UVA - 11525
Permutation
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
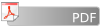
Problem F
Permutation
Input: Standard Input
Output: Standard Output
Given N and K find the N’th permutation of the integers from 1 to K when those permutations are lexicographically ordered. N starts from 0. Since N is very large N will be represented by a sequence of K non-negative integers S1, S2 ,…, Sk. From this sequence of integers N can be calculated with the following expression.
Input
First line of the input contains T(≤10) the number of test cases. Each of these test cases consists of 2 lines. First line contains a integer K(1≤K≤50000). Next line contains K integers S1, S2 ,…, Sk.(0≤Si≤K-i).
Output
For each test case output contains N’th permutation of the integers from 1 to K. These K integers should be separated by a single space.
Sample Input Output for Sample Input
4 3 2 1 0 3 1 0 0 4 2 1 1 0 4 1 2 1 0
| 3 2 1 2 1 3 3 2 4 1 2 4 3 1
|
Problemsetter: Abdullah al Mahmud
Special Thanks: Manzurur Rahman Khan
Source
Root :: Competitive Programming 3: The New Lower Bound of Programming Contests (Steven & Felix Halim) :: More Advanced Topics :: Problem Decomposition :: Two Components - Mixed with Efficient Data Structure
Root :: AOAPC I: Beginning Algorithm Contests -- Training Guide (Rujia Liu) :: Chapter 3. Data Structures :: Maintaining Interval Data :: Exercises: Beginner
Root :: Competitive Programming 2: This increases the lower bound of Programming Contests. Again (Steven & Felix Halim) :: Data Structures and Libraries :: Data Structures with Our-Own Libraries :: Tree-related Data Structures
题意:
给定整数n和k,输出1-k的所有排列中,按照字典序从小到大排序后的第n个(编号0开始)。
由于n通过下面的方式给出
n =
康拓展开式逆运算
线段树版本
#include <cstdio>
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstring>
#include <string>
#include <map>
#include <cmath>
#include <queue>
#include <set>
using namespace std;
//#define WIN
#ifdef WIN
typedef __int64 LL;
#define iform "%I64d"
#define oform "%I64d\n"
#define oform1 "%I64d"
#else
typedef long long LL;
#define iform "%lld"
#define oform "%lld\n"
#define oform1 "%lld"
#endif
#define S64I(a) scanf(iform, &(a))
#define P64I(a) printf(oform, (a))
#define P64I1(a) printf(oform1, (a))
#define REP(i, n) for(int (i)=0; (i)<n; (i)++)
#define REP1(i, n) for(int (i)=1; (i)<=(n); (i)++)
#define FOR(i, s, t) for(int (i)=(s); (i)<=(t); (i)++)
#define lowbit(x) (x&-x)
const int INF = 0x3f3f3f3f;
const double eps = 1e-9;
const double PI = (4.0*atan(1.0));
const int maxn = 50000 + 20;
const int maxo = maxn * 4;
int S[maxn];
int ans[maxn];
int sumv[maxo];
void pushUp(int o) {
sumv[o] = sumv[o<<1] + sumv[o<<1|1];
}
void build(int o, int L, int R) {
if(L == R) {
sumv[o] = 1;
return ;
}
int M = L + (R-L) / 2;
build(o<<1, L, M);
build(o<<1|1, M+1, R);
pushUp(o);
}
int qx, qv;
int update(int o, int L, int R) {
if(L == R) {
sumv[o] = qv;
return L;
}
int M = L + (R-L) / 2;
int ret;
if(sumv[o<<1] >= qx) ret = update(o<<1, L, M);
else {
qx -= sumv[o<<1];
ret = update(o<<1|1, M+1, R);
}
pushUp(o);
return ret;
}
int main() {
int T;
scanf("%d", &T);
while(T--) {
int n;
scanf("%d", &n);
for(int i=0; i<n; i++) scanf("%d", &S[i]);
build(1, 1, n);
for(int i=0; i<n; i++) {
qx = S[i]+1, qv = 0;
ans[i] = update(1, 1, n);
}
for(int i=0; i<n; i++) {
if(i) putchar(' ');
printf("%d", ans[i]);
}
putchar('\n');
}
return 0;
}
树状数组+二分
#include <cstdio>
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstring>
#include <string>
#include <map>
#include <cmath>
#include <queue>
#include <set>
using namespace std;
//#define WIN
#ifdef WIN
typedef __int64 LL;
#define iform "%I64d"
#define oform "%I64d\n"
#define oform1 "%I64d"
#else
typedef long long LL;
#define iform "%lld"
#define oform "%lld\n"
#define oform1 "%lld"
#endif
#define S64I(a) scanf(iform, &(a))
#define P64I(a) printf(oform, (a))
#define P64I1(a) printf(oform1, (a))
#define REP(i, n) for(int (i)=0; (i)<n; (i)++)
#define REP1(i, n) for(int (i)=1; (i)<=(n); (i)++)
#define FOR(i, s, t) for(int (i)=(s); (i)<=(t); (i)++)
#define lowbit(x) (x&-x)
const int INF = 0x3f3f3f3f;
const double eps = 1e-9;
const double PI = (4.0*atan(1.0));
const int maxn = 50000 + 20;
int S[maxn];
int ans[maxn];
int C[maxn];
int vis[maxn];
int n;
void add(int x, int v) {
while(x <= n) {
C[x] += v;
x += lowbit(x);
}
}
int sum(int x) {
int ret = 0;
while(x) {
ret += C[x];
x -= lowbit(x);
}
return ret;
}
int main() {
int T;
scanf("%d", &T);
while(T--) {
scanf("%d", &n);
for(int i=0; i<n; i++) scanf("%d", &S[i]);
memset(C, 0, sizeof(C));
memset(vis, 0, sizeof(vis));
for(int i=1; i<=n; i++) add(i, 1);
for(int i=0; i<n; i++) {
int L = 1, R = n;
int ansi = 1;
while(L <= R) {
int M = L + (R-L) / 2;
int tot = sum(M);
if(tot == S[i]+1 && !vis[M]) {
ansi = M;
break;
}
if(tot < S[i]+1) L = M + 1;
else R = M - 1;
}
vis[ansi] = 1;
add(ansi, -1);
ans[i] = ansi;
}
for(int i=0; i<n; i++) {
if(i) putchar(' ');
printf("%d", ans[i]);
}
putchar('\n');
}
return 0;
}