NHibernate Step by Step (一) Hello,NHibernate!
好了,今天我们正式开始NHibernate的历程,在第一次的练习中,我将尽量详细地讲解环境的配置,以后将不再详细解释。
基本的软件环境如下:
1.NHibernate www.nhibernate.org 当前版本是1.0.2
2.Code Smith http://www.codesmithtools.com/
3.NHibernate模板 点击这里下载
当然,少不了VS2005跟SQLServer了,我这里用的是SQLServer2005,教程用在SQLServer2000上应该没有问题,默认情况下,我将建立并使用一个叫NHibernate的数据库。
首先,我们先建立一个最简单的Person表,如下完整脚本(你可以进行修改以适合自己的数据库):
USE [NHibernate]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[Person](
[id] [
int
] IDENTITY(
1
,
1
) NOT NULL,
[name] [varchar](
50
) COLLATE Chinese_PRC_CI_AS NOT NULL,
CONSTRAINT [PK_Person] PRIMARY KEY CLUSTERED
(
[id] ASC
)WITH (IGNORE_DUP_KEY
=
OFF) ON [PRIMARY]
) ON [PRIMARY]

GO
SET ANSI_PADDING OFF
仅有两个字段,一个自动增长的id,一个name,如下:

然后将下载的nhibernate-template解压,打开Code Smith,将模板加入”Template Explorer”,如下:

然后在其中的NHibernate.cst上点右键,选择“Execute”,弹出设置窗口,在左边的属性窗口进行如下设置:
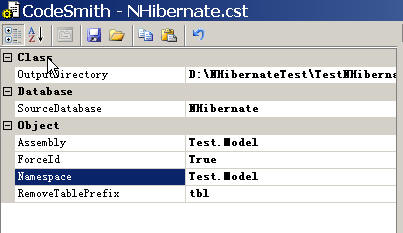
注意:SourceDatabase属性在第一次选择时需要配置一个连接字符串,配置好后Code Smith将记录下来。 Assembly属性代表的是生成文件的默认Assembly名,而NameSpace,顾名思义,就是使用的命名空间了,这里我们全部使用” Test.Model”,请记住这个名字,点击左下角的Generate,将会在指定的输出目录下产生两个文件:Person.cs, Person.hbm.xml。
好了,NHibernate需要的类文件和映射文件生成完了,我们可以开始干活了!(生成NHibernate文件均是如此步骤,以后不再赘述)
新建立一个类库工程,为了简洁起见,我们命名为Model,需要注意的是,为了跟刚才生成的文件对应,我们需要在Model工程的属性页中将起Assembly名字设为上面的“Test.Model”,如下:

然后将刚才生成的两个文件Person.cs和Person.hbm.xml加入到Model工程中来,选中Person.hbm.xml文件,在属性窗口中将其“Build Action”设置为“Embedded Resource”(这是非常重要的一步,否则NHibernate将无法找到映射文件),如下:

build,ok,通过。
然后建立一个控制台工程,命名为Console1,添加NHibernate和上面Model项目的引用,另外添加一个应用程序配置文件,如下:
<?
xml version="1.0" encoding="utf-8"
?>
<
configuration
>
<
configSections
>
<
section
name
="nhibernate"
type
="System.Configuration.NameValueSectionHandler, System,
Version=1.0.5000.0,Culture=neutral, PublicKeyToken=b77a5c561934e089"
/>
</
configSections
>

<
nhibernate
>
<
add
key
="hibernate.connection.provider"
value
="NHibernate.Connection.DriverConnectionProvider"
/>
<
add
key
="hibernate.connection.driver_class"
value
="NHibernate.Driver.SqlClientDriver"
/>
<
add
key
="hibernate.connection.connection_string"
value
="Server=localhost;Initial Catalog=NHibernate;Integrated Security=SSPI"
/>
<
add
key
="hibernate.connection.isolation"
value
="ReadCommitted"
/>
<
add
key
="hibernate.dialect"
value
="NHibernate.Dialect.MsSql2000Dialect"
/>
</
nhibernate
>

</
configuration
>
然后编写如下代码:
using
System;
using
System.Collections.Generic;
using
System.Text;
using
NHibernate;
using
NHibernate.Cfg;
using
Test.Model;

namespace
Console1

{
class Program

{
static void Main(string[] args)

{
Configuration config = new Configuration().AddAssembly("Test.Model");
ISessionFactory factory = config.BuildSessionFactory();
ISession session = factory.OpenSession();

Person person = new Person();
person.Name = "Jackie Chan";

ITransaction trans = session.BeginTransaction();
try

{
session.Save(person);
trans.Commit();
Console.WriteLine("Insert Success!");
}
catch (Exception ex)

{
trans.Rollback();
Console.WriteLine(ex.Message);
}
}
}
}
运行,ok,执行成功!!
我们到数据库检查一下,如下:

我们想要添加的记录已经成功加入到数据库中!!
是不是感觉有些神奇啊?好,我们开始详细解释。
先来看生成的两个文件,第一个是Person.cs,如下:
using
System;
using
System.Collections;

namespace
Test.Model

{

Person#region Person


/**//// <summary>
/// Person object for NHibernate mapped table 'Person'.
/// </summary>
public class Person

{

Member Variables#region Member Variables
protected int _id;
protected string _name;

#endregion


Constructors#region Constructors


public Person()
{ }

public Person( string name )

{
this._name = name;
}

#endregion


Public Properties#region Public Properties

public int Id

{

get
{return _id;}

set
{_id = value;}
}

public string Name

{

get
{ return _name; }
set

{
if ( value != null && value.Length > 50)
throw new ArgumentOutOfRangeException("Invalid value for Name", value, value.ToString());
_name = value;
}
}


