用于展现重复性的东西,Listview比较好用,看了别人的自定义Adapter(item是EditText,能够很好地获取到每一个item的EditText值)。又由于在做项目的需要,故特制了一个item包含RadioGroup的Listview的自定义Adapter。
主要功能:
1.将任意数目的单选题(题干和4个选项)展现在界面上;
2.每一题都选择后,提交,可以讲每一题的答案获取到
涉及到两个entity:
public class TopicItem implements Serializable {
private String questionId;
private String question;
private String answerId;
private String userAnswerId;
private List<OptionItem> optionList;
public String getQuestionId() {
return questionId;
}
public void setQuestionId(String questionId) {
this.questionId = questionId;
}
public String getQuestion() {
return question;
}
public void setQuestion(String question) {
this.question = question;
}
public String getAnswerId() {
return answerId;
}
public void setAnswerId(String answerId) {
this.answerId = answerId;
}
public String getUserAnswerId() {
return userAnswerId;
}
public void setUserAnswerId(String userAnswerId) {
this.userAnswerId = userAnswerId;
}
public List<OptionItem> getOptionList() {
return optionList;
}
public void setOptionList(List<OptionItem> optionList) {
this.optionList = optionList;
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
public class OptionItem implements Serializable {
private String answerOption;
private String answer;
public String getAnswerOption() {
return answerOption;
}
public void setAnswerOption(String answerOption) {
this.answerOption = answerOption;
}
public String getAnswer() {
return answer;
}
public void setAnswer(String anwer) {
this.answer = anwer;
}
}
自定义的adapter:
public class TopicAdapter extends BaseAdapter {
private static final String TAG = "TopicAdapter"
String KEY = "list_topic_item"
private LayoutInflater mInflater
private Context context
private List<Map<String, TopicItem>> mData
public TopicAdapter(Context context, List<Map<String, TopicItem>> data) {
mData = data
mInflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE)
this.context = context
init()
}
private void init() {
mData.clear()
}
@Override
public int getCount() {
int count = mData == null ? 0 : mData.size()
return count
}
@Override
public Object getItem(int position) {
return null
}
@Override
public long getItemId(int position) {
//return position
return 0
}
private Integer index = -1
@Override
public View getView(final int position, View convertView, ViewGroup parent) {
