Vue广告表树 (vue-ads-table-tree)
Vue ads table tree is a vue js table component, with a tree functionality.
Vue ads表树是vue js表组件,具有树功能。
The default features of a table component like filtering, sorting and paginating are implemented. On top of that, you can add a tree structure: Rows can have child rows, that can be hidden or expanded.
表组件的默认功能(如过滤,排序和分页)已实现。 最重要的是,您可以添加树形结构:行可以具有子行,可以隐藏或扩展。
Another cool feature, is the choice to load your rows in advance or via an async call to the backend. You can even load a small part of your data, for example the first page, and then load all the other pages with async calls to the backend.
另一个很酷的功能是选择预先加载行或通过对后端的异步调用来加载行。 您甚至可以加载一小部分数据(例如第一页),然后使用对后端的异步调用来加载其他所有页面。
It uses the handy tailwind css library for styling.
它使用方便的顺风CSS库进行样式设置。
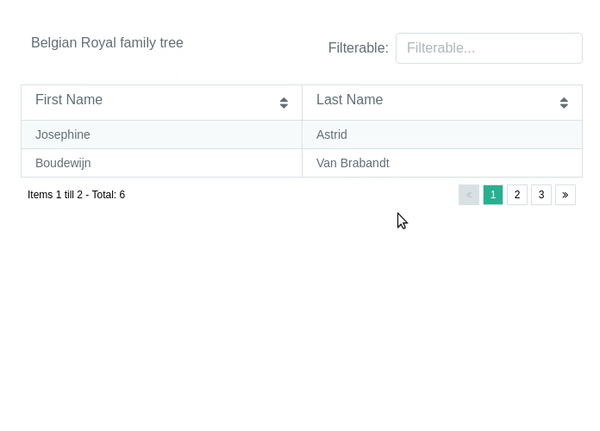
安装 (Installation)
You can install the package via npm or yarn.
您可以通过npm或yarn安装软件包。
NPM (NPM)
npm install vue-ads-table-tree --save
npm install vue-ads-table-tree --save
纱 (YARN)
yarn add vue-ads-table-tree
yarn add vue-ads-table-tree
用法 (Usage)
You can add the vue-ads-table-tree component by using the following code in your project. This is the most simple example.
您可以通过在项目中使用以下代码来添加vue-ads-table-tree组件。 这是最简单的例子。
<template>
<div id="app">
<table-tree
:columns="columns"
:rows="rows"
>
</table-tree>
</div>
</template>
<script>
import TableTree from './components/TableTree';
export default {
name: 'app',
components: {
TableTree,
},
data () {
return {
columns: [
{
property: 'firstName',
title: 'First Name',
sortable: true,
filterable: true,
},
{
property: 'lastName',
title: 'Last Name',
filterable: true,
sortable: true,
},
],
rows: [
{
firstName: 'Albert',
lastName: 'Einstein',
},
{
firstName: 'Barack',
lastName: 'Obama',
},
],
};
},
};
</script>
物产 (Properties)
styling
: (type: object) Contains styling classes from the tailwind library. Here you can find all available classes. Combinations are possible by separating them by a space.styling
:( 类型:对象)包含来自windwind库的样式类。 在这里您可以找到所有可用的类。 通过用空格隔开组合是可能的。rowsEven
: (type: string, default: 'bg-grey-lightest') Classes to add on the even rows.-
rowsEven
:( 类型:字符串,默认值:“ bg-grey-rowsEven
”)要在偶数行上添加的类。 rowsOdd
:rowsOdd
:( (type: string, default: 'bg-white') Classes to add on the odd rows.类型:字符串,默认:'bg-white')在奇数行上添加的类。rowsAll
:rowsAll
:( (type: string, default: 'hover:bg-grey-lighter') Classes to add on all rows.类型:字符串,默认值:“ hover:bg-grey-lighter”)要在所有行上添加的类。rowsAllExceptLast
:rowsAllExceptLast
:( (type: string, default: 'border-b') Classes to add on all rows except on the last one.类型:字符串,默认值:'border-b')要在除最后一行之外的所有行上添加的类。columnsEven
:columnsEven
:( (type: string) Classes to add on the even columns.类型:字符串)要在偶数列上添加的类。columnsOdd
:columnsOdd
:( (type: string) Classes to add on the odd columns.类型:字符串)要添加到奇数列的类。columnsAll
: *(type: string)*Classes to add on all columns.columnsAll
:*(type:string)*要在所有列上添加的类。columnsAllExceptLast
:columnsAllExceptLast
:( (type: string, default: 'border-r') Classes to add on all columns except on the last one.类型:字符串,默认值:'border-r')要在除最后一列之外的所有列上添加的类。table
:table
:( (type: string, default: 'border') Classes to add on the whole table.类型:字符串,默认值:'border')要在整个表格上添加的类。
columns
: (type: array, required) An array containing all the column objects. Each column object can contain the following properties:columns
: (type:array,必需)包含所有列对象的数组。 每个列对象可以包含以下属性:property
:property
:( (type: string, required) The corresponding value will be shown in the column of the given row property.类型:字符串,必需) 。相应的值将显示在给定row属性的列中。title
