1、选秀节目打分,分为专家评委和大众评委,score[]数组里面存储每个评委打的分数,judge_type[]里存储与 score[]数组对应的评委类别,judge_type[i] == 1,表示专家评委,judge_type[i] == 2,表示大众评委,n表示评委总数。打分规则如下:专家评委和大众评委的分数先分别取一个平均分(平均分取整),然后,总分 = 专家评委平均分 * 0.6 +大众评委 * 0.4,总分取整。如果没有大众评委,则总分 =专家评委平均分,总分取整。函数最终返回选手得分。
函数接口 intcal_score(int score[], int judge_type[], int n)
- #include <iostream>
- using namespace std;
-
- int cal_score(int score[],int judge_type[],int n)
- {
- int expert = 0;
- int publicJudge = 0;
-
- int number_of_expert = 0;
- int averageScore = 0;
-
- int index;
-
- for(index = 0; index < n;index++)
- {
- if(judge_type[index] = 1)
- {
- expert += score[index];
- number_of_expert ++;
- }
- else
- {
- publicJudge += score[index];
- }
- }
-
- if(number_of_expert == n)
- averageScore = expert/n;
- else
- averageScore = expert/number_of_expert*0.6 + publicJudge/(n-number_of_expert)*0.4;
-
- return averageScore;
- }
-
- int main()
- {
- int n = 10;
- int score[10] = {80,85,90,80,75,95,80,90,95};
- int judge_type[10] = {1,2,1,1,2,2,1,1,2,1};
- int average_score = cal_score(score,judge_type,n);
- cout << average_score << endl;
- return 0;
- }
2、给定一个数组input[],如果数组长度n为奇数,则将数组中最大的元素放到 output[]数组最中间的位置,如果数组长度n为偶数,则将数组中最大的元素放到 output[]数组中间两个位置偏右的那个位置上,然后再按从大到小的顺序,依次在第一个位置的两边,按照一左一右的顺序,依次存放剩下的数。
例如:input[] = {3, 6,1, 9, 7} output[] = {3, 7, 9, 6,1}; input[] = {3, 6, 1, 9, 7, 8} output[] = {1, 6, 8, 9, 7,3}
函数接口 voidsort(int input[[, int n, int output[])
- #include <iostream>
- using namespace std;
-
- #define N 1000
-
- void bubbleSort(int a[],int len)
- {
- int i,j,temp;
- for(i = 0;i < len-1;i++)
- for(j = i+1;j < len;j++)
- {
- if(a[i] > a[j])
- {
- temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
-
- void sort(int input[], int len, int output[])
- {
-
- int mid = len/2;
- int left = mid - 1;
- int right = mid + 1;
-
-
- bubbleSort(input,len);
-
- int index = len - 1;
- output[mid] = input[index--];
- while(index >= 0)
- {
- output[left--] = input[index--];
- output[right++] = input[index--];
- }
-
- for(left = 0;left < len;left++)
- cout << output[left] << " ";
- }
-
- int main()
- {
- int arr[] = {3,6,1,9,7,8};
- int destArr[N];
- sort(arr,6,destArr);
-
- cout << endl;
-
- int arr1[] = {31,6,15,9,72,8,99,5,6};
- int destArr1[N];
- sort(arr1,9,destArr1);
-
- return 0;
- }
3、操作系统任务调度问题。操作系统任务分为系统任务和用户任务两种。其中,系统任务的优先级 < 50,用户任务的优先级 >= 50且 <= 255。优先级大于255的为非法任务,应予以剔除。现有一任务队列task[],长度为n,task中的元素值表示任务的优先级,数值越小,优先级越高。函数scheduler实现如下功能,将task[]中的任务按照系统任务、用户任务依次存放到 system_task[]数组和 user_task[]数组中(数组中元素的值是任务在task[]数组中的下标),并且优先级高的任务排在前面,优先级相同的任务按照入队顺序排列(即先入队的任务排在前面),数组元素为-1表示结束。
例如:task[] = {0, 30,155, 1, 80, 300, 170, 40, 99} system_task[] = {0, 3, 1, 7,-1} user_task[] = {4, 8, 2, 6, -1}
函数接口 void scheduler(int task[], int n, int system_task[], int user_task[])
- #include <iostream>
- using namespace std;
-
- typedef struct stask{
- int priority;
- int index;
- }task_node;
-
- void scheduler(int task[], int n, int system_task[], int user_task[])
- {
- int i;
