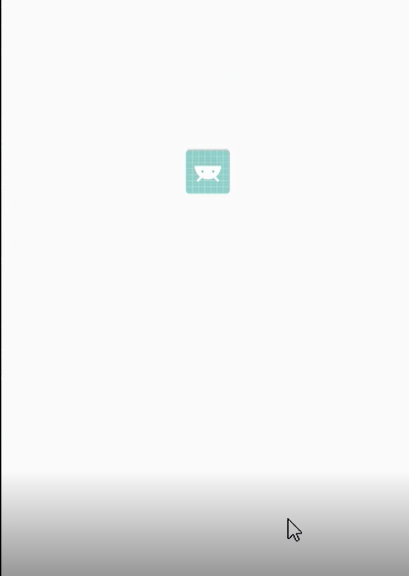
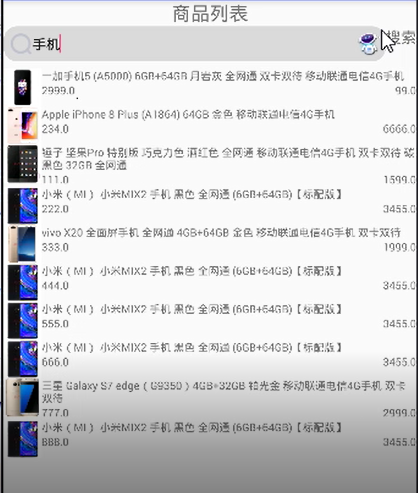
一.http
1.HttpConfig
public class HttpConfig {
public static String goodslist_url = "http://120.27.23.105/product/searchProducts";
}
1.HttpConfig
public class HttpConfig {
public static String goodslist_url = "http://120.27.23.105/product/searchProducts";
}
二.model
1.GetGoodsListener (监听接口)
public interface GetGoodsListener {
void getSuccess(String json);
1.GetGoodsListener (监听接口)
public interface GetGoodsListener {
void getSuccess(String json);
void getError(String error);
}
}
2.IModel
public interface IModel {
//获取商品列表数据
void getGoodsListData(String url, Map<String,String> map,GetGoodsListener getGoodsListener);
}
public interface IModel {
//获取商品列表数据
void getGoodsListData(String url, Map<String,String> map,GetGoodsListener getGoodsListener);
}
3.ModelImpl
public class ModelImpl implements IModel {
@Override
public void getGoodsListData(String url, Map<String, String> map, final GetGoodsListener getGoodsListener) {
HttpUtils httpUtils = HttpUtils.getHttpUtils();
httpUtils.okPost(url, map);
httpUtils.setOkLoadListener(new OkLoadListener() {
@Override
public void okLoadSuccess(String json) {
getGoodsListener.getSuccess(json);
}
public class ModelImpl implements IModel {
@Override
public void getGoodsListData(String url, Map<String, String> map, final GetGoodsListener getGoodsListener) {
HttpUtils httpUtils = HttpUtils.getHttpUtils();
httpUtils.okPost(url, map);
httpUtils.setOkLoadListener(new OkLoadListener() {
@Override
public void okLoadSuccess(String json) {
getGoodsListener.getSuccess(json);
}
@Override
public void okLoadError(String error) {
getGoodsListener.getError(error);
}
});
}
}
public void okLoadError(String error) {
getGoodsListener.getError(error);
}
});
}
}
三.presenter
1.IPresenter
public interface IPresenter {
void showGoodsListToView(IModel iModel, IMainView iMainView);
}
1.IPresenter
public interface IPresenter {
void showGoodsListToView(IModel iModel, IMainView iMainView);
}
2.PresenterImpl
public class PresenterImpl implements IPresenter {
private static final String TAG = "PresenterImpl---";
@Override
public void showGoodsListToView(IModel iModel, final IMainView iMainView) {
Map<String,String> map = new HashMap<>();
map.put("keywords",iMainView.getContent());
map.put("page","1");
iModel.getGoodsListData(HttpConfig.goodslist_url, map, new GetGoodsListener() {
@Override
public void getSuccess(String json) {
Log.d(TAG, "数据----: "+json);
Gson gson = new Gson();
GoodsListBean goodsListBean = gson.fromJson(json, GoodsListBean.class);
List<GoodsListBean.DataBean> list = goodsListBean.getData();
//放入view
iMainView.showGoodsList(list);
}
public class PresenterImpl implements IPresenter {
private static final String TAG = "PresenterImpl---";
@Override
public void showGoodsListToView(IModel iModel, final IMainView iMainView) {
Map<String,String> map = new HashMap<>();
map.put("keywords",iMainView.getContent());
map.put("page","1");
iModel.getGoodsListData(HttpConfig.goodslist_url, map, new GetGoodsListener() {
@Override
public void getSuccess(String json) {
Log.d(TAG, "数据----: "+json);
Gson gson = new Gson();
GoodsListBean goodsListBean = gson.fromJson(json, GoodsListBean.class);
List<GoodsListBean.DataBean> list = goodsListBean.getData();
//放入view
iMainView.showGoodsList(list);
}
@Override
public void getError(String error) {
Log.d(TAG, "getError: "+error);
}
});
}
}
public void getError(String error) {
Log.d(TAG, "getError: "+error);
}
});
}
}
四.view
1.IMainView
public interface IMainView {
//显示商品也
void showGoodsList(List<GoodsListBean.DataBean> list);
1.IMainView
public interface IMainView {
//显示商品也
void showGoodsList(List<GoodsListBean.DataBean> list);
//获取输入框内容
String getContent();
String getContent();
}
2.SplashActivity (属性动画跳转界面)
public class SplashActivity extends AppCompatActivity {
