目录
Java 中使用 selenium 和 chrome 浏览器下载动态网页
Java 使用Selenium调用浏览器(chrome)下载动态网页源代码
Java 中使用 selenium 和 chrome 浏览器下载动态网页
前言
上一篇文章《用 jsoup 分析下载的 html 内容》 中提到过,当我们用 OkHttp 下载网页的时候,发现下载的内容中没有我们在浏览器上看到的阅读数、评论数、喜欢数这三部分的内容。当时解释过是因为这些内容是浏览器通过 JS 动态渲染的结果。也就是浏览器先下载了 html 页面内容,然后通过 AJAX 请求了新的数据,再通过 JS 将元素添加到 Dom 树中的。所以直接下载的 html 代码中是看不到这部分内容的。那么是否我们就无法通过爬虫获取到这部分的内容呢。也不是。这就要引入一个新的工具 selenium 来实现。
selenium 介绍
selenium 是一套 web 自动化测试的软件。他能够通过编程的方式调用系统的浏览器,并驱动浏览器模拟人的方式进行操作。例如点击、输入信息、滚动屏幕等。通过这样的方式,我们就可以在程序中自动运行我们的 web 页面。同时,对于爬虫程序来说,也可以驱动浏览器访问我们要的目标页面,并且因为是在浏览器中访问的网页,所以浏览器会自动渲染动态的内容。这样就可以解决上次发生的用 OkHttp 下载网页无法获取到动态内容的问题。
selenium Windows 环境安装
selenium 可以支持几种主流的浏览器。chrome、firefox 都在支持之列,浏览器是通过不同的 WebDriver 来驱动的。所以除了对应的浏览器,我们就是要安装对应的 WebDriver。这里来演示如何在 Windows 环境中安装 WebDriver。
对于 Chrome 的 WebDriver 来说,不同版本的 WebDriver 支持的 Chrome 浏览器的版本范围都不一样。所以我们要针对自己安装的 Chrome 版本选择安装不同的 Chrome Web Driver 。例如,现在最新的版本 2.44 支持的 chrome 版本范围是 v69-71 。
首先我们要确认自己机器安装的 chrome 的版本号。启动 chrome 浏览器,点击右上角的菜单,依次选择“帮助”、;“关于 Google Chrome” 选项,如下所示
然后出现下面的界面
其中红字部分是版本号,我这里是最新版本70。
然后我们要去地址 ChromeDriver - WebDriver for Chrome - Downloads 去下载对应的 chrome 版本。
这里会有不同版本对不同 chrome 版本支持的说明,选择一个对应的就可以了。我的版本是70 ,所以选择了最新版本 2.44 下载
在具体的下载页面选择对应的平台即可。我这里选择了 chromedriver_win32.zip 。下载后解压出来一个 chromedriver.exe 文件,保存到一个指定的目录即可。
这样我们将一个windows 的 selenium 环境设置好了。
使用 selenium 和 chrome 下载动态网页
我们是在 Java 中使用 selenium ,所以在前文的基础上增加 selenium 相关的依赖
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
然后我们来改造一下 用 jsoup 分析下载的 html 内容 一文中的程序。原来是通过 OkHttp 来下载网页,这里就通过 selenium 结合 chrome 浏览器来进行
WebDriver webDriver = null;
try {
String url = "https://www.jianshu.com/p/675ea919230e";
//启动一个 chrome 实例
webDriver = new ChromeDriver();
//访问网址
webDriver.get(url);
Document document = Jsoup.parse(webDriver.getPageSource());
Element titleElement = document.selectFirst("div.article h1.title");
Element authorElement = document.selectFirst("div.article div.author span.name");
Element timeElement = document.selectFirst("div.article span.publish-time");
Element wordCountElement = document.selectFirst("div.article span.wordage");
Element viewCountElement = document.selectFirst("div.article span.views-count");
Element commentCountElement = document.selectFirst("div.article span.comments-count");
Element likeCountElement = document.selectFirst("div.article span.likes-count");
Element contentElement = document.selectFirst("div.article div.show-content");
if (titleElement != null) {
System.out.println("标题:" + titleElement.text());
}
if (authorElement != null) {
System.out.println("作者:" + authorElement.text());
}
if (timeElement != null) {
System.out.println("发布时间:" + timeElement.text());
}
if (wordCountElement != null) {
System.out.println(wordCountElement.text());
}
if (viewCountElement != null) {
System.out.println(viewCountElement.text());
}
if (commentCountElement != null) {
System.out.println(commentCountElement.text());
}
if (likeCountElement != null) {
System.out.println(likeCountElement.text());
}
if (contentElement != null && contentElement.text() != null) {
System.out.println("正文长度:" + contentElement.text().length());
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (webDriver != null) {
//退出 chrome
webDriver.quit();
}
}
运行这段代码之前,我们需要在虚拟机参数中指定 webdriver.chrome.driver 参数,值应该是我们下载的 chromedriver.exe 的路径。例如
