FatMouse's Speed
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 7816 Accepted Submission(s): 3470
Special Judge
Problem Description
FatMouse believes that the fatter a mouse is, the faster it runs. To disprove this, you want to take the data on a collection of mice and put as large a subset of this data as possible into a sequence so that the weights are increasing, but the speeds are decreasing.
Input
Input contains data for a bunch of mice, one mouse per line, terminated by end of file.
The data for a particular mouse will consist of a pair of integers: the first representing its size in grams and the second representing its speed in centimeters per second. Both integers are between 1 and 10000. The data in each test case will contain information for at most 1000 mice.
Two mice may have the same weight, the same speed, or even the same weight and speed.
The data for a particular mouse will consist of a pair of integers: the first representing its size in grams and the second representing its speed in centimeters per second. Both integers are between 1 and 10000. The data in each test case will contain information for at most 1000 mice.
Two mice may have the same weight, the same speed, or even the same weight and speed.
Output
Your program should output a sequence of lines of data; the first line should contain a number n; the remaining n lines should each contain a single positive integer (each one representing a mouse). If these n integers are m[1], m[2],..., m[n] then it must be the case that
W[m[1]] < W[m[2]] < ... < W[m[n]]
and
S[m[1]] > S[m[2]] > ... > S[m[n]]
In order for the answer to be correct, n should be as large as possible.
All inequalities are strict: weights must be strictly increasing, and speeds must be strictly decreasing. There may be many correct outputs for a given input, your program only needs to find one.
W[m[1]] < W[m[2]] < ... < W[m[n]]
and
S[m[1]] > S[m[2]] > ... > S[m[n]]
In order for the answer to be correct, n should be as large as possible.
All inequalities are strict: weights must be strictly increasing, and speeds must be strictly decreasing. There may be many correct outputs for a given input, your program only needs to find one.
Sample Input
6008 1300 6000 2100 500 2000 1000 4000 1100 3000 6000 2000 8000 1400 6000 1200 2000 1900
Sample Output
4 4 5 9 7
hdu 1160
题目大意:求出最长的串使得,串里面任意的i<j,都有w[i]<w[j]&&s[i]>s[j]。
解题思路:我们根据w先排个序然后对s求一个严格递减就可以了,由于n最大为1000,可以采取O(n*n)的算法。然后输出序列记住路径就可以了。详见代码。
题目地址:FatMouse's Speed
AC代码:
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
int index[1005];
int dp[1005];
int ans[1005];
struct node
{
int a;
int b;
int pos;
}nod[1005];
int cmp(node p1,node p2)
{
if(p1.a<p2.a) return 1;
//if(p1.b>p2.b) return 1;
return 0;
}
void debug()
{
int i;
cout<<"******"<<endl;
for(i=0;i<9;i++)
cout<<nod[i].a<<" "<<nod[i].b<<" "<<nod[i].pos<<endl;
cout<<"#######"<<endl;
}
int main()
{
int n=0;
int p1,p2;
int i,j;
while(cin>>p1>>p2)
{
nod[n].a=p1;
nod[n].b=p2;
nod[n].pos=n+1;
n++;
}
sort(nod,nod+n,cmp);
//memset(dp,1,sizeof(dp));
//debug();
for(i=0;i<=1000;i++)
{
dp[i]=1;
index[i]=0;
}
int tmp,ma=1;
for(i=0;i<n;i++)
for(j=i+1;j<n;j++)
{
if(nod[i].a<nod[j].a&&nod[i].b>nod[j].b)
{
if(dp[i]+1>dp[j])
{
dp[j]=dp[i]+1;
if(dp[j]>ma)
{
ma=dp[j];
tmp=j;
}
index[j]=i;
}
}
}
//cout<<ma<<endl;
int k=0;
while(tmp)
{
ans[k++]=nod[tmp].pos;
tmp=index[tmp];
}
cout<<k<<endl;
for(i=k-1;i>=0;i--)
cout<<ans[i]<<endl;
return 0;
}
/*
6008 1300
6000 2100
500 2000
1000 4000
1100 3000
6000 2000
8000 1400
6000 1200
2000 1900
*/
Constructing Roads In JGShining's Kingdom
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 13635 Accepted Submission(s): 3877
Problem Description
JGShining's kingdom consists of 2n(n is no more than 500,000) small cities which are located in two parallel lines.
Half of these cities are rich in resource (we call them rich cities) while the others are short of resource (we call them poor cities). Each poor city is short of exactly one kind of resource and also each rich city is rich in exactly one kind of resource. You may assume no two poor cities are short of one same kind of resource and no two rich cities are rich in one same kind of resource.
