一.String的构造
1 package test; 2 3 public class StringCase { 4 5 /** 6 * @param args 7 **/ 8 public static void main(String[] args) { 9 10 11 char a[] = {'J','a','r','v','i','s'}; 12 //第一种 13 String s1 = new String(a);//直接等于String("good"); 14 System.out.println("Jarvis Birthday!!!" + " " + s1); 15 int b = 1; 16 System.out.println(""+b); 17 System.out.println(a); 18 System.out.println(""+a); 19 //第二种 String s = new (char [],int first,int last); 20 21 String s2 = new String(a,2,4); 22 23 System.out.println(s2); 24 25 //第三种,来源于同一条字符串的String的比较; 26 String sc1,sc2; 27 sc1 = "We are Jarvis"; 28 sc2 = "We are Jarvis"; 29 30 if(sc1 == sc2) 31 System.out.println("yes"); 32 else 33 System.out.println("no"); 34 35 //此处的sc1 与 sc2 的 比较,之所以为yes是由于两个对象指向的是同一实体!!!!!一定要明确!!! 36 } 37 38 }
注意点:
1)第三行的输出解释很重要!!!!(感谢cch同学指导)
1 public void println(Object x) { 2 String s = String.valueOf(x); 3 synchronized (this) { 4 print(s); 5 newLine(); 6 } 7 }
1 public static String valueOf(Object obj) { 2 return (obj == null) ? "null" : obj.toString(); 3 }
println(""+a);此处隐式调用了toString 函数。
值得注意的是,a虽然是字符串数组,存的在C++/C中是地址,但是要记住,Java虚拟机中是不允许直接调用地址的!!!!
java中大多变量(也可能是所有变量),分为引用类型等等,此处的a是引用类型,而object是所有类型的父类,一般的将,所有的类型都需要将toString 函数重写!!!
toString函数
而char a[]中并没有重写此函数。
但是问题来了:如果说System.println(a);那么输出是正常的,这是为什么???
此问题带补充
2)sc1 与 sc2 对同一字符串常量进行了构造函数,为啥两者就相同了?这个与c++的处理机制有何不同?
另外java中处理不了char c[] = "We are Jarvis";么?------是的!!!java中字符串变量就是String类型,""就是字符串类型。而char c[]是char类型!
String sc1 = new String("Jarvis"); String sc2 = new String("Jarvis"); System.out.println(sc1 == sc2);
此处结果输出为false!!!!
1 public static void main(String[] args) { 2 3 4 //1连接多个字符串 5 String s1 = new String("hello"); 6 String s2 = new String("Jarvis"); 7 8 String s = s1 + " " + s2; 9 System.out.println(s); 10 11 //2连接其他类型数据 12 int a = 42; 13 System.out.println("I am Mark"+a); 14 15 }
三.获取字符串信息
1 package test; 2 3 public class StringCase { 4 5 /** 6 * @param args 7 **/ 8 public static void main(String[] args) { 9 10 11 //1获取字符串长度 12 String s2 = new String("Jarvis"); 13 System.out.println(s2.length()); 14 //2字符串查找 15 16 //indexOf返回的是第一次出现的位置 17 String s1 = new String("Jarvis Java"); 18 System.out.println(s1.indexOf(1)); 19 System.out.println(s1.indexOf(97));//int char 是查找asscII码 20 System.out.println(s1.indexOf("a"));//subString 21 System.out.println(s1.indexOf('a'));//char 类型字符出现的位置---->实际上通过多态实现 22 23 //lastIndexOf(subStr/int) 24 25 String str = "We are Jarvis"; 26 System.out.println(str.lastIndexOf("a")); 27 System.out.println(str.lastIndexOf(97)); 28 System.out.println(str.lastIndexOf("")+" "+str.length());//实际上输出的是其长度 29 30 //3 指定索引位置的字符 31 System.out.println(str.charAt(1));//注意全都是从0开始的哦 32 33 34 } 35 36 }
