iOS中用系统提供的API能实现能实现文件的上传与下载,分别有两种方式。NSURLConnection与NSURLSession。
其中NSURLConnection是使用很久的的一种方式,NSURLSession是新出来的一种方式。
文件上传
一、 POST方式上传
POST方式提交信息默认使用的是
:
*
Content-Type
:
application/x-www-form-urlencoded
.
*
输入中文时
,
post
方式自动进行转义
(
苹果中自动
).
国内的绝大多数网站都采用这种方式
上传文件
(
支持二进制文件
)
*
Content-Type
:
multipart/form-data
(
上传文件
)
*
都会限制上传文件的大小
一般是
2M
或者更小。
在苹果中进行上传操作十分麻烦。需要拼接好上传所需要的字符串格式,然后才能实现上传。(还要加上头部)
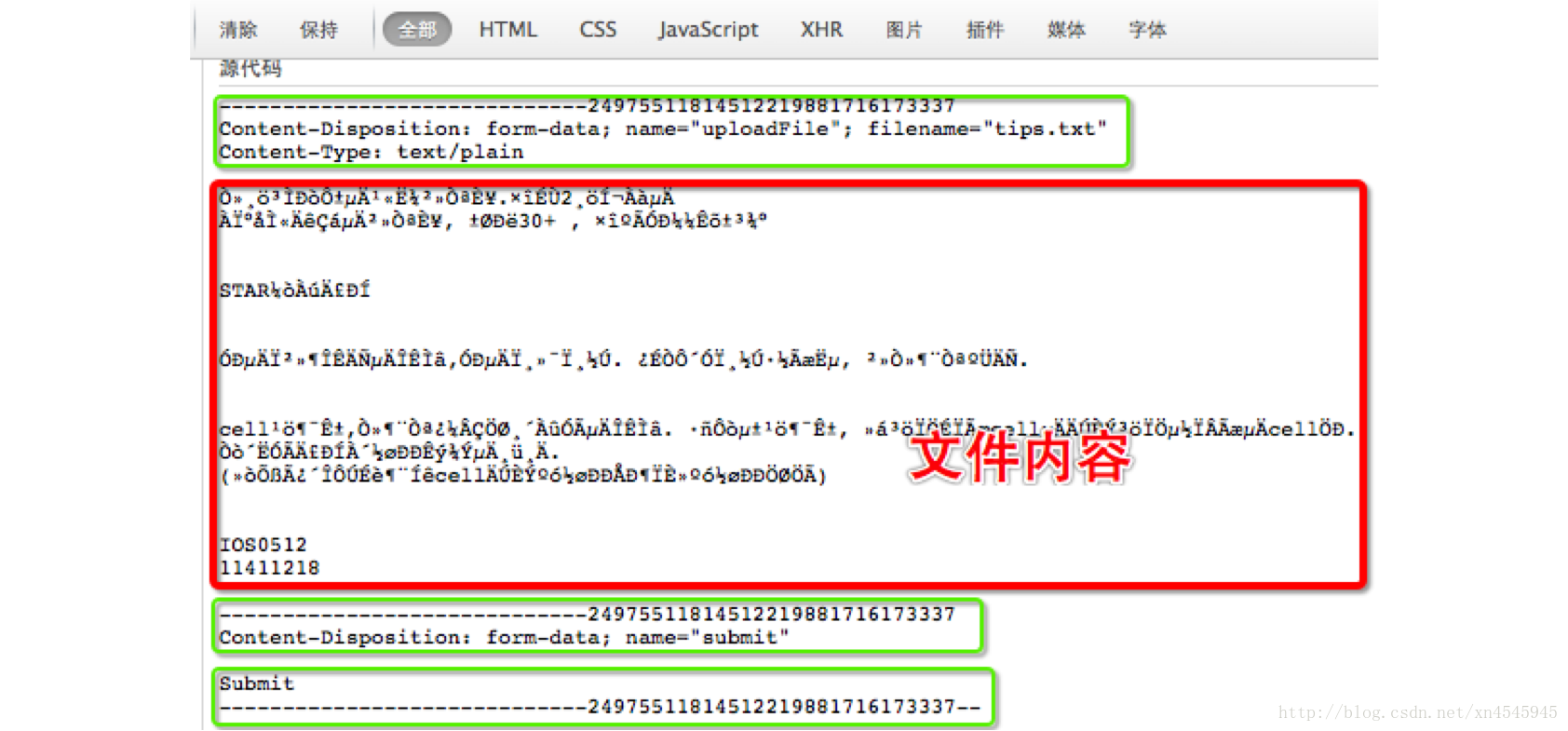
其他平台做的好一点的可能封装好了,不需要自己拼接字符串格式。因此iOS中很少用这种方式上传。但是AFNetworking很好地封装了POST请求,也很好用。
示例代码:
- #import "XNUploadFile.h"
-
- #define kTimeOut 5.0f
-
- @implementation XNUploadFile
-
- static NSString *boundaryStr = @"--";
-
- static NSString *randomIDStr;
-
- static NSString *uploadID;
-
- - (instancetype)init
- {
- self = [super init];
- if (self) {
-
- randomIDStr = @"itcastupload";
-
-
-
- uploadID = @"uploadFile";
- }
- return self;
- }
-
- #pragma mark - 成员方法. 用NSURLSession来完成上传
- - (void)uploadFile:(NSString *)path fileName:(NSString *)fileName completion:(void (^)(NSString *string))completion
- {
-
- NSURL *url = [NSURL URLWithString:@"http://localhost/new/post/upload.php"];
-
-
- NSURLRequest *request = [self requestForUploadURL:url uploadFileName:fileName localFilePath:path];
-
-
-
- NSURLSession *session = [NSURLSession sharedSession];
-
-
-
- [[session dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
-
- id result = [NSJSONSerialization JSONObjectWithData:data options:0 error:NULL];
-
- NSLog(@"%@ %@", result, [NSThread currentThread]);
-
- dispatch_async(dispatch_get_main_queue(), ^{
- if (completion) {
- completion(@"下载完成");
- }
- });
- }] resume];
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- }
-
- #pragma mark - 私有方法 : 拼字符串
-
- - (NSString *)topStringWithMimeType:(NSString *)mimeType uploadFile:(NSString *)uploadFile
- {
- NSMutableString *strM = [NSMutableString string];
-
- [strM appendFormat:@"%@%@\n", boundaryStr, randomIDStr];
- [strM appendFormat:@"Content-Disposition: form-data; name=\"%@\"; filename=\"%@\"\n", uploadID, uploadFile];
- [strM appendFormat:@"Content-Type: %@\n\n", mimeType];
-
- NSLog(@"顶部字符串:%@", strM);
- return [strM copy];