public class SplashActivity extends AppCompatActivity {
private int width;
private int height;
private int height;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_splash2);
//获取屏幕宽高
WindowManager wm = (WindowManager) this
.getSystemService(Context.WINDOW_SERVICE);
width = wm.getDefaultDisplay().getWidth();
height = wm.getDefaultDisplay().getHeight();
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_splash2);
//获取屏幕宽高
WindowManager wm = (WindowManager) this
.getSystemService(Context.WINDOW_SERVICE);
width = wm.getDefaultDisplay().getWidth();
height = wm.getDefaultDisplay().getHeight();
//初始化页面
initViews();
}
initViews();
}
private void initViews() {
ImageView imageView=findViewById(R.id.splash_pic);
//属性动画
ObjectAnimator translationY = ObjectAnimator.ofFloat(imageView, "translationY", 0, height / 2);
ObjectAnimator scaleX = ObjectAnimator.ofFloat(imageView, "scaleX", 2, 1);
ObjectAnimator scaleY = ObjectAnimator.ofFloat(imageView, "scaleY", 2, 1);
ObjectAnimator alpha = ObjectAnimator.ofFloat(imageView, "alpha", 0, 1);
ObjectAnimator rotation = ObjectAnimator.ofFloat(imageView, "rotation", 360);
ImageView imageView=findViewById(R.id.splash_pic);
//属性动画
ObjectAnimator translationY = ObjectAnimator.ofFloat(imageView, "translationY", 0, height / 2);
ObjectAnimator scaleX = ObjectAnimator.ofFloat(imageView, "scaleX", 2, 1);
ObjectAnimator scaleY = ObjectAnimator.ofFloat(imageView, "scaleY", 2, 1);
ObjectAnimator alpha = ObjectAnimator.ofFloat(imageView, "alpha", 0, 1);
ObjectAnimator rotation = ObjectAnimator.ofFloat(imageView, "rotation", 360);
//添加到动画集合
AnimatorSet animatorSet = new AnimatorSet();
animatorSet.playTogether(translationY,scaleX,scaleY,alpha,rotation);
AnimatorSet animatorSet = new AnimatorSet();
animatorSet.playTogether(translationY,scaleX,scaleY,alpha,rotation);
//设置
animatorSet.setDuration(3000);
animatorSet.start();
animatorSet.addListener(new Animator.AnimatorListener() {
@Override
public void onAnimationStart(Animator animation) {
animatorSet.setDuration(3000);
animatorSet.start();
animatorSet.addListener(new Animator.AnimatorListener() {
@Override
public void onAnimationStart(Animator animation) {
}
@Override
public void onAnimationEnd(Animator animation) {
//动画结束的时候,跳转页面
Intent intent = new Intent(SplashActivity.this, MainActivity.class);
startActivity(intent);
}
public void onAnimationEnd(Animator animation) {
//动画结束的时候,跳转页面
Intent intent = new Intent(SplashActivity.this, MainActivity.class);
startActivity(intent);
}
@Override
public void onAnimationCancel(Animator animation) {
public void onAnimationCancel(Animator animation) {
}
@Override
public void onAnimationRepeat(Animator animation) {
public void onAnimationRepeat(Animator animation) {
}
});
});
}
}
2.5 activity_splash2(动画对应的布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
}
2.5 activity_splash2(动画对应的布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ImageView
android:id="@+id/splash_pic"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:src="@mipmap/ic_launcher" />
android:id="@+id/splash_pic"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:src="@mipmap/ic_launcher" />
</LinearLayout>
3.MainActivity (主方法)
public class MainActivity extends AppCompatActivity implements IMainView, View.OnClickListener {
private static final String TAG = "MainActivity-----";
private RecyclerView recyclerView;
private MySearchView mySearchView;
private TextView sousuo;
private PresenterImpl presenter;
private boolean flag = true;
private ImageView change;
public class MainActivity extends AppCompatActivity implements IMainView, View.OnClickListener {
private static final String TAG = "MainActivity-----";
private RecyclerView recyclerView;
private MySearchView mySearchView;
private TextView sousuo;
private PresenterImpl presenter;
private boolean flag = true;