With the development of industry, poor cities wanna import resource from rich ones. The roads existed are so small that they're unable to ensure the heavy trucks, so new roads should be built. The poor cities strongly BS each other, so are the rich ones. Poor cities don't wanna build a road with other poor ones, and rich ones also can't abide sharing an end of road with other rich ones. Because of economic benefit, any rich city will be willing to export resource to any poor one.
Rich citis marked from 1 to n are located in Line I and poor ones marked from 1 to n are located in Line II.
The location of Rich City 1 is on the left of all other cities, Rich City 2 is on the left of all other cities excluding Rich City 1, Rich City 3 is on the right of Rich City 1 and Rich City 2 but on the left of all other cities ... And so as the poor ones.
But as you know, two crossed roads may cause a lot of traffic accident so JGShining has established a law to forbid constructing crossed roads.
For example, the roads in Figure I are forbidden.
In order to build as many roads as possible, the young and handsome king of the kingdom - JGShining needs your help, please help him. ^_^
Half of these cities are rich in resource (we call them rich cities) while the others are short of resource (we call them poor cities). Each poor city is short of exactly one kind of resource and also each rich city is rich in exactly one kind of resource. You may assume no two poor cities are short of one same kind of resource and no two rich cities are rich in one same kind of resource.
With the development of industry, poor cities wanna import resource from rich ones. The roads existed are so small that they're unable to ensure the heavy trucks, so new roads should be built. The poor cities strongly BS each other, so are the rich ones. Poor cities don't wanna build a road with other poor ones, and rich ones also can't abide sharing an end of road with other rich ones. Because of economic benefit, any rich city will be willing to export resource to any poor one.
Rich citis marked from 1 to n are located in Line I and poor ones marked from 1 to n are located in Line II.
The location of Rich City 1 is on the left of all other cities, Rich City 2 is on the left of all other cities excluding Rich City 1, Rich City 3 is on the right of Rich City 1 and Rich City 2 but on the left of all other cities ... And so as the poor ones.
But as you know, two crossed roads may cause a lot of traffic accident so JGShining has established a law to forbid constructing crossed roads.
For example, the roads in Figure I are forbidden.
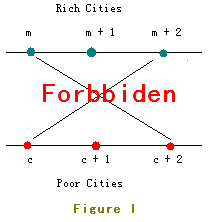
In order to build as many roads as possible, the young and handsome king of the kingdom - JGShining needs your help, please help him. ^_^
Input
Each test case will begin with a line containing an integer n(1 ≤ n ≤ 500,000). Then n lines follow. Each line contains two integers p and r which represents that Poor City p needs to import resources from Rich City r. Process to the end of file.
Output
For each test case, output the result in the form of sample.
You should tell JGShining what's the maximal number of road(s) can be built.
You should tell JGShining what's the maximal number of road(s) can be built.
Sample Input
2 1 2 2 1 3 1 2 2 3 3 1
Sample Output
Case 1: My king, at most 1 road can be built. Case 2: My king, at most 2 roads can be built.HintHuge input, scanf is recommended.
题目大意:找一个序列,使得这个序列的任意一个i<j,都有a[i]<a[j]&&b[i]<b[j]。
解题思路:先对a排个序,然后对b找一个LIS即可。这个题目的n有5*10^5,显然O(n*n)的算法会超时,可以在基础的算法上加上二分查找位置的思想,使得算法复杂度降低为O(n*log(n)),详见代码:
AC代码:
#include<iostream>
#include<cstdio>
#include<algorithm>
using namespace std;
struct node
{
int x;
int y;
}nod[500005];
int ans[500005];
int cmp(node a,node b)
{
return a.x<b.x;
}
int main()
{
int cas=0;
int n,i;
while(cin>>n)
{
for(i=0;i<n;i++)
scanf("%d%d",&nod[i].x,&nod[i].y);
sort(nod,nod+n,cmp);
for(i=1;i<=5e5;i++)
ans[i]=1e6;
for(i=0;i<n;i++)
{
int x=nod[i].y;
int l=0,r=5e5,mid;
while(r>l+1)
{
mid=(l+r)>>1;
if(ans[mid]<x) l=mid;
else r=mid;
}
ans[l+1]=x;
}
int res;
for(i=5e5;i>=1;i--)
if(ans[i]<1e6)
{
res=i;
break;
}
printf("Case %d:\n",++cas);
if(res>1)
printf("My king, at most %d roads can be built.\n\n",res);
else
printf("My king, at most %d road can be built.\n\n",res);
}
return 0;
}
/*
2
1 2
2 1
3
1 2
2 3
3 1
5
2 2
3 3
4 1
1 4
5 5
*/
还有一个题目,也是需要O(n*log(n))的LIS的。
题目地址:Prince and Princess