- task_node *task_list;
- task_list = new task_node[n*sizeof(task_node)];
-
- for(i = 0;i < n; i++)
- {
- task_list[i].priority = task[i];
- task_list[i].index = i;
- }
-
-
- task_node temp;
- for(i = 0;i < n - 1;i++)
- {
- for(int j = i+1;j < n;j++)
- if(task_list[i].priority > task_list[j].priority)
- {
- temp = task_list[i];
- task_list[i] = task_list[j];
- task_list[j] = temp;
- }
- }
- int k1= 0,k2 = 0;
-
- for(i = 0;i < n - 1;i++)
- {
- if(task_list[i].priority<50)
- system_task[k1++] = task_list[i].index;
- else
- if(task_list[i].priority >= 50 && task_list[i].priority <= 255)
- user_task[k2++] = task_list[i].index;
- }
- system_task[k1] = -1;
- user_task[k2] = -1;
-
-
- for(i = 0;i <= k1;i++)
- cout << system_task[i] << " ";
- cout << endl;
- for(i = 0;i <= k2;i++)
- cout << user_task[i] << " ";
-
- delete [] task_list;
- }
-
- int main()
- {
- int task[] = {0, 30,155, 1, 80, 300, 170, 40, 99};
- int system_task[10];
- int user_task[10];
- scheduler(task, 9, system_task, user_task);
-
- return 0;
- }
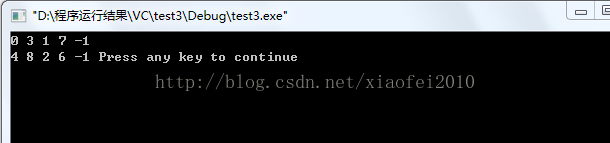
4.身份证号码合法性判断 问题描述
我国公民的身份证号码特点如下:
1.长度为18位
2.1-17位只能为数字
3.第十八位可以是数字或者小写英文字母
4.身份证号码的第7-14位表示持有人生日的年月日信息
请实现身份证号码合法性判断的函数,除满足以上要求外,需要对持有人生日的年月日信息进行校验,年份大于等于1900,小于等于2100年。需要考虑闰年、大小月的情况。所谓闰年,能被4整除且不能被100整除或能被400整除的年份。闰年2月份为29天,非闰年的2月份为28天。其他情况的合法性校验,不用考虑
函数返回值:
1.如果身份证号合法,返回0
2.如果身份证号长度不合法,返回1
3.如果身份证号第1-17位含有非数字的字符,返回2
4.如果第十八位既不是数字也不是英文小写字母x,返回3 5.如果身份证号的年信息非法,返回4
6.如果身份证号的月信息非法,返回5
7.如果身份证号的日信息非法,返回6(请注意闰年的情况)
注:除成功的情况外,以上其他情况合法性判断的优先级依次降低,也就是说,如果判断出长度不合法,直接返回1即可,不需要再做其他合法性判断
要求实现函数
int verifyIDCard(char *input)
示例:
1.输入:"511002 111222"返回1
2.输入:"511002 abc123456789" 返回2
3.输入:"511002 19880808123a"返回3
4.输入:"511002 188808081234" 返回4
5.输入:"511002 198813081234" 返回5
6.输入:"511002 198808321234"返回6
7.输入:"511002 198902291234"返回7
8.输入:"511002 198808081234"返回0
5. 链表逆序
- #include <iostream>
- #include <assert.h>
- using namespace std;
-
- typedef struct NODE{
- int value;
- NODE *pNext;
- }Node,*pNode;
-
-
- Node* creat()
- {
- int nuberOfNode = 5;
- int data;
- pNode head = new Node;
-
- if(NULL == head)
- cout << "分配内存失败\r\n";
-
- head->pNext = NULL;
-
- pNode p = head;
- pNode s = NULL;
- cout << "请输入数据:\r\n";
- while(nuberOfNode--)
- {
- cin >> data;
- s = new Node;
- assert(NULL != s);
- s->value = data;
- p->pNext = s;
- p = s;
- }
- p->pNext = NULL;
- return(head);
- }
-
-
- pNode reverseList(pNode Header)
- {
-
- if(Header == NULL || Header->pNext == NULL)
- return 0;
-
-
- pNode pre = Header->pNext;
- pNode cur = pre->pNext;
- pre->pNext = NULL;
- pNode next = NULL;
-
- while(NULL != cur)
- {
- next = cur->pNext;
- cur->pNext = pre;
- pre = cur;
- cur = next;
- }
- Header->pNext = pre;
-
- return Header;
- }
-
-
- void printList(pNode head)
- {
- pNode start = head->pNext;
- while(start)
- {
- cout << start->value << " ";
- start = start->pNext;
- }
- }
-
- int main()
- {
- pNode pl = creat();
- printList(pl);
- cout << endl;
- printList(reverseList(pl));