private ImageView change;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//初始化界面
initViews();
//初始化数据
initDatas();
}
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//初始化界面
initViews();
//初始化数据
initDatas();
}
private void initDatas() {
presenter = new PresenterImpl();
presenter.showGoodsListToView(new ModelImpl(), this);
}
presenter = new PresenterImpl();
presenter.showGoodsListToView(new ModelImpl(), this);
}
private void initViews() {
recyclerView = findViewById(R.id.recyclerView);
mySearchView = findViewById(R.id.mysearch);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView = findViewById(R.id.recyclerView);
mySearchView = findViewById(R.id.mysearch);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
sousuo = findViewById(R.id.sousuo);
sousuo.setOnClickListener(this);
sousuo.setOnClickListener(this);
change = findViewById(R.id.change);
change.setOnClickListener(this);
}
change.setOnClickListener(this);
}
//显示商品列表
@Override
public void showGoodsList(List<GoodsListBean.DataBean> list) {
Log.d(TAG, "showGoodsList: " + list);
MyAdapter myAdapter = new MyAdapter(MainActivity.this, list);
recyclerView.setAdapter(myAdapter);
}
@Override
public void showGoodsList(List<GoodsListBean.DataBean> list) {
Log.d(TAG, "showGoodsList: " + list);
MyAdapter myAdapter = new MyAdapter(MainActivity.this, list);
recyclerView.setAdapter(myAdapter);
}
@Override
public String getContent() {
String content = mySearchView.getContent();
if (TextUtils.isEmpty(content)) {
content = "笔记本";
}
return content;
}
public String getContent() {
String content = mySearchView.getContent();
if (TextUtils.isEmpty(content)) {
content = "笔记本";
}
return content;
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.sousuo:
public void onClick(View v) {
switch (v.getId()) {
case R.id.sousuo:
presenter.showGoodsListToView(new ModelImpl(), this);
break;
case R.id.change:
if (flag) {
recyclerView.setLayoutManager(new GridLayoutManager(MainActivity.this, 2));
change.setImageResource(R.drawable.kind_liner);
} else {
recyclerView.setLayoutManager(new LinearLayoutManager(this));
change.setImageResource(R.drawable.kind_grid);
}
flag = !flag;
break;
}
}
}
3.5(主布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.daydayup.day15_lianxi.view.MainActivity">
recyclerView.setLayoutManager(new GridLayoutManager(MainActivity.this, 2));
change.setImageResource(R.drawable.kind_liner);
} else {
recyclerView.setLayoutManager(new LinearLayoutManager(this));
change.setImageResource(R.drawable.kind_grid);
}
flag = !flag;
break;
}
}
}
3.5(主布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.daydayup.day15_lianxi.view.MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center_horizontal"
android:text="商品列表"
android:textSize="25sp" />
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center_horizontal"
android:text="商品列表"
android:textSize="25sp" />
<ImageView
android:id="@+id/change"
android:layout_width="40dp"
android:layout_height="40dp"
android:src="@drawable/kind_grid" />
</LinearLayout>
android:id="@+id/change"
android:layout_width="40dp"
android:layout_height="40dp"
android:src="@drawable/kind_grid" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="0.75dp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<com.daydayup.day15_lianxi.view.MySearchView
android:id="@+id/mysearch"
android:layout_width="0dp"
android:layout_height="55dp"
android:layout_weight="1"></com.daydayup.day15_lianxi.view.MySearchView>
android:id="@+id/mysearch"
android:layout_width="0dp"
android:layout_height="55dp"
android:layout_weight="1"></com.daydayup.day15_lianxi.view.MySearchView>
<TextView
android:id="@+id/sousuo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="搜索"
android:textSize="20sp" />
android:id="@+id/sousuo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="搜索"
android:textSize="20sp" />
</LinearLayout>