:title
:( (type: string) The title that will be shown in the header.类型:字符串)标题将显示在标题中。filterable
:filterable
:( (type: boolean) Filter on this column?类型:布尔值)是否在此列上进行过滤?sortable
:sortable
: (type: boolean) Is this column sortable?(type:boolean)此列可排序吗?direction
:direction
:( (type: boolean or null) The initial sort direction. If null, the column is not sorted. If true, the sorting is ascending. If false, the sorting is descending.类型:布尔值或null)初始排序方向。 如果为null,则不对列进行排序。 如果为true,则排序升序。 如果为false,则排序为降序。
rows
: (type: array, default: []) An array containing all the row objects. Each row object has his own key value pairs and extra meta data:rows
: (type:array,默认:[])一个包含所有行对象的数组。 每个行对象都有自己的键值对和额外的元数据:children
:children
:( (type: array) An array with child row objects.类型:数组)带有子行对象的数组。hasChildren
:hasChildren
:( (type: boolean, default: false) Indicates if this row has children. This property will automatically be set to true if the children attribute is set.类型:布尔值,默认值:false)指示此行是否有子级。 如果设置了children属性,此属性将自动设置为true。showChildren
:showChildren
: (type: boolean) Indicates if the children has to be shown on create. If this is true, and hasChildren is true, but no children attribute is found, an async call will be initiated to get the children.(type:boolean)指示是否必须在创建时显示子项。 如果为true,并且hasChildren为true,但是未找到children属性,则将启动异步调用以获取child。
totalRows
: (type: number, default: rows.length) The total number of rows. If this is greater than the current rows length, async calls will be executed if the unknown rows are requested.totalRows
:( 类型:数字,默认值:rows.length)总行数。 如果该长度大于当前行的长度,则在请求未知行时将执行异步调用。asyncCall
: (type: function) The function that is called when making an async request. It takes the following parameters:asyncCall
: (type:function)发出异步请求时调用的函数。 它采用以下参数:range
:range
:( (type: object) The paginated range with a start and end index. 类型:对象)具有开始和结束索引的分页范围。start
:start
: (type: number) Contains the current zero based start index.(type:number)包含当前从零开始的索引。end
:end
: (type: number) Contains the current zero based end index.(type:number)包含当前从零开始的结束索引。
filter
:filter
:( (type: string) The filter value.类型:字符串)过滤器值。sortColumns
:sortColumns
: (type: array) A list of all columns that are sortable. The last sorted column is the last column in this list. A column has the following interesting properties for sorting: (type:array)可排序的所有列的列表。 最后排序的列是此列表中的最后一列。 列具有以下有趣的排序属性:direction
:direction
:( (type: boolean or null) See columns => direction类型:布尔值或null),请参见列=>方向
parent
:parent
: (type: Row) If the child rows are called. This value is a Row object that contains all the info from the parent row.(type:Row)如果调用子行。 该值是一个Row对象,其中包含来自父行的所有信息。
useCache
: (type: boolean) Async called rows will be stored in the cache if no sorting or filtering is done and this value is true.useCache
: (type:boolean)如果未进行排序或过滤并且该值为true,则异步调用的行将存储在缓存中。itemsPerPage
: (type: number, default: 10) The max amount of items on one page.itemsPerPage
:( 类型:数字,默认值:10)一页上的最大项目数。page
: (type: number, default: 0) A zero-based number to set the initial page.page
:( 类型:数字,默认值:0)从零开始的数字,用于设置初始页面。filter
: (type: string) The initial filter value.filter
:( 类型:字符串)初始过滤器值。
测试中 (Testing)
We use the jest framework for testing the table tree component. Run the following command to test it:
我们使用jest框架来测试表树组件。 运行以下命令进行测试:
npm run test:unit
翻译自: https://vuejsexamples.com/a-vue-js-table-component-with-a-tree-functionality/