- }
-
-
- - (NSString *)bottomString
- {
- NSMutableString *strM = [NSMutableString string];
-
- [strM appendFormat:@"%@%@\n", boundaryStr, randomIDStr];
- [strM appendString:@"Content-Disposition: form-data; name=\"submit\"\n\n"];
- [strM appendString:@"Submit\n"];
- [strM appendFormat:@"%@%@--\n", boundaryStr, randomIDStr];
-
- NSLog(@"底部字符串:%@", strM);
- return [strM copy];
- }
-
-
- - (NSString *)mimeTypeWithFilePath:(NSString *)filePath
- {
-
- if (![[NSFileManager defaultManager] fileExistsAtPath:filePath]) {
- return nil;
- }
-
-
- NSURL *url = [NSURL fileURLWithPath:filePath];
- NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url];
-
-
- NSURLResponse *response = nil;
- [NSURLConnection sendSynchronousRequest:request returningResponse:&response error:NULL];
-
- return response.MIMEType;
- }
-
-
- - (NSURLRequest *)requestForUploadURL:(NSURL *)url uploadFileName:(NSString *)fileName localFilePath:(NSString *)filePath
- {
-
- NSString *mimeType = [self mimeTypeWithFilePath:filePath];
- if (!mimeType) return nil;
-
-
- NSMutableData *dataM = [NSMutableData data];
- [dataM appendData:[[self topStringWithMimeType:mimeType uploadFile:fileName] dataUsingEncoding:NSUTF8StringEncoding]];
-
- [dataM appendData:[NSData dataWithContentsOfFile:filePath]];
- [dataM appendData:[[self bottomString] dataUsingEncoding:NSUTF8StringEncoding]];
-
-
- NSMutableURLRequest *requestM = [NSMutableURLRequest requestWithURL:url cachePolicy:0 timeoutInterval:kTimeOut];
-
- requestM.HTTPMethod = @"POST";
-
- requestM.HTTPBody = dataM;
-
- NSString *typeStr = [NSString stringWithFormat:@"multipart/form-data; boundary=%@", randomIDStr];
- [requestM setValue:typeStr forHTTPHeaderField:@"Content-Type"];
-
- NSString *lengthStr = [NSString stringWithFormat:@"%@", @([dataM length])];
- [requestM setValue:lengthStr forHTTPHeaderField:@"Content-Length"];
-
- return [requestM copy];
- }
注意:
POST
上传时
,
是
不允许重名
的
.
(
否则出错
)
二、 PUT方式上传
session
中的
upload
方法只能用于
PUT
上传
,
不能用于
POST
上传
.
用
PUT
方式上传的
好处
:
(
需要身份验证
)
*
不用像
POST
一样
,
拼一堆字符串
.
*
直接
base64
编码一下身份验证
,
session
的
upload
一调用就行了
.
*
没有文件大小限制
.
*
即时通讯
里面用的多
.