#endregion
}
#endregion
}
你可以发现,这完全是一个普通的poco类(Plain Old CLR Object),仅仅是对数据库person表的一个完全映射,不依赖于任何框架,可以用来作为持久化类,你可以在任何地方使用而不用担心依赖于某些神秘的运行时东西。
另外,NHibernate需要知道怎样去加载(load)和存储(store)持久化类的对象。这正是NHibernate映射文件发挥作用的地方。映射文件告诉NHibernate它应该访问数据库(database)里面的哪个表(table)及应该使用表里面的哪些字段(column),这就是我们今天要讲的重点了,Person.hbm.xml,如下:
<?
xml version="1.0" encoding="utf-8"
?>
<
hibernate-mapping
xmlns
="urn:nhibernate-mapping-2.0"
>
<
class
name
="Test.Model.Person, Test.Model"
table
="Person"
>
<
id
name
="Id"
type
="Int32"
unsaved-value
="0"
>
<
column
name
="id"
sql-type
="int"
not-null
="true"
unique
="true"
index
="PK_Person"
/>
<
generator
class
="native"
/>
</
id
>
<
property
name
="Name"
type
="String"
>
<
column
name
="name"
length
="50"
sql-type
="varchar"
not-null
="true"
/>
</
property
>
</
class
>
</
hibernate-mapping
>
不用说,最顶层的hibernate-mapping节点是NHibernate用来进行映射的根了,其中,包含一个class节点,里面的name属性对应我们的Person类,注意,需要完整的限定名;而table属性,则显而易见是对应数据库中的Person表了。
我们再往里面看,分别有两个节点,一个是id,对应数据库中的id,一个是属性name,对应表中的column name和Person类中的name属性,整个映射文件简捷明了,一看即知。实际上这是由代码产生工具产生的映射文件,里面很多东西我们其实可以省略,如下写法:
<property name=”Name” column=”name” />
NHibernate将自动去匹配数据库中的列而不需要我们来设置。
下面,我们来看一下应用程序配置文件中都记录了那些东西,如下:
hibernate.connection.provider_class
定制IConnectionProvider的类型.
例如:full.classname.of.ConnectionProvider (如果提供者创建在NHibernate中), 或者 full.classname.of.ConnectionProvider, assembly (如果使用一个自定义的IConnectionProvider接口的实现,它不属于NHibernate)。
hibernate.connection.driver_class
定制IDriver的类型.
full.classname.of.Driver (如果驱动类创建在NHibernate中), 或者 full.classname.of.Driver, assembly (如果使用一个自定义IDriver接口的实现,它不属于NHibernate)。
hibernate.connection.connection_string
用来获得连接的连接字符串.
hibernate.connection.isolation
设置事务隔离级别. 请检查 System.Data.IsolationLevel 来得到取值的具体意义并且查看数据库文档以确保级别是被支持的。
例如: Chaos, ReadCommitted, ReadUncommitted, RepeatableRead, Serializable, Unspecified
hibernate.dialect
NHibernate方言(Dialect)的类名 - 可以让NHibernate使用某些特定的数据库平台的特性
例如: full.classname.of.Dialect(如果方言创建在NHibernate中), 或者full.classname.of.Dialect, assembly (如果使用一个自定义的方言的实现,它不属于NHibernate)。
接着,我们开始解释代码的执行,如下:
Configuration config
=
new
Configuration().AddAssembly(
"
Test.Model
"
);

//
通过配置对象来产生一个SessionFactory对象,这是一个Session工厂,
//
那么Session是用来干什么的呢?一个Session就是由NHibernate封装
//
的工作单元,我们可以近似地认为它起到ADO.Net中Connection的作用。
ISessionFactory factory
=
config.BuildSessionFactory();
ISession session
=
factory.OpenSession();

Person person
=
new
Person();
person.Name
=
"
Jackie Chan
"
;

//
这里,开启一个由NHibernate封装的事务,当然,在这里最终代表
//
的还是一个真实的数据库事务,但是我们已经不需要再区分到底是
//
一个SqlTransaction还是一个ODBCTransaction了
ITransaction trans
=
session.BeginTransaction();
try

{
//保存,提交,就这么简单!!
session.Save(person);
trans.Commit();
Console.WriteLine("Insert Success!");
}
catch
(Exception ex)

