/*
* 程序的版权和版本声明部分
* Copyright (c)2013, 在校学生
* All rightsreserved.
* 文件名称: Snack.cpp
* 作 者:刘旺
* 完成日期:2014年3月26日
* 版本号: v1.0
*
* 输入描述:
* 问题描述:贪吃蛇
* 程序输出:
* 问题分析:
*/
#include <iostream>
#include <vector>
#include <windows.h>
#include <conio.h>
#include <cstdio>
#include <ctime>
using namespace std ;
class Game{
public:
Game():x(0),y(0){}
Game(int x1, int y1):x(x1),y(y1){}
void cheek() ; //边框
void gotoxy() ; //坐标
private:
int x ; //x坐标
int y ; //y坐标
};
//定义边框
void Game::cheek()
{
cout << "╔" ;
cout << "═══════════════════════════════╦══════" ;
cout << "╗" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ 难度: ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ 分数: ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ 排行榜 ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ 1. ║" ;
cout << "║ ║ 2. ║" ;
cout << "║ ║ 3. ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "║ ║ ║" ;
cout << "╚" ;
cout << "═══════════════════════════════╩══════" ;
cout << "╝" ;
}
//定义边框内显示内容的坐标
void Game::gotoxy()
{
HANDLE hout ;
COORD coord ;
coord.X= x ;
coord.Y= y ;
hout=GetStdHandle(STD_OUTPUT_HANDLE) ;
SetConsoleCursorPosition(hout,coord) ;
}
//定义蛇类
class Snack{
public:
Snack *next ; //指向下一个蛇身的指针
Snack(){}
Snack(int x1, int y1):x(x1),y(y1){}
int getX() ; //获取x坐标
int getY() ; //获取y坐标
int getN() ; //获取蛇的长度
void setN(int n1) ; //设置蛇长度
void setX(int x1) ; //设置y坐标
void setY(int y1) ; //设置y坐标
private:
static int n ; //蛇长度
int x ; //x坐标
int y ; //y坐标
};
int Snack::n = 3 ;//初始化舍身
//定义获取x坐标函数
int Snack::getX()
{
return x ;
}
//定义获取y坐标函数
int Snack::getY()
{
return y ;
}
//定义获取蛇身函数
int Snack::getN()
{
return n ;
}
//定义设置x坐标函数
void Snack::setX(int x1)
{
x = x1 ;
}
//定义设置y坐标函数
void Snack::setY(int y1)
{
y = y1 ;
}
//定义设置蛇身长度函数
void Snack::setN(int n1)
{
n = n1 ;
}
//定义操控类
class Console{
public:
Console():head(NULL){}
void snack_Add() ; //控制蛇的生长
void snack_body() ; //控制蛇身
void gotoxy(Snack *p) ; //控制蛇身的坐标
void console() ; //控制输入的按键
void snack_Run(int m) ; //控制蛇身移动
void snack_food() ; //控制蛇的食物出现
~Console() ; //释放动态内存
private:
Snack *head ;//蛇头
Snack *food ;//蛇的食物
};
//定义蛇坐标函数
void Console::snack_food()
{
int x ,y ;
srand(time(NULL)) ;
x = rand()%45+2 ;
y = rand()%15+1 ;
food = new Snack(x,y) ;
}
//定义控制蛇增长函数
void Console::snack_Add()
{
if(head==NULL)
{
Snack *p = new Snack(4,1) ;
Snack *p1 = new Snack(3,1) ;
Snack *p2 = new Snack(2,1) ;
head = p ;
p->next = p1 ;
p1->next = p2 ;
p2->next = NULL ;
}
else
{
int x = food->getX() ;
int y = food->getY() ;