这里,为了区分版本,我将 chromedriver.exe 改名为 chromedriver_2.44.exe 了。运行这个代码,会看到程序自动打开了一个 chrome 窗口,并且自动访问 url 地址
运行完毕后,窗口自动推出。其中红字部分表明这个 chrome 是被 selenium 自动控制得。在控制台会输出如下内容
标题:是什么支撑了淘宝双十一,没错就是它java编程语言。
作者:Java帮帮
发布时间:2018.08.29 14:49
字数 561
阅读 628
评论 0
喜欢 4
正文长度:655
可以看到,上一次通过 OkHttp 下载是没有得阅读、评论、喜欢数量能够被解析并且输出来了。
selenium chrome 的一些操作说明
上面的例子只是说明了如何在 selenium 中打开一个网页并获取他的内容。实际上我们前面说过了 selenium 是一个 web 的自动化测试框架,他是可以模拟人对页面的元素进行操作的。例如定位元素,在文本框里输入内容,点击元素等。下面的代码就演示了如何通过程序自动打开简书的首页并且在网站内查询 Spring Boot 相关的内容
WebDriver webDriver;
try {
String url = "https://www.jianshu.com/";
webDriver = new ChromeDriver();
webDriver.get(url);
webDriver.findElement(By.cssSelector("#q")).sendKeys("SpringBoot");
webDriver.findElement(By.cssSelector(".search-btn")).click();
} catch (Exception e) {
e.printStackTrace();
}
执行这段代码,程序会自动打开一个 chrome 窗口,并且在首页的搜索窗口输入 Spring Boot 文本,并且自动点击搜索按钮,然后得到搜索结果页面。
更多详细的用法大家可以自己去探索,这里就不一一详细介绍了。
作者:阿土伯已经不是我
链接:https://www.jianshu.com/p/b5b48f1b9a9e
来源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
Java 使用Selenium调用浏览器(chrome)下载动态网页源代码
本文主要介绍Java中,使用Selenium调用浏览器(chrome)下载动态网页源代码,并且实现WebDriverPool来进行性能优化,从WebDriverPool池中获取WebDriver对象,以及相关实现示例代码。
1、下载引用Selenium
参考文档:Java Selenium(Chrome)载取滚动条网页长图的方法及示例代码-CJavaPy
2、WebDriverPool实现代码
package us.codecraft.webmagic.downloader.selenium;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.phantomjs.PhantomJSDriver;
import org.openqa.selenium.phantomjs.PhantomJSDriverService;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.FileReader;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Properties;
import java.util.concurrent.BlockingDeque;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.atomic.AtomicInteger;
class WebDriverPool {
private Logger logger = LoggerFactory.getLogger(getClass());
private final static int DEFAULT_CAPACITY = 5;
private final int capacity;
private final static int STAT_RUNNING = 1;
private final static int STAT_CLODED = 2;
private AtomicInteger stat = new AtomicInteger(STAT_RUNNING);
private WebDriver mDriver = null;
private boolean mAutoQuitDriver = true;
private static final String DEFAULT_CONFIG_FILE = "/data/webmagic/webmagic-selenium/config.ini";
private static final String DRIVER_FIREFOX = "firefox";
private static final String DRIVER_CHROME = "chrome";
private static final String DRIVER_PHANTOMJS = "phantomjs";
protected static Properties sConfig;
protected static DesiredCapabilities sCaps;
public void configure() throws IOException {
// Read config file
sConfig = new Properties();
String configFile = DEFAULT_CONFIG_FILE;
if (System.getProperty("selenuim_config")!=null){
configFile = System.getProperty("selenuim_config");
}
sConfig.load(new FileReader(configFile));
// Prepare capabilities
sCaps = new DesiredCapabilities();
sCaps.setJavascriptEnabled(true);
sCaps.setCapability("takesScreenshot", false);
String driver = sConfig.getProperty("driver", DRIVER_PHANTOMJS);
// Fetch PhantomJS-specific configuration parameters
if (driver.equals(DRIVER_PHANTOMJS)) {
// "phantomjs_exec_path"
if (sConfig.getProperty("phantomjs_exec_path") != null) {
sCaps.setCapability(
PhantomJSDriverService.PHANTOMJS_EXECUTABLE_PATH_PROPERTY,
sConfig.getProperty("phantomjs_exec_path"));
} else {
throw new IOException(
String.format(
"Property '%s' not set!",
