vuejs 组件
Vue头像 (VueAvatar)
Avatar component for VueJS 2
VueJS 2的头像组件
Use username to create letter avatar (up to 3 chars)
使用用户名创建字母头像(最多3个字符)
If an image src is provided, will try to use it as avatar
如果提供了图片src,将尝试将其用作头像
Images are lazy loaded. If it is not loaded successfully, it will not be used (letter avatar as fallback)
图像是延迟加载的。 如果未成功加载,则将不使用它(字母头像作为后备)
安装 (Installation)
npm install --save @lossendae/vue-avatar
用法 (Usage)
ES6 (ES6)
import Vue from 'vue'
import VueAvatar from '@lossendae/vue-avatar'
// If not installed globally
export default {
...
components: {
VueAvatar
},
...
}
CommonJS
普通JS
const Vue = require('vue')
const VueAvatar = require('@lossendae/vue-avatar')
// In your component if not installed globally
Vue.extend({
...
components: {
'vue-avatar': VueAvatar.VueAvatar
},
...
})
在全球范围内提供 (Make available globally)
Vue.component('vue-avatar', VueAvatar)
用法 (Usage)
<vue-avatar :username="'Franck Zappatta'" :src="'/path/to/img'"></vue-avatar>
道具 (Props)
Name | Type | Required | Default | Description |
---|---|---|---|---|
username | String | true | - | String used for the letter avatar (up to three characters) |
size | Number | false | 50 | Size in pixels of the avatar |
src | String | false | - | Optional image source path to use for the avatar |
colors | Array | false | DEFAULT_COLORS[] (see below) | Pool of colors used for avatar background image (expect array of hsl values) |
borderRadius | Number | false | 50 | Value of the border radius for the avatar |
customStyles | Object | false | {} | Custom style object to merge with the default style |
delay | Number | false | 0 | * See below |
名称 | 类型 | 需要 | 默认 | 描述 |
---|---|---|---|---|
用户名 | 串 | true | -- | 用于字母头像的字符串(最多三个字符) |
尺寸 | 数 | false | 50 | 化身的大小(以像素为单位) |
src | 串 | false | -- | 用于化身的可选图像源路径 |
颜色 | 数组 | false | DEFAULT_COLORS[] (如下所示) | 用于化身背景图片的颜色池(预期hsl值数组) |
borderRadius | 数 | false | 50 | 化身的边框半径值 |
customStyles | 目的 | false | {} | 自定义样式对象以与默认样式合并 |
延迟 | 数 | false | 0 | * 见下文 |
delay
道具 (delay
prop)
Specify the delay in milliseconds to wait before attempting to load the image src.
指定尝试加载图像src之前要等待的延迟(以毫秒为单位)。
This allows to show the letter avatar for the given time and then eventually load the image.
这样可以显示给定时间的字母头像,然后最终加载图像。
Vue-avatar uses vuejs built-in transitions to let you switch smoothly between the letters and the image.
Vue-avatar使用vuejs内置的过渡功能 ,可让您在字母和图像之间平稳切换。
By default, the transition will not do anything, but with a little bit of css you can for example flip the avatar to the image once loaded :
默认情况下,过渡不会执行任何操作,但是只需一点点CSS,您就可以将头像转换为加载后的图像:
/* Add this to your css file or into your component style to flip the avatar into the image if the image is loaded successfully */
/* The animation should also be triggered when the prop src changes */
.vue-avatar-enter-active {
animation: vue-avatar-in .8s;
}
.vue-avatar-leave-active {
animation: vue-avatar-in reverse;
}
@keyframes vue-avatar-in {
from {
transform: perspective(400px) rotate3d(0, 1, 0, 90deg);
animation-timing-function: ease-in;
opacity: 0;
}
40% {
transform: perspective(400px) rotate3d(0, 1, 0, -20deg);
animation-timing-function: ease-in;
}
60% {
transform: perspective(400px) rotate3d(0, 1, 0, 10deg);
opacity: 1;
}
80% {
transform: perspective(400px) rotate3d(0, 1, 0, -5deg);
}
to {
transform: perspective(400px);
}
}
@keyframes vue-avatar-out {
from {
transform: perspective(400px);
}
30% {
transform: perspective(400px) rotate3d(0, 1, 0, -15deg);
opacity: 1;
}
to {
transform: perspective(400px) rotate3d(0, 1, 0, 90deg);
opacity: 0;
}
}
The above transition was made using animate.css
上面的转换是使用animate.css进行的
默认颜色 (Default colors)
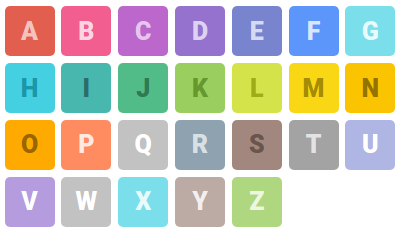
In you want to override those colors, use the colors props by passing an array of hsl colors
在您要覆盖这些颜色的情况下,通过传递hsl颜色数组来使用颜色道具
See below the values used for the above example:
请参见下面用于以上示例的值:
const COLORS_HSL = [
[6, 71, 60],
[340, 85, 66],
[291, 49, 60],
[263, 49, 63],
[232, 46, 64],
[218, 93, 67],
[187, 73, 70],
[187, 73, 58],
[175, 43, 50],
[151, 44, 53],
[88, 53, 59],
[66, 73, 59],
[51, 95, 53],
[47, 100, 49],
[40, 100, 50],
[16, 100, 69],
[0, 0, 76],
[201, 17, 62],
[17, 16, 56],
[0, 0, 64],
[233, 47, 79],
[262, 49, 74],
[0, 0, 76],
[187, 73, 70],
[15, 15, 69],
[88, 52, 67],
]
发展历程 (Development)
# Install dependencies
yarn install
# run the unit tests
yarn test
# Build
yarn build
翻译自: https://vuejsexamples.com/avatar-component-for-vuejs-2/
vuejs 组件