项目地址:
ProgressLayout

简介:An extension of RelativeLayout that helps show loading, empty and error layout.
An extension of RelativeLayout that helps show loading, empty and error layout.
Screenshot
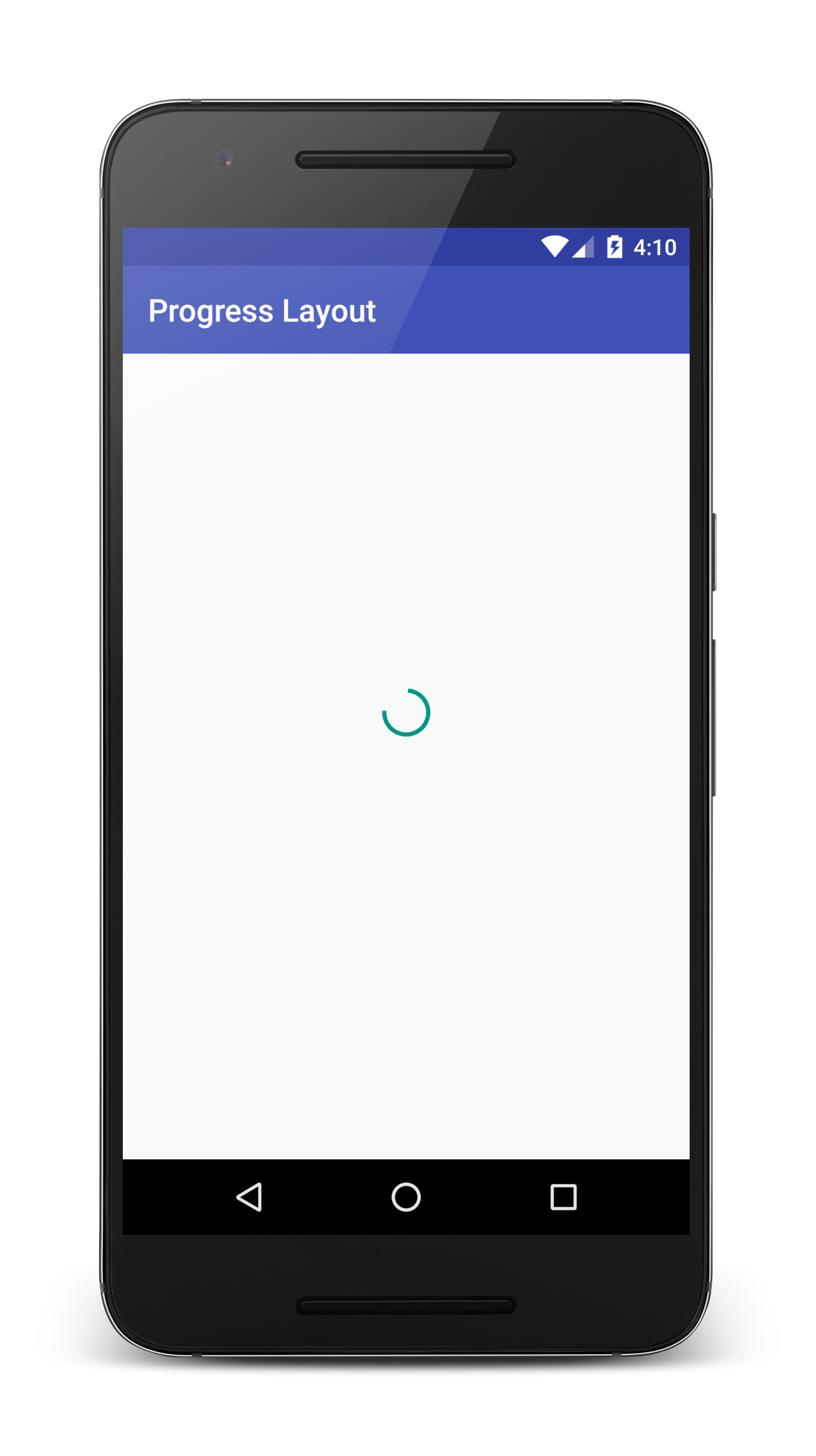
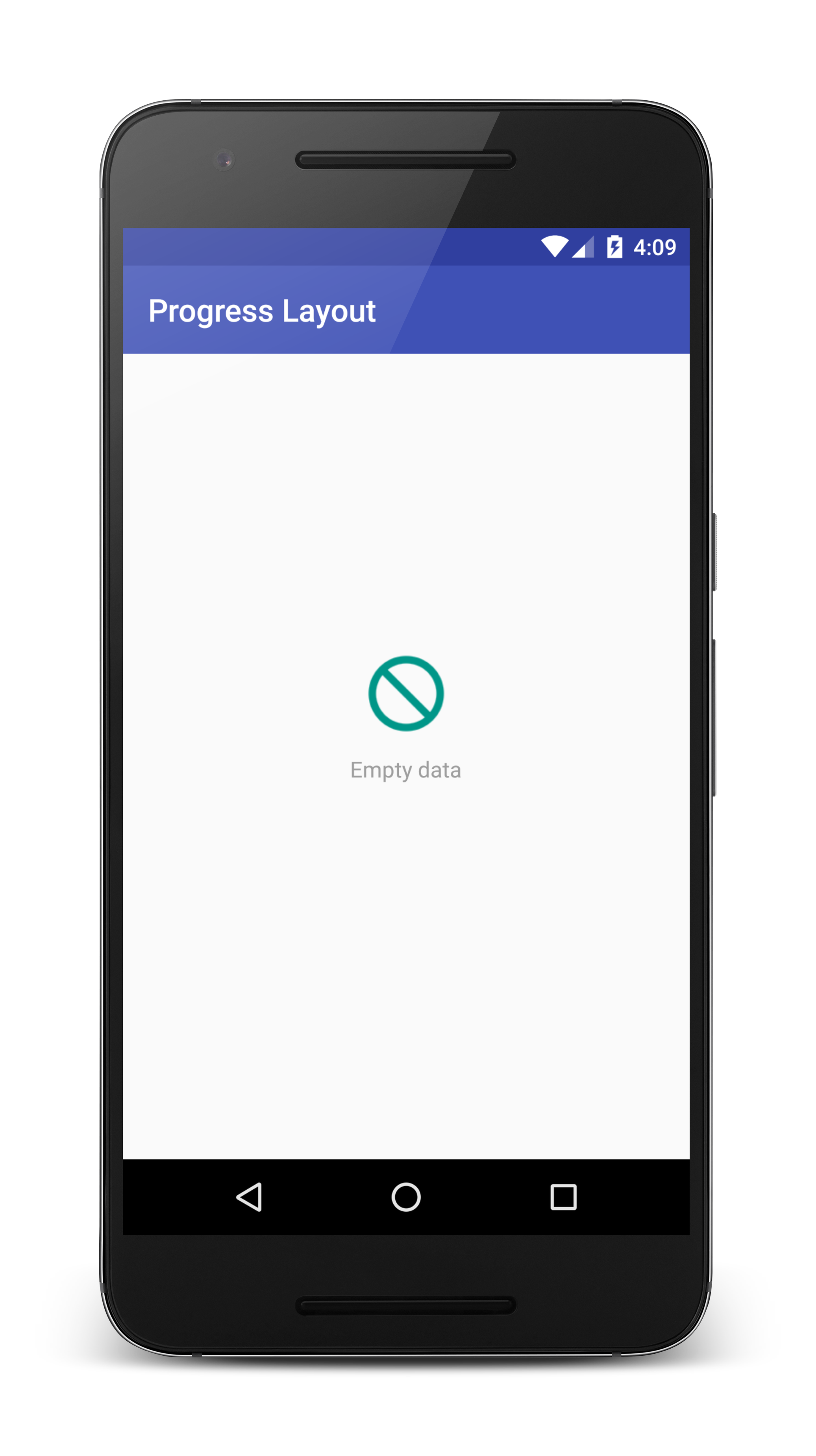
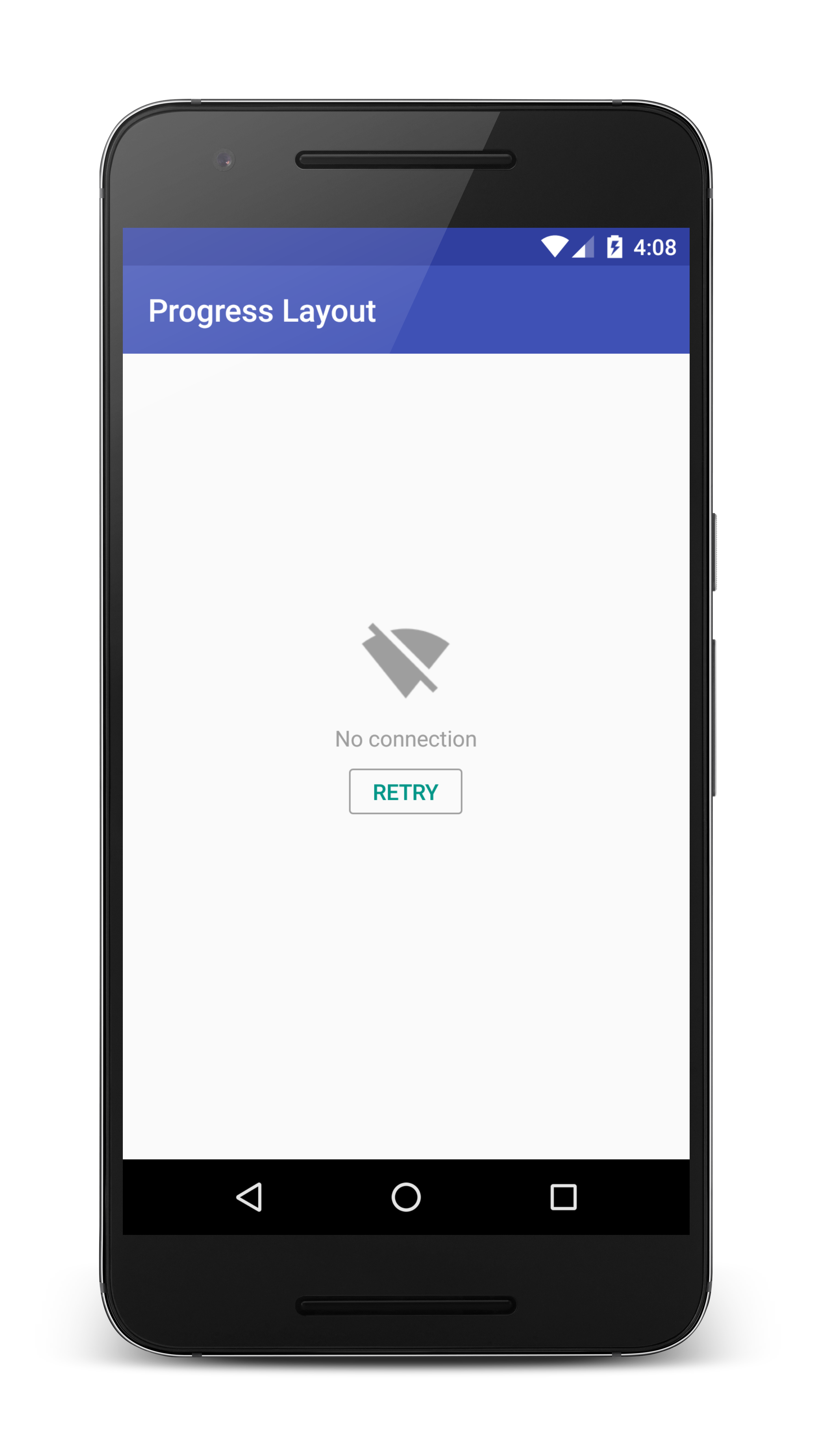
Download
Add to your module's build.gradle:
allprojects {
repositories {
maven { url "https://jitpack.io" }
}
}
and:
dependencies {
compile 'com.github.nguyenhoanglam:ProgressLayout:1.0.0'
}
How to use
-
Use ProgressLayout like RelativeLayout in xml file.
<?xml version="1.0" encoding="utf-8"?> <com.nguyenhoanglam.progresslayout.ProgressLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/progressLayout" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:layout_alignParentTop="true" android:background="@color/colorPrimary" app:theme="@style/CustomToolbarTheme" /> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@id/toolbar" android:layout_centerInParent="true" android:gravity="center" android:text="YOUR CONTENT HERE" android:textSize="24sp" /> </com.nguyenhoanglam.progresslayout.ProgressLayout>
