The author selected Creative Commons to receive a donation as part of the Write for DOnations program.
作者选择了创用CC来接受捐赠,这是Write for DOnations计划的一部分。
介绍 (Introduction)
In this tutorial, you’ll learn how to describe elements with JSX. JSX is an abstraction that allows you to write HTML-like syntax in your JavaScript code and will enable you to build React components that look like standard HTML markup. JSX is the templating language of React elements, and is therefore the foundation for any markup that React will render into your application.
在本教程中,您将学习如何使用JSX描述元素。 JSX是一种抽象,它允许您在JavaScript代码中编写类似HTML的语法,并使您能够构建类似于标准HTML标记的React组件。 JSX是React元素的模板语言,因此是React将呈现到您的应用程序中的任何标记的基础。
Since JSX enables you to also write JavaScript in your markup, you’ll be able to take advantage of JavaScript functions and methods, including array mapping and short-circuit evaluation for conditionals.
由于JSX还使您能够在标记中编写JavaScript,因此您将能够利用JavaScript函数和方法,包括数组映射和对条件的短路评估。
As part of the tutorial, you’ll capture click events on buttons directly in the markup and catch instances when the syntax does not match exactly to standard HTML, such as with CSS classes. At the end of this tutorial, you’ll have a working application that uses a variety of JSX features to display a list of elements that have a built-in click listener. This is a common pattern in React applications that you will use often in the course of learning the framework. You’ll also be able to mix standard HTML elements along with JavaScript to see how React gives you the ability to create small, reusable pieces of code.
作为本教程的一部分,您将直接在标记中捕获按钮上的click事件,并在语法与标准HTML(例如CSS类)不完全匹配时捕获实例。 在本教程的最后,您将拥有一个使用各种JSX功能的有效应用程序,以显示具有内置单击侦听器的元素列表。 这是React应用程序中的一种常见模式,您在学习框架的过程中会经常使用它。 您还可以将标准HTML元素与JavaScript混合使用,以了解React如何使您能够创建小的,可重用的代码段。
先决条件 (Prerequisites)
You will need a development environment running Node.js; this tutorial was tested on Node.js version 10.19.0 and npm version 6.13.4. To install this on macOS or Ubuntu 18.04, follow the steps in How to Install Node.js and Create a Local Development Environment on macOS or the Installing Using a PPA section of How To Install Node.js on Ubuntu 18.04.
您将需要一个运行Node.js的开发环境; 本教程已在Node.js 10.19.0版和npm 6.13.4版上进行了测试。 要将其安装在macOS或Ubuntu 18.04上,请遵循如何在macOS上安装Node.js并创建本地开发环境中的步骤,或如何在Ubuntu 18.04上安装Node.js的 使用PPA安装部分中的步骤 。
You will need to be able to create apps with Create React App. You can find instructions for installing an application with Create React App at How To Set Up a React Project with Create React App.
您将需要能够使用Create React App创建应用程序 。 您可以在如何使用创建React App 设置React项目中找到有关使用Create React App安装应用程序的说明。
You will also need a basic knowledge of JavaScript, which you can find in How To Code in JavaScript, along with a basic knowledge of HTML and CSS. A good resource for HTML and CSS is the Mozilla Developer Network.
您还将需要JavaScript的基本知识,以及HTML和CSS的基本知识,这些知识可以在JavaScript的How To Code中找到。 Mozilla开发人员网络是HTML和CSS的良好资源。
第1步-将标记添加到React元素 (Step 1 — Adding Markup to a React Element)
As mentioned earlier, React has a special markup language called JSX. It is a mix of HTML and JavaScript syntax that looks something like this:
如前所述,React具有一种称为JSX的特殊标记语言。 它是HTML和JavaScript语法的组合,看起来像这样:
<div>
{inventory.filter(item => item.available).map(item => (
<Card>
<div className="title"}>{item.name}</div>
<div className="price">{item.price}</div>
</Card>
))
}
</div>
You will recognize some JavaScript functionality such as .filter
and .map
, as well as some standard HTML like <div>
. But there are other parts that look like both HTML and JavaScript, such as <Card>
and className
.
您将认识到一些JavaScript功能,例如.filter
和.map
,以及一些标准HTML例如<div>
。 但是还有其他看起来像HTML和JavaScript的部分,例如<Card>
和className
。
This is JSX, the special markup language that gives React components the feel of HTML with the power of JavaScript.
这就是JSX,这是一种特殊的标记语言,它使React组件具有JavaScript的功能,并具有HTML的感觉。
In this step, you’ll learn to add basic HTML-like syntax to an existing React element. To start, you’ll add standard HTML elements into a JavaScript function, then see the compiled code in a browser. You’ll also group elements so that React can compile them with minimal markup leaving clean HTML output.
在这一步中,您将学习将类似HTML的基本语法添加到现有的React元素中。 首先,将标准HTML元素添加到JavaScript函数中,然后在浏览器中查看编译后的代码。 您还将对元素进行分组,以便React可以用最少的标记来编译它们,从而留下干净HTML输出。
To start, make a new project. On your command line run the following script to install a fresh project using create-react-app
:
首先,创建一个新项目。 在命令行上,运行以下脚本以使用create-react-app
安装新项目:
npx create-react-app jsx-tutorial
npx create-react-app jsx教程
After the project is finished, change into the directory:
项目完成后,转到目录:
cd jsx-tutorial
cd jsx-教程
In a new terminal tab or window, start the project using the Create React App start
script. The browser will autorefresh on changes, so leave this script running the whole time that you work:
在新的终端标签或窗口中,使用Create React App start
脚本启动项目。 浏览器将自动刷新更改,因此请在您工作期间始终运行以下脚本:
- npm start npm开始
You will get a running local server. If the project did not open in a browser window, you can find it at http://localhost:3000/
. If you are running this from a remote server, the address will be http://your_IP_address:3000
.