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="wrap_content"></android.support.v7.widget.RecyclerView>
</LinearLayout>
4.MyAdapter (适配器)
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> {
private Context context;
private List<GoodsListBean.DataBean> list;
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> {
private Context context;
private List<GoodsListBean.DataBean> list;
public MyAdapter(Context context, List<GoodsListBean.DataBean> list) {
this.list = list;
this.context = context;
this.context = context;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(context).inflate(R.layout.item_layout, parent, false);
MyViewHolder myViewHolder = new MyViewHolder(view);
return myViewHolder;
}
MyViewHolder myViewHolder = new MyViewHolder(view);
return myViewHolder;
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
String pic_url = list.get(position).getImages().split("\\|")[0];
Glide.with(context).load(pic_url).into(holder.getImageView());
public void onBindViewHolder(MyViewHolder holder, int position) {
String pic_url = list.get(position).getImages().split("\\|")[0];
Glide.with(context).load(pic_url).into(holder.getImageView());
holder.getTitle().setText(list.get(position).getTitle());
holder.getPrice().setText(list.get(position).getPrice() + "");
holder.getPrice2().setText(list.get(position).getBargainPrice() + "");
}
@Override
public int getItemCount() {
return list.size();
}
public int getItemCount() {
return list.size();
}
class MyViewHolder extends RecyclerView.ViewHolder {
private final ImageView imageView;
private final TextView title;
private final TextView price;
private final TextView price2;
private final TextView title;
private final TextView price;
private final TextView price2;
public MyViewHolder(View itemView) {
super(itemView);
//找控件
imageView = itemView.findViewById(R.id.item_pic);
title = itemView.findViewById(R.id.itme_title);
price = itemView.findViewById(R.id.item_price);
price2 = itemView.findViewById(R.id.item_price2);
}
super(itemView);
//找控件
imageView = itemView.findViewById(R.id.item_pic);
title = itemView.findViewById(R.id.itme_title);
price = itemView.findViewById(R.id.item_price);
price2 = itemView.findViewById(R.id.item_price2);
}
public MyViewHolder(View itemView, ImageView imageView, TextView title, TextView price, TextView price2) {
super(itemView);
this.imageView = imageView;
this.title = title;
this.price = price;
this.price2 = price2;
}
super(itemView);
this.imageView = imageView;
this.title = title;
this.price = price;
this.price2 = price2;
}
public ImageView getImageView() {
return imageView;
}
return imageView;
}
public TextView getTitle() {
return title;
}
return title;
}
public TextView getPrice() {
return price;
}
return price;
}
public TextView getPrice2() {
return price2;
}
}
return price2;
}
}
}
4.5 item_layout(对应的布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/item_pic"
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/ic_launcher" />
android:id="@+id/item_pic"
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/ic_launcher" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/itme_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="标题" />
android:id="@+id/itme_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="标题" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/item_price"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="价格" />
android:id="@+id/item_price"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="价格" />
<TextView
android:id="@+id/item_price2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="折扣价格" />
</LinearLayout>
android:id="@+id/item_price2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="折扣价格" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
5.MySearchView (搜索框)
public class MySearchView extends LinearLayout {
public class MySearchView extends LinearLayout {
private EditText search_content;
//1.
