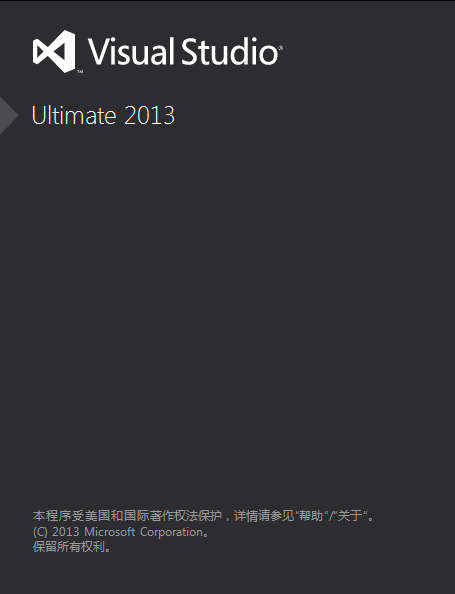
题目
解决代码及点评
/* 链表去重复,只去除相邻重复的元素 如果要去除所有,那么先排序即可 */ #include <iostream> using namespace std; struct student { int id; struct student *next; }; typedef struct student STD; STD *creat(int id) { STD *head; head=new STD; head->id=id; head->next=NULL; return head; } STD *insert(STD *head,int id) { STD *nod; nod=new STD; STD *head2=head; while(head2->next!=NULL) head2=head2->next; head2->next=nod; nod->id=id; nod->next=NULL; return head; } STD *loop(STD *head) { STD *head2=head; while(head2->next!=NULL) head2=head2->next; head2->next=head; return head; } STD *mergeloop(STD *h1,STD *h2,int m,int n)//两循环链表合并,循环短的,插入到HEAD,HEADNEXT之间 { if(m>n) { STD *tail=h2; while(tail->next!=h2) tail=tail->next; tail->next=h1->next; h1->next=h2; } if(m<n) { STD *tail=h1; while(tail->next!=h1) tail=tail->next; tail->next=h2->next; h2->next=h1; } return h1; } STD *merge(STD *h1,STD *h2) { STD *ha1=h1; STD *ha2=h2; while (ha1!=NULL&&ha2!=NULL) { if (ha2->id>ha1->id&&ha2->id<ha1->next->id) //插入一个B链后移 { //STD *h1next=ha1->next; STD *h2next=ha2->next; ha2->next=ha1->next; ha1->next=ha2; ha2=h2next; continue; } if (ha2->id==ha1->id) //相等则越过B中的相等项 { ha2=ha2->next; continue; } ha1=ha1->next; //插不进去则A索引后移 } return h1; } void print(STD *head) { STD *head2=head; cout<<head2->id<<"->"; head2=head2->next; while(head2!=NULL&&head2!=head) { cout<<head2->id<<"->"; head2=head2->next; } } // 去重函数 void drop_chongfu(STD *head) { STD *head2=head; //cout<<head2->id<<"->"; head2=head2->next; while(head2->next!=NULL) { if (head2->id==head2->next->id) { head2->next=head2->next->next; continue; //可能多次重复 } head2=head2->next; } } int main() { STD *h; h=creat(1); h=insert(h,3); h=insert(h,6); h=insert(h,9); h=insert(h,9); h=insert(h,13); h=insert(h,13); h=insert(h,13); h=insert(h,17); h = insert(h, 13); //print(h); drop_chongfu(h); //递增链表删除重复值 print(h); system("pause"); return 0; }
代码下载及其运行
代码下载地址:http://download.csdn.net/detail/yincheng01/6704519
解压密码:c.itcast.cn
下载代码并解压后,用VC2013打开interview.sln,并设置对应的启动项目后,点击运行即可,具体步骤如下:
1)设置启动项目:右键点击解决方案,在弹出菜单中选择“设置启动项目”
2)在下拉框中选择相应项目,项目名和博客编号一致
3)点击“本地Windows调试器”运行
程序运行结果