您将获得正在运行的本地服务器。 如果未在浏览器窗口中打开项目,则可以在http://localhost:3000/
找到它。 如果从远程服务器运行此地址,则该地址将为http:// your_IP_address :3000
。
Your browser will load with a React application included as part of Create React App.
您的浏览器将加载包含在Create React App中的React应用程序。
You will be building a completely new set of custom components, so you’ll need to start by clearing out some boilerplate code so that you can have an empty project. To start open App.js
in a text editor. This is the root component that is injected into the page. All components will start from here.
您将构建一套全新的自定义组件,因此您需要从清除一些样板代码开始,以便拥有一个空项目。 要开始在文本编辑器中打开App.js
这是注入页面的根组件。 所有组件将从此处开始。
In a new terminal, move into the project folder and open src/App.js
with the following command:
在新的终端中,移至项目文件夹, src/App.js
使用以下命令打开src/App.js
:
- nano src/App.js 纳米src / App.js
You will see a file like this:
您将看到如下文件:
import React from 'react';
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
Now, delete the line import logo from './logo.svg
and everything after the return statement in the function. Change it to return null
. The final code will look like this:
现在, import logo from './logo.svg
删除行import logo from './logo.svg
以及该函数中return语句之后的所有内容。 将其更改为返回null
。 最终代码如下所示:
import React from 'react';
import './App.css';
function App() {
return null;
}
export default App;
Save and exit the text editor.
保存并退出文本编辑器。
Finally, delete the logo. In the terminal window type the following command:
最后,删除徽标。 在终端窗口中,输入以下命令:
- rm src/logo.svg rm src / logo.svg
You won’t be using this SVG file in your application, and you should remove unused files as you work. It will better organize your code in the long run.
您将不会在应用程序中使用此SVG文件,并且应在工作时删除未使用的文件。 从长远来看,它将更好地组织您的代码。
Now that these parts of your project are removed, you can move on to exploring the facets of JSX. This markup language is compiled by React and eventually becomes the HTML you see on a web page. Without going too deeply into the internals, React takes the JSX and creates a model of what your page will look like, then creates the necessary elements and adds them to the page.
现在已经删除了项目的这些部分,您可以继续探索JSX的各个方面。 该标记语言由React编译,最终成为您在网页上看到HTML。 在不深入内部的情况下 ,React使用JSX并创建页面外观的模型,然后创建必要的元素并将其添加到页面中。
What that means is that you can write what looks like HTML and expect that the rendered HTML will be similar. However, there are a few catches.
这意味着您可以编写看起来像HTML的内容,并期望呈现HTML将相似。 但是,有一些问题。
First, if you look at the tab or window running your server, you’ll see this:
首先,如果查看运行服务器的选项卡或窗口,则会看到以下内容:
Output
...
./src/App.js
Line 1:8: 'React' is defined but never used no-unused-vars
...
That’s the linter telling you that you aren’t using the imported React code. When you add the line import React from 'react'
to your code, you are importing JavaScript code that converts the JSX to React code. If there’s no JSX, there’s no need for the import.
这就是linter告诉您您没有使用导入的React代码。 当您将行import React from 'react'
到代码中时,您就是在导入将JSX转换为React代码JavaScript代码。 如果没有JSX,则无需导入。
Let’s change that by adding a small amount of JSX. Start by replacing null
with a Hello, World
example:
让我们通过添加少量JSX来更改它。 首先用Hello, World
示例替换null
:
import React from 'react';
import './App.css';
function App() {
return <h1>Hello, World</h1>;
}
export default App;
Save the file. If you look at the terminal with the server running, the warning message will be gone. If you visit your browser, you will see the message as an h1
element.
保存文件。 如果您在运行服务器的终端上查看,则警告消息将消失。 如果您访问浏览器,则将消息视为h1
元素。
Next, below the <h1>
tag, add a paragraph tag that contains the string I am writing JSX
. The code will look like this:
接下来,在<h1>
标记下方,添加一个包含I am writing JSX
的字符串的段落标记。 该代码将如下所示:
import React from 'react';
import './App.css';
function App() {
return(
<h1>Hello, World</h1>
<p>I am writing JSX</p>
)
}
export default App;
Since the JSX spans multiple lines, you’ll need to wrap the expression in parentheses.
由于JSX跨越多行,因此您需要将表达式包装在括号中。
Save the file. When you do you’ll see an error in the terminal running your server:
保存文件。 当您执行此操作时,将在运行服务器的终端中看到错误:
Output
./src/App.js
Line 7:5: Parsing error: Adjacent JSX elements must be wrapped in an enclosing tag. Did you want a JSX fragment <>...</>?
5 | return(
6 | <h1>Hello, World</h1>
> 7 | <p>I am writing JSX</p>
| ^
8 | )
9 | }
10 |
When you return JSX from a function or statement, you must return a single element. That element may have nested children, but there must be a single top-level element. In this case, you are returning two elements.
从函数或语句返回JSX时,必须返回单个元素。 该元素可能具有嵌套的子元素,但必须有一个顶级元素。 在这种情况下,您将返回两个元素。
The fix is a small code change. Surround the code with an empty tag. An empty tag is an HTML element without any words. It looks like this: <></>
.
