发邮件需要用到python两个模块,smtplib和email,这俩模块是python自带的,只需import即可使用。smtplib模块主要负责发送邮件,email模块主要负责构造邮件。其中MIMEText()定义邮件正文,Header()定义邮件标题。MIMEMulipart模块构造带附件
发送HTML格式的邮件:
send_email_html.py
- import smtplib
- from email.mime.text import MIMEText
- from email.header import Header
-
-
- smtpserver = 'smtp.sina.com'
-
-
- user = "username@sina.com"
- password = "password"
-
-
- sender = "username@sina.com"
-
-
- receiver = "8888@qq.com"
-
-
- subject = 'email by python'
-
-
- msg = MIMEText('<html><body><a href="">百度一下</a></p></body></html>','html','utf-8')
- msg['Subject'] = Header(subject,'utf-8')
-
-
- smtp = smtplib.SMTP()
- smtp.connect(smtpserver)
- smtp.login(user,password)
- smtp.sendmail(sender,receiver,msg.as_string())
- smtp.quit()
说明:
smtplib.SMTP():实例化SMTP()
connect(host,port):
host:指定连接的邮箱服务器。
port:指定连接服务器的端口号,默认为25.
login(user,password):user:登录邮箱的用户名。password:登录邮箱的密码。
sendmail(from_addr,to_addrs,msg,...):
from_addr:邮件发送者地址
to_addrs:邮件接收者地址。字符串列表['接收地址1','接收地址2','接收地址3',...]或'接收地址'
msg:发送消息:邮件内容。一般是msg.as_string(),as_string()是将msg(MIMEText对象或者MIMEMultipart对象)变为str。
quit():用于结束SMTP会话。
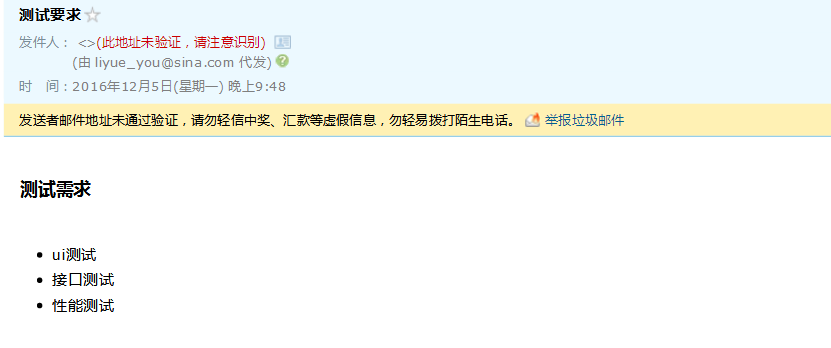
发送带附件的邮件
send_email_file.py
- import smtplib
- from email.mime.text import MIMEText
- from email.mime.multipart import MIMEMultipart
-
-
- smtpserver = 'smtp.sina.com'
-
-
- user ="xxx@sina.com"
- password = "xxx"
-
-
- sender = "xxx@sina.com"
-
-
- receiver = "1xxx@qq.com"
-
-
- subject = "附件的邮件"
-
-
- sendfile = open("C:\\Users\\Administrator\\Desktop\\html5.txt","r").read()
-
- att = MIMEText(sendfile,"base64","utf-8")
- att["Content-Type"] = "application/octet-stream"
- att["Content-Disposition"] = "attachment;filename = 'html5.txt'"
-
- msgRoot = MIMEMultipart('related')
- msgRoot['Subject'] = subject
- msgRoot.attach(att)
-
- smtp = smtplib.SMTP()
- smtp.connect(smtpserver)
- smtp.login(user,password)
- smtp.sendmail(sender,receiver,msgRoot.as_string())
- smtp.quit()
查找最新的测试报告
find_file.py
- import os
-
-
- result_dir = "E:\\自动化测试项目\\子项目_bbs\\report"
-
- lists = os.listdir(result_dir)
-
-
- lists.sort(key=lambda fn: os.path.getmtime(result_dir + "\\" + fn))
-
- print(('最新的文件是:' + lists[-1]))
- file = os.path.join(result_dir,lists[-1])
-
- print(file)
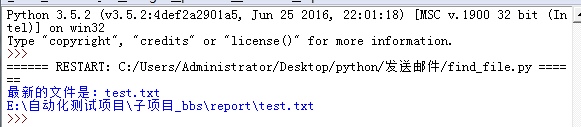
整合自动化测试发送测试报告邮件
- from HTMLTestRunner import HTMLTestRunner
- from email.mime.text import MIMEText
- from email.header import Header
- import smtplib
- import unittest
- import time
- import os
-
-
- def send_mail(file_new):
- f = open(file_new,'rb')
- mail_body = f.read()
- f.close()
-
- msg = MIMEText(mail_body,'html','utf-8')
- msg['Subject'] = Header("自动化测试报告",'utf-8')
-
- smtp = smtplib.SMTP()
- smtp.connect('smtp.sina.com')
- smtp.login("sender@sina.com","password")
- smtp.sendmail("sender@sina.com","receiver@qq.com",msg.as_string())
- smtp.quit()
- print("邮件已发出!注意查收。")
-
-
-
- def new_report(test_report):
- lists = os.listdir(test_report)
- lists.sort(key=lambda fn:os.path.getmtime(test_report + "\\" + fn))
- file_new = os.path.join(test_report,lists[-1])
- print(file_new)
- return file_new
-
- if __name__ == "__main__":
-
- test_dir = "测试用例存放目录"
- test_report = "测试报告存放目录"
-
- discover = unittest.defaultTestLoader.discover(test_dir,
- pattern = 'test_*.py')
- now = time.strftime("%Y-%m-%d_%H-%M-%S")
- filename = test_report + '\\' + now + 'result.html'
- fp = open(filename,'wb')
- runner = HTMLTestRunner(stream = fp,
- title = '测试报告',
- description = '用例执行情况:')
- runner.run(discover)
- fp.close()
-
- new_report = new_report(test_report)
- send_mail(new_report)
1.通过unittest框架的discover()找到匹配的测试用例,由HTMLTestRunner的run()方法执行测试用例并生成最新的测试报告。
2.调用new_report()函数找到测试报告目录下最新生成的测试报告,返回测试报告的路径。
3.将得到的最新测试报告的完整路径传给send_mail()函数,实现发邮件功能。
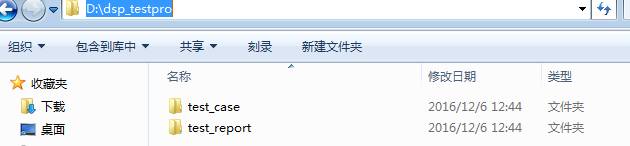
参考Python自动发送邮件总结:http://www.cnblogs.com/yufeihlf/p/5726619.html