
package com.imooc.work;
public abstract class Graph {
public abstract void show();
}
package com.imooc.work;
public class Circle extends Graph {
private int r;
public Circle() {}
public Circle(int r) {
this.setR(r) ;
}
public int getR() {
return r;
}
public void setR(int r) {
this.r = r;
}
@Override
public void show() {
// TODO Auto-generated method stub
System.out.println("圆形,半径为"+this.getR());
}
}
package com.imooc.work;
public class Square extends Graph {
private int SideLength;
public int getSideLength() {
return SideLength;
}
public void setSideLength(int sideLength) {
SideLength = sideLength;
}
public Square() {}
public Square(int sideLength) {
super();
this.setSideLength(sideLength);
}
@Override
public void show() {
// TODO Auto-generated method stub
System.out.println("正方形,边长为"+this.getSideLength());
}
}
package com.imooc.work;
public class TestGraph {
public static void main(String[] args) {
getShape(0).show();
getShape(1).show();
getShape(2).show();
}
public static Graph getShape (int i) {
if(i==0){
return new Circle(1);
}else if(i==1){
return new Rectangle(3,2);
}else
return new Square(2);
}
}
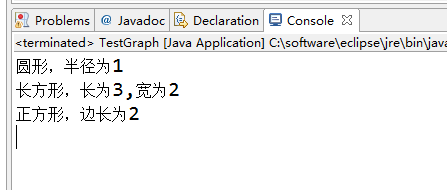