修复是一个小的代码更改。 用空标签将代码括起来 。 空标记是没有任何单词HTML元素。 看起来像这样: <></>
。
Go back to ./src/App.js
in your editor and add the empty tag:
返回编辑器中的./src/App.js
并添加空标记:
import React from 'react';
import './App.css';
function App() {
return(
<>
<h1>Hello, World</h1>
<p>I am writing JSX</p>
</>
)
}
export default App;
The empty tag creates a single element, but when the code is compiled, it is not added to the final markup. This will keep your code clean while still giving React a single element.
empty标签创建单个元素,但是在编译代码时,不会将其添加到最终标记中。 这将使您的代码保持干净,同时仍给React一个元素。
Note: You could have also wrapped the code with a div
instead of empty tags, as long as the code returns one element. In this example, an empty tag has the advantage of not adding extra markup to the parsed output.
注意:您也可以使用div
而不是空标签来包装代码,只要代码返回一个元素即可。 在此示例中,空标记的优点是不会在解析的输出中添加额外的标记。
Save the code and exit the file. Your browser will refresh and show the updated page with the paragraph element. In addition, when the code is converted the empty tags are stripped out:
保存代码并退出文件。 您的浏览器将刷新并显示带有段落元素的更新页面。 另外,在转换代码时,空标签被剥离:
You’ve now added some basic JSX to your component and learned how all JSX needs to be nested in a single component. In the next step, you’ll add some styling to your component.
现在,您已向组件中添加了一些基本的JSX,并了解了如何将所有JSX嵌套在单个组件中。 在下一步中,您将向您的组件添加一些样式。
第2步-向具有属性的元素添加样式 (Step 2 — Adding Styling to an Element with Attributes)
In this step, you’ll style the elements in your component to learn how HTML attributes work with JSX. There are many styling options in React. Some of them involve writing CSS in Javascript, others use preprocessors. In this tutorial you’ll work with imported CSS and CSS classes.
在此步骤中,您将对组件中的元素进行样式设置,以了解HTML属性如何与JSX一起使用。 React中有很多样式选项 。 其中一些涉及使用Javascript编写CSS,另一些则使用预处理器。 在本教程中,您将使用导入CSS和CSS类。
Now that you have your code, it’s time to add some styling. Open App.css
in your text editor:
现在您已经有了代码,是时候添加一些样式了。 在文本编辑器中打开App.css
:
- nano src/App.css 纳米src / App.css
Since you are starting with new JSX, the current CSS refers to elements that no longer exist. Since you don’t need the CSS, you can delete it.
由于您是从新的JSX开始的,因此当前CSS引用的元素不再存在。 由于不需要CSS,因此可以将其删除。
After deleting the code, you’ll have an empty file.
删除代码后,您将拥有一个空文件。
Next, you will add in some styling to center the text. In src/App.css
, add the following code:
接下来,您将添加一些样式以使文本居中。 在src/App.css
,添加以下代码:
.container {
display: flex;
flex-direction: column;
align-items: center;
}
In this code block, you created a CSS class selector called .container
and used that to center the content using display: flex
.
在此代码块中,您创建了一个名为.container
的CSS类选择器,并使用它来使用display: flex
来使内容居中。
Save the file and exit. The browser will update, but nothing will change. Before you can see the change, you need to add the CSS class to your React component. Open the component JavaScript code:
保存文件并退出。 浏览器将更新,但是什么都不会改变。 在看到更改之前,您需要将CSS类添加到React组件中。 打开组件JavaScript代码:
- nano src/App.js 纳米src / App.js
The CSS code is already imported with the line import './App.css'
. That means that webpack will pull in the code to make a final style sheet, but to apply the CSS to your elements, you need to add the classes.
CSS代码已使用import './App.css'
行import './App.css'
。 这意味着webpack将拉入代码以制作最终的样式表,但是要将CSS应用于元素,则需要添加类。
First, in your text editor, change the empty tags, <>
, to <div>
.
首先,在文本编辑器中,将空标签<>
更改为<div>
。
import React from 'react';
import './App.css';
function App() {
return(
<div>
<h1>Hello, World</h1>
<p>I am writing JSX</p>
</div>
)
}
export default App;
In this code, you replaced the empty tags—<>
—with div
tags. Empty tags are useful for grouping your code without adding any extra tags, but here you need to use a div
because empty tags do not accept any HTML attributes.
在此代码中,您用div
标签替换了空标签- <>
。 空标记可用于在不添加任何额外标记的情况下对代码进行分组,但是在这里您需要使用div
因为空标记不接受任何HTML属性 。
Next, you need to add the class name. This is where JSX will start to diverge from HTML. If you wanted to add a class to a usual HTML element you would do it like this:
接下来,您需要添加类名称。 这就是JSX将开始与HTML脱节的地方。 如果您想向通常HTML元素中添加类,则可以这样做:
<div class="container">
But since JSX is JavaScript, it has a few limitations. One of the limitations is that JavaScript has reserved keywords. That means you can’t use certain words in any JavaScript code. For example, you can’t make a variable called null
because that word is already reserved.
但是由于JSX是JavaScript,所以有一些限制。 限制之一是JavaScript 保留了关键字 。 这意味着您不能在任何JavaScript代码中使用某些单词。 例如,您不能创建一个名为null
的变量,因为该单词已被保留。
One of the reserved words is class
. React gets around this reserved word by changing it slightly. Instead of adding the attribute class
, you will add the attribute className
. As a rule, if an attribute is not working as expected, try adding the camel case version. Another attribute that is slightly different is the for
attribute that you’d use for labels. There are a few other cases, but fortunately the list is fairly short.