Holder holder = null
if( convertView == null ){
convertView = mInflater.inflate(R.layout.topic_item, null)
holder = new Holder()
holder.question = (TextView)convertView.findViewById(R.id.topic_item_question)
holder.option = (RadioGroup) convertView.findViewById(R.id.topic_item_option)
holder.option1 = (RadioButton) convertView.findViewById(R.id.topic_item_option1)
holder.option2 = (RadioButton) convertView.findViewById(R.id.topic_item_option2)
holder.option3 = (RadioButton) convertView.findViewById(R.id.topic_item_option3)
holder.option4 = (RadioButton) convertView.findViewById(R.id.topic_item_option4)
holder.option.setTag(position)
holder.option.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_UP) {
index = (Integer) v.getTag()
}
return false
}
})
class MyOnCheckedChangeListener implements RadioGroup.OnCheckedChangeListener {
public MyOnCheckedChangeListener(Holder holder) {
mHolder = holder
}
private Holder mHolder
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
// TODO Auto-generated method stub
int position = (Integer) mHolder.option.getTag()
TopicItem item = mData.get(position).get(KEY)
int RadioButtonId = mHolder.option.getCheckedRadioButtonId()
group.check(RadioButtonId)
RadioButton rb = (RadioButton)mHolder.option.findViewById(RadioButtonId)
if(RadioButtonId==mHolder.option1.getId()){
item.setUserAnswerId(item.getOptionList().get(0).getAnswerOption().trim())
}else if(RadioButtonId==mHolder.option2.getId()){
item.setUserAnswerId(item.getOptionList().get(1).getAnswerOption().trim())
}else if(RadioButtonId==mHolder.option3.getId()){
item.setUserAnswerId(item.getOptionList().get(2).getAnswerOption().trim())
}else if(RadioButtonId==mHolder.option4.getId()){
item.setUserAnswerId(item.getOptionList().get(3).getAnswerOption().trim())
}
mData.get(position).put(KEY, item)
}
}
holder.option.setOnCheckedChangeListener(new MyOnCheckedChangeListener(holder))
convertView.setTag(holder)
}else{
holder = (Holder)convertView.getTag()
holder.option.setTag(position)
}
TopicItem item = (TopicItem)mData.get(position).get(KEY)
if( item != null ){
holder.question.setText(item.getQuestion())
holder.option1.setText(item.getOptionList().get(0).getAnswer().toString())
