一、概念:继承于UIScrollView,可以滚动。每一条数据对应的单元格叫做cell,是UITableViewCell的一个对象,cell继承于UIView。UITableView可以分区显示,每一个分区称为section,每一行称为row,编号都从0开始。系统提供了一个专门的类来整合section和row,叫做NSIndexPath。
二、基本使用:
//创建一个表视图
UITableView *tableView = [[UITableView alloc] initWithFrame:[[UIScreen mainScreen] bounds] style:UITableViewStylePlain];
这个初始化方法中包含一个UITableViewStyle类型的参数,这是一个枚举类型
UITableViewStylePlain //regular table view
UITableViewStyleGrouped // preferences styletable view
//分割线样式
tableView.separatorStyle = UITableViewCellSeparatorStyleSingleLine; //单线
UITableViewCellSeparatorStyleNone//没有分割线
UITableViewCellSeparatorStyleSingleLineEtched// 只支持Grouped类型
//分割线颜色
tableView.separatorColor = [UIColor blackColor];
//UITableView的置顶视图
_tableView.tableHeaderView = _scrollView;
tableFooterView//UITableView的置底视图
//指定cell的高度
tableView.rowHeight = 150;
三、显示数据
//签订UITableView协议
@interface ViewController : UIViewController<UITableViewDataSource,UITableViewDelegate>
//tabView如果想要显示数据必须接受UITableViewDataSource,UITableViewDelegeae协议,并且实现协议中必须实现的两个方法
//设置当前的ViewController为UITableView代理
tableView.dataSource = self; // 设置dataSource代理
tableView.delegate = self; // 设置delegate代理
#pragma mark -----数据源协议中必须实现的方法-----
//UITableView每个分区包含的⾏数
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return [_dataArray[section] count];//返回数组容量
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return [_dataArray[section] count];//返回数组容量
}
//设置每行显示什么内容,也就是指定每一行的cell
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
//创建一个重用标识符(标识符可以有多个,以便取到不同类型的cell)
static NSString *reuseIdentitfier = @"reuse";
//表视图通过重用标识去重用池(可变集合NSMutableSet)中查找是否有能够被重用的cell
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
//创建一个重用标识符(标识符可以有多个,以便取到不同类型的cell)
static NSString *reuseIdentitfier = @"reuse";
//表视图通过重用标识去重用池(可变集合NSMutableSet)中查找是否有能够被重用的cell
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:reuseIdentitfier];
if (cell == nil) {
//创建一个cell
/*
cell样式有四种
default、subtitle、value1、value2
*/
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:reuseIdentitfier] autorelease];
cell.selectionStyle = UITableViewCellSelectionStyleNone;
}
//指定cell内部控件的显示内容
//创建一个cell
/*
cell样式有四种
default、subtitle、value1、value2
*/
cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:reuseIdentitfier] autorelease];
cell.selectionStyle = UITableViewCellSelectionStyleNone;
}
//指定cell内部控件的显示内容
//重用池中取出的cell,并没有释放,所以会保留原有的内容,如果想要显示自己的信息,需要对cell内部的控件进行重新赋值
//UITableView的每一个单元格是UITableViewCell类的对象,继承于UIView。UITableViewCell默认提供了3个视图属性:
cell.imageView.image = [UIImage imageNamed:@"mm.JPG
”
];
//设置头像的弧度
cell.imageView.layer.masksToBounds = YES;
cell.imageView.layer.cornerRadius = tableView.rowHeight / 2;
cell.imageView.layer.masksToBounds = YES;
cell.imageView.layer.cornerRadius = tableView.rowHeight / 2;
cell.textLabel.text = _dataArray[indexPath.section] [indexPath.row];
cell.detailTextLabel.text = @"葫芦娃";
return cell;
}
}
//UITableView包含多少分区
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return [_dataArray count];
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return [_dataArray count];
}
#pragma mark -----代理协议-----
//返回分区头的高度
- (CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section {
return 60;
- (CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section {
return 60;
}
//指定分区头显示的视图
- (UIView *)tableView:(UITableView *)tableView viewForHeaderInSection:(NSInteger)section {
switch (section) {
case 0:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"狗狗,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;}
case 1:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"葫芦娃,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;
}
default:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"七仙女,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;
}
}
- (UIView *)tableView:(UITableView *)tableView viewForHeaderInSection:(NSInteger)section {