保留字之一是class
。 React通过稍微更改它来解决这个保留字。 代替添加属性class
,您将添加属性className
。 通常,如果属性无法正常工作,请尝试添加骆驼保护套版本。 另一个稍有不同的属性是用于标签的for
属性。 还有其他几种情况 ,但幸运的是,清单很短。
Note: In React, attributes are often called props. Props are pieces of data that you can pass to other custom components. They look the same as attributes except that they do not match any HTML specs. In this tutorial, we’ll call them attributes since they are mainly used like standard HTML attributes. This will distinguish them from props that do not behave like HTML attributes, which will be covered later in this series.
注意:在React中,属性通常称为props 。 道具是可以传递给其他自定义组件的数据。 它们看起来与属性相同,只是它们不匹配任何HTML规范。 在本教程中,我们将它们称为属性,因为它们主要像标准HTML属性一样使用。 这将使它们与行为不像HTML属性的道具区别开来,本系列稍后将对此进行介绍。
Now that you know how the class
attribute is used in React, you can update your code to include the styles. In your text editor, add className="container"
to your opening div
tag:
现在您知道了如何在React中使用class
属性,接下来可以更新代码以包括样式。 在文本编辑器中,将className="container"
添加到开头的div
标签中:
import React from 'react';
import './App.css';
function App() {
return(
<div className="container">
<h1>Hello, World</h1>
<p>I am writing JSX</p>
</div>
)
}
export default App;
Save the file. When you do, the page will reload and the content will be centered.
保存文件。 完成后,页面将重新加载,内容将居中。
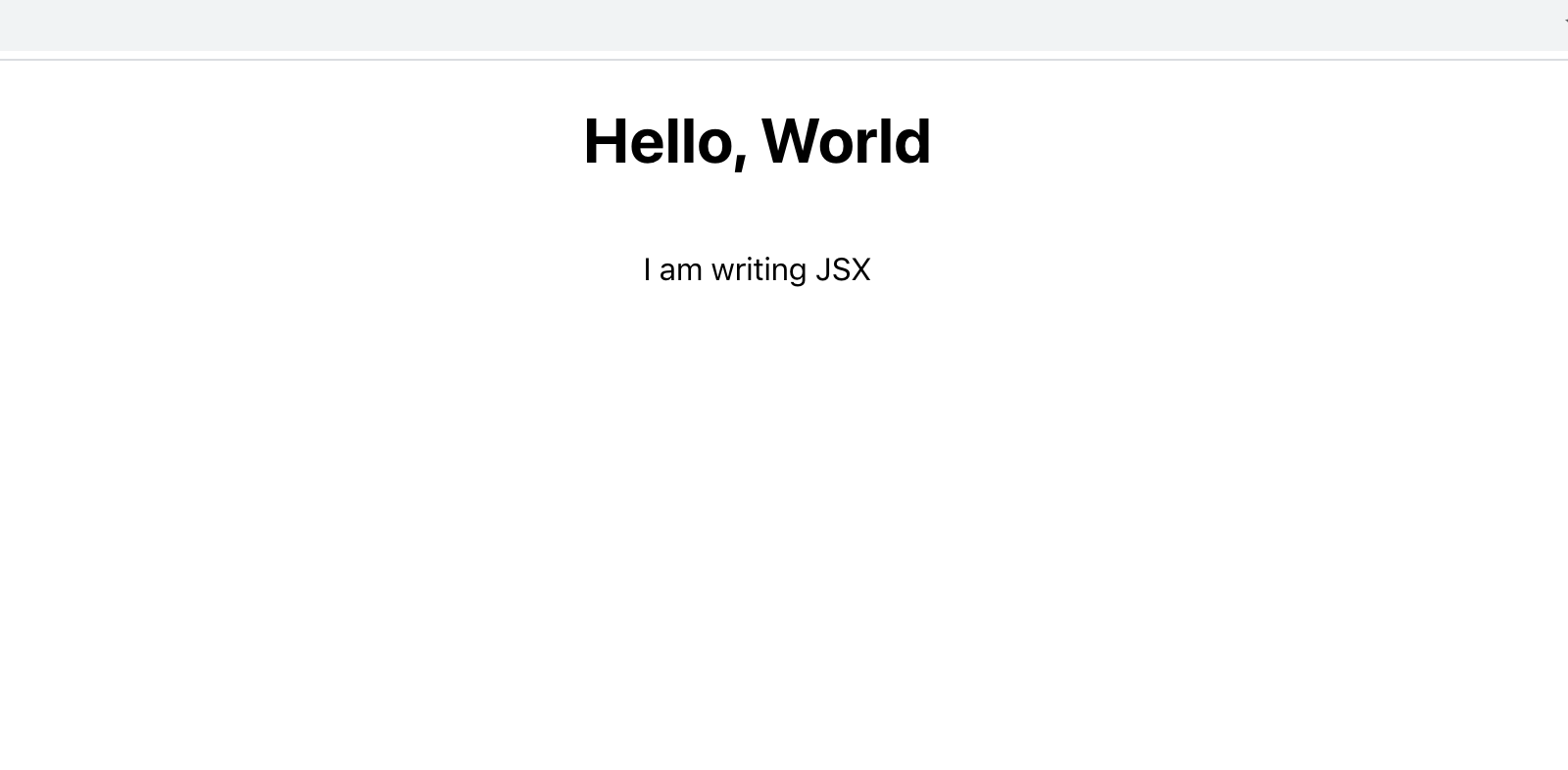
The className
attribute is unique in React. You can add most HTML attributes to JSX without any change. As an example, go back to your text editor and add an id
of greeting
to your <h1>
element. It will look like standard HTML:
className
属性在React中是唯一的。 您可以将大多数HTML属性添加到JSX,而无需进行任何更改。 例如,返回到文本编辑器,并向<h1>
元素添加greeting
id
。 看起来像标准HTML:
import React from 'react';
import './App.css';
function App() {
return(
<div className="container">
<h1 id="greeting">Hello, World</h1>
<p>I am writing JSX</p>
</div>
)
}
export default App;
Save the page and reload the browser. It will be the same.