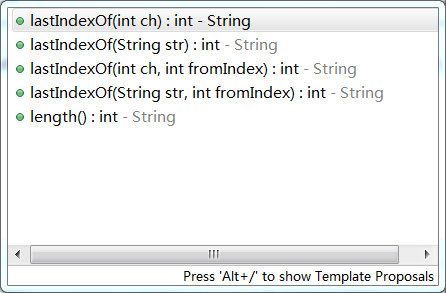
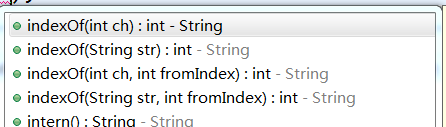
四.字符串操作
1 //1获取字符串长度 2 String s1 = "Jarvis is Jarvis's AI cpp program"; 3 System.out.println(s1.substring(3)); 4 System.out.println(s1.substring(3,10));
1 //2 trim()去除两边空格 2 String s1 = " Jarvis is Jarvis's AI cpp program "; 3 System.out.println(s1); 4 System.out.println(s1.trim());
1 public static void main(String[] args) { 2 3 //3 replace(char old,char new); ----->原字符串并不改变 4 String s1 = " Jarvis is Jarvis's AI cpp program "; 5 s1 = s1.trim(); 6 s1.replace("a", "A"); 7 System.out.println(s1); 8 String s2 = s1.replace('a', 'A'); 9 System.out.println(s2); 10 }
1 s1.startsWith("a");//返回的都是布尔类型 2 s1.endsWith("m");
5 判断其是否相等
返回类型是布尔类型
str.equals(String str2);
str.equalsIgnoreCase(anotherString);忽略大小写
注意str1 == str2 比较的是是不是一个实体!!!
6 大小写转换
1 public static void main(String[] args) { 2 3 //3 replace(char old,char new); ----->原字符串并不改变 4 String s1 = " Jarvis is Jarvis's AI cpp program "; 5 s1.toLowerCase(); 6 String s2 = s1.toLowerCase(); 7 System.out.println(s1); 8 System.out.println(s2); 9 System.out.println(s1.toUpperCase()); 10 }
7 大小比较
str.compareTo(String str2);
8 字符分割
str.split(String sign);
str.split(String sign,int limit);
1 public static void main(String[] args) { 2 3 4 String s1 = "____Jarvis_is_Jarvis's_AI_cpp_program____"; 5 String arr[] = s1.split("_"); 6 for(int i = 0;i < arr.length;i++) 7 System.out.println(arr[i]); 8 System.out.println("end"); 9 10 String arr2[] = s1.split("_",8); 11 for(int i = 0;i < arr2.length;i++) 12 System.out.println(arr2[i]); 13 }
此处前面多出空字符串需要留意!!!!注意里面的实现
String类的 输入!!!!
1 public static void main(String[] args) { 2 3 Scanner input = new Scanner(System.in); 4 5 String s1 = input.next(); 6 String s2 = input.next(); 7 System.out.println(s1); 8 System.out.println(s2); 9 10 s1 = input.nextLine(); 11 12 System.out.println(s1); 13 14 15 }
六 String向基本类型的转换:
1 String s1 = "4"; 2 String s2 = "4.5"; 3 int a; 4 double b; 5 a = Integer.parseInt(s1); 6 b = Double.parseDouble(s2); 7 System.out.println(a); 8 System.out.println(b);
1 //a = Integer.parseInt(s2); 这样是会报错的。 2 //System.out.println(a); 3 //System.out.println(s1);
七 对话框输入
1 String input = JOptionPane.showInputDialog("ENTER AN INT"); 2 int a; 3 double b; 4 a = Integer.parseInt(input); 5 JOptionPane.showMessageDialog(null, a);//""+a也是可以的,一般建立一个字符串然后加上一个数字