GoogleGear是Google发布的离线数据管理解决方案,也就是位于客户端浏览器中的DB,下面我们来看一看GoogleGear是如何使用的。
还是来看一看效果吧。
随意输入文字后,就可以将这个文字保存到GoogleGear的DB中。
怎么实现的呢?
看源代码:
提交给handleSummit函数,那么这个函数怎么样呢?看下面的代码:
首先是init.js:
正如注释所讲,这段代码只有一个函数,作用就是建立一个跨浏览器的GoogleFactory对象,我们后来用到的DB应该都是建立在这个对象之上的。
再看Sample.js:
这段代码的主要功能是提示用户如何查看源代码以及显示消息的,因此不必理会。
看完这两段,整体上就比较清楚了,首先是建立一个db对象,然后执行init方法,在init方法内部添加一个table,然后显示这个表中的记录。因为刚开始没有数据,所以会不显示什么,当再次登录这个页面的时候,就可以显示了,整个添加的代码非常类似JDBC的操作,对于Java程序员来说没有gap。
当用户提交输入时,触发添加操作。
因此整个例子展示了googleGear的添加、删除、查询功能,从这个例子里也可以看出,GoogleGear的理念是客户端的db,至于这个db的查询语言如何,现在还不是很好下结论。
还是来看一看效果吧。
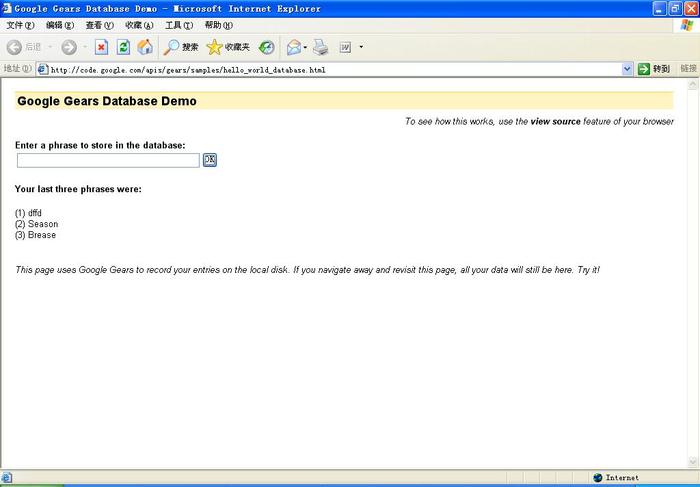
随意输入文字后,就可以将这个文字保存到GoogleGear的DB中。
怎么实现的呢?
看源代码:
- <form onsubmit="handleSubmit(); return false;">
- <b>Enter a phrase to store in the database:</b> <br>
- <table>
- <tr>
- <td valign="middle"><input type="text" id="submitValue"
- style="width:20em;"></td>
- <td valign="middle"><input type="submit" value="OK"></td>
- </tr>
- </table>
- </form>
- <script type="text/javascript" src="gears_init.js"></script>
- <script type="text/javascript" src="sample.js"></script>
- <script>
- var db;
- init();
- // Open this page's local database.
- function init() {
- var success = false;
- if (window.google && google.gears) {
- try {
- db = google.gears.factory.create('beta.database', '1.0');
- if (db) {
- db.open('database-demo');
- db.execute('create table if not exists Demo' +
- ' (Phrase varchar(255), Timestamp int)');
- success = true;
- // Initialize the UI at startup.
- displayRecentPhrases();
- }
- } catch (ex) {
- setError('Could not create database: ' + ex.message);
- }
- }
- // Enable or disable UI elements
- var inputs = document.getElementsByTagName('input');
- for (var i = 0, el; el = inputs[i]; i++) {
- el.disabled = !success;
- }
- }
- function handleSubmit() {
- if (!google.gears.factory || !db) {
- return;
- }
- var elm = document.getElementById('submitValue');
- var phrase = elm.value;
- var currTime = new Date().getTime();
- // Insert the new item.
- // The Gears database automatically escapes/unescapes inserted values.
- db.execute('insert into Demo values (?, ?)', [phrase, currTime]);
- // Update the UI.
- elm.value = '';
- displayRecentPhrases();
- }
- function displayRecentPhrases() {
- var recentPhrases = ['', '', ''];
- // We re-throw Gears exceptions to make them play nice with certain tools.
- // This will be unnecessary in a future version of Gears.
- try {
- // Get the 3 most recent entries. Delete any others.