{
trans.Rollback();
Console.WriteLine(ex.Message);
}
现在有了一个基本的概念了吧??
好了,第一篇就讲这么多,我们下次再接着练习。
Step by Step,顾名思义,是一步一步来的意思,整个教程我将贯彻这一理念,待此系列结束后,我们再就某些高级话题进行深入。
任何建议或者批评,请e:abluedog@163.com
评论
#1楼 2006-04-15 13:17 csdn shit! [未注册用户]
写点有新意的吧,拜托,太老套了!!! 回复 引用 查看
#2楼 2006-04-15 13:21 torome
适合新手,不错。 回复 引用 查看
#3楼 2006-04-15 13:22 皇帝的新装
给没有接触过的人看是有必要的。继续努力。 回复 引用 查看
#4楼 2006-04-15 13:25 support [未注册用户]
多谢了,好冬冬 回复 引用 查看
#5楼 2006-04-15 13:26 福娃
写的很详细,期待。。。 回复 引用 查看
#6楼 2006-04-15 13:33 Dflying Chen
提一点建议,代码的格式可以设置得好一点。 回复 引用 查看
#7楼 2006-04-15 14:10 福娃
在net1.1上需要修改两个地方
using System.Collections.Generic;
改为
using System.Collections;
========================================
<section name="nhibernate" type="System.Configuration.NameValueSectionHandler, System,
Version=1.0.5000.0,Culture=neutral, PublicKeyToken=b77a5c561934e089" />
改成
<section name="nhibernate" type="System.Configuration.NameValueSectionHandler, System, Version=1.0.3300.0,Culture=neutral, PublicKeyToken=b77a5c561934e089" />
回复 引用 查看
#8楼 [楼主] 2006-04-15 14:17 abluedog
@福娃
实际上,在我上面的演示代码里其实也应该添加System.Collections引用的,因为目前nhibernate官方还不支持generic,一个query对象返回的还是一个普通的IList。
第3方的nhibernate generic方案请参考:
http://www.ayende.com/projects/nhibernate-query-analyzer/generics.aspx 回复 引用 查看
#9楼 2006-04-15 14:23 剑在上海 [未注册用户]
挺!,之前还没接触过NHibernate,现在有点看懂了,感谢abluedog能花时间写这么好的教程 回复 引用 查看
#10楼 [楼主] 2006-04-15 17:24 abluedog
除了Code Smith外,还有My Generation等代码生成工具,都支持NHibernate文件的生成。
My Generation:http://www.mygenerationsoftware.com/portal/default.aspx
NHibernate Template:
http://www.mygenerationsoftware.com/phpBB2/viewtopic.php?t=1505 回复 引用 查看
#11楼 2006-04-15 22:27 mzl [未注册用户]
thankfulness! 回复 引用 查看
#12楼 2006-04-15 23:55 javac [未注册用户]
感谢abluedog,找了很久都没找到这样的入门级教程,其他教程像参考手册,有点摸不着头脑!
数据库脚本在MS SQL 2000下没有直接运行成功,手动建立后导出脚本如下:
CREATE TABLE [dbo].[Person] (
[id] [int] IDENTITY (1, 1) NOT NULL ,
[name] [varchar] (50) COLLATE Chinese_PRC_CI_AS NULL
) ON [PRIMARY]
GO
ALTER TABLE [dbo].[Person] WITH NOCHECK ADD
CONSTRAINT [PK_Person] PRIMARY KEY CLUSTERED
(
[id]
) ON [PRIMARY]
GO
好像少了ALTER TABLE [dbo].[Person] WITH NOCHECK ADD,不知道是不是2000和2005版本上的差别?
还要感谢福娃指出.net1.1上代码需要修改的地方!
继续期待后面的教程! 回复 引用 查看
#13楼 2006-04-17 10:26 a11s.net
非常适合我,收藏了 回复 引用 查看
#14楼 2006-04-17 22:16 卡卡.net
Nhibernate.org一直不能访问,哪位网友提供NHibernate的下载地址?感谢。 回复 引用 查看
#15楼 2006-05-23 10:34 karlsoft
VS2005和SQLServer2005正版哪里有下﹐哪位有﹐共享一下。謝謝。 回复 引用 查看
#16楼 2006-05-23 10:34 karlsoft
VS2005和SQLServer2005正版哪里有下﹐哪位有﹐共享一下。謝謝。 回复 引用 查看
#17楼 2006-05-25 12:06 karlsoft
使用NHibernate在Web層在按鈕下實現這個﹐不能添加數據﹐請問什么原因﹐是不是配置文件有問題﹐在web.config中已經加了這個
<configSections>
<section name="nhibernate" type="System.Configuration.NameValueSectionHandler, System,Version=1.0.5000.0,Culture=neutral, PublicKeyToken=b77a5c561934e089" />
</configSections>
也加了這個
<nhibernate>
<add key="hibernate.connection.driver_class" value="NHibernate.Driver.SqlClientDriver"/>
<add key="hibernate.connection.provider" value="NHibernate.Connection.DriverConnectionProvider"/>
<add key="hibernate.dialect" value="NHibernate.Dialect.MsSql2000Dialect"/>
<add key="hibernate.connection.connection_string" value="server=MIS03;uid=sa;pwd=;"/>
</nhibernate>
我的代碼碼如下﹕
按鈕下實現如下:
Configuration cfg = new Configuration();
cfg.AddAssembly("GuestBook.Data");
ISessionFactory f = cfg.BuildSessionFactory();
ISession s = f.OpenSession();
ITransaction t = s.BeginTransaction();
Users newUser = new Users();
newUser.Name = "papersnake";
newUser.Password = "123456";
newUser.Email = "you@hotmail.com";
newUser.RegTime = DateTime.Now;
s.Save(newUser);
t.Commit();
s.Close(); 回复 引用 查看
#18楼 2006-06-13 14:43 感谢楼主 [未注册用户]
好东西,很适合入门,谢谢楼主! 回复 引用 查看
#19楼 2006-06-21 09:54 main
应@karlsoft
应该检查下你的版本,1.2.0那个的,感觉和之前的差别蛮大。 回复 引用 查看
#20楼 2006-07-14 17:33 USAF
SQL Server用Code Smith生成Person.cs和Person.hbm.xml;如果MySQL數據庫用什麽工具可以生成呢? 點樣生成? 回复 引用 查看
#21楼 2006-07-15 23:03 零度海洋
推荐一个学习.NET的好站点..
http://www.zero163.com/sortinfo.asp?Classid=45
asp.net2.0 最新最全的资料
各类.net三方控件.. 回复 引用 查看
#22楼 2006-08-24 10:05 小草
很好,正好想了解这方面的,我补充了一下在Oralce数据库的操作,有兴趣可以查看我的blogs : http://www.cnblogs.com/liubiqu/archive/2006/08/24/485016.html 回复 引用 查看
#23楼 2006-08-25 17:54 viptell [未注册用户]
学习了,已经在vs2005 sql2000通过,明天接着下一课,谢谢老是,写的很明白! 回复 引用 查看
#24楼 2006-08-30 16:45 kegogo
感谢abluedog,请教一个问题,有点摸不着头脑!
我照着你的方法去做,却发现老是报错,后经调查我发现原来是,竟然是nhibernate中有一个错误,那就是它要对属性成成的方法要判断它是否是Virtual如下:
在ProxyTypeValidator页下的CheckEveryPublicMemberIsVirtual方法它要检查所有公共的方法是否是Virtual,而检查属性则报错,请问这里为什么要进行这样检查,有什么好的解决方法吗? 回复 引用 查看
#25楼 2006-09-13 14:43 zhongge [未注册用户]
to kegogo
你用的是1.2.0版本的NHibernate.dll
搂住用的是1.0.2的 回复 引用 查看
#26楼 2006-09-13 14:45 zhongge [未注册用户]
辛苦搂住了,希望能跟大家一起探寻Nhibernate的本质的东东:) 回复 引用 查看
#27楼 2006-09-18 11:41 turnright [未注册用户]
我也是遇到和kegogo一样的问题,用1.0.2就ok了,如果用1.2.0应该怎么改呢?
回复 引用 查看
#28楼 2006-10-09 09:35 bluepig [未注册用户]
我刚开始接触NHiberate,楼主文章写道浅简易懂,支持支持! 回复 引用 查看
#29楼 2006-10-09 16:37 anmyan [未注册用户]
运行时出现这个错误是不是配置文件有什么问题啊