保存页面并重新加载浏览器。 会是一样的。
So far, JSX looks like standard markup, but the advantage of JSX is that even though it looks like HTML, it has the power of JavaScript. That means you can assign variables and reference them in your attributes. To reference an attribute, wrap it with curly braces—{}
—instead of quotes.
到目前为止,JSX看起来像标准标记,但是JSX的优点是,即使看起来像HTML,它也具有JavaScript的功能。 这意味着您可以分配变量并在属性中引用它们。 要引用属性,请用大括号{}
代替引号将其引起来。
In your text editor, add the following highlighted lines to reference an attribute:
在文本编辑器中,添加以下突出显示的行以引用属性:
import React from 'react';
import './App.css';
function App() {
const greeting = "greeting";
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
<p>I am writing JSX</p>
</div>
)
}
export default App;
In this code, you created a variable above the return
statement called greeting
with the value of "greeting"
, then referenced the variable in the id
attribute of your <h1>
tag.
在这段代码中,创建了高于变量return
称为声明greeting
与价值"greeting"
,则引用的变量id
您的属性<h1>
标签。
Save and exit the file. The page will be the same, but with an id
tag.
保存并退出文件。 该页面将相同,但带有id
标签。
So far you’ve worked with a few elements on their own, but you can also use JSX to add many HTML elements and nest them to create complex pages.
到目前为止,您已经自己处理了一些元素,但是您也可以使用JSX添加许多HTML元素并将它们嵌套以创建复杂的页面。
To demonstrate this, you’ll make a page with a list of emoji. These emoji will be wrapped with a <button>
element. When you click on the emoji, you’ll get their CLDR Short Name.
为了说明这一点,您将制作一个带有表情符号列表的页面。 这些表情符号将使用<button>
元素包装。 单击表情符号后,您将获得其CLDR简称 。
To start, you’ll need to add a few more elements to the page. Open src/App.js
in your text editor. Keep it open during this step.
首先,您需要向页面添加更多元素。 在文本编辑器中打开src/App.js
在此步骤中使其保持打开状态。
- nano src/App.js 纳米src / App.js
First, add a list of emojis by adding the following highlighted lines:
首先,通过添加以下突出显示的行来添加表情符号列表:
import React from 'react';
import './App.css';
function App() {
const greeting = "greeting";
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
<p>I am writing JSX</p>
<ul>
<li>
<button>
<span role="img" aria-label="grinning face" id="grinning face">😀</span>
</button>
</li>
<li>
<button>
<span role="img" aria-label="party popper" id="party popper">🎉</span>
</button>
</li>
<li>
<button>
<span role="img" aria-label="woman dancing" id="woman dancing">💃</span>
</button>
</li>
</ul>
</div>
)
}
export default App;
Here you created a <ul>
tag to hold a list of emojis. Each emoji is in a separate <li>
element and is surrounded with a <button>
element. In the next step you’ll add an event to this button.
在这里,您创建了一个<ul>
标签来保存表情符号列表。 每个表情符号都位于单独的<li>
元素中,并由<button>
元素包围。 在下一步中,您将向该按钮添加一个事件 。
You also surrounded the emoji with a <span>
tag that has a few more attributes. Each span
has the role
attribute set to the img
role. This will signal to accessibility software that the element is acting like an image. In addition, each <span>
also has an aria-label
and an id
attribute with the name of the emoji. The aria-label
will tell visitors with screen readers what is displayed. You will use the id
when writing events in the next step.
您还用<span>
标签包围了表情符号,该标签具有更多属性。 每个span
的role
属性都设置为img
角色。 这将向可访问性软件发出信号,通知该元素的行为类似于图像 。 另外,每个<span>
还具有一个aria-label
和一个带有表情符号名称的id
属性。 aria-label
将告诉屏幕阅读器的访问者显示了什么。 在下一步中编写事件时,将使用id
。
When you write code this way, you are using semantic elements, which will help keep the page accessible and easy to parse for screen readers.
以这种方式编写代码时,您将使用语义元素 ,这将有助于保持页面的可访问性并易于屏幕阅读器解析。
Save and exit the file. Your browser will refresh and you will see this:
保存并退出文件。 您的浏览器将刷新,您将看到以下信息:
Now add a little styling. Open the CSS code in your text editor:
现在添加一些样式。 在文本编辑器中打开CSS代码:
- nano src/App.css 纳米src / App.css
Add the following highlighted code to remove the default background and border for the buttons while increasing the font size:
添加以下突出显示的代码,以在增加字体大小的同时删除按钮的默认背景和边框:
.container {
display: flex;
flex-direction: column;
align-items: center;
}
button {
font-size: 2em;
border: 0;
padding: 0;
background: none;
cursor: pointer;
}
ul {
display: flex;
padding: 0;
}
li {
margin: 0 20px;
list-style: none;
padding: 0;
}
In this code, you used font-size
, border
, and other parameters to adjust the look of your buttons and change the font. You also removed the list styles and added display: flex
to the <ul>
element to make it horizontal.