holder.option2.setText(item.getOptionList().get(1).getAnswer().toString())
holder.option3.setText(item.getOptionList().get(2).getAnswer().toString())
holder.option4.setText(item.getOptionList().get(3).getAnswer().toString())
if(item.getUserAnswerId().trim().equalsIgnoreCase(item.getOptionList().get(0).getAnswerOption().trim())){
holder.option.check(holder.option1.getId())
}
else if(item.getUserAnswerId().trim().equalsIgnoreCase(item.getOptionList().get(1).getAnswerOption().trim())){
holder.option.check(holder.option2.getId())
}else if(item.getUserAnswerId().trim().equalsIgnoreCase(item.getOptionList().get(2).getAnswerOption().trim())){
holder.option.check(holder.option3.getId())
}else if(item.getUserAnswerId().trim().equalsIgnoreCase(item.getOptionList().get(3).getAnswerOption().trim())){
holder.option.check(holder.option4.getId())
}
}
holder.option.clearFocus()
if (index != -1 && index == position) {
holder.option.requestFocus()
}
return convertView
}
private class Holder{
private TextView question
private RadioGroup option
private RadioButton option1
private RadioButton option2
private RadioButton option3
private RadioButton option4
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
应用步骤:
1.定义一些变量,为后面做准备
public Context context;
private ListView topic_listview;
private Button button_sent;
private BaseAdapter mAdapter;
private List<Map<String, TopicItem>> data = new ArrayList<Map<String, TopicItem>>();
JSONObject data_json;
String KEY = "list_topic_item";
2.初始化题目数据,这里直接本地编了几道题,可以从服务器上获取
private void initData(){
try {
data_json = new JSONObject();
data_json.put("result", "success");
JSONArray array = new JSONArray();
for(int i=0;i<3;i++){
JSONObject object = new JSONObject();
object.put("questionId", "1");
object.put("question", "康熙是乾隆的谁?");
object.put("answerId", "");
object.put("userAnswerId", "3");
JSONArray sarray = new JSONArray();
JSONObject sobject1 = new JSONObject();
sobject1.put("answerOption", "1");
sobject1.put("answer", "儿子");
sarray.put(sobject1);
JSONObject sobject2 = new JSONObject();
sobject2.put("answerOption", "2");
sobject2.put("answer", "爷爷");
sarray.put(sobject2);
JSONObject sobject3 = new JSONObject();
sobject3.put("answerOption", "3");
sobject3.put("answer", "父亲");
sarray.put(sobject3);
