In Struts 2, the dojo ajax tag “<sx:datetimepicker>” will render a text box and append a calender icon behind, click on the calender icon will prompt a date time picker component.
To create a date time pick component, just ensure :
1. Download the struts2-dojo-plugin.jar library.
2. Include the “struts-dojo-tags” tag and output its header.
开始时把struts2-dojo-plugin.jar包导入到项目WEB-INF/libx下
<%@ taglib prefix="sx" uri="/struts-dojo-tags" %>
<html>
<head>
<sx:head />
</head>
For example,
<%@ taglib prefix="s" uri="/struts-tags" %>
<%@ taglib prefix="sx" uri="/struts-dojo-tags" %>
<html>
<head>
<sx:head />
</head>
<body>
<sx:datetimepicker name="date2" label="Format (dd-MMM-yyyy)"
displayFormat="dd-MMM-yyyy" value="%{'2010-01-01'}"/>
...
Resulting the following HTML, few dojo and JavaScript libraries to create a date time pick component.
<html>
<head>
<script language="JavaScript" type="text/javascript">
// Dojo configuration
djConfig = {
isDebug: false,
bindEncoding: "UTF-8"
,baseRelativePath: "/Struts2Example/struts/dojo/"
,baseScriptUri: "/Struts2Example/struts/dojo/"
,parseWidgets : false
};
</script>
<script language="JavaScript" type="text/javascript"
src="/Struts2Example/struts/dojo/struts_dojo.js"></script>
<script language="JavaScript" type="text/javascript"
src="/Struts2Example/struts/ajax/dojoRequire.js"></script>
<link rel="stylesheet" href="/Struts2Example/struts/xhtml/styles.css" type="text/css"/>
<script language="JavaScript" src="/Struts2Example/struts/utils.js"
type="text/javascript"></script>
<script language="JavaScript" src="/Struts2Example/struts/xhtml/validation.js"
type="text/javascript"></script>
<script language="JavaScript" src="/Struts2Example/struts/css_xhtml/validation.js"
type="text/javascript"></script>
</head>
...
<td class="tdLabel">
<label for="widget_1291193434" class="label">Format (dd-MMM-yyyy):
</label></td>
<td>
<div dojoType="struts:StrutsDatePicker" id="widget_1291193434"
value="2010-01-01" name="date2" inputName="dojo.date2"
displayFormat="dd-MMM-yyyy" saveFormat="rfc"></div>
</td>
</tr>
<script language="JavaScript" type="text/javascript">
djConfig.searchIds.push("widget_1291193434");</script>
Struts 2 <S:datetimepicker> Example
A complete full example of the <s:datetimepicker> tag to generate a datetimepicker component, and show the use of OGNL and Java property to set the default date to the “datetimepicker” component.
1. Pom.Xml
Download the Struts 2 dojo dependency libraries.
pom.xml
//... <!-- Struts 2 --> <dependency> <groupId>org.apache.struts</groupId> <artifactId>struts2-core</artifactId> <version>2.1.8</version> </dependency> <!-- Struts 2 Dojo Ajax Tags --> <dependency> <groupId>org.apache.struts</groupId> <artifactId>struts2-dojo-plugin</artifactId> <version>2.1.8</version> </dependency> //...
2. Action Class
Action class to store the selected date.
DateTimePickerAction.java
package com.mkyong.common.action;
import java.util.Date;
import com.opensymphony.xwork2.ActionSupport;
public class DateTimePickerAction extends ActionSupport{
private Date date1;
private Date date2;
private Date date3;
//return today date
public Date getTodayDate(){
return new Date();
}
//getter and setter methods
public String execute() throws Exception{
return SUCCESS;
}
public String display() {
return NONE;
}
}
3. Result Page
Render the date time picker component via “<s:datetimepicker>” tag, set the default date via Java property and OGNL.
<%@ taglib prefix="sx" uri="/struts-dojo-tags" %>
<html>
<head>
<sx:head />
</head>
datetimepicker.jsp
<%@ taglib prefix="s" uri="/struts-tags" %>
<%@ taglib prefix="sx" uri="/struts-dojo-tags" %>
<html>
<head>
<sx:head />
</head>
<body>
<h1>Struts 2 datetimepicker example</h1>
<s:form action="resultAction" namespace="/" method="POST" >
<sx:datetimepicker name="date1" label="Format (dd-MMM-yyyy)"
displayFormat="dd-MMM-yyyy" value="todayDate" />
<sx:datetimepicker name="date2" label="Format (dd-MMM-yyyy)"
displayFormat="dd-MMM-yyyy" value="%{'2010-01-01'}"/>
<sx:datetimepicker name="date3" label="Format (dd-MMM-yyyy)"
displayFormat="dd-MMM-yyyy" value="%{'today'}"/>
<s:submit value="submit" name="submit" />
</s:form>
</body>
</html>
result.jsp
<%@ taglib prefix="s" uri="/struts-tags" %>
<html>
<body>
<h1>Struts 2 datetimepicker example</h1>
<h4>
Date1 : <s:property value="date1"/>
</h4>
<h4>
Date 2 : <s:property value="date2"/>
</h4>
<h4>
Date 3 : <s:property value="date3"/>
</h4>
</body>
</html>
3. Struts.Xml
Link it all ~
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <constant name="struts.devMode" value="true" /> <package name="default" namespace="/" extends="struts-default"> <action name="dateTimePickerAction" class="com.mkyong.common.action.DateTimePickerAction" method="display"> <result name="none">pages/datetimepicker.jsp</result> </action> <action name="resultAction" class="com.mkyong.common.action.DateTimePickerAction" > <result name="success">pages/result.jsp</result> </action> </package> </struts>
4. Demo
http://localhost:8080/Struts2Example/dateTimePickerAction.action
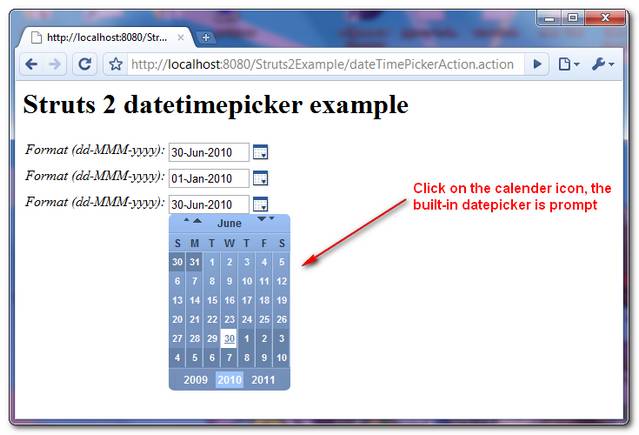
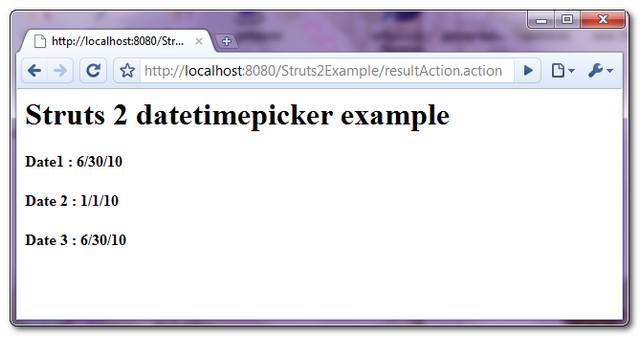