- var rs = db.execute('select * from Demo order by Timestamp desc');
- var index = 0;
- while (rs.isValidRow()) {
- if (index < 3) {
- recentPhrases[index] = rs.field(0);
- } else {
- db.execute('delete from Demo where Timestamp=?', [rs.field(1)]);
- }
- ++index;
- rs.next();
- }
- rs.close();
- } catch (e) {
- throw new Error(e.message);
- }
- var status = document.getElementById('status');
- status.innerHTML = '';
- for (var i = 0; i < recentPhrases.length; ++i) {
- var bullet = '(' + (i + 1) + ') ';
- status.appendChild(document.createTextNode(bullet + recentPhrases[i]));
- status.appendChild(document.createElement('br'));
- }
- }
- </script>
首先是init.js:
- // Sets up google.gears.*, which is *the only* supported way to access Gears.
- //
- // Circumvent this file at your own risk!
- //
- // In the future, Gears may automatically define google.gears.* without this
- // file. Gears may use these objects to transparently fix bugs and compatibility
- // issues. Applications that use the code below will continue to work seamlessly
- // when that happens.
- (function() {
- // We are already defined. Hooray!
- if (window.google && google.gears) {
- return;
- }
- var factory = null;
- // Firefox
- if (typeof GearsFactory != 'undefined') {
- factory = new GearsFactory();
- } else {
- // IE
- try {
- factory = new ActiveXObject('Gears.Factory');
- } catch (e) {
- // Safari
- if (navigator.mimeTypes["application/x-googlegears"]) {
- factory = document.createElement("object");
- factory.style.display = "none";
- factory.width = 0;
- factory.height = 0;
- factory.type = "application/x-googlegears";
- document.documentElement.appendChild(factory);
- }
- }
- }
- // *Do not* define any objects if Gears is not installed. This mimics the
- // behavior of Gears defining the objects in the future.
- if (!factory) {
- return;
- }
- // Now set up the objects, being careful not to overwrite anything.
- if (!window.google) {
- window.google = {};
- }
- if (!google.gears) {
- google.gears = {factory: factory};
- }
- })();
正如注释所讲,这段代码只有一个函数,作用就是建立一个跨浏览器的GoogleFactory对象,我们后来用到的DB应该都是建立在这个对象之上的。
再看Sample.js:
- function setupSample() {
- // Make sure we have Gears. If not, tell the user.
- if (!window.google || !google.gears) {
- if (confirm("This demo requires Gears to be installed. Install now?")) {
- var spliceStart = location.href.indexOf("/samples");
- location.href =
- location.href.substring(0, spliceStart) + "/install.html";
- return;
- }
- }
- var viewSourceElem = document.getElementById("view-source");
- if (!viewSourceElem) {
- return;
- }
- var elm;
- if (navigator.product == "Gecko") {
- // If we're gecko, we can show the source of the application with the
- // view-source protocol.
- elm = document.createElement("a");
- elm.href = "view-source:" + location.href;
- elm.innerHTML = "View Demo Source";
- } else {
- // Otherwise, just tell users how to do it manually.
- elm = document.createElement("em");
- elm.innerHTML = "To see how this works, use the <strong>view "+
- "source</strong> feature of your browser";
- }
- viewSourceElem.appendChild(elm);
- }
- function checkProtocol() {
- if (location.protocol.indexOf('http') != 0) {
- setError('This sample must be hosted on an HTTP server');
- return false;
- } else {
- return true;
- }
- }
- function addStatus(s, opt_class) {
- var elm = document.getElementById('status');
- if (!elm) return;
- var node = document.createTextNode(s);
- if (opt_class) {
- var span = document.createElement('span');
- span.className = opt_class;
- span.appendChild(node);
- node = span;
- }
- elm.appendChild(node);
- elm.appendChild(document.createElement('br'));
- }
- function clearStatus() {
- var elm = document.getElementById('status');
- while (elm && elm.firstChild) {
- elm.removeChild(elm.firstChild);
- }
- }
- function setError(s) {
- clearStatus();
- addStatus(s, 'error');
- }
- setupSample();
看完这两段,整体上就比较清楚了,首先是建立一个db对象,然后执行init方法,在init方法内部添加一个table,然后显示这个表中的记录。因为刚开始没有数据,所以会不显示什么,当再次登录这个页面的时候,就可以显示了,整个添加的代码非常类似JDBC的操作,对于Java程序员来说没有gap。
当用户提交输入时,触发添加操作。
因此整个例子展示了googleGear的添加、删除、查询功能,从这个例子里也可以看出,GoogleGear的理念是客户端的db,至于这个db的查询语言如何,现在还不是很好下结论。