vue markdown
显示标记 (vue-mark-display)
A Vue Component to generate Markdown-based slides.
Vue组件,用于生成基于Markdown的幻灯片。
安装 (Installation)
npm install vue-mark-display
用法 (Usage)
<template>
<mark-display
:markdown="markdown"
@title="setTitle"
keyboard-ctrl
url-hash-ctrl
auto-font-size
auto-blank-target
></mark-display>
</template>
<script>
import MarkDisplay from "vue-mark-display";
const markdown = `# Hello World
----
This is Vue Mark Display`;
export default {
components: { MarkDisplay },
data() {
return { markdown };
},
methods: {
setTitle({ title }) {
document.title = title;
}
}
};
</script>
<style>
body {
margin: 0;
overflow: hidden;
}
</style>
Markdown语法的扩展 (The Extension for Markdown Syntax)
It's based on marked.
它基于标记 。
You can separate pages by horizon lines (
----
).您可以按水平线(
----
)分隔页面。You can use HTML comments for meta info of each slides. The format is like
<!-- key: value -->
. Here are all useful meta keys below:您可以将HTML注释用于每张幻灯片的元信息。 格式类似于
<!-- key: value -->
。 以下是所有有用的元键:background
: the background style of the slidebackground
:幻灯片的背景样式backgroundColor
: the background color of the slidebackgroundColor
:幻灯片的背景色backgroundImage
: URL of the background image of the slidebackgroundImage
:幻灯片背景图片的网址color
: the default font color of the slidecolor
:幻灯片的默认字体颜色style
: inline css text attached to the slidestyle
:附在幻灯片上的嵌入式CSS文字stageBackground
: the background style attached to the stage when the current slide is shownstageBackground
:显示当前幻灯片时附加到舞台的背景样式
Example:
例:
<!-- color: red; -->
<!-- style: font-weight: bold; -->
# Hello
---
<!-- stageBackground: silver -->
![./favicon.ico] this is content
API (API)
默认导出:组件 (Default Export: the Component)
道具 (Props)
{
// markdown content
markdown: String,
// or give a markdown URL
src: String,
// initial page number
page: Number,
// set `baseUrl` for the whole document
// so all the relative URLs in markdown content would be applied
baseUrl: String,
// whether use `src` as the `baseUrl` automatically
autoBaseUrl: Boolean,
// whether open links in a blank target by default
autoBlankTarget: Boolean,
// whether adjust font-size to adapt the screen size
autoFontSize: Boolean,
// whether support keyboard shortcuts (Arrows, Enter, Ctrl+G)
keyboardCtrl: Boolean,
// whether update URL hash when page changed
urlHashCtrl: Boolean,
// support opening an iframe on top of the page to preview a URL
// when click the `<a>` link with `altKey` pressed
supportPreview: Boolean
}
大事记 (Events)
@change="func({ from, to })"
: when page changed@change="func({ from, to })"
:页面更改时@title="func({ title })"
: when title changed@title="func({ title })"
:标题更改时
款式 (Styles)
The root element of the <mark-display>
component has a class named mark-display
which you can use for styling.
<mark-display>
组件的根元素具有一个名为mark-display
的类,可用于样式设置。
Also you can overwite the default transition style below on each slides:
您还可以在每张幻灯片上覆盖下面的默认过渡样式:
.slide {
position: absolute;
top: 0;
left: 0;
width: 100vw;
height: 100vh;
transition: all 0.3s;
}
.slide-enter {
opacity: 0;
}
.slide-leave-to {
opacity: 0;
}
To learn more about transitions on Vue, see here.
要了解有关Vue过渡的更多信息,请参见此处 。
At the end, it's a good choice to "normalizing" the body by your own:
最后, 通过自己的方法 “标准化”身体是一个不错的选择:
body {
margin: 0;
overflow: hidden;
}
And feel free to set other styles for common HTML tags as you like.
并且可以随意为常用HTML标签设置其他样式。
If you want to customize other styles in the component, please be careful. Because they are not guaranteed which means they may be changed in the future.
如果要在组件中自定义其他样式,请小心。 因为不能保证它们,这意味着它们将来可能会更改。
方法 (Methods)
With these methods you can call them outside through its ref
. For example on the bottom you can use them to support touchable screens.
使用这些方法,您可以通过ref
在外部调用它们。 例如,在底部,您可以使用它们来支持可触摸的屏幕。
goto(page: number)
: go to a given page which is counted from1
(not0
)goto(page: number)
:转到从1
(不是0
)开始计数的给定页面goNext()
: go to next page if possiblegoNext()
:如果可能,请转到下一页goPrev()
: go to previous page if possiblegoPrev()
:如果可能,请转到上一页goFirst()
: go to the first pagegoFirst()
:转到第一页goLast()
: go to the last pagegoLast()
:转到最后一页
命名为Export: setHighlighter()
(Named Export: setHighlighter()
)
Set a centralized code highlighter.