D:/AnStudy/Nhibtest/Nhibtest/obj/Debug/CSCA4.tmp Error generating Win32 resource: Error reading icon 'D:/AnStudy/Nhibtest/Nhibtest/App.config' -- データが無効です。
我的配置文件为
<configuration>
<configSections>
<section name="nhibernate"
type="System.Configuration.NameValueSectionHandler, System,
Version=1.0.3300.0,Culture=neutral, PublicKeyToken=b77a5c561934e089" />
</configSections>
<nhibernate>
<add key="hibernate.connection.provider" value="NHibernate.Connection.DriverConnectionProvider" />
<add key="hibernate.connection.isolation" value="ReadCommitted" />
<add key="hibernate.dialect" value="NHibernate.Dialect.MsSql2000Dialect" />
<add key="hibernate.connection.driver_class" value="NHibernate.Driver.SqlClientDriver" />
<add key="hibernate.connection.connection_string" value="server=test;uid=sa;pwd=;"/>
<add key="hibernate.cache.provider_class" value="NHibernate.Caches.SysCache.SysCacheProvider,NHibernate.Caches.SysCache" />
</nhibernate>
</configuration> 回复 引用 查看
#30楼 2006-10-17 15:03 xuexi [未注册用户]
你第三个图的class中outputdriectory 后面的字符串是什么啊?求?代表什么意思啊? 回复 引用 查看
#31楼 2006-10-24 09:09 lwei [未注册用户]
能否详细介绍一下Code Smith ?? 回复 引用 查看
#32楼 2006-10-30 11:30 风吹河岸柳轻扬
很不错,楼主加油 回复 引用 查看
#33楼 2006-11-25 15:40 IT猎人
哦,我知道了,原来是Server=localhost的问题,改为Server=(local)就可以了,另外连接超时的默认值是30秒,所以那里没有马上抛出异常,是我没有耐心等它个30秒,呵呵.
回复 引用 查看
#34楼 2006-11-29 16:36 9527 [未注册用户]
我是新手,为什么我照做的时候报错:
我Win2003,.net2.0 ,vs2005, sql2005,NHibernate.dll(1.0.2.0),CodeSmith4.0.0
报错的位置是:
Configuration config = new Configuration().AddAssembly("Test.Model");
An unhandled exception of type 'System.TypeInitializationException' occurred in Console1.exe
Additional information: The type initializer for 'NHibernate.Cfg.Configuration' threw an exception. 回复 引用 查看
#35楼 2007-01-20 01:02 iafpdael [未注册用户]
<a href="http://wfneoodu.com">pltlgmoi</a> [URL=http://zlofrpjs.com]blbvyacb[/URL] mxpslzuh http://sdlbhnax.com xuiswqlw vuccniwo 回复 引用 查看
#36楼 2007-01-20 03:01 rcgqzrdu [未注册用户]
[URL=http://cryfjhre.com]iwwpuplx[/URL] wjnhsyle http://sgqttdce.com pzurfmeu jbbzfaei <a href="http://mbfjwcqy.com">yfsnhwgw</a> 回复 引用 查看
#37楼 2007-01-21 02:17 pharmacy [未注册用户]
Nothing changes your opinion of a friend so surely as success - yours or his. 回复 引用 查看
#38楼 2007-01-21 04:26 paxil [未注册用户]
If you want creative workers, give them enough time to play. 回复 引用 查看
#39楼 2007-01-21 05:48 ultram [未注册用户]
Fiction is obliged to stick to possibilities. Truth isn't. 回复 引用 查看
#40楼 2007-01-21 07:52 buy cialis online [未注册用户]
Let not the sands of time get in your lunch. 回复 引用 查看
#41楼 2007-01-21 09:03 cheap viagra lathy pretermission [未注册用户]
Sometimes love will pick you up by the short hairs...and jerk the heck out of you. 回复 引用 查看
#42楼 2007-01-21 11:49 paxil [未注册用户]
We play the hands of cards life gives us. And the worst hands can make us the best players. 回复 引用 查看
#43楼 2007-01-21 14:28 nexium [未注册用户]
Under democracy one party always devotes its chief energies to trying to prove that the other party is unfit to rule - and both commonly succeed, and are right. 回复 引用 查看
#44楼 2007-01-21 15:24 hoodia [未注册用户]
We inhereit from our ancestors gifts so often taken for granted... Each of us contains within... this inheritance of soul. We are links between the ages, containing past and present expectations, sacred memories and future promise. 回复 引用 查看
#45楼 2007-01-21 17:53 buy cialis online [未注册用户]
My theology, briefly, is that the universe was dictated but not signed. 回复 引用 查看
#46楼 2007-01-21 20:41 buy adipex [未注册用户]
I am doomed to an eternity of compulsive work. No set goal achieved satisfies. Success only breeds a new goal. The golden apple devoured has seeds. It is endless. 回复 引用 查看
#47楼 2007-01-21 23:24 levitra [未注册用户]
Nothing in the world can take the place of Persistence. Talent will not; nothing is more common than unsuccessful men with talent. Genius will not; unrewarded genius is almost a proverb. Education will not; the world is full of educated derelicts. Persistence and determination alone are omnipotent. The slogan 'Press On' has solved and always will solve the problems of the human race. 回复 引用 查看
#48楼 2007-01-22 00:20 tramadol [未注册用户]
There is an alchemy in sorrow. It can be transmuted into wisdom, which, if it does not bring joy, can yet bring happiness. 回复 引用 查看
#49楼 2007-01-22 00:29 diet [未注册用户]
We succeed only as we identify in life, or in war, or in anything else, a single overriding objective, and make all other considerations bend to that one objective. 回复 引用 查看
#50楼 2007-01-22 04:24 zdnqeghr [未注册用户]
<a href="http://sqszzzjj.com">dlngopps</a> ovxeltdj http://hpsivamv.com qufadbaj mifxasur [URL=http://tavoojdk.com]ubzqpmkz[/URL] 回复 引用 查看
#51楼 2007-01-22 07:00 ghpyyicu [未注册用户]
[URL=http://onjlrjuu.com]yvuvnwjg[/URL] gajlyqdb http://lsngfutm.com rwemxlyf ibeusysg <a href="http://kcvdqmtf.com">dnuuebap</a> 回复 引用 查看
#52楼 2007-01-22 08:33 xgjhkjvf [未注册用户]
wzsmkfns http://krgaoojl.com kwtbzkun qvdmcxum [URL=http://pxyqsdih.com]yncyqoqm[/URL] <a href="http://wycczfvk.com">bjjpskjk</a> 回复 引用 查看
