vuejs 非模块化 js
自动提示 (v-autosuggest)
A simple modular Vuejs component that autosuggest input from a dyanamic or static data querying.
一个简单的模块化Vuejs组件,可以自动建议来自动态或静态数据查询的输入。
安装 (Installation)
npm install --save v-autosuggest
用法 (Usage)
import VAutoSuggest from 'v-autosuggest'
Vue.component('v-autosuggest', VAutosuggest )
In your template you can use this syntax:
在模板中,您可以使用以下语法:
<VAutosuggest
v-model="searchData"
:getItemFromAjax="ajaxCall"
:suggValue="'name'"
:suggStatusComp="statusComponent"
:suggItemComp="suggItemComponent"
:items="staticSuggArray"
:maxSuggestion="maxSugg"
/>
物产 (Properties)
Property Name | type | Required | Default Value | Description |
---|---|---|---|---|
getItemFromAjax | Function | false | null | contains function that queries and returns the data ( if property "items" is present, this property function will not be fired ) |
suggValue | String | false | "value" | the property name that is the main thing that is being queried |
suggStatusComp | Object (vue component) | false | base status component | Vue component that show the status of the querying |
suggItemComp | Object (vue component) | false | base item component | Vue component that show the suggestion items |
items | Array | false | null | Contains a static array of items that is gonna be queried (if this property is present, the getItemFromAjax function will not be fired) |
maxSuggestion | Number | false | 5 | Max number of suggestion item is being shown on screen |
物业名称 | 类型 | 需要 | 默认值 | 描述 |
---|---|---|---|---|
getItemFromAjax | 功能 | 假 | 空值 | 包含查询并返回数据的函数(如果存在属性“ items”,则不会触发此属性函数) |
建议值 | 串 | 假 | “值” | 属性名称是要查询的主要内容 |
suggStatusComp | 对象(Vue组件) | 假 | 基本状态组件 | 显示查询状态的Vue组件 |
suggItemComp | 对象(Vue组件) | 假 | 基本项目组件 | 显示建议项的Vue组件 |
项目 | 数组 | 假 | 空值 | 包含要查询的静态项目数组(如果存在此属性,则不会触发getItemFromAjax函数) |
maxSuggestion | 数 | 假 | 5 | 屏幕上显示建议项的最大数量 |
例 (Example)
There's 2 ways of inserting the data for v-autosuggest
有2种方式为v-autosuggest插入数据
基本用法 (Basic Usage)
Through online querying (ie: ajax, firebase , etc...)
通过在线查询(即:ajax,firebase等)
Static JSON file or equivalent
静态JSON文件或等效文件
在线查询的基本用法(不仅限于ajax) (Basic usage with Online querying (not limited to ajax))
<template>
<VAutosuggest v-model="searchData" :getItemFromAjax="ajaxCall"/>
</template>
<script>
import axios from 'axios'
import VAutosuggest from 'v-autosuggest'
export default {
name: 'app',
components: {
VAutosuggest
},
data () {
return {
searchData: ''
}
},
methods: {
ajaxCall: async function (query) {
const response = await axios.get(`https://swapi.co/api/people/?search=${query}`)
return response.data.results.reduce((Accumulative, current) => {
Accumulative.push({value: current.name, description: 'Height: '+ current.height + 'cm'})
return Accumulative
})
}
}
}
</script>
Important Note:
重要说明 :
ajaxCall: Return either a promise or await the function and return the value
I use
reduce
in the ajaxCall to only get the the name and height of each of the data, but for the height i putdescription
, this is because the default suggestion component shows description in thedescription
property.我用
reduce
在AjaxCall的只得到每个数据的名称和高度,但我把高度description
,这是因为在默认的建议组件节目描述description
属性。
静态数据的基本用法(例如:JSON文件,数组,xml) (Basic usage with static data (eg: JSON file, array, xml))
<template>
<VAutosuggest v-model="searchData" :suggValue="'name'"/>
</template>
<script>
import axios from 'axios'
import VAutosuggest from 'v-autosuggest'
export default {
name: 'app',
components: {
VAutosuggest
},
data () {
return {
searchData: '',
arrayData: [{name:'Ben', description:'180cm'},{name:'Jon', description:'179cm'},{name:'Smith', description:'190cm'}]
}
}
}
</script>
高级用法 (Advanced Usage)
You are able to change the component for the status and suggestion to suit your own website.