设置集中的代码突出显示器。
-
parameters:
参数:
highlighter: function (code: string, lang: string): string
: the customized code highlighter from youhighlighter: function (code: string, lang: string): string
:您自定义的代码荧光笔
-
examples:
例子:
Take highlight.js for example:
以Highlight.js为例:
import hljs from "highlight.js"; import "highlight.js/styles/github.css"; import { setHighlighter } from "vue-mark-display"; setHighlighter(code => hljs.highlightAuto(code).value);
对于触摸屏 (For Touchable Screen)
You can using some open source touch event libs to bring touch controls into the slides. For example the code below is using Hammer.js and methods goNext()
/goPrev()
to support swipe
gestures:
您可以使用一些开源的touch事件库将触摸控件引入幻灯片。 例如,以下代码使用Hammer.js和方法goNext()
/ goPrev()
来支持swipe
手势:
<template>
<mark-display ref="main" markdown="..." />
</template>
<script>
import Hammer from "hammerjs";
import MarkDisplay from "vue-mark-display";
export default {
components: { MarkDisplay },
mounted() {
const mc = new Hammer(this.$el);
const main = this.$refs.main;
mc.on("swipe", event => {
if (event.pointerType === 'mouse') {
return
}
switch (event.direction) {
case Hammer.DIRECTION_LEFT:
main.goNext();
return;
case Hammer.DIRECTION_RIGHT:
main.goPrev();
return;
}
});
},
}
</script>
导出成PDF (Export into PDF)
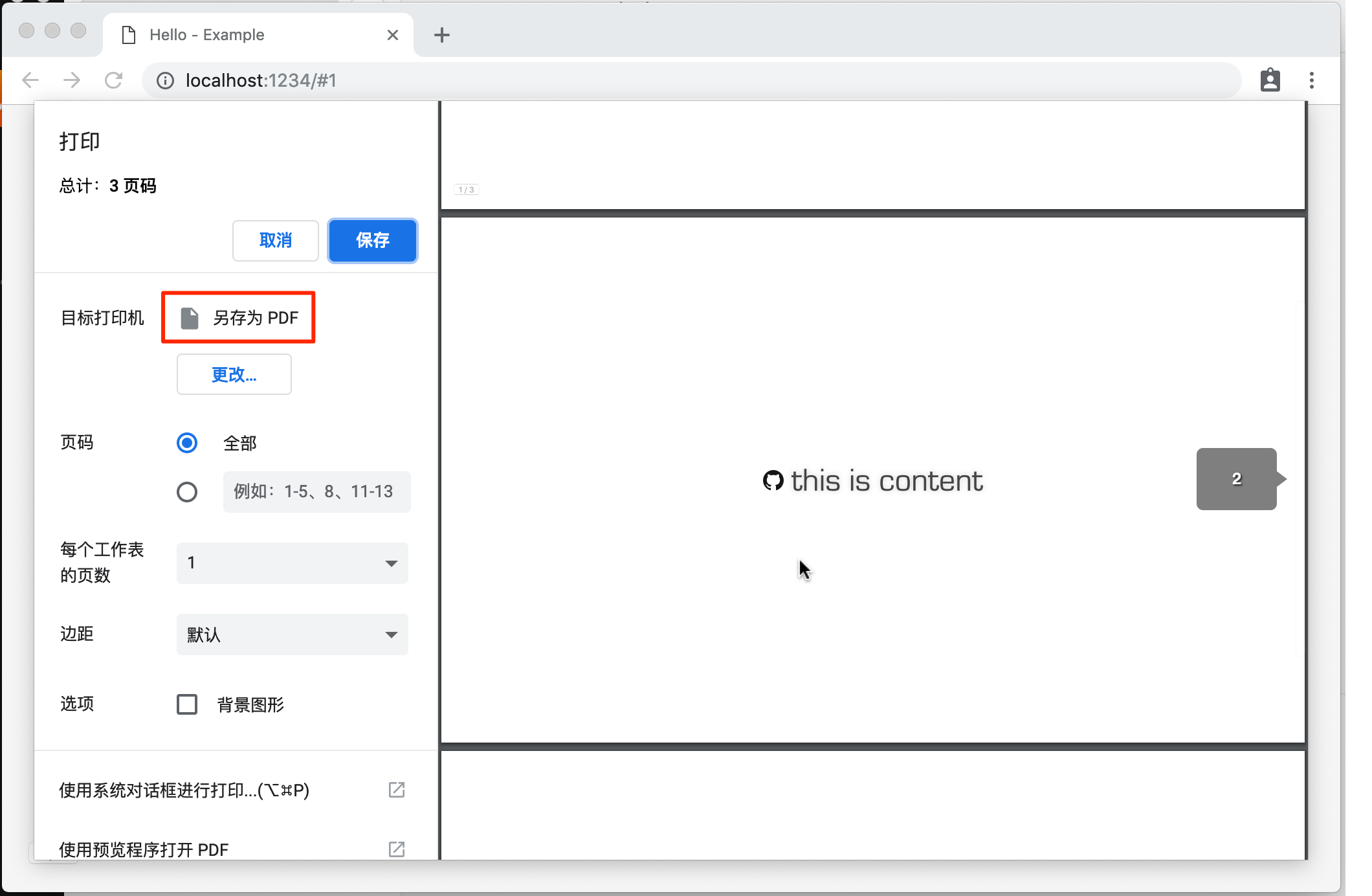
You can print the slides simply by CMD+P. The page style has been automatically formated by CSS page media & fragmentation specs under the hook. Then just select "export to PDF" on the print dialog to finish it. That makes your slides easy to export and share on/off the internet.
您只需通过CMD + P即可打印幻灯片。 该页面样式已通过钩子下的CSS页面媒体和碎片规范自动设置了格式。 然后只需在打印对话框中选择“导出为PDF”即可完成。 这样一来,您的幻灯片就可以轻松导出并在Internet上/在Internet外共享。
Notice that when you print the slides, only current screen-displayed stageBackground
meta info is active. So it would be applied to all pages of the exported PDF.
请注意,在打印幻灯片时,只有当前屏幕显示的stageBackground
元信息处于活动状态。 因此,它将应用于导出的PDF的所有页面。
翻译自: https://vuejsexamples.com/a-vue-component-for-markdown-based-slides/
vue markdown