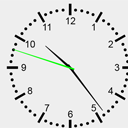
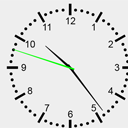
HTML5 Clocks For our new lesson I have prepared nice pure HTML5 clocks. This is pretty easy script, but it is very easy and impressive (as usual). Of course – anything necessary will be at canvas object.
HTML5时钟在我们的新课程中,我准备了不错的纯HTML5时钟。 这是一个非常简单的脚本,但是却非常容易且令人印象深刻(与往常一样)。 当然-画布对象上有任何必要的东西。
Here are our demo and downloadable package:
这是我们的演示和可下载的软件包:
现场演示
打包下载
Ok, download the source files and lets start coding !
好的,下载源文件并开始编码!
步骤1. HTML (Step 1. HTML)
This is markup of our clocks. Here it is.
这是我们时钟的标记。 这里是。
index.html (index.html)
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<title>HTML5 Clocks | Script Tutorials</title>
<link href="css/main.css" rel="stylesheet" type="text/css" />
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script src="js/script.js"></script>
</head>
<body>
<header>
<h2>HTML5 Clocks</h2>
<a href="https://www.script-tutorials.com/html5-clocks/" class="stuts">Back to original tutorial on <span>Script Tutorials</span></a>
</header>
<div class="clocks">
<canvas id="canvas" width="500" height="500"></canvas>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="utf-8" />
<title>HTML5 Clocks | Script Tutorials</title>
<link href="css/main.css" rel="stylesheet" type="text/css" />
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script src="js/script.js"></script>
</head>
<body>
<header>
<h2>HTML5 Clocks</h2>
<a href="https://www.script-tutorials.com/html5-clocks/" class="stuts">Back to original tutorial on <span>Script Tutorials</span></a>
</header>
<div class="clocks">
<canvas id="canvas" width="500" height="500"></canvas>
</div>
</body>
</html>
步骤2. CSS (Step 2. CSS)
Here are all required stylesheets
这是所有必需的样式表
css / main.css (css/main.css)
.clocks {
height: 500px;
margin: 25px auto;
position: relative;
width: 500px;
}
.clocks {
height: 500px;
margin: 25px auto;
position: relative;
width: 500px;
}
步骤3. JS (Step 3. JS)
js / script.js (js/script.js)
// inner variables
var canvas, ctx;
var clockRadius = 250;
var clockImage;
// draw functions :
function clear() { // clear canvas function
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
}
function drawScene() { // main drawScene function
clear(); // clear canvas
// get current time
var date = new Date();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
hours = hours > 12 ? hours - 12 : hours;
var hour = hours + minutes / 60;
var minute = minutes + seconds / 60;
// save current context
ctx.save();
// draw clock image (as background)
ctx.drawImage(clockImage, 0, 0, 500, 500);
ctx.translate(canvas.width / 2, canvas.height / 2);
ctx.beginPath();
// draw numbers
ctx.font = '36px Arial';
ctx.fillStyle = '#000';
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
for (var n = 1; n <= 12; n++) {
var theta = (n - 3) * (Math.PI * 2) / 12;
var x = clockRadius * 0.7 * Math.cos(theta);
var y = clockRadius * 0.7 * Math.sin(theta);
ctx.fillText(n, x, y);
}
// draw hour
ctx.save();
var theta = (hour - 3) * 2 * Math.PI / 12;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -5);
ctx.lineTo(-15, 5);
ctx.lineTo(clockRadius * 0.5, 1);
ctx.lineTo(clockRadius * 0.5, -1);
ctx.fill();
ctx.restore();
// draw minute
ctx.save();
var theta = (minute - 15) * 2 * Math.PI / 60;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -4);
