RESTful API 这玩意不用多说了,用Go做了个很简单的例子:
服务端在被调用时返回JSON,
客户端解析得到相关JSON信息.
服务端源码:
- package main
-
-
-
-
-
- import (
- "encoding/json"
- "fmt"
- "net/http"
- "time"
- )
-
- type Item struct {
- Seq int
- Result map[string]int
- }
-
- type Message struct {
- Dept string
- Subject string
- Time int64
- Detail []Item
- }
-
- func getJson() ([]byte, error) {
- pass := make(map[string]int)
- pass["x"] = 50
- pass["c"] = 60
- item1 := Item{100, pass}
-
- reject := make(map[string]int)
- reject["l"] = 11
- reject["d"] = 20
- item2 := Item{200, reject}
-
- detail := []Item{item1, item2}
- m := Message{"IT", "KPI", time.Now().Unix(), detail}
- return json.MarshalIndent(m, "", "")
- }
-
- func handler(w http.ResponseWriter, r *http.Request) {
- resp, err := getJson()
- if err != nil {
- panic(err)
- }
- fmt.Fprintf(w, string(resp))
- }
-
- func main() {
- http.HandleFunc("/", handler)
- http.ListenAndServe("localhost:8085", nil)
- }
服务端源码运行后,可在浏览器中执行看下效果:
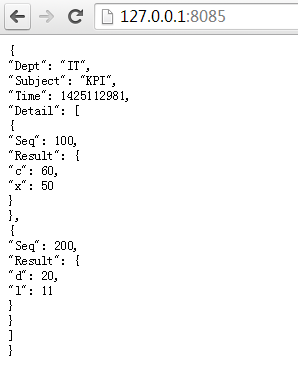
说明返回是正常的。再用Go写个调用程序测试下:
- package main
-
-
-
-
-
- import (
- "encoding/json"
- "fmt"
- "io/ioutil"
- "net/http"
- "time"
- )
-
- type Item struct {
- Seq int
- Result map[string]int
- }
-
- type Message struct {
- Dept string
- Subject string
- Time int64
- Detail []Item
- }
-
- func main() {
- url := "http://localhost:8085"
- ret, err := http.Get(url)
-
- if err != nil {
- panic(err)
- }
- defer ret.Body.Close()
-
- body, err := ioutil.ReadAll(ret.Body)
- if err != nil {
- panic(err)
- }
-
- var msg Message
- err = json.Unmarshal(body, &msg)
- if err != nil {
- panic(err)
- }
-
- strTime := time.Unix(msg.Time, 0).Format("2006-01-02 15:04:05")
- fmt.Println("Dept:", msg.Dept)
- fmt.Println("Subject:", msg.Subject)
- fmt.Println("Time:", strTime, "\n", msg.Detail)
- }
-
-
-
-
-
-
-
-
-
-
从运行结果中可以看到,已正确得到相关JSON信息。
from : http://blog.csdn.net/xcltapestry/article/category/1728845/1