(
发图片
/
发语音
)
- - (void)putFile
- {
-
- NSURL *url = [NSURL URLWithString:@"http://localhost/uploads/abcd"]; //abcd为文件名
-
-
- NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url];
- request.HTTPMethod = @"PUT";
-
-
-
-
-
-
- NSString *authStr = @"admin:123";
- NSString *authBase64 = [NSString stringWithFormat:@"Basic %@", [self base64Encode:authStr]];
- [request setValue:authBase64 forHTTPHeaderField:@"Authorization"];
-
-
- NSURLSession *session = [NSURLSession sharedSession];
-
-
- NSURL *localURL = [[NSBundle mainBundle] URLForResource:@"001.png" withExtension:nil];
- [[session uploadTaskWithRequest:request fromFile:localURL completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
-
- NSString *result = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
-
- NSLog(@"sesult---> %@ %@", result, [NSThread currentThread]);
- }] resume];
- }
-
- - (NSString *)base64Encode:(NSString *)str
- {
-
- NSData *data = [str dataUsingEncoding:NSUTF8StringEncoding];
-
-
- NSString *result = [data base64EncodedStringWithOptions:0];
-
- NSLog(@"base464--> %@", result);
-
- return result;
- }
PUT方式与DELETE对应,DELETE用于删除PUT方式上传的文件。
TIPS:session使用注意
*
网络会话
,
方便程序员使用网络服务
.
*
如
:
可以获得当前上传文件的进度
.
*
NSURLSession
的任务
,
默认都是
异步
的
.
(
在其他线程中工作
)
*
Task
是由
会话
发起的
.
*
注意网络请求都要进行出错处理
.
*
session
默认是挂起的
,
需要
resume
一下才能启动
.
文件下载
文件的下载分为NSURLConnection与NSURLSession两种,前一种有恨悠久的历史了。使用相对麻烦,后者是新出来的,增加了一些额外的功能。
一、NSURLConnection实现下载
TIPS:
1、
当
NSURLConnection
下载时
,
得到的
NSData
写入文件
时
,
data
并没有
占用多大内存
.
(
即使文件很大
)
2、
一点点在传
.
做的是磁盘缓存
.
而不是内存缓存机制。
3、了解在
NSURLConnection
上加代理。
[con
setDelegateQueue
:[[NSOperationQueue
alloc]
init]]
4、
NSURLResponse
记录的了
url, mineType, exceptedContentLength, suggestedFileName
等属性
.
下载时用得着
.
以下程序实现追踪下载百分比的下载(URLConnection自带的方法):
- #import "XNDownload.h"
-
- typedef void(^ProgressBlock)(float percent);
-
- @interface XNDownload() <NSURLConnectionDataDelegate>
-
- @property (nonatomic, strong) NSMutableData *dataM;
-
-
- @property (nonatomic, strong) NSString *cachePath;
-
- @property (nonatomic, assign) long long fileLength;
-
- @property (nonatomic, assign) long long currentLength;
-
-
- @property (nonatomic, copy) ProgressBlock progress;
-
- @end
-
- @implementation XNDownload
-
- - (NSMutableData *)dataM
- {
- if (!_dataM) {
- _dataM = [NSMutableData data];
- }
- return _dataM;
- }
-
- - (void)downloadWithURL:(NSURL *)url progress:(void (^)(float))progress
- {
-
- self.progress = progress;
-
-
- NSURLRequest *request = [NSURLRequest requestWithURL:url];
-
-
- NSURLConnection *connection = [NSURLConnection connectionWithRequest:request delegate:self];
-
-
-
- [connection setDelegateQueue:[[NSOperationQueue alloc] init]];
-
-
- [connection start];
- }
-
- #pragma mark - 代理方法
-
- - (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
- {
- NSLog(@"%@ %lld", response.suggestedFilename, response.expectedContentLength);
-
- NSString *cachePath = [NSSearchPathForDirectoriesInDomains(NSCachesDirectory, NSUserDomainMask, YES) lastObject];
- self.cachePath = [cachePath stringByAppendingPathComponent:response.suggestedFilename];
-
- self.fileLength = response.expectedContentLength;
-
- self.currentLength = 0;
-
-
- [self.dataM setData:nil];
- }
-
-
- - (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
- {
-
- [self.dataM appendData:data];
-
-
- self.currentLength += data.length;
-
- float progress = (float)self.currentLength / self.fileLength;
-
-
- if (self.progress) {
- [[NSOperationQueue mainQueue] addOperationWithBlock:^{
-
- [[NSRunLoop currentRunLoop] runMode:NSDefaultRunLoopMode beforeDate:[NSDate date]];
-
- self.progress(progress);
- }];
- }
- }
-
-
- - (void)connectionDidFinishLoading:(NSURLConnection *)connection
- {
- NSLog(@"%s %@", __func__, [NSThread currentThread]);
-
-
- [self.dataM writeToFile:self.cachePath atomically:YES];
- }
-
-
- - (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
- {
- NSLog(@"%@", error.localizedDescription);
- }
-
- @end
二、NSURLSession实现下载
NSURLSession能实现断点续传,暂停下载等功能。
1、session提供的是
开了多个线程的异步下载.
2、下载的暂停与
续传: (session的
代理中的方法)
*弄一个NSData变量来保存下载东西.暂停时将下载任务task清空.
*
续传:将暂停时的data交给session继续下载,并将先前的data清空.
3、
task
一定要
resume
才开始执行
.
出处:
http://blog.csdn.net/xn4545945