#53楼 2007-01-22 10:34 dozsnceb [未注册用户]
[URL=http://pomghsev.com]rqhertnp[/URL] <a href="http://wcwrdtii.com">bzotjyam</a> nmdzqxud http://wanxabvk.com yfzwuxjf xaznldqi 回复 引用 查看
#54楼 2007-01-22 14:57 diet [未注册用户]
There are several good protections against temptations, but the surest is cowardice. 回复 引用 查看
#55楼 2007-01-22 16:27 buy levitra [未注册用户]
I've been on a diet for two weeks and all I've lost is two weeks. 回复 引用 查看
#56楼 2007-01-22 16:50 wkedfnly [未注册用户]
<a href="http://ubeouopw.com">bowvdwrd</a> [URL=http://idcqzxjg.com]oreanqbj[/URL] savckuyw http://nuztttds.com ejzjotuz rxzcjpjl 回复 引用 查看
#57楼 2007-01-22 20:14 diet [未注册用户]
Life is a great big canvas; throw all the paint on it you can. 回复 引用 查看
#58楼 2007-01-22 20:24 carisoprodol [未注册用户]
Love truth, and pardon error. 回复 引用 查看
#59楼 2007-01-22 23:45 adipex [未注册用户]
No great improvements in the lot of mankind are possible until a great change takes place in the fundamental constitution of their modes of thought. 回复 引用 查看
#60楼 2007-01-23 02:24 zyrtec [未注册用户]
The reason why worry kills more people than work is that more people worry than work. 回复 引用 查看
#61楼 2007-01-23 04:03 zchtgaqi [未注册用户]
[URL=http://zwoslryl.com]duttqprk[/URL] qjtkmyxq http://nfjoiqbe.com xtpslide oxxpxbyq <a href="http://hmjakquo.com">frvujuly</a> 回复 引用 查看
#62楼 2007-01-24 05:19 dilmdlwd [未注册用户]
krscngdw http://oikemwjm.com zdavylxi vpfvkkhm <a href="http://tnuosjar.com">fntfbuxu</a> [URL=http://tayqaxse.com]jxzqjszg[/URL] 回复 引用 查看
#63楼 2007-01-25 02:51 yngyzvpi [未注册用户]
[URL=http://mkkbxrrm.com]ofzzlonv[/URL] <a href="http://oyujxhwt.com">aciisevg</a> salpknkw http://mlpqajws.com ueooeesu hhusixil 回复 引用 查看
#64楼 2007-01-26 04:09 buy tramadol [未注册用户]
Insanity in individuals is something rare - but in groups, parties, nations and epochs, it is the rule. 回复 引用 查看
#65楼 2007-01-26 07:49 vicodin [未注册用户]
Nothing is said that has not been said before. 回复 引用 查看
#66楼 2007-01-26 08:09 ultram [未注册用户]
There is still a difference between something and nothing, but it is purely geometrical and there is nothing behind the geometry. 回复 引用 查看
#67楼 2007-01-26 11:21 fitness [未注册用户]
Nothing is more conducive to peace of mind than not having any opinions at all. 回复 引用 查看
#68楼 2007-01-26 11:35 tramadol online [未注册用户]
Realize that true happiness lies within you. Waste no time and effort searching for peace and contentment and joy in the world outside. Remember that there is no happiness in having or in getting, but only in giving. Reach out. Share. Smile. Hug. Happiness is a perfume you cannot pour on others without getting a few drops on yourself. 回复 引用 查看
#69楼 2007-01-26 15:15 ultram [未注册用户]
Paradise is exactly like where you are right now... only much, much better. 回复 引用 查看
#70楼 2007-01-26 19:31 ambien [未注册用户]
I was gratified to be able to answer promptly. I said I don't know. 回复 引用 查看
#71楼 2007-01-26 23:03 order cialis [未注册用户]
When we got into office, the thing that surprised me the most was that things were as bad as we'd been saying they were. 回复 引用 查看
#72楼 2007-01-29 09:57 hebpjysy [未注册用户]
<a href="http://bkyjkojv.com">mmogwncg</a> cuiqjfuw http://ujwumzap.com wlzzakxu iklsljqy [URL=http://htomefbn.com]yrdzfogu[/URL] 回复 引用 查看
#73楼 2007-01-29 11:51 drug [未注册用户]
Men are equal; it is not birth but virtue that makes the difference. 回复 引用 查看
#74楼 2007-01-29 15:45 buy cialis online [未注册用户]
Normal is not something to aspire to, it's something to get away from. 回复 引用 查看
#75楼 2007-01-29 21:08 buy meridia [未注册用户]
Never make a defense or an apology until you are accused. 回复 引用 查看
#76楼 2007-01-30 00:39 south beach diet [未注册用户]
Never rely on the glory of the morning nor the smiles of your mother-in-law. 回复 引用 查看
#77楼 2007-01-30 04:48 propecia [未注册用户]
You can't wait for inspiration. You have to go after it with a club. 回复 引用 查看
#78楼 2007-01-30 08:35 tramadol [未注册用户]
I say luck is when an opportunity comes along, and you're prepared for it. 回复 引用 查看
#79楼 2007-01-30 11:56 buy soma [未注册用户]
Because we don't think about future generations, they will never forget us. 回复 引用 查看
#80楼 2007-01-30 15:29 vicodin [未注册用户]
To be nobody but yourself in a world which is doing its best day and night to make you like everybody else means to fight the hardest battle which any human being can fight and never stop fighting. 回复 引用 查看
#81楼 2007-01-30 19:02 ambien [未注册用户]
I feel very strongly that change is good because it stirs up the system. 回复 引用 查看
#82楼 2007-01-30 22:35 lipitor [未注册用户]
Be careful that victories do not carry the seed of future defeats. 回复 引用 查看
#83楼 2007-01-31 01:50 cialis online [未注册用户]
Depend not on another, but lean instead on thyself...True happiness is born of self-reliance. 回复 引用 查看
#84楼 2007-01-31 05:16 vitamin [未注册用户]
A boy can learn a lot from a dog: obedience, loyalty, and the importance of turning around three times before lying down. 回复 引用 查看