JSONObject sobject4 = new JSONObject();
sobject4.put("answerOption", "4");
sobject4.put("answer", "孙子");
sarray.put(sobject4);
object.put("optionList", sarray);
array.put(object);
}
data_json.put("list", array);
} catch (JSONException e) {
e.printStackTrace();
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
3.将Listview与自定义的TopicAdapter关联起来
this.context = this;
topic_listview = (ListView)findViewById(R.id.topic_quest_answer);
mAdapter = new TopicAdapter(context, data);
topic_listview.setAdapter(mAdapter);
4.将Json中的题目数据解析并放入data,更新给Adapter,这样关联的Listview此时才将数据显示出来
private void getData(){
System.out.println(data_json.toString())
data.clear()
if(data_json.optString("result").equals("success")){
if(data_json.optJSONArray("list")!=null){
for(int i=0
JSONObject object = data_json.optJSONArray("list").optJSONObject(i)
TopicItem topic = new TopicItem()
topic.setAnswerId(object.optString("answerId"))
topic.setQuestionId(object.optString("questionId"))
topic.setQuestion(object.optString("question"))
topic.setUserAnswerId(object.optString("userAnswerId"))
List<OptionItem> optionList = new ArrayList<OptionItem>()
for(int j=0
JSONObject object_option = object.optJSONArray("optionList").optJSONObject(j)
OptionItem option = new OptionItem()
option.setAnswerOption(object_option.optString("answerOption"))
option.setAnswer(object_option.optString("answer"))
optionList.add(option)
}
topic.setOptionList(optionList)
Map<String, TopicItem> list_item = new HashMap<String, TopicItem>()
list_item.put(KEY, topic)
data.add(list_item)
}
//提醒Adapter更新数据,紧接着Listview显示新的数据
mAdapter.notifyDataSetChanged()
}
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
5.用户答题,提交题目的答案时,我们可以看到所有的题目及选择的选项:
button_sent = (Button) findViewById(R.id.topic_sent_comment);
button_sent.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
String t="";
for (int i = 0; i < data.size(); i++) {
t = t+"第 "+(i+1)+" 题 ----------->您选择了【选项"
+data.get(i).get(KEY).getUserAnswerId().trim()+"】 \n";
}
Toast.makeText(context, t, Toast.LENGTH_SHORT).show();
}
});
接下来,我们来看具体运行情况:
a. 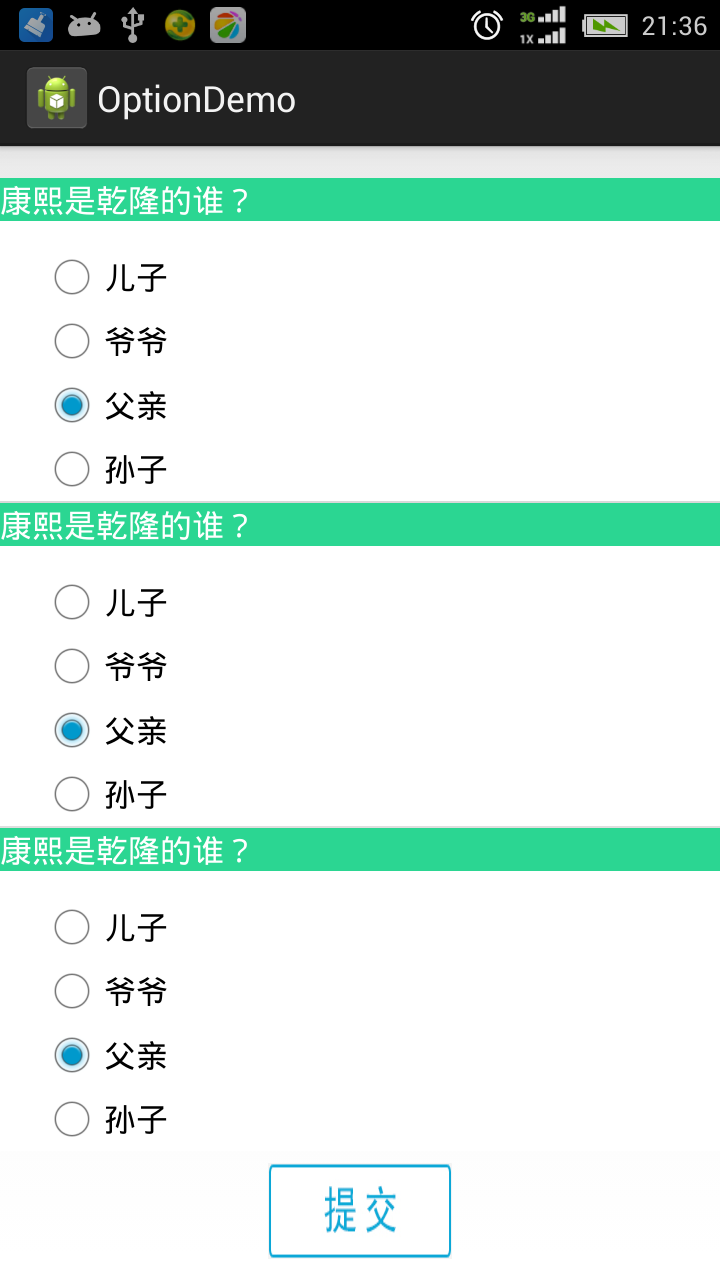
每一题我设选项都是第三项,object.put(“userAnswerId”, “3”);
b.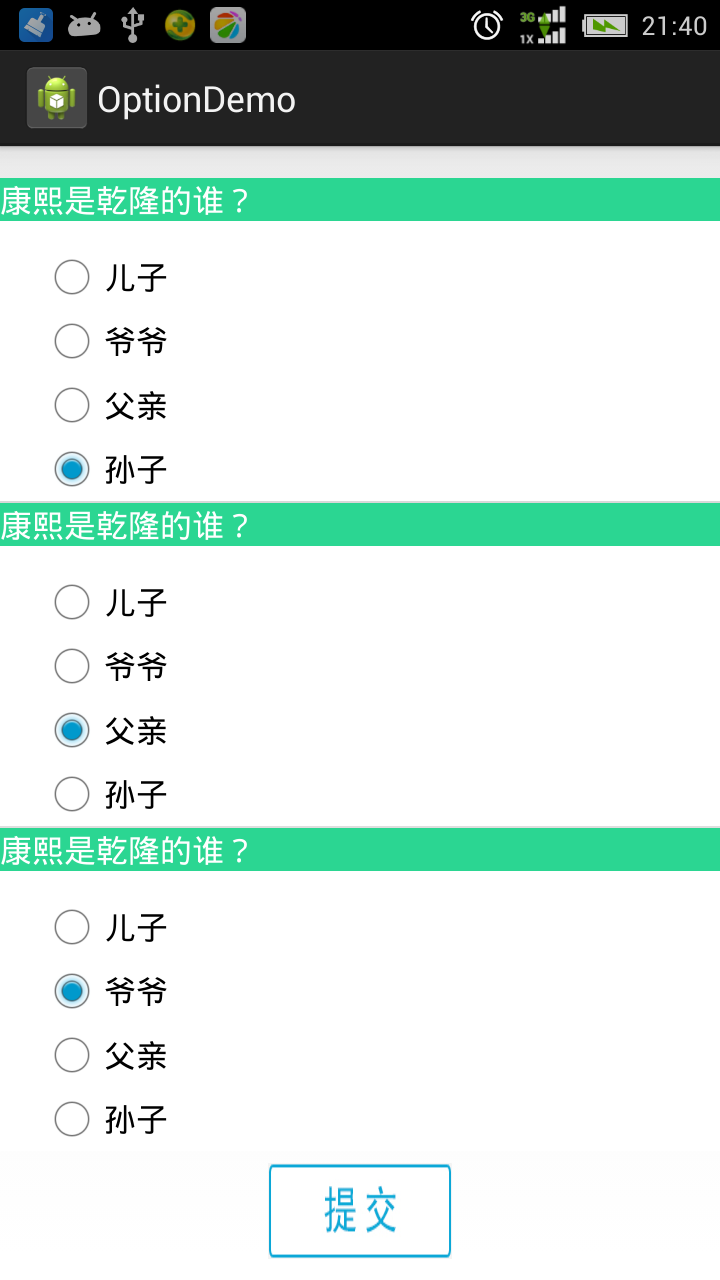
接下来人工选择,分别为4,3,2项
c.提交,可以看到提取结果正确
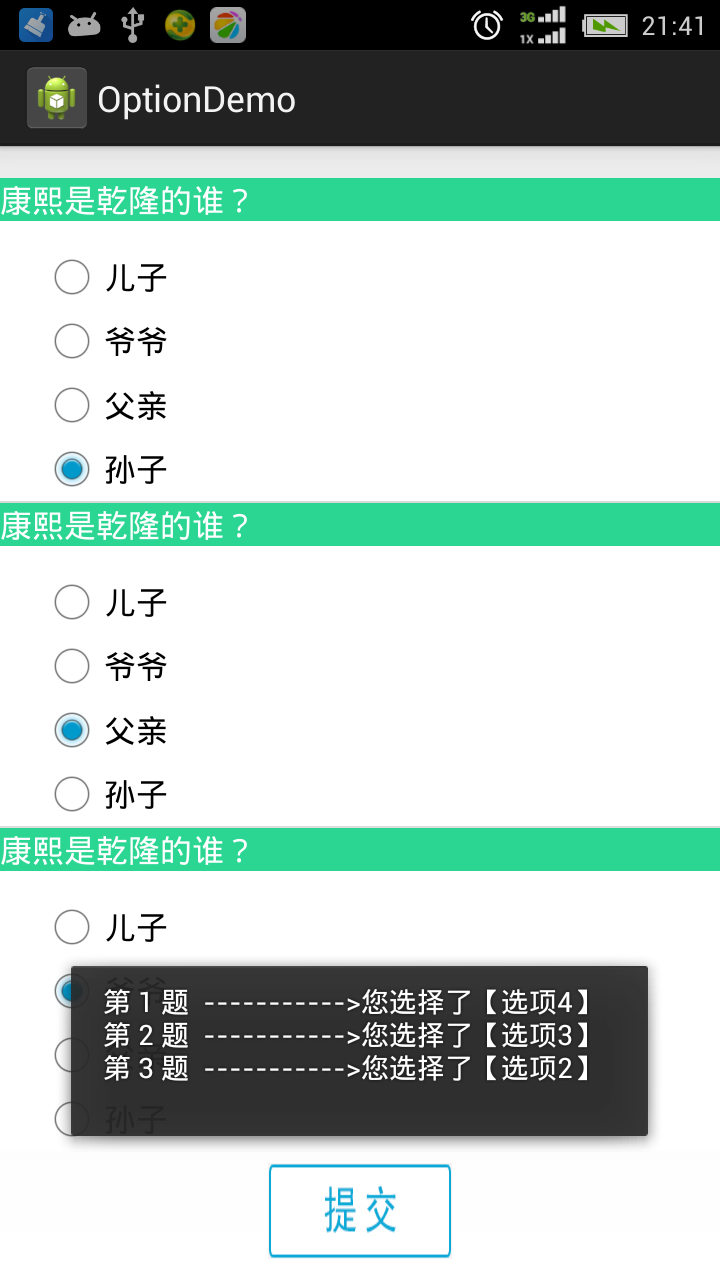