-
Call methods to show loading, empty or error when needed. ```java @Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); toolbar.setTitle("Progress Layout"); ProgressLayout progressLayout = (ProgressLayout) findViewById(R.id.progressLayout); // Show progress layout and keep main views visible // skipIds is a list of view's ids which you want to show with ProgressLayout (in this case is the Toobar) List<Integer> skipIds = new ArrayList<>(); skipIds.add(R.id.toolbar); progressLayout.showLoading(skipIds); progressLayout.showEmpty(ContextCompat.getDrawable(this, R.drawable.ic_empty), "Empty data",skipIds); progressLayout.showError(ContextCompat.getDrawable(this, R.drawable.ic_no_connection), "No connection", "RETRY", new View.OnClickListener() { @Override public void onClick(View view) { Toast.makeText(MainActivity.this, "Reloading...", Toast.LENGTH_SHORT).show(); } },skipIds);
// Show progress layout, hide all main views
progressLayout.showLoading();
progressLayout.showEmpty(ContextCompat.getDrawable(this, R.drawable.ic_empty), "Empty data");
progressLayout.showError(ContextCompat.getDrawable(this, R.drawable.ic_no_connection), "No connection", "RETRY", new View.OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(MainActivity.this, "Reloading...", Toast.LENGTH_SHORT).show();
}
});
}
- Custom ProgressLayout's attributes
```java
<com.nguyenhoanglam.progresslayout.ProgressLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/progressLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:emptyContentTextColor="@color/grey"
app:emptyContentTextSize="14sp"
app:emptyImageHeight="200dp"
app:emptyImageWidth="200dp"
app:errorButtonTextColor="@color/teal"
app:errorButtonTextSize="14sp"
app:errorContentTextColor="@color/grey"
app:errorContentTextSize="14sp"
app:errorImageHeight="200dp"
app:errorImageWidth="200dp"
app:loadingProgressBarColor="@color/teal"
app:loadingProgressBarRadius="100dp"
app:loadingProgressBarSpinWidth="8dp"/>