PhantomJSDriverService.PHANTOMJS_EXECUTABLE_PATH_PROPERTY));
}
// "phantomjs_driver_path"
if (sConfig.getProperty("phantomjs_driver_path") != null) {
System.out.println("Test will use an external GhostDriver");
sCaps.setCapability(
PhantomJSDriverService.PHANTOMJS_GHOSTDRIVER_PATH_PROPERTY,
sConfig.getProperty("phantomjs_driver_path"));
} else {
System.out
.println("Test will use PhantomJS internal GhostDriver");
}
}
// Disable "web-security", enable all possible "ssl-protocols" and
// "ignore-ssl-errors" for PhantomJSDriver
// sCaps.setCapability(PhantomJSDriverService.PHANTOMJS_CLI_ARGS, new
// String[] {
// "--web-security=false",
// "--ssl-protocol=any",
// "--ignore-ssl-errors=true"
// });
ArrayList<String> cliArgsCap = new ArrayList<String>();
cliArgsCap.add("--web-security=false");
cliArgsCap.add("--ssl-protocol=any");
cliArgsCap.add("--ignore-ssl-errors=true");
sCaps.setCapability(PhantomJSDriverService.PHANTOMJS_CLI_ARGS,
cliArgsCap);
// Control LogLevel for GhostDriver, via CLI arguments
sCaps.setCapability(
PhantomJSDriverService.PHANTOMJS_GHOSTDRIVER_CLI_ARGS,
new String[] { "--logLevel="
+ (sConfig.getProperty("phantomjs_driver_loglevel") != null ? sConfig
.getProperty("phantomjs_driver_loglevel")
: "INFO") });
// String driver = sConfig.getProperty("driver", DRIVER_PHANTOMJS);
// Start appropriate Driver
if (isUrl(driver)) {
sCaps.setBrowserName("phantomjs");
mDriver = new RemoteWebDriver(new URL(driver), sCaps);
} else if (driver.equals(DRIVER_FIREFOX)) {
mDriver = new FirefoxDriver(sCaps);
} else if (driver.equals(DRIVER_CHROME)) {
mDriver = new ChromeDriver(sCaps);
} else if (driver.equals(DRIVER_PHANTOMJS)) {
mDriver = new PhantomJSDriver(sCaps);
}
}
private boolean isUrl(String urlString) {
try {
new URL(urlString);
return true;
} catch (MalformedURLException mue) {
return false;
}
}
/**
* store webDrivers created
*/
private List<WebDriver> webDriverList = Collections
.synchronizedList(new ArrayList<WebDriver>());
/**
* store webDrivers available
*/
private BlockingDeque<WebDriver> innerQueue = new LinkedBlockingDeque<WebDriver>();
public WebDriverPool(int capacity) {
this.capacity = capacity;
}
public WebDriverPool() {
this(DEFAULT_CAPACITY);
}
public WebDriver get() throws InterruptedException {
checkRunning();
WebDriver poll = innerQueue.poll();
if (poll != null) {
return poll;
}
if (webDriverList.size() < capacity) {
synchronized (webDriverList) {
if (webDriverList.size() < capacity) {
// add new WebDriver instance into pool
try {
configure();
innerQueue.add(mDriver);
webDriverList.add(mDriver);
} catch (IOException e) {
e.printStackTrace();
}
// ChromeDriver e = new ChromeDriver();
// WebDriver e = getWebDriver();
// innerQueue.add(e);
// webDriverList.add(e);
}
}
}
return innerQueue.take();
}
public void returnToPool(WebDriver webDriver) {
checkRunning();
innerQueue.add(webDriver);
}
protected void checkRunning() {
if (!stat.compareAndSet(STAT_RUNNING, STAT_RUNNING)) {
throw new IllegalStateException("Already closed!");
}
}
public void closeAll() {
boolean b = stat.compareAndSet(STAT_RUNNING, STAT_CLODED);
if (!b) {
throw new IllegalStateException("Already closed!");
}
for (WebDriver webDriver : webDriverList) {
logger.info("Quit webDriver" + webDriver);
webDriver.quit();
