我们先来看个实验:
#include <iostream>
#include <unordered_map>
#include <map>
#include <vector>
using namespace std;
int main()
{
map<int, int> map;
unordered_map<int, int> hash;
cout << "map[2]=" << map[2] << endl;
cout << "hash[2]=" << hash[2] << endl;
cout << "map.size()=" << map.size() << endl;
cout << "hash.size()=" << hash.size() << endl;
cout << "map[3]=" << map[3] << endl;
cout << "hash[3]=" << hash[3] << endl;
cout << "map.size()=" << map.size() << endl;
cout << "hash.size()=" << hash.size() << endl;
system("pause");
return 0;
}
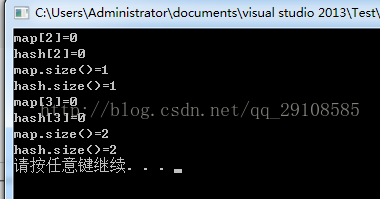
特别注意:先,会在map查找这个键值的项,map如果不包含某个键值,会返回map的end,
然后它发现此键值没有找到(_Where == this->end())的话,
会自动在末尾插入(this->insert(_Where)一个以你输入的键值和value的默认值(mapped_type())构成的对儿(pair),
然后返回这个插入项的值(second,键是first)。而int的默认构造函数int(),就是0。
也就是时候,哪怕你没有对map进行插入操作,哪怕只是用[]判断了下返回值是否是0,map对象也会自动添加一项。
不过一般判断map是否包含一个键,是用map的find方法,判断find的返回结果是否是map的end。
题目描述
现在我们需要查出一些作弊的问答社区中的ID,作弊有两种:1.A回答了B的问题,同时B回答了A的问题。那么A和B都是作弊。2.作弊ID用户A和作弊ID用户B同时回答了C的问题,那么C也是作弊。已知每个用户的ID是一串数字,一个问题可能有多个人回答。
输入描述:
每组数据第一行为总问题数N(N小于等于200000),第二行开始每行一个问题,第一个数字为提问人ID,第二个数字为回答人数,后面则为所有回答人的ID。(ID均为0-1000000的整数)
输出描述:
第一行为作弊ID数量,第二行开始为从小到大的每行一个作弊ID。
输入例子:
3
1 1 2
2 1 1
3 2 1 2
输出例子:
3
1 2 3
超时解法:
#include <iostream>
#include <unordered_map>
#include <map>
using namespace std;
/*
首先,会在map查找这个键值的项,map如果不包含某个键值,会返回map的end,
然后它发现此键值没有找到(_Where == this->end())的话,
会自动在末尾插入(this->insert(_Where)一个以你输入的键值和value的默认值(mapped_type())构成的对儿(pair),
然后返回这个插入项的值(second,键是first)。而int的默认构造函数int(),就是0。
也就是时候,哪怕你没有对map进行插入操作,哪怕只是用[]判断了下返回值是否是0,map对象也会自动添加一项。
不过一般判断map是否包含一个键,是用map的find方法,判断find的返回结果是否是map的end。
*/
int main()
{
int N;
while (cin >> N){
map<int, int> cheat;
unordered_map<int, unordered_map<int, int>> hash;
for (int i = 0; i < N; ++i)
{
int ID, problems;
cin >> ID >> problems;
for (int k = 0; k < problems; ++k)
{
int ansID; cin >> ansID;
if (ansID == ID) continue;
if (hash.find(ansID) != hash.end()){
if (hash[ansID].find(ID) != hash[ansID].end()){//if(hash[ansID][ID]==1
cheat[ansID] = ID;
cheat[ID] = ansID;
}
}
hash[ID][ansID] = 1;
}
}
unordered_map<int, unordered_map<int, int>>::iterator it_all=hash.begin();
for (; it_all!=hash.end(); ++it_all)
{
int ID=it_all->first;
unordered_map<int, int>::iterator it = hash[ID].begin();
for (; it != hash[ID].end(); ++it)
{
if (cheat.find(it->first) == cheat.end())
continue;
if (hash[ID].find(cheat[it->first]) != hash[ID].end()){//if(hash[ID][cheat[it->first]]==1)
cheat[ID] = -1;
break;
}
}
}
cout << cheat.size() << endl;
map<int, int>::iterator chit = cheat.begin();
int cnt = 0;
for (;cnt<cheat.size() - 1; ++cnt,++chit)
{
cout << chit->first <<" ";//千万别把自己坑死,最后一个不能输出一个多余的空格
}
cout << chit->first<<endl;//千万别把自己坑死,最后一个要输出换行
}
return 0;
}
正确解法:
#include <iostream>
using namespace std;
#define M 1030
int main()
{
int N;
while(cin>>N){
int ans[M][M]={0};
int cheat[M]={0};
for (int i = 0; i < N; ++i)
{
int ID,ansNum;cin>>ID>>ansNum;
for (int j = 0; j < ansNum; ++j)
{
int answerID;cin>>answerID;
if(answerID!=ID){
ans[ID][answerID]=1;
}
}
}
for (int i = 0; i < M; ++i)
{
//第一种作弊方法
for (int j = 0; j < M; ++j)
{
if(ans[i][j]==1&&ans[j][i]==1){
cheat[i]=1;
cheat[j]=1;
}
}
//第二种作弊方法
if(cheat[i]==0){
int c=0;
for (int j = 0; j < M; ++j)
{
if(cheat[j]==1&&ans[i][j]==1){
++c;
if(c>=2){
cheat[i]=1;
break;
}
}
}
}
}
int countall=0;
for (int i = 0; i < M; ++i)
{
if(cheat[i]==1){
++countall;
}
}
cout<<countall<<endl;
int k=0;
for (int i = 0; i < M; ++i)
{
if(cheat[i]==1){
++k;
if(k<countall)
cout<<i<<" ";
if(k==countall)
cout<<i<<endl;
}
}
}
return 0;
}