ctx.lineTo(-15, 4);
ctx.lineTo(clockRadius * 0.8, 1);
ctx.lineTo(clockRadius * 0.8, -1);
ctx.fill();
ctx.restore();
// draw second
ctx.save();
var theta = (seconds - 15) * 2 * Math.PI / 60;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -3);
ctx.lineTo(-15, 3);
ctx.lineTo(clockRadius * 0.9, 1);
ctx.lineTo(clockRadius * 0.9, -1);
ctx.fillStyle = '#0f0';
ctx.fill();
ctx.restore();
ctx.restore();
}
// initialization
$(function(){
canvas = document.getElementById('canvas');
ctx = canvas.getContext('2d');
// var width = canvas.width;
// var height = canvas.height;
clockImage = new Image();
clockImage.src = 'images/cface.png';
setInterval(drawScene, 1000); // loop drawScene
});
// inner variables
var canvas, ctx;
var clockRadius = 250;
var clockImage;
// draw functions :
function clear() { // clear canvas function
ctx.clearRect(0, 0, ctx.canvas.width, ctx.canvas.height);
}
function drawScene() { // main drawScene function
clear(); // clear canvas
// get current time
var date = new Date();
var hours = date.getHours();
var minutes = date.getMinutes();
var seconds = date.getSeconds();
hours = hours > 12 ? hours - 12 : hours;
var hour = hours + minutes / 60;
var minute = minutes + seconds / 60;
// save current context
ctx.save();
// draw clock image (as background)
ctx.drawImage(clockImage, 0, 0, 500, 500);
ctx.translate(canvas.width / 2, canvas.height / 2);
ctx.beginPath();
// draw numbers
ctx.font = '36px Arial';
ctx.fillStyle = '#000';
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
for (var n = 1; n <= 12; n++) {
var theta = (n - 3) * (Math.PI * 2) / 12;
var x = clockRadius * 0.7 * Math.cos(theta);
var y = clockRadius * 0.7 * Math.sin(theta);
ctx.fillText(n, x, y);
}
// draw hour
ctx.save();
var theta = (hour - 3) * 2 * Math.PI / 12;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -5);
ctx.lineTo(-15, 5);
ctx.lineTo(clockRadius * 0.5, 1);
ctx.lineTo(clockRadius * 0.5, -1);
ctx.fill();
ctx.restore();
// draw minute
ctx.save();
var theta = (minute - 15) * 2 * Math.PI / 60;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -4);
ctx.lineTo(-15, 4);
ctx.lineTo(clockRadius * 0.8, 1);
ctx.lineTo(clockRadius * 0.8, -1);
ctx.fill();
ctx.restore();
// draw second
ctx.save();
var theta = (seconds - 15) * 2 * Math.PI / 60;
ctx.rotate(theta);
ctx.beginPath();
ctx.moveTo(-15, -3);
ctx.lineTo(-15, 3);
ctx.lineTo(clockRadius * 0.9, 1);
ctx.lineTo(clockRadius * 0.9, -1);
ctx.fillStyle = '#0f0';
ctx.fill();
ctx.restore();
ctx.restore();
}
// initialization
$(function(){
canvas = document.getElementById('canvas');
ctx = canvas.getContext('2d');
// var width = canvas.width;
// var height = canvas.height;
clockImage = new Image();
clockImage.src = 'images/cface.png';
setInterval(drawScene, 1000); // loop drawScene
});
I have defined main timer to redraw scene. Each step (tick) script defines current time, and draw clock arrows (hour arrow, minute arrow and second arrow).
我定义了主计时器来重绘场景。 每个步骤(刻度)脚本都定义当前时间,并绘制时钟箭头(小时箭头,分钟箭头和第二箭头)。
现场演示
打包下载
结论 (Conclusion)
Hope that today’s html5 clocks was impressive for you as always. We have made another one nice html5 tutorial. I will be glad to see your thanks and comments. Good luck!
希望今天的html5时钟像往常一样给您留下深刻的印象。 我们制作了另一个不错的html5教程。 看到您的感谢和评论,我将非常高兴。 祝好运!