在此代码中,您使用了font-size
, border
和其他参数来调整按钮的外观并更改字体。 您还删除了列表样式并添加了display: flex
到<ul>
元素以使其变为水平。
Save and close the CSS file. Your browser will refresh and you will see this:
保存并关闭CSS文件。 您的浏览器将刷新,您将看到以下信息:
You’ve now worked with several JSX elements that look like regular HTML. You’ve added classes, ids, and aria tags, and have worked with data as strings and variables. But React also uses attributes to define how your elements should respond to user events. In the next step, you’ll start to make the page interactive by adding events to the button.
现在,您已经使用了一些看起来像普通HTML的JSX元素。 您已经添加了类,id和aria标签,并将数据作为字符串和变量进行了处理。 但是React也使用属性来定义您的元素应如何响应用户事件。 在下一步中,您将通过向按钮添加事件来开始使页面具有交互性。
步骤3 —将事件添加到元素 (Step 3 — Adding Events to Elements)
In this step, you’ll add events to elements using special attributes and capture a click event on a button element. You’ll learn how to capture information from the event to dispatch another action or use other information in the scope of the file.
在此步骤中,您将使用特殊属性将事件添加到元素,并捕获按钮元素上的click事件。 您将学习如何从事件中捕获信息以调度其他操作或在文件范围内使用其他信息。
Now that you have a basic page with information, it’s time to add a few events to it. There are many event handlers that you can add to HTML elements. React gives you access to all of these. Since your JavaScript code is coupled with your markup, you can quickly add the events while keeping your code well-organized.
现在您已经有了一个包含信息的基本页面,是时候向其中添加一些事件了。 您可以将许多事件处理程序添加到HTML元素中。 React使您可以访问所有这些内容。 由于您JavaScript代码与标记结合在一起,因此您可以快速添加事件,同时保持代码井井有条。
To start, add the onclick
event handler. This lets you add some JavaScript code directly to your element rather than attaching an event listener:
首先,添加onclick
事件处理程序 。 这使您可以将一些JavaScript代码直接添加到元素中,而不是附加事件侦听器:
import React from 'react';
import './App.css';
function App() {
const greeting = "greeting";
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
<p>I am writing JSX</p>
<ul>
<li>
<button
onClick={event => alert(event.target.id)}
>
<span role="img" aria-label="grinning face" id="grinning face">😀</span>
</button>
</li>
<li>
<button
onClick={event => alert(event.target.id)}
>
<span role="img" aria-label="party popper" id="party popper">🎉</span>
</button>
</li>
<li>
<button
onClick={event => alert(event.target.id)}
>
<span role="img" aria-label="woman dancing" id="woman dancing">💃</span>
</button>
</li>
</ul>
</div>
)
}
export default App;
Since this is JSX, you camelCased onclick
, which means you added it as onClick
. This onClick
attribute uses an anonymous function to retrieve information about the item that was clicked.
由于这是JSX,因此您可以将onclick
驼峰化,这意味着您将其添加为onClick
。 此onClick
属性使用匿名函数来检索有关被单击项目的信息。
You added an anonymous arrow function that will get the event from the clicked button, and the event will have a target that is the <span>
element. The information you need is in the id
attribute, which you can access with event.target.id
. You can trigger the alert with the alert()
function.
您添加了一个匿名箭头功能 ,该功能将从单击的按钮获取事件,并且该事件的目标是<span>
元素。 您需要的信息在id
属性中,您可以使用event.target.id
进行访问。 您可以使用alert()
函数触发警报。
Save the file. In your browser, click on one of the emoji and you will get an alert with the name.
保存文件。 在浏览器中,单击其中一个表情符号,您将收到一个带有名称的警报。
You can reduce a duplication by declaring the function once and passing it to each onClick
action. Since the function does not rely on anything other than inputs and outputs, you can declare it outside the main component function. In other words, the function does not need to access the scope of the component. The advantage to keeping them separate is that your component function is slightly shorter and you could move the function out to a separate file later if you wanted to.
您可以通过声明一次函数并将其传递给每个onClick
操作来减少重复。 由于该函数不依赖输入和输出以外的任何内容,因此可以在主要组件函数之外声明它。 换句话说,该功能不需要访问组件的范围。 将它们分开的好处是您的组件函数会略短一些,如果需要,您可以稍后将该函数移到单独的文件中。
In your text editor, create a function called displayEmojiName
that takes the event and calls the alert()
function with an id. Then pass the function to each onClick
attribute:
在您的文本编辑器中,创建一个名为displayEmojiName
的函数,该函数接收事件并使用ID调用alert()
函数。 然后将函数传递给每个onClick
属性:
import React from 'react';
import './App.css';
const displayEmojiName = event => alert(event.target.id);
function App() {
const greeting = "greeting";
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
<p>I am writing JSX</p>
<ul>
<li>
<button
onClick={displayEmojiName}
>
<span role="img" aria-label="grinning face" id="grinning face">😀</span>
</button>
</li>
<li>
<button
onClick={displayEmojiName}
>
<span role="img" aria-label="party popper" id="party popper">🎉</span>
</button>
</li>
<li>
<button
onClick={displayEmojiName}
>
<span role="img" aria-label="woman dancing" id="woman dancing">💃</span>
</button>
</li>
</ul>
</div>
)
}
export default App;
Save the file. In your browser, click on an emoji and you will see the same alert.
保存文件。 在浏览器中,单击表情符号,您将看到相同的警报。
In this step, you added events to each element. You also saw how JSX uses slightly different names for element events, and you started writing reusable code by taking the function and reusing it on several elements. In the next step, you will write a reusable function that returns JSX elements rather than writing each element by hand. This will further reduce duplication.