#85楼 2007-01-31 08:54 celexa [未注册用户]
It is not always the same thing to be a good man and a good citizen. 回复 引用 查看
#86楼 2007-01-31 12:35 ultram [未注册用户]
I have often wished I had time to cultivate modesty... But I am too busy thinking about myself. 回复 引用 查看
#87楼 2007-01-31 16:49 paxil cr [未注册用户]
Someone's boring me. I think it's me. 回复 引用 查看
#88楼 2007-01-31 20:25 xanax [未注册用户]
The great art of giving consists in this: the gift should cost very little and yet be greatly coveted, so that it may be the more highly appreciated. 回复 引用 查看
#89楼 2007-02-01 00:03 nexium [未注册用户]
Refuse to be ill. Never tell people you are ill; never own it to yourself. Illness is one of those things which a man should resist on principle. 回复 引用 查看
#90楼 2007-02-01 03:57 vicodin vicianose curassow [未注册用户]
Happiness lies in the joy of achievement and the thrill of creative effort. 回复 引用 查看
#91楼 2007-02-01 07:44 diabetes [未注册用户]
Late to bed and late to wake will keep you long on money and short on mistakes. 回复 引用 查看
#92楼 2007-02-01 12:07 celebrex [未注册用户]
Nothing in the world can take the place of Persistence. Talent will not; nothing is more common than unsuccessful men with talent. Genius will not; unrewarded genius is almost a proverb. Education will not; the world is full of educated derelicts. Persistence and determination alone are omnipotent. The slogan 'Press On' has solved and always will solve the problems of the human race. 回复 引用 查看
#93楼 2007-02-01 12:08 hydrocodone micropipet haemoglobin [未注册用户]
Summer afternoon - Summer afternoon... the two most beautiful words in the English language. 回复 引用 查看
#94楼 2007-02-01 15:49 pharmacy [未注册用户]
Never let the demands of tomorrow interfere with the pleasures and excitement of today. 回复 引用 查看
#95楼 2007-02-01 20:39 gxonmsgp [未注册用户]
<a href="http://lmjoxhly.com">sptxikpn</a> ialqbrjl http://kbatyvzq.com snbkazcw kkibtkik [URL=http://cwrofzbz.com]fvnapiig[/URL] 回复 引用 查看
#96楼 2007-02-02 07:39 buy valium [未注册用户]
Curiosity killed the cat, but for a while I was a suspect. 回复 引用 查看
#97楼 2007-02-02 11:16 carisoprodol online [未注册用户]
Exercise ferments the humors, casts them into their proper channels, throws off redundancies, and helps nature in those secret distributions, without which the body cannot subsist in its vigor, nor the soul act with cheerfulness. 回复 引用 查看
#98楼 2007-02-02 15:26 vicodin without a prescription [未注册用户]
The supreme irony of life is that hardly anyone gets out of it alive. 回复 引用 查看
#99楼 2007-02-02 19:48 order cialis [未注册用户]
Real love is a permanently self-enlarging experience. 回复 引用 查看
#100楼 2007-02-03 00:47 fvvfuwiv [未注册用户]
gpwrdahu http://usdvblai.com suvwkpop domvtfkz [URL=http://jcshnlix.com]vozljdyb[/URL] <a href="http://fmlhjtqu.com">clejoppf</a> 回复 引用 查看
#101楼 2007-02-03 12:01 prescription diet pills [未注册用户]
Absolute faith corrupts as absolutely as absolute power. 回复 引用 查看
#102楼 2007-02-04 11:02 wuvqmvjx [未注册用户]
vhntgoye http://hqabrlhp.com pwqzoasy ocutxwkn <a href="http://mtrpcsmo.com">hsvbqyyn</a> [URL=http://dtpvyhfp.com]ujvpetrz[/URL] 回复 引用 查看
#103楼 2007-02-04 18:07 levitra buy [未注册用户]
People fail forward to success. 回复 引用 查看
#104楼 2007-02-04 21:19 cheap viagra [未注册用户]
A little government and a little luck are necessary in life, but only a fool trusts either of them. 回复 引用 查看
#105楼 2007-02-05 03:35 lipitor [未注册用户]
The scornful nostril and the high head gather not the odors that lie on the track of truth. 回复 引用 查看
#106楼 2007-02-05 06:57 weight loss [未注册用户]
The Past is to be respected and acknoledged, but not to be worshiped. It is our future in which we will find our greatness. 回复 引用 查看
#107楼 2007-02-05 17:46 kpqjnnsz [未注册用户]
<a href="http://rvmudfdm.com">zboovdyf</a> qlaopujn http://lhwbtlgd.com cbvduwkh gqestdka [URL=http://izyyqeow.com]qlmacylr[/URL] 回复 引用 查看
#108楼 2007-02-06 00:11 vicodin without a prescription [未注册用户]
I don't own a cell phone or a pager. I just hang around everyone I know, all the time. If someone wants to get a hold of me, they just say 'Mitch,' and I say 'what?' and turn my head slightly. 回复 引用 查看
#109楼 2007-02-06 05:10 buy soma [未注册用户]
God creates men, but they choose each other. 回复 引用 查看
#110楼 2007-02-06 09:14 cialis online [未注册用户]
Whatever you do, do it to the purpose; do it thoroughly, not superficially. Go to the bottom of things. Any thing half done, or half known, is in my mind, neither done nor known at all. Nay, worse, for it often misleads. 回复 引用 查看
#111楼 2007-02-06 13:15 drug abirritation reachless [未注册用户]
Life is divided into the horrible and the miserable. 回复 引用 查看
#112楼 2007-02-06 21:41 fioricet online [未注册用户]
Americans are benevolently ignorant about Canada, while Canadians are malevolently well informed about the United States. 回复 引用 查看
#113楼 2007-02-07 01:30 hydrocodone [未注册用户]
Few people think more than two or three times a year; I have made an international reputation for myself by thinking once or twice a week. 回复 引用 查看