Snack *head1 = new Snack(x,y) ;
head1->next = head ;
int n1 = head->getN()+1 ;
head = head1 ;
head->setN(n1) ;
snack_food() ;
}
}
//定义蛇的运动函数
void Console::snack_Run(int m)
{
Snack *p=head->next ;
Snack *p1 ;
int x1 = head->getX() ;
int y1 = head->getY() ;
int n = p->getN() ;
int j = 2 ;
while(j<n)
{
if((x1==p->getX())&&y1==p->getY())
{
exit(0) ;
}
p = p->next ;
j++;
}
if((head->getX()==food->getX())&&(head->getY()==food->getY()))
{
snack_Add() ;
}
p = head ;
Snack *head1;
head1 = new Snack(0,0) ;
*head1 = *head ;
int x,y ;
x = p->getX() ;
y = p->getY() ;
j = 1 ;
while(j<=n)
{
p = p->next ;
j++ ;
}
switch(m)
{
case 1: head1->setY(y-1) ;break ;
case 2: head1->setY(y+1) ;break ;
case 3: head1->setX(x-1) ;break ;
case 4: head1->setX(x+1) ;break ;
}
head1->next = head ;
head = head1 ;
}
//定义蛇的身体函数
void Console::snack_body()
{
Snack *p = head ;
int n = p->getN() ;
int j = 1 ;
Game m(73,5) ;
m.gotoxy() ;
cout << n-3 ;
while(j<n)
{
if(p==head)
{
gotoxy(p) ;
cout << '@' ;
}
else
{
gotoxy(p) ;
cout << '#' ;
}
p = p->next ;
j++;
}
gotoxy(p) ;
cout << ' ' ;
gotoxy(food) ;
cout << '$' ;
}
//定义蛇身的坐标函数
void Console::gotoxy(Snack *p)
{
int x,y ;
x = p->getX() ;
y = p->getY() ;
HANDLE hout ;
COORD coord ;
if(x>64){exit(0);}
if(y>24){exit(0);}
if(x<1){exit(0);}
if(y<1){exit(0);}
coord.X= x ;
coord.Y= y ;
hout=GetStdHandle(STD_OUTPUT_HANDLE) ;
SetConsoleCursorPosition(hout,coord) ;
}
//定义判断输入键盘的函数
void Console::console()
{
char i ;
char j ;
while(1)
{
i = getch() ;
if((i=='s'&&j=='w')||(i=='w'&&j=='s')||(i=='a'&&j=='d')||(i=='d'&&j=='a'))
{
i = j ;
}
switch(i)
{
case 'w': while(!kbhit()){Sleep(100);snack_Run(1);snack_body();} break ;
case 's': while(!kbhit()){Sleep(100);snack_Run(2);snack_body();} break ;
case 'a': while(!kbhit()){Sleep(100);snack_Run(3);snack_body();} break ;
case 'd': while(!kbhit()){Sleep(100);snack_Run(4);snack_body();} break ;
case 27 : return ;
}
j = i ;
}
}
//定义释放动态内存的函数
Console::~Console()
{
int i=1 ;
int n1 = head->getN() ;
Snack *p =head,*p2 ;
for(i=1; i<=n1; i++)
{
p2 = p ;
p = p->next ;
delete p2 ;
}
}
//主函数
int main()
{
system("color fc");
//system("mode con cols=80 lines=25") ;
system("title 贪吃蛇") ;
Console s ;
Game g ;
g.cheek() ;
s.snack_Add() ;
s.snack_food() ;
s.snack_body() ;
s.console() ;
return 0 ;
}
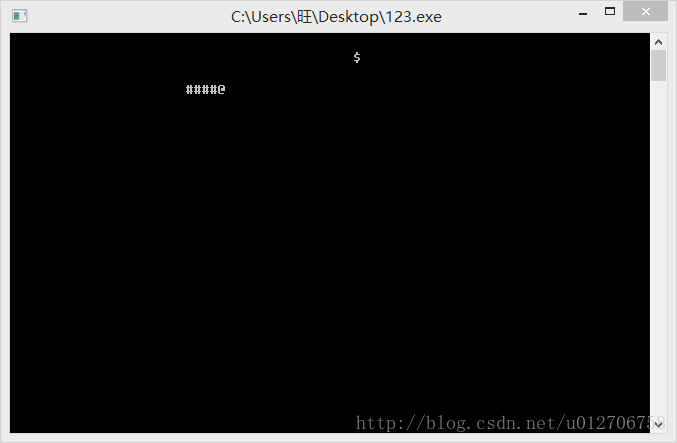
经过这几天自己不断画图,终于把蛇的基本工作做好啦!
________________________________________________
2014.3.26再度更新。嘿嘿,这次修改不少bug,比如蛇吃食物吃着吃着食物没有了,原来是食物的坐标被蛇身覆盖了。这次更新还增加了一些边框颜色,注释,分数等。