Audio Player
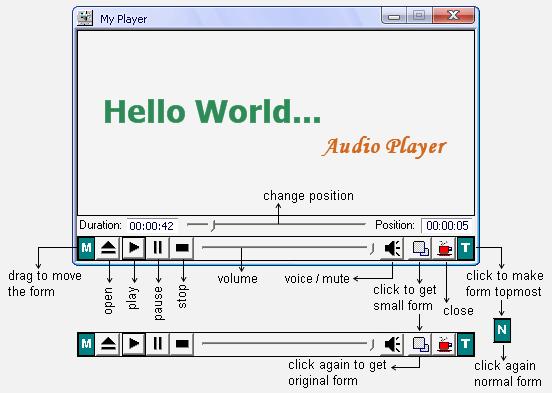
Introduction
I had some trials to create audio player using "Microsoft.DirectX.AudioVideoPlayback
", but I found the mciSendString
function (from Windows API) is better. So, I created a class using mciSendString
functions to play audio files, then compile it to a DLL file. The purpose of this article is not to use my player, but the goal is to answer some questions asked by some readers, so the reader will read about the following methods in this article:
- How to get Random number
- Draw examples: how to draw string and draw line
- Using
FillRectangle
method to draw a point - Using (
SendMessage
) API to move a form (read it in source file) - Using (
SetWindowPos
) API to make form topmost (read it in source file) - How to use the position of audio file as
Timer
(read it in source file)
Background
To use my DLL file, please add MediaControl.dll to your project reference, then declare an instance as follows:
private MediaControl.AudioPlayer MyPlayer = new MediaControl.AudioPlayer();
if your code is under C#, or:
Private MyPlayer As MediaControl.AudioPlayer = new MediaControl.AudioPlayer()
if your code is under VB.NET.
I created two projects, I wrote the code of one under C# (2003) and wrote another under VBNet (2003). The code in this article will be under C#, you can go to VB project and read the code from the source file.
Please extract MyPlayer_C.zip file to read more about the C# code and extract MyPlayer_VB.zip file to read about the VB code.
In the following table, you can read about some commands of the player as examples.
Command | Explanation | Example |
Open | Open music file. (*.mp3, *.ram, *.wav, ...) | MyPlayer.Open(FileName); |
Play | Play music file | MyPlayer.Play(); |
Pause | Stop the song temporary | MyPlayer.Pause = true; |
Close | Stop playing | MyPlayer.Close(); |
Volume | Set and get volume | MyPlayer.Volume = Value; |
Position | Set and get position | MyPlayer.position = Value; |
Duration | Get the duration | label1.Text = MyPlayer.Duration(); |
SetAudioOff | Set voice off | MyPlayer.SetAudioOff(); |
SetAudioOn | Set voice on | MyPlayer.SetAudioOn(); |
You can test this project with the file ..\..\Music\S1.mp3 or any audio file.
Using the Code
Open file dialog to load music file:

private void LoadMusicFile()
{
DialogResult ResultDialog;
FoundIt = false;
try
{
this.OpenDialog.Filter = "Music Formats|" +
"*.mp3;*.ram;*.rm;*.wav;*.wma;*.mid|" +
"mp3 (*.mp3)|*.mp3|ram (*.ram)|*.ram|rm (*.rm)|*.rm|" +
"wav (*.wav)|*.wav|wma (*.wma)|*.wma|mid (*.mid)|*.mid";
this.OpenDialog.RestoreDirectory = true;
this.OpenDialog.FileName = "";
this.OpenDialog.Title = "Choose Music File:";
this.OpenDialog.CheckFileExists = true;
this.OpenDialog.CheckPathExists = true;
this.OpenDialog.Multiselect = false; //choose one file
ResultDialog = OpenDialog.ShowDialog();
if (ResultDialog == DialogResult.OK)
{
MusicFileName = this.OpenDialog.FileName;
FoundIt = true;
}
}
catch
{
string Msg = "Not Sound file, Search for another..!";
MessageBox.Show(Msg);
return;
}
}
Draw lines and use the FillRectangle
method to draw a point when playing audio:

private void DrawSomething()
{
int PageWidth = PicView.Width;
int PageHeight = PicView.Height;
int yStart = PageHeight - 20;
double Pi = Math.PI;
if(Muted == false)
MyPlayer.Volume = this.tbVolume.Value;
MyPlayer.Volume = MyPlayer.Pause == true? 0 : this.tbVolume.Value;
/* you can use the following code instead previous line:
if(MyPlayer.Pause == true)
MyPlayer.Volume = 0;
else
MyPlayer.Volume = this.tbVolume.Value; */
//ratio between PicView.Height and maximum of player volume
double yRatio = Convert.ToDouble(PageHeight)/1000;
Graphics g;
// Create graphics object
g = PicView.CreateGraphics();
System.Threading.Thread.Sleep(150); // waiting a moment before clear drawing
g.Clear(PicView.BackColor);
Random randNum = new Random();
double W = PageWidth/20;
Pen NewPen = new Pen(Color.SteelBlue);
SolidBrush brush = new SolidBrush(Color.SkyBlue);
for (double X = -3*Pi ; X < 3*Pi; X+=0.5)
{
double Z = Math.Sin(X);
double Y = Math.Abs(Z);
double PX = PageWidth/2 + W*X; // Multiply X * W to enlarge X.
int maxY = (int)(yRatio * MyPlayer.Volume);
if(maxY < 40)
maxY = 40;
double H = randNum.Next(5, maxY - 30); // Random height:
double PY = yStart - H*Y; // Multiply Y * H to enlarge Y.
Point p1 = new Point((int)PX, PageHeight - 10);
Point p2 = new Point((int)PX, (int)PY);
// draw Line
g.DrawLine(NewPen, p1, p2);
Point dPoint = new Point((int)PX, (int)PY);
dPoint.X = dPoint.X - 1;
dPoint.Y = dPoint.Y - 10;
Rectangle rect = new Rectangle(dPoint, new Size(3, 3)); // size of Rectangle as point
//using FillRectangle to draw a point
g.FillRectangle(brush, rect);
}
// Release graphics object
NewPen.Dispose();
g.Dispose();
}
Draw string
:
private void DrawText()
{
PicView.Image = null;
int PageWidth = PicView.Width;
int PageHeight = PicView.Height;
// Initiate 'ImageToDraw' Object:
Bitmap ImageToDraw = new Bitmap(PageWidth, PageHeight);
// Create graphics object:
Graphics g = Graphics.FromImage(ImageToDraw);
// Draw "Hello World..." string:
Font txtFont = new Font("Tahoma", 24, FontStyle.Bold);
string txt = "Hello World...";
g.DrawString(txt, txtFont, new SolidBrush(Color.SeaGreen), 20, 60);
// Draw "Audio Player" string:
txtFont = new Font("Monotype Corsiva", 18, FontStyle.Bold | FontStyle.Italic);
txt = "Audio Player";
g.DrawString(txt, txtFont, new SolidBrush(Color.Chocolate), 240, 100);
// Display the bitmap:
PicView.Image = ImageToDraw;
// Release graphics object
g.Dispose();
ImageToDraw = null;
}
Remark
When extract the *.zip files, you can find MediaControl.dll file in (..\..\bin) folder. You can read about commands: Play, Pause, Stop, ... etc.
You can also read about resizing the form and the work of all buttons.
Final Words
I hope this article is useful. If you have any ideas, please tell me. Thanks to Code Project and thanks to all.
Mostafa Kaisoun
m_kaisoun@hotmail.com