webDriver = null;
}
}
}
3、调用Selenium(WebDriver)下载网页
通过WebDriverPool中获取WebDriver对象,调用浏览器下载动态静态网页代码。
package us.codecraft.webmagic.downloader.selenium;
import org.openqa.selenium.By;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import us.codecraft.webmagic.Page;
import us.codecraft.webmagic.Request;
import us.codecraft.webmagic.Site;
import us.codecraft.webmagic.Task;
import us.codecraft.webmagic.downloader.Downloader;
import us.codecraft.webmagic.selector.Html;
import us.codecraft.webmagic.selector.PlainText;
import java.io.Closeable;
import java.io.IOException;
import java.util.Map;
/**
* 使用Selenium调用浏览器进行渲染。目前仅支持chrome。<br>
* 需要下载Selenium driver支持。<br>
*/
public class SeleniumDownloader{
private volatile WebDriverPool webDriverPool;
private Logger logger = LoggerFactory.getLogger(getClass());
private int sleepTime = 0;
private int poolSize = 1;
private static final String DRIVER_PHANTOMJS = "phantomjs";
public SeleniumDownloader(String chromeDriverPath) {
System.getProperties().setProperty("webdriver.chrome.driver",
chromeDriverPath);
}
public SeleniumDownloader() {
// System.setProperty("phantomjs.binary.path",
// "/Users/Bingo/Downloads/phantomjs-1.9.7-macosx/bin/phantomjs");
}
public SeleniumDownloader setSleepTime(int sleepTime) {
this.sleepTime = sleepTime;
return this;
}
public String download(Request request, Task task) {
checkInit();
WebDriver webDriver;
try {
webDriver = webDriverPool.get();
} catch (InterruptedException e) {
logger.warn("interrupted", e);
return null;
}
logger.info("downloading page " + request.getUrl());
webDriver.get(request.getUrl());
try {
Thread.sleep(sleepTime);
} catch (InterruptedException e) {
e.printStackTrace();
}
WebDriver.Options manage = webDriver.manage();
Site site = task.getSite();
if (site.getCookies() != null) {
for (Map.Entry<String, String> cookieEntry : site.getCookies()
.entrySet()) {
Cookie cookie = new Cookie(cookieEntry.getKey(),
cookieEntry.getValue());
manage.addCookie(cookie);
}
}
WebElement webElement = webDriver.findElement(By.xpath("/html"));
String content = webElement.getAttribute("outerHTML");
return content;
}
private void checkInit() {
if (webDriverPool == null) {
synchronized (this) {
webDriverPool = new WebDriverPool(poolSize);
}
}
}
@Override
public void setThread(int thread) {
this.poolSize = thread;
}
@Override
public void close() throws IOException {
webDriverPool.closeAll();
}
}
config.ini配置文件:
# What WebDriver to use for the tests
driver=phantomjs
#driver=firefox
#driver=chrome
#driver=http://localhost:8910
#driver=http://localhost:4444/wd/hub
# PhantomJS specific config (change according to your installation)
#phantomjs_exec_path=/Users/Bingo/bin/phantomjs-qt5
phantomjs_exec_path=/Users/Bingo/Downloads/phantomjs-1.9.8-macosx/bin/phantomjs
#phantomjs_driver_path=/Users/Bingo/Documents/workspace/webmagic/webmagic-selenium/src/main.js
phantomjs_driver_loglevel=DEBUG
基于selenium实现动态爬取页面(java)
参考文章:https://blog.csdn.net/qq_22003641/article/details/79137327?spm=1001.2014.3001.5506
前言
使用selenium进行爬虫,需要下载相关浏览器的驱动程序,和添加selenium相关的jar包。
浏览器驱动程序地址:
Firefox浏览器驱动:Firefox
Chrome浏览器驱动:chrome淘宝镜像
Edge浏览器驱动:edge
selenium相关依赖:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.4.0</version>
</dependency>
jar包下载地址:https://mvnrepository.com/
一、Selenium是什么?