在此步骤中,您向每个元素添加了事件。 您还了解了JSX如何对元素事件使用略有不同的名称,并且通过使用该函数并将其在多个元素上重用来开始编写可重用的代码。 在下一步中,您将编写一个可重用的函数,该函数返回JSX元素,而不是手工编写每个元素。 这将进一步减少重复。
步骤4 —映射数据以创建元素 (Step 4 — Mapping Over Data to Create Elements)
In this step, you’ll move beyond using JSX as simple markup. You’ll learn to combine it with JavaScript to create dynamic markup that reduces code and improves readability. You’ll refactor your code into an array that you will loop over to create HTML elements.
在这一步中,您将超越使用JSX作为简单标记的范围。 您将学习将其与JavaScript结合在一起以创建动态标记,从而减少代码并提高可读性。 您将代码重构为一个数组,然后将其循环创建HTML元素。
JSX doesn’t limit you to an HTML-like syntax. It also gives you the ability to use JavaScript directly in your markup. You tried this a little already by passing functions to attributes. You also used variables to reuse data. Now it’s time to create JSX directly from data using standard JavaScript code.
JSX并不限制您使用类似HTML的语法。 它还使您能够直接在标记中使用JavaScript。 您已经通过将函数传递给属性来进行一些尝试。 您还使用了变量来重用数据。 现在是时候使用标准JavaScript代码直接从数据创建JSX。
In your text editor, you will need to create an array of the emoji data in the src/App.js
file. Reopen the file if you have closed it:
在文本编辑器中,您将需要在src/App.js
文件中创建一个表情符号数据数组。 如果已关闭文件,请重新打开它:
- nano src/App.js 纳米src / App.js
Add an array that will contain objects that have the emoji and the emoji name. Note that emojis need to be surrounded by quote marks. Create this array above the App
function:
添加将包含一个数组对象有表情符号和表情符号的名字。 请注意,表情符号必须用引号引起来。 在App
函数上方创建此数组:
import React from 'react';
import './App.css';
const displayEmojiName = event => alert(event.target.id);
const emojis = [
{
emoji: "😀",
name: "grinning face"
},
{
emoji: "🎉",
name: "party popper"
},
{
emoji: "💃",
name: "woman dancing"
}
];
function App() {
...
}
export default App;
Now that you have the data you can loop over it. To use JavaScript inside of JSX, you need to surround it with curly braces: {}
. This is the same as when you added functions to attributes.
现在您有了数据,可以对其进行循环。 要在JSX中使用JavaScript,您需要将其用花括号包围: {}
。 这与将函数添加到属性时相同。
To create React components, you’ll need to convert the data to JSX elements. To do this, you’ll map over the data and return a JSX element. There are a few things you’ll need to keep in mind as you write the code.
要创建React组件,您需要将数据转换为JSX元素。 为此,您将映射数据并返回JSX元素。 在编写代码时,您需要牢记一些事情。
First, a group of items needs to be surrounded by a container <div>
. Second, every item needs a special property called key
. The key
needs to be a unique piece of data that React can use to keep track of the elements so it can know when to update the component. The key will be stripped out of the compiled HTML, since it is for internal purposes only. Whenever you are working with loops you will need to add a simple string as a key.
首先,一组项目需要用容器<div>
包围。 其次,每个项目都需要一个称为key
的特殊属性。 key
必须是React可以用来跟踪元素的唯一数据,以便它知道何时更新组件 。 密钥将从编译HTML中剥离,因为它仅用于内部目的。 每当使用循环时,都需要添加一个简单的字符串作为键。
Here’s a simplified example that maps a list of names into a containing <div>
:
这是一个简化的示例,将名称列表映射到包含<div>
的名称中:
...
const names = [
"Atul Gawande",
"Stan Sakai",
"Barry Lopez"
];
return(
<div>
{names.map(name => <div key={name}>{name}</div>)}
</div>
)
...
The resulting HTML would look like this:
生成HTML将如下所示:
...
<div>
<div>Atul Gawande</div>
<div>Stan Sakai</div>
<div>Barry Lopez</div>
</div>
...
Converting the emoji list will be similar. The <ul>
will be the container. You’ll map over data and return a <li>
with a key of the emoji short name. You will replace the hard-coded data in the <button>
and <span>
tags with information from the loop.
转换表情符号列表将类似。 <ul>
将是容器。 您将映射数据并返回带有表情符号简称的键的<li>
。 您将用循环中的信息替换<button>
和<span>
标记中的硬编码数据。
In your text editor, add the following:
在文本编辑器中,添加以下内容:
import React from 'react';
import './App.css';
const displayEmojiName = event => alert(event.target.id);
const emojis = [
{
emoji: '😀',
name: "test grinning face"
},
{
emoji: '🎉',
name: "party popper"
},
{
emoji: '💃',
name: "woman dancing"
}
];
function App() {
const greeting = "greeting";
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
<p>I am writing JSX</p>
<ul>
{
emojis.map(emoji => (
<li key={emoji.name}>
<button
onClick={displayEmojiName}
>
<span role="img" aria-label={emoji.name} id={emoji.name}>{emoji.emoji}</span>
</button>
</li>
))
}
</ul>
</div>
)
}
export default App;
In the code, you mapped over the emojis
array in the <ul>
tag and returned a <li>
. In each <li>
you used the emoji name as the key
prop. The button will have the same function as normal. In the <span>
element, replace the aria-label
and id
with the name
. The content of the <span>
tag should be the emoji.
