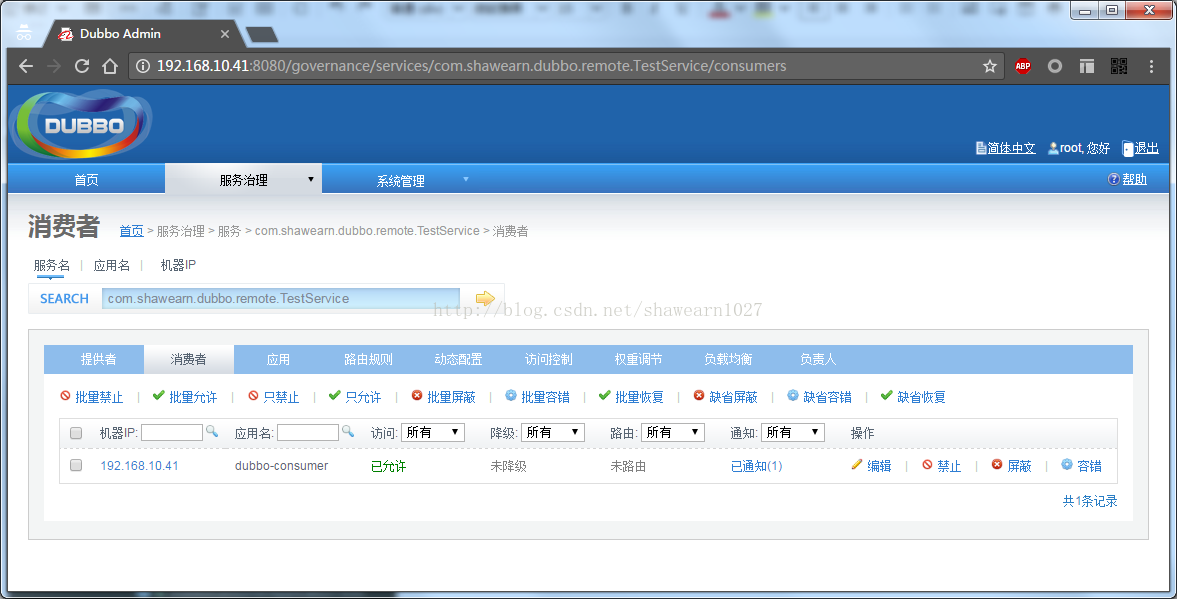
10. 通过浏览器访问 http://192.168.10.41:8012/test/shawearn (此路径为 dubbo-consumer 项目的 WEB 访问路径),可以看到如下页面,证明 dubbo-consumer 已经成功远程调用了 dubbo-provider 项目提供的 Dubbo 服务;
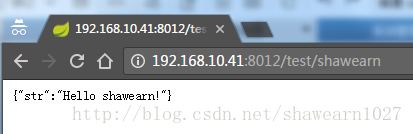
本文示例项目代码可从此地址下载:下载地址