switch (section) {
case 0:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"狗狗,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;}
case 1:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"葫芦娃,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;
}
default:{
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 0, 0)];
label.text = [NSString stringWithFormat:@"七仙女,分区:%ld",section];
label.textAlignment = NSTextAlignmentCenter;
return [label autorelease];
break;
}
}
}// custom view for header. will be adjusted to default or specified header height
//指定每一行的高度,也就是每一个cell的高度
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath {
switch (indexPath.section) {
case 0:
return 50;
break;
case 1:
return 100;
break;
default:
return 150;
break;
}
}
//指定右侧边栏索引
- (NSArray *)sectionIndexTitlesForTableView:(UITableView *)tableView {
return @[@"?",@"?",@"女"];
}
//点击单元格之后触发的方法
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
NSLog(@"%ld, %ld",indexPath.section, indexPath.row);
//跳转,传值都在这个方法内部完成
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath {
switch (indexPath.section) {
case 0:
return 50;
break;
case 1:
return 100;
break;
default:
return 150;
break;
}
}
//指定右侧边栏索引
- (NSArray *)sectionIndexTitlesForTableView:(UITableView *)tableView {
return @[@"?",@"?",@"女"];
}
//点击单元格之后触发的方法
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
NSLog(@"%ld, %ld",indexPath.section, indexPath.row);
//跳转,传值都在这个方法内部完成
}
//模型类异常处理的方法
- (void)setValue:(id)value forUndefinedKey:(NSString *)key {
//内部什么都不做,异常处理,发现photo时,直接过掉
- (void)setValue:(id)value forUndefinedKey:(NSString *)key {
//内部什么都不做,异常处理,发现photo时,直接过掉
}
四、重用机制
tableview每次要显示一个cell都会调用- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath;方法获取
UITableView有⼀个重⽤ 机池机制管理cell, ⽬的 是使⽤尽可能少的cell显 ⽰所有数据。
1. 当⼀个cell被滑出屏幕,这个cell会被系统放到相应的重⽤池中。
2.当tableView需要显⽰⼀个cell,会先去重⽤池中尝试获取⼀个 cell。
3.如果重⽤池没有cell,就会创建⼀个cell。
4.取得cell之后会重新赋值进⾏使⽤。
在创建UITableView之后,需要注册⼀个cell类,当重⽤池中没有 cell的时候,系统可以⾃动创建cell。 相关⽅法:
- (void)registerClass:(Class)cellClass forCellReuseIdentifier: (NSString *)identifier;
.系统提供了⼀个获取重⽤池中cell的⽅法(需要提供⼀个重⽤标 识):
- (UITableViewCell *)dequeueReusableCellWithIdentifier:(NSString *)identifier;
//参数1:当重用池没有cell的时候使用什么类创建cell
//参数2:这个重用池的标识符
//参数2:这个重用池的标识符
[tableView registerClass:<#(Class)#> forCellReuseIdentifier:@"reuse"];
五、其他常用协议方法
//分区顶部标题
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section {
NSString *title = [NSString stringWithFormat:@"分区顶部标题,分区:%ld",section];
return title;
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section {
NSString *title = [NSString stringWithFormat:@"分区顶部标题,分区:%ld",section];
return title;
}
//分区底部标题
- (NSString *)tableView:(UITableView *)tableView titleForFooterInSection:(NSInteger)section {
NSString *foot = [NSString stringWithFormat:@"分区底部标题,分区:%ld",section];
return foot;
- (NSString *)tableView:(UITableView *)tableView titleForFooterInSection:(NSInteger)section {
NSString *foot = [NSString stringWithFormat:@"分区底部标题,分区:%ld",section];
return foot;
}
//数据解析,将模型数据添加到模型数组中
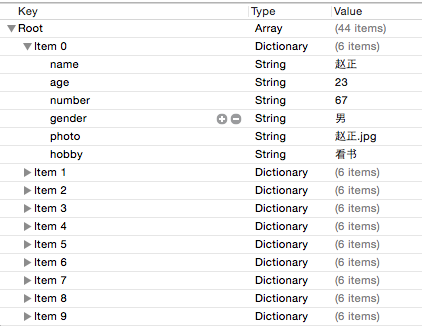
- (void)handleData {
NSString *filePath = [[NSBundle mainBundle] pathForResource:@"Student" ofType:@"plist"];
NSArray *array = [NSArray arrayWithContentsOfFile:filePath];
_dataArray = [[NSMutableArray alloc] initWithCapacity:0];
for (NSDictionary *dic in array) {
Student *student = [[Student alloc] init];
[student setValuesForKeysWithDictionary:dic];
[_dataArray addObject:student];
[student release];
}
NSArray *array = [NSArray arrayWithContentsOfFile:filePath];
_dataArray = [[NSMutableArray alloc] initWithCapacity:0];
for (NSDictionary *dic in array) {
Student *student = [[Student alloc] init];
[student setValuesForKeysWithDictionary:dic];
[_dataArray addObject:student];
[student release];
}
}