在代码中,您映射了<ul>
标签中的emojis
数组,并返回了<li>
。 在每个<li>
您都使用表情符号名称作为key
道具。 该按钮将具有与普通按钮相同的功能。 在<span>
元素中,将aria-label
和id
替换为name
。 <span>
标记的内容应为表情符号。
Save the file. Your window will refresh and you’ll see the data. Notice that the key is not present in the generated HTML.
保存文件。 您的窗口将刷新,您将看到数据。 请注意,该键在生成HTML中不存在。
Combining JSX with standard JavaScript gives you a lot of tools to create content dynamically, and you can use any standard JavaScript you want. In this step, you replaced hard-coded JSX with an array and a loop to create HTML dynamically. In the next step, you’ll conditionally show information using short circuiting.
将JSX与标准JavaScript结合使用可为您提供许多用于动态创建内容的工具,并且您可以使用所需的任何标准JavaScript。 在此步骤中,您用数组和循环替换了硬编码的JSX以动态创建HTML。 在下一步中,您将有条件地使用短路显示信息。
步骤5 —有条件地显示具有短路的元素 (Step 5 — Conditionally Showing Elements with Short Circuiting)
In this step, you’ll use short circuiting to conditionally show certain HTML elements. This will let you create components that can hide or show HTML based on additional information giving your components flexibility to handle multiple situations.
在此步骤中,您将使用短路条件有条件地显示某些HTML元素。 这样,您就可以基于其他信息创建可以隐藏或显示HTML的组件,从而使您的组件可以灵活地处理多种情况。
There are times when you will need a component to show information in some cases and not others. For example, you may only want to show an alert message for the user if certain cases are true, or you may want to display some account information for an admin that you wouldn’t want a normal user to see.
有时在某些情况下您需要一个组件来显示信息,而在其他情况下则不需要。 例如,您可能只想在某些情况属实的情况下为用户显示警报消息,或者您可能希望为管理员显示一些普通用户不希望看到的帐户信息。
To do this you will use short circuting. This means that you will use a conditional, and if the first part is truthy, it will return the information in the second part.
为此,您将使用短循环 。 这意味着您将使用条件语句,并且如果第一部分为真,则它将在第二部分中返回信息。
Here’s an example. If you wanted to show a button only if the user was logged in, you would surround the element with curly braces and add the condition before.
这是一个例子。 如果只想在用户登录后才显示按钮,则可以用大括号括住元素并在前面加上条件。
{isLoggedIn && <button>Log Out</button>}
In this example, you are using the &&
operator, which returns the last value if everything is truthy. Otherwise, it returns false
, which will tell React to return no additional markup. If isLoggedIn
is truthy, React will display the button. If isLoggedIn
is falsy, it will not show the button.
在此示例中,您使用的是&&
运算符,如果一切正常,则返回最后一个值。 否则,它返回false
,这将告诉React不返回额外的标记。 如果isLoggedIn
是真实的,React将显示该按钮。 如果isLoggedIn
错误,则不会显示该按钮。
To try this out, add the following highlighted lines:
要尝试此操作,请添加以下突出显示的行:
import React from 'react';
import './App.css';
...
function App() {
const greeting = "greeting";
const displayAction = false;
return(
<div className="container">
<h1 id={greeting}>Hello, World</h1>
{displayAction && <p>I am writing JSX</p>}
<ul>
...
</ul>
</div>
)
}
export default App;
In your text editor, you created a variable called displayAction
with a value of false.
You then surrounded the <p>
tag with curly braces. At the start of the curly braces, you added displayAction &&
to create the conditional.
在文本编辑器中,您创建了一个名为false.
的变量displayAction
false.
然后,您用花括号将<p>
标记括起来。 在花括号的开头,添加了displayAction &&
以创建条件。
Save the file and you will see the element disappear in your browser. Crucially, it will also not appear in the generated HTML. This is not the same as hiding an element with CSS. It won’t exist at all in the final markup.
保存文件,您将看到元素在浏览器中消失。 至关重要的是,它也不会出现在生成HTML中。 这与使用CSS隐藏元素不同。 它在最终标记中根本不存在。
Right now the value of displayAction
is hard-coded, but you can also store that value as a state or pass it as a prop from a parent component.
现在, displayAction
的值是硬编码的,但是您也可以将该值存储为状态,或者将其作为prop从父组件传递。
In this step, you learned how to conditionally show elements. This gives you the ability to create components that are customizable based on other information.
在这一步中,您学习了如何有条件地显示元素。 这使您能够创建可基于其他信息进行自定义的组件。
结论 (Conclusion)
At this point, you’ve created a custom application with JSX. You’ve learned how to add HTML-like elements to your component, add styling to those elements, pass attributes to create semantic and accessible markup, and add events to the components. You then mixed JavaScript into your JSX to reduce duplicate code and to conditionally show and hide elements.
至此,您已经使用JSX创建了一个自定义应用程序。 您已经了解了如何向组件中添加类似HTML的元素,为这些元素添加样式,如何传递属性以创建语义和可访问标记以及如何向组件添加事件。 然后,您将JavaScript混合到JSX中,以减少重复的代码并有条件地显示和隐藏元素。
This is the basis you need to make future components. Using a combination of JavaScript and HTML, you can build dynamic components that are flexible and allow your application to grow and change.
这是制作未来组件所需的基础。 结合使用JavaScript和HTML,您可以构建灵活的动态组件,并允许您的应用程序增长和更改。
If you’d like to learn more about React, check out our React topic page.
如果您想了解更多有关React的信息,请查看我们的React主题页面 。
翻译自: https://www.digitalocean.com/community/tutorials/how-to-create-react-elements-with-jsx