您可以更改状态和建议的组件以适合您自己的网站。
这是状态组件 (This is the status component)
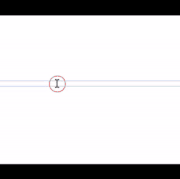
这些是建议项组件 (These are the suggestion items component)
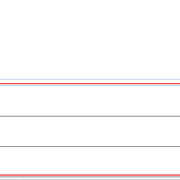
You will be able to change the status and suggestion item component by passing your own into the suggStatusComp and suggItemComp respectively.
通过将自己的值分别传递给suggStatusComp和suggItemComp,您将能够更改状态和建议项组件。
But there are some caveats.
但是有一些警告。
建议状态组件 (Suggestion Status Component)
When trying to make this component, be sure to include this prop and data
尝试制作此组件时,请确保包含此道具和数据
...props: {
suggestionStatusEnum: {
required: true,
type: Number,
default: 0
}
},
data () {
return {
suggestionStatus: {
nuetralStatus: 0,
noDataFound: 1,
loading: 2,
closeStatus: 3
}
}
}...
As you can see the suggestionStatus act as a enum to check the current status of the suggestion querying
如您所见, committionStatus充当枚举,用于检查建议查询的当前状态
Property Name | Description |
suggestionStatusEnum | represents the current status of the suggestion querying |
valueProp | Represents the key value data that you are trying to find/suggest |
物业名称 | 描述 |
proposalStatusEnum | 表示建议查询的当前状态 |
valueProp | 表示您要查找/建议的键值数据 |
状态枚举表 (Status enum table)
Status Name | Status Number | Status Description |
nuetralStatus | 0 | Shown as a neutral stands as no suggestion is being shown but will still be able to shown as empty li tag to the user |
noDataFound | 1 | This is to show an error message to the user that data can be found on their query |
loading | 2 | Shows a loading spinner to tell user it is querying the data |
closeStatus | 3 | Hides the li tag completely even if query finds the data |
状态名称 | 状态编号 | 状态说明 |
中立状态 | 0 | 显示为中立立场,表示未显示任何建议,但仍可以向用户显示为空li 标签 |
没有找到数据 | 1个 | 这是向用户显示一条错误消息,表明可以在其查询中找到数据 |
装货 | 2 | 显示加载微调器以告知用户正在查询数据 |
closeStatus | 3 | 即使查询找到数据也完全隐藏li 标签 |
<template>
<div v-show="suggestionStatusEnum != suggestionStatus.nuetralStatus || suggestionStatusEnum != suggestionStatus.closeStatus">
<div class="loader" v-show="suggestionStatusEnum == suggestionStatus.loading">
</div>
<div v-show="suggestionStatusEnum == suggestionStatus.noDataFound">
<h2>No result found</h2>
</div>
</div>
</template>
<style>
.loader {
border: 2px solid #f3f3f3;
border-radius: 100%;
border-top: 2px solid black;
width: 20px;
height: 20px;
-webkit-animation: spin 0.5s linear infinite;
animation: spin 0.5s linear infinite;
margin: 0 auto;
}
/* Safari */
@-webkit-keyframes spin {
0% { -webkit-transform: rotate(0deg); }
100% { -webkit-transform: rotate(360deg); }
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
</style>
<script>
export default {
name: 'suggestionStatus',
props: {
suggestionStatusEnum: {
required: true,
type: Number,
default: 0
}
},
data () {
return {
suggestionStatus: {
nuetralStatus: 0,
noDataFound: 1,
loading: 2,
closeStatus: 3
}
}
}
}
</script>
建议状态组件 (Suggestion Status Component)
When trying to make this component, be sure to include these 2 props
尝试制作此组件时,请确保包括这两个道具
...props: {
item: {
type: Object,
required: true
},
valueProp:{
type: String,
required: false,
default: 'value',
}
}...
property Name | Description |
item | Object that represents a suggestion item |
valueProp | Represents the key value data that you are trying to find/suggest |
物业名称 | 描述 |
项目 | 代表建议项的对象 |
valueProp | 表示您要查找/建议的键值数据 |
建议项目组件示例 (Example Suggestion Item component)
<template>
<div>
<h2 v-text="item[this.valueProp]"></h2>
<p v-text=" item.subDescription"></p>
<p v-text=" item.createAt"></p>
</div>
</template>
<script>
export default {
name: 'suggItemComponent',
props: {
item: {
type: Object,
required: true
},
valueProp:{
type: String,
required: false,
default: 'value',
}
}
}
</script>
翻译自: https://vuejsexamples.com/a-simple-modular-vuejs-component-that-autosuggest-input/
vuejs 非模块化 js