//直接new的时候
public MySearchView(Context context) {
this(context, null);
}
//直接new的时候
public MySearchView(Context context) {
this(context, null);
}
public MySearchView(Context context, @Nullable AttributeSet attrs) {
this(context, attrs, 0);
}
this(context, attrs, 0);
}
//在布局文件里面时候控件的时候
public MySearchView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//初始化
View view = View.inflate(context, R.layout.layout_search, this);
search_content = view.findViewById(R.id.search_content);
// search_content.setOnClickListener(new OnClickListener() {
// @Override
// public void onClick(View v) {
// search_content.setFocusable(true);
// }
// });
}
public MySearchView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//初始化
View view = View.inflate(context, R.layout.layout_search, this);
search_content = view.findViewById(R.id.search_content);
// search_content.setOnClickListener(new OnClickListener() {
// @Override
// public void onClick(View v) {
// search_content.setFocusable(true);
// }
// });
}
//获取输入的内容
public String getContent() {
return search_content.getText().toString();
}
return search_content.getText().toString();
}
}
5.5 layout_search(搜索框对应的布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/search_bg"
android:orientation="horizontal"
android:padding="8dp">
5.5 layout_search(搜索框对应的布局)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/search_bg"
android:orientation="horizontal"
android:padding="8dp">
<ImageView
android:layout_width="@dimen/search_height"
android:layout_height="@dimen/search_height"
android:src="@drawable/a_4" />
android:layout_width="@dimen/search_height"
android:layout_height="@dimen/search_height"
android:src="@drawable/a_4" />
<EditText
android:id="@+id/search_content"
android:layout_width="0dp"
android:layout_height="@dimen/search_height"
android:layout_weight="1"
android:background="@null" />
android:id="@+id/search_content"
android:layout_width="0dp"
android:layout_height="@dimen/search_height"
android:layout_weight="1"
android:background="@null" />
<ImageView
android:layout_width="@dimen/search_height"
android:layout_height="@dimen/search_height"
android:src="@drawable/root" />
android:layout_width="@dimen/search_height"
android:layout_height="@dimen/search_height"
android:src="@drawable/root" />
</LinearLayout>
一些补充
1.写在drawable里的(search_bg)
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<size
android:width="50dp"
android:height="@dimen/search_height" />
<solid android:color="#55999999" />
<corners android:radius="18dp" />
android:width="50dp"
android:height="@dimen/search_height" />
<solid android:color="#55999999" />
<corners android:radius="18dp" />
</shape>
2.写在values里的(dimens)
<resources>
<resources>
<dimen name="search_height">30dp</dimen>
</resources>
3.依赖
compile 'com.google.code.gson:gson:2.6.2'
compile 'com.google.code.gson:gson:2.6.2'
compile 'com.squareup.okhttp3:okhttp:3.3.0'
compile 'com.github.bumptech.glide:glide:3.7.0'
compile 'com.android.support:recyclerview-v7:26.0.0-alpha1'
4.权限
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.INTERNET"/>
//需要把SplashActivity设为主启动页面
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".view.MainActivity"></activity>
<activity android:name=".view.SplashActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".view.MainActivity"></activity>
<activity android:name=".view.SplashActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</intent-filter>
</activity>
</application>