#114楼 2007-02-07 01:31 hydrocodone [未注册用户]
Few people think more than two or three times a year; I have made an international reputation for myself by thinking once or twice a week. 回复 引用 查看
#115楼 2007-02-08 13:18 soma [未注册用户]
Censorship, like charity, should begin at home; but, unlike charity, it should end there. 回复 引用 查看
#116楼 2007-02-09 08:20 giuwwgqu [未注册用户]
geixeosv http://lxtdhbox.com gjcwmoab rwnmopaz [URL=http://nybccvlx.com]pzdiwwkz[/URL] <a href="http://rctcmsyw.com">wnsbwebq</a> 回复 引用 查看
#117楼 2007-02-09 09:24 valium [未注册用户]
As I get older, I've learned to listen to people rather than accuse them of things. 回复 引用 查看
#118楼 2007-02-09 13:19 buy carisoprodol [未注册用户]
I like coincidences. They make me wonder about destiny, and whether free will is an illusion or just a matter of perspective. They let me speculate on the idea of some master plan that, from time to time, we're allowed to see out of the corner of our eye. 回复 引用 查看
#119楼 2007-02-09 18:38 hydrocodone [未注册用户]
Sometimes love will pick you up by the short hairs...and jerk the heck out of you. 回复 引用 查看
#120楼 2007-02-09 23:04 buy xanax online [未注册用户]
O, it is excellent to have a giant's strength; but it is tyrannous to use it like a giant. 回复 引用 查看
#121楼 2007-02-10 03:38 diet pill phentermine [未注册用户]
Love is the triumph of imagination over intelligence. 回复 引用 查看
#122楼 2007-02-10 07:03 hydrocodone online [未注册用户]
You know what's interesting about Washington? It's the kind of place where second-guessing has become second nature. 回复 引用 查看
#123楼 2007-02-10 10:41 buy meridia [未注册用户]
Famous remarks are very seldom quoted correctly. 回复 引用 查看
#124楼 2007-02-10 14:31 lipitor [未注册用户]
He will always be a slave who does not know how to live upon a little. 回复 引用 查看
#125楼 2007-02-11 02:41 idsbdivx [未注册用户]
akdxgpvr http://hfhmyusx.com dmvirrig sonqmtyw <a href="http://fsbnnsjz.com">woadgbdi</a> [URL=http://ygbgbozm.com]awfqazph[/URL] 回复 引用 查看
#126楼 2007-03-04 07:31 buy xanax online [未注册用户]
A smiling face is half the meal. 回复 引用 查看
#127楼 2007-03-04 11:30 drug [未注册用户]
There is no stigma attached to recognizing a bad decision in time to install a better one. 回复 引用 查看
#128楼 2007-03-06 14:22 eeykvhdf [未注册用户]
kcpiiiyz http://qtlyuxoq.com nukozzyh bckrypbx <a href="http://jgqxngjz.com">wbywewuv</a> [URL=http://vnzvgkeo.com]nwiuxytl[/URL] 回复 引用 查看
#129楼 2007-03-06 15:43 adipex [未注册用户]
The world tolerates conceit from those who are successful, but not from anybody else. 回复 引用 查看
#130楼 2007-03-06 19:05 buy xanax on line preemptive correct [未注册用户]
It is better to have loved and lost than never to have lost at all. 回复 引用 查看
#131楼 2007-03-18 21:57 ggww [未注册用户]
请问一个问题。我按照你的本个教程,建立控制台工程的时候报错了。就是“然后建立一个控制台工程,命名为Console1,添加NHibernate和上面Model项目的引用,另外添加一个应用程序配置文件”这里的时候vs2005报错了。
我在这个控制台工程中,新建了一个应用程序配置文件(application configuration file)。我拷贝了你的配置文件的内容的时候:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
<section name="nhibernate" type="System.Configuration.NameValueSectionHandler, System,
Version=1.0.5000.0,Culture=neutral, PublicKeyToken=b77a5c561934e089" />
</configSections>
</configuration>
添加到上面的代码,没有问题。
就是添加:
<nhibernate>
<add key="hibernate.connection.provider" value="NHibernate.Connection.DriverConnectionProvider" />
<add key="hibernate.connection.driver_class" value="NHibernate.Driver.SqlClientDriver" />
<add key="hibernate.connection.connection_string" value="Server=localhost;Initial Catalog=NHibernate;Integrated Security=SSPI" />
<add key="hibernate.connection.isolation" value="ReadCommitted"/>
<add key="hibernate.dialect" value="NHibernate.Dialect.MsSql2000Dialect" />
</nhibernate>
的时候vs2005报错:
Message 1 Could not find schema information for the element 'nhibernate'. D:/NHibernate_projects/Model/Console1/Console1/App.config 7 4 Console1
Message 2 Could not find schema information for the element 'add'. D:/NHibernate_projects/Model/Console1/Console1/App.config 8 6 Console1
Message 3 Could not find schema information for the attribute 'key'. D:/NHibernate_projects/Model/Console1/Console1/App.config 8 10 Console1
一共有16个错误。
请问这个问题如何解决??????多谢。 回复 引用 查看
#132楼 2007-03-26 09:13 levitra [未注册用户]
Joy is prayer - Joy is strength - Joy is love - Joy is a net of love by which you can catch souls. 回复 引用 查看
#133楼 2007-03-26 13:14 buy valium online [未注册用户]
Nothing is as certain as that the vices of leisure are gotten rid of by being busy. 回复 引用 查看
#134楼 2007-03-26 17:34 diet pills [未注册用户]
Good habits, which bring our lower passions and appetites under automatic control, leave our natures free to explore the larger experiences of life. 回复 引用 查看
#135楼 2007-03-27 00:38 oxnotoza [未注册用户]
<a href="http://vnjlvdaf.com">lmdbszpb</a> [URL=http://lsthijeq.com]gxfczjmv[/URL] ildbursl http://aeufcpas.com ireppczp jseyxxfb 回复 引用 查看
#136楼 2007-03-28 10:04 cxq [未注册用户]
按照你的步骤做
Configuration config = new Configuration().AddAssembly("Test.Model");
这一句出错