-
- return 0;
- }
6. 查找子字符串出现次数,并从原字符串中删除。
-
-
-
-
- #include <iostream>
- using namespace std;
-
-
- void delSpecifiedSubString(char *str,int start,int end)
- {
- char *p1 = &str[start];
- char *p2 = &str[end + 1];
- while((*p1++ = *p2++) != '\0');
- }
-
- int delSubString(char *str,char *subStr)
- {
- int count = 0;
- int index = 0;
-
- char *pstr = str;
- char *psubStr = subStr;
-
- if(str == NULL ||subStr == NULL)
- return 0;
-
- while(*pstr != '\0')
- {
- if(*pstr == *psubStr)
- {
- pstr++;
- psubStr++;
- index++;
- }
- else
- {
- psubStr = subStr;
- pstr = pstr - index + 1;
- index = 0;
- }
- if(*psubStr == '\0')
- {
- count++;
- psubStr = subStr;
- delSpecifiedSubString(str,
- pstr - index - str,
- pstr - 1 - str);
- }
- }
- cout << str << " " << count << endl;
- return count;
- }
-
- int main()
- {
- char str[50];
- char subStr[30];
- cout << "输入str和subStr:" << endl;
- gets(str);
- gets(subStr);
- delSubString(str,subStr);
-
- return 0;
- }
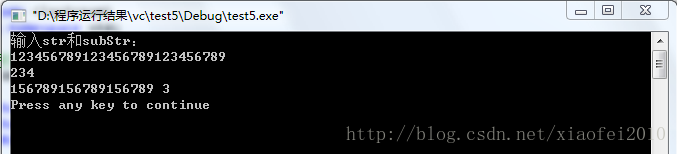
- <span style="color:#ff0000;">
- #include <iostream>
- #include <string>
- using namespace std;
-
- char *fun(char *str,char *subStr)
- {
- string s(str);
- while(s.find(subStr) != string::npos)
- {
- cout << s.find(subStr) << endl;
-
- s.erase(s.find(subStr),strlen(subStr));
- }
- char *m = new char[50];
- strcpy(m,s.c_str());
-
-
-
- return m;
- }
-
- int main()
- {
- char str[50];
- char subStr[30];
- gets(str);
- gets(subStr);
- cout << str << endl;
- cout << subStr << endl;
- cout << fun(str,subStr) << endl;
-
- return 0;
- }
- <span style="color:#ff0000;">
-
- #include <iostream>
- #include <string>
- using namespace std;
-
- char *fun(char *str,char *subStr)
- {
- string s(str);
- int index = 0;
- while(s.find(subStr,index) != string::npos)
- {
- index = s.find(subStr,index);
-
- s.erase(s.find(subStr),strlen(subStr));
- index++;
- }
- char *m = new char[50];
- strcpy(m,s.c_str());
-
-
-
- return m;
- }
-
- int main()
- {
- char str[50];
- char subStr[30];
- gets(str);
- gets(subStr);
- cout << "str:" << str << endl;
- cout << "subStr:" << subStr << endl;
- cout << "result:" << fun(str,subStr) << endl;
-
- return 0;
- }
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- #include <iostream>
- using namespace std;
-
- void sortVowel(char *input,char *output)
- {
- char arr[10] = {'a','e','i','o','u','A','E','I','O','U'};
- int number[10] = {0};
- int i = 0;
- int sum = 0;
-
- while('\0'!= *input)
- {
- for(i = 0;i < 10;i++)
- {
- if(arr[i] == *input)
- {
- number[i]++;
- break;
- }
- }
- input++;
- }
-
-
- for(i = 0;i < 10;i++)
- sum += number[i];
-
- output = new char[sum+1];
- char *start = output;
-
- for(i = 0;i < 10;i++)
- {
- while(number[i]--)
- *start++ = arr[i];
- }
- *start = '\0';
- while(*output!= '\0')
- cout << *output++;
-
- cout << endl;
- }
-
- int main()
- {
- char input[] = "Abort!May Be Some Errors In Out System.";
- char *output = NULL;
- sortVowel(input,output);
-
- return 0;
- }