Selenium是一个用于web应用程序测试的工具。Selenium测试直接运行在浏览器中,就像真正的用户在操作一样。Selenium完全开源,对商业用户也没有任何限制,支持分布式,拥有成熟的社区与学习文档。使用Selenium实现爬虫最大的好处在于能够模拟一个用户正常访问页面,网站后台不容易检测出来,而且使用简单,可以使用java或python等多种编程语言编写用例脚本。
二、Selenium常见的API
1.Selenium定位的方法
Selenium提供了8种定位方式,这些方式在Java中所对应的方法如下:
id-------------------------------------------------------------findElement(By.id())
name--------------------------------------------------------findElement(By.name())
class name------------------------------------------------findElement(By.className())
tag name--------------------------------------------------findElement(By.tagName())
link text----------------------------------------------------findElement(By.linkText())
partial link text-------------------------------------------findElement(By.partialLinkText())
xpath-------------------------------------------------------findElement(By.xpath())
css selector----------------------------------------------findElement(By.cssSelector())
具体使用例子:
<html>
<head></head>
<body link="#0000cc">
<a id="result_logo" href="/" onmousedown="return c({'fm':'tab','tab':'logo'})">
<form id="form" class="fm" name="f" action="/s">
<span class="soutu-btn"></span>
<input id="kw" class="s_ipt" name="wd" value="" maxlength="255" autocomplete="off">
</form>
<a class="mnav" href="http://news.baidu.com" name="tj_trnews">新闻</a>
</body>
</html>
driver.findElement(By.id("kw"));//通过id定位的方式定位到input标签上面。
driver.findElement(By.name("wd"));//通过name定位方式定位到input标签上面。
driver.findElement(By.className("s_ipt"));//通过class name定位到input标签上面。
driver.findElement(By.tagName("input"))//通过tag name定位到input标签上面。
driver.findElement(By.xpath("//*[@id='kw']"));//通过xpath定位到input标签上面。
driver.findElement(By.cssSelector("#kw");//通过css定位到input标签上面。
driver.findElement(By.linkText("新闻");//通过link text定位到a标签上面。
driver.findElement(By.partialLinkText("新");//通过partialLink text定位到a标签上面。
提示:鼠标对准元素右键 > 检查 > 对准标签右键 > 点击copy,即可直接复制通过xpath或css等定位方式的路径。
2.控制浏览器窗口大小
maximize() 设置浏览器最大化
setSize() 设置浏览器宽高
例子:讲浏览器窗口的大小设置成(500*800)
driver.manage().window().setSize(new Dimension(480, 800));
3.控制浏览器后退、前进
在使用浏览器浏览网页时,浏览器提供了后退和前进按钮,可以方便地在浏览过的网页之间切换,WebDriver也提供了对应的back()和forward()方法来模拟后退和前进按钮。下面通过例子来演示这两个方法的使用。
back() 模拟浏览器后退按钮