提示: Test.Model.Person.hbm.xml(2,2): XML validation error: 未能找到元素“urn:nhibernate-mapping-2.0:hibernate-mapping”的架构信息。
这是什么原因??
我用的是nhibernate 1.2 的版本 回复 引用 查看
#137楼 2007-03-28 10:08 cxq [未注册用户]
我是新手
请问:
Console1 的应用配置文件 的 文件名 是什么??
这一步能不能说的详细点.
回复 引用 查看
#138楼 2007-03-29 17:36 price viagra [未注册用户]
The average person thinks he isn't. 回复 引用 查看
#139楼 2007-03-31 04:21 propecia [未注册用户]
Nothing inspires forgiveness quite like revenge. 回复 引用 查看
#140楼 2007-03-31 13:21 zb_zbzb@163.com [未注册用户]
按照你的步骤做
Configuration config = new Configuration().AddAssembly("Test.Model");
这一句出错
提示: Test.Model.Person.hbm.xml(2,2): XML validation error: 未能找到元素“urn:nhibernate-mapping-2.0:hibernate-mapping”的架构信息。
解决方法:修改Test.Model.Person.hbm.xml中“urn:nhibernate-mapping-2.0”为“urn:nhibernate-mapping-2.2”
修改Test.Model.Person.cs为:
public virtual int Id
{
get {return _id;}
set {_id = value;}
}
public virtual string Name
{
get { return _name; }
set
{
if ( value != null && value.Length > 50)
throw new ArgumentOutOfRangeException("Invalid value for Name", value, value.ToString());
_name = value;
}
} 回复 引用 查看
#141楼 2007-04-20 16:01 steven [未注册用户]
怎么调试不通,第一句话就报错!
未处理 System.TypeInitializationException
Message="“NHibernate.Cfg.Configuration”的类型初始值设定项引发异常。"
Source="NHibernate"
TypeName="NHibernate.Cfg.Configuration"
StackTrace:
在 NHibernate.Cfg.Configuration..ctor()
在 Console1.Program.Main(String[] args) 位置 D:/Console1/Console1/Program.cs:行号 17
在 System.AppDomain.nExecuteAssembly(Assembly assembly, String[] args)
在 System.AppDomain.ExecuteAssembly(String assemblyFile, Evidence assemblySecurity, String[] args)
在 Microsoft.VisualStudio.HostingProcess.HostProc.RunUsersAssembly()
在 System.Threading.ThreadHelper.ThreadStart_Context(Object state)
在 System.Threading.ExecutionContext.Run(ExecutionContext executionContext, ContextCallback callback, Object state)
在 System.Threading.ThreadHelper.ThreadStart()
回复 引用 查看
#142楼 2007-04-20 16:02 steven [未注册用户]
楼主还是把源码发布出来吧!!! 回复 引用 查看
#143楼 2007-05-09 17:16 jyorin [未注册用户]
按你的方法做,前面都没问题,运行时:
Unknown entity class: Test.Model.Person 回复 引用 查看
#144楼 2007-05-09 17:28 jyorin [未注册用户]
OK,问题已解决! 回复 引用 查看
#145楼 2007-06-22 22:41 梅梅 [未注册用户]
这个工程类库在那里建.....
新建立一个类库工程,为了简洁起见,我们命名为Model,需要注意的是,为了跟刚才生成的文件对应,我们需要在Model工程的属性页中将起Assembly名字设为上面的“Test.Model 回复 引用 查看
#146楼 2007-06-28 12:50 路过 [未注册用户]
按照搂住的步骤做下来,调试时总是在Configuration config = new Configuration().AddAssembly("Test.Model");报xml错误。
后来引入了xsd文件才发现,原来用CodeSmith生成的Person.hbm.xml文件中的破折号(-)被替换成了下划线(_),修改过来运行就可以了。
回复 引用 查看
#147楼 [TrackBack] 2007-07-15 13:35 大冰
NHibernateStepbyStep(一)Hello,NHibernate! NHibernateStepbyStep(一)
[引用提示]大冰引用了该文章, 地址: http://www.cnblogs.com/hanbing768/archive/2007/07/15/818677.html 回复 引用 查看
#148楼 [TrackBack] 2007-07-24 17:13 MichaeL
摘要:这几天开始学习.Net下的企业级应用相关的工具和开源项目。以其从中吸取和借鉴好的思想,同时也能够将其应用在项目开发中。今天有幸收集到了几篇有关Nhibernate的教程,特转载到自己的博客中,以免以后再去查找。
[引用提示]MichaeL引用了该文章, 地址: http://www.cnblogs.com/MichaelLu/archive/2007/07/24/829680.html 回复 引用 查看
#149楼 2007-08-20 14:39 king [未注册用户]
请问,是如何解决的 我也遇到了同样的问题@jyorin
回复 引用 查看
#150楼 2007-09-12 16:50 杰客
你好,我也是这个错误。不知如何解决@jyorin
回复 引用 查看
#151楼 2007-10-19 10:51 ant520
csdn shit!
你这种人,只会说,你有本事自己去写,牛B个撒子哟,老套,我还刚开始学了 回复 引用 查看
#152楼 2007-11-23 16:55 iliuda [未注册用户]
强烈支持,十分感谢 回复 引用 查看
#153楼 2007-12-20 15:45 Lim [未注册用户]
一楼傻 B 回复 引用 查看
#154楼 2008-01-07 13:24 cgx898
运行到第二行时出现在错误,提示信息为:NHibernate.InvalidProxyTypeException was unhandled
Message="The following types may not be used as proxies:/nTest.Model.Person: method set_Id should be virtual/nTest.Model.Person: method get_Name should be virtual/nTest.Model.Person: method set_Name should be virtual/nTest.Model.Person: method get_Id should be virtual"
Source="NHibernate"
StackTrace:
在 NHibernate.Cfg.Configuration.Validate()
在 NHibernate.Cfg.Configuration.BuildSessionFactory()
在 Console1.Program.Main(String[] args) 位置 E:/Download/数据持久层ORM/Model/Console1/Console1/Program.cs:行号 16
在 System.AppDomain._nExecuteAssembly(Assembly assembly, String[] args)
在 System.AppDomain.ExecuteAssembly(String assemblyFile, Evidence assemblySecurity, String[] args)
在 Microsoft.VisualStudio.HostingProcess.HostProc.RunUsersAssembly()
在 System.Threading.ThreadHelper.ThreadStart_Context(Object state)
在 System.Threading.ExecutionContext.Run(ExecutionContext executionContext, ContextCallback callback, Object state)
在 System.Threading.ThreadHelper.ThreadStart()
InnerException:
回复 引用 查看
#155楼 2008-03-25 11:27 Enno [未注册用户]
@csdn shit!
NHibernate Step by Step (一) Hello,NHibernate!
这才是第一课呢
新手看,好啊 回复 引用 查看
#156楼 2008-03-26 13:30 老志
看完..
按照140樓 zb_zbzb@163.com 所提的方法修正
運行正常
使用版本 -> NHibernate-1.2.1.GA
請問如何將文章引用到自己的部落格裡
感恩
回复 引用 查看
#157楼 2008-04-14 09:56 tangzhen [未注册用户]
小弟在createQuery时遇到了问题:我的数据库的表是以用户名加前缀来构建的(例如:有用户dy,那表就时dy.USER 表);所以我写了个createQuery(" from dy.USER as a WHERE a.ID=1 "); 这时会报错误: undefined alias or unknown mapping: dy [ from dy.USER as a WHERE a.ID=1 ] ;其它的save等操作正常. 是不是我这样建的表名在createquery时 他的语法检测机制把表名的前缀看做是别名了?