forward() 模拟浏览器前进按钮
driver.navigate().back();
driver.navigate().forward();
4.刷新页面
有时候需要手动刷新(F5) 页面。
refresh() 刷新页面(F5)
driver.navigate().refresh();
三、WebDriver常用方法
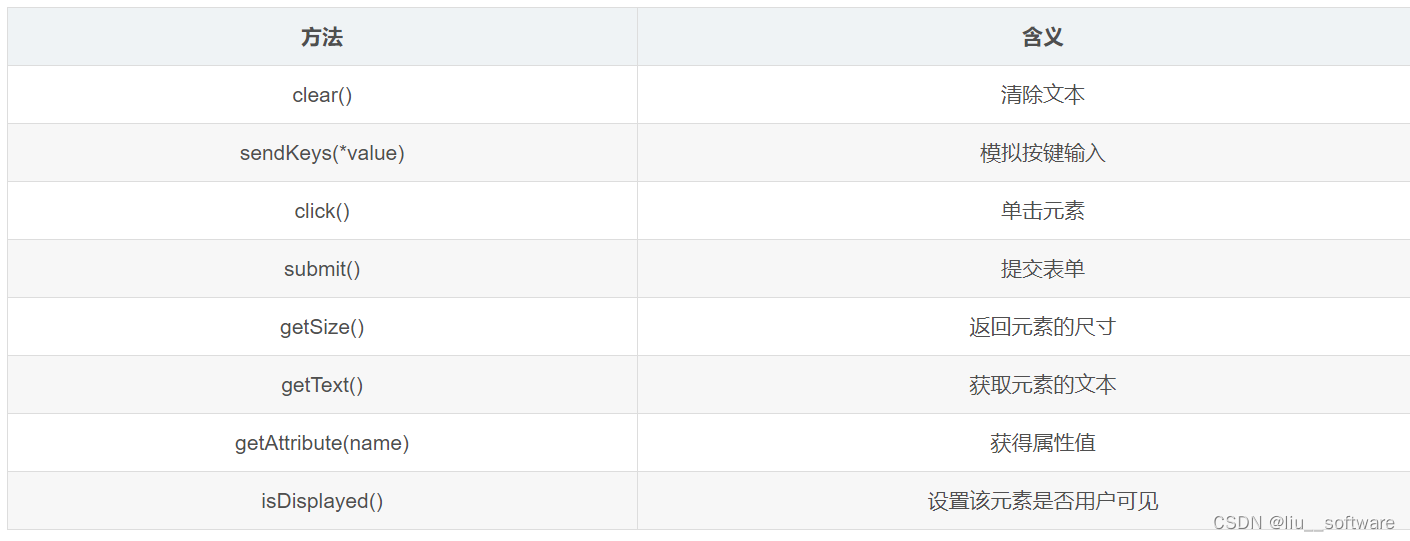
四、模拟鼠标的操作
五、模拟键盘操作
Keys()类提供了键盘上几乎所有按键的方法。 前面了解到, sendKeys()方法可以用来模拟键盘输入, 除此之 外, 我们还可以用它来输入键盘上的按键, 甚至是组合键, 如 Ctrl+A、 Ctrl+C 等。
例子:
input.sendKeys(Keys.SPACE);
input.sendKeys("教程");
Thread.sleep(2000);
input.sendKeys(Keys.CONTROL,"a");
Thread.sleep(2000);
六、获取断言信息

七、设置元素等待
WebDriver提供了两种类型的等待:显式等待和隐式等待。
显示等待
WebDriverWait类是由WebDirver提供的等待方法。在设置时间内,默认每隔一段时间检测一次当前页面元素是否存在,如果超过设置时间检测不到则抛出异常。具体格式如下:
WebDriverWait(driver, 10, 1)
driver: 浏览器驱动。 10: 最长超时时间, 默认以秒为单位。 1: 检测的的间隔(步长) 时间, 默认为 0.5s。
public static void main(String[]args) throws InterruptedException {
WebDriver driver = new ChromeDriver();
driver.get("https://www.baidu.com");
//显式等待, 针对某个元素等待
WebDriverWait wait = new WebDriverWait(driver,10,1);
wait.until(new ExpectedCondition<WebElement>(){
@Override
public WebElement apply(WebDriver text) {
return text.findElement(By.id("kw"));
}
}).sendKeys("selenium");
driver.findElement(By.id("su")).click();
Thread.sleep(2000);
driver.quit();
}
隐式等待
八、多表单切换
- driver.switchTo().frame(xf),xf为另一个表单的元素。
WebElement xf = driver.findElement(By.xpath("//*[@id='loginDiv']/iframe"));
driver.switchTo().frame(xf);
九、多窗口切换
switchTo().window()
getWindowHandle(): 获得当前窗口句柄。
getWindowHandles(): 返回的所有窗口的句柄到当前会话。
switchTo().window():用于切换到相应的窗口,与上一节的switchTo().frame()类似,前者用于不同窗口的切换, 后者用于不同表单之间的切换。
driver.switchTo().window(handle);
十、下拉框选择
<select id="nr" name="NR">
<option value="10" selected>每页显示 10 条</option>
<option value="20">每页显示 20 条</option>
<option value="50">每页显示 50 条</option>
<select>
public static void main(String[] args) throws InterruptedException {
WebDriver driver = new ChromeDriver();
driver.get("https://www.baidu.com");
driver.findElement(By.linkText("设置")).click();
driver.findElement(By.linkText("搜索设置")).click();
Thread.sleep(2000);
//<select>标签的下拉框选择
WebElement el = driver.findElement(By.xpath("//select"));
Select sel = new Select(el);
sel.selectByValue("20");
Thread.sleep(2000);
driver.quit();
}
十一、警告框处理
- getText(): 返回 alert/confirm/prompt 中的文字信息。
- accept(): 接受现有警告框。
- dismiss(): 解散现有警告框。
- sendKeys(keysToSend): 发送文本至警告框。
- keysToSend:将文本发送至警告框。
public static void main(String[] args) throws InterruptedException {
WebDriver driver = new ChromeDriver();
driver.get("https://www.baidu.com");
driver.findElement(By.linkText("设置")).click();
driver.findElement(By.linkText("搜索设置")).click();
Thread.sleep(2000);
//保存设置
driver.findElement(By.className("prefpanelgo")).click();
//接收弹窗
driver.switchTo().alert().accept();
Thread.sleep(2000);
driver.quit();
}
十二、浏览器cookie操作
getCookies() 获得所有 cookie 信息。
getCookieNamed(String name) 返回字典的key为“name”的Cookie信息。
addCookie(cookie dict) 添加Cookie。“cookie_dict”指字典对象,必须有 name和value值。
deleteCookieNamed(String name) 删除Cookie 信息。 “name”是要删除的 cookie的名称;“optionsString” 是该Cookie的选项,目前支持的选项包括“路径” , “域” 。
deleteAllCookies() 删除所有 cookie 信息。
public static void main(String[] args){
WebDriver driver = new ChromeDriver();
driver.get("https://www.baidu.com");
Cookie c1 = new Cookie("name", "key-aaaaaaa");
Cookie c2 = new Cookie("value", "value-bbbbbb");
driver.manage().addCookie(c1);
driver.manage().addCookie(c2);
//获得 cookie
Set<Cookie> coo = driver.manage().getCookies();
System.out.println(coo);
//删除所有 cookie
//driver.manage().deleteAllCookies();
driver.quit();
}
十三、调用JavaScript代码
虽然WebDriver提供了操作浏览器的前进和后退方法,但对于浏览器滚动条并没有提供相应的操作方法。在这种情况下,就可以借助JavaScript来控制浏览器的滚动条。WebDriver提供了executeScript()方法来执行JavaScript代码。
public static void main(String[] args) throws InterruptedException{
WebDriver driver = new ChromeDriver();
//设置浏览器窗口大小
driver.manage().window().setSize(new Dimension(700, 600));
driver.get("https://www.baidu.com");
//进行百度搜索
driver.findElement(By.id("kw")).sendKeys("webdriver api");
driver.findElement(By.id("su")).click();
Thread.sleep(2000);
//将页面滚动条拖到底部
((JavascriptExecutor)driver).executeScript("window.scrollTo(100,450);");
Thread.sleep(3000);
driver.quit();
}
注意事项
下载浏览器驱动程序时,尽量与自己电脑的浏览器版本一致或相近。
其实我也不知道为什么要这样,理论上来说下载的驱动程序应该不与电脑本身的浏览器有联系,但之前在爬虫时遇到一些问题,上网搜一下,基本上都是说驱动程序版本不一致。为了快速的实现功能,还是下载对应的版本驱动程序吧,避免后面出现问题。
————————————————
版权声明:本文为CSDN博主「润火」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。
原文链接:https://blog.csdn.net/weixin_45893787/article/details/124535153
转载于:Java 中使用 selenium 和 chrome 浏览器下载动态网页 - 简书