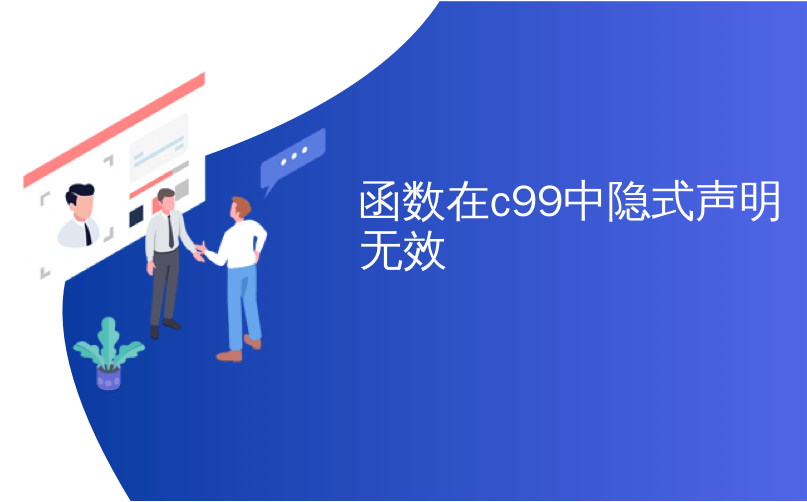
函数在c99中隐式声明无效
We are very much familiar with the flow control in C where it follows the Top-Down approach, and when we are writing a C program and if we are using any function, we might have come across a very common error ‘Implicit declaration of function’.
我们非常熟悉C中遵循自顶向下方法的流控制,并且当我们编写C程序并且使用任何函数时,我们可能会遇到一个非常常见的错误“函数的隐式声明” '。
Now why this error occurred? The answer is already in the error.
现在为什么会发生此错误? 答案已经在错误中。
We have used a function in our program which is not declared yet or we can say that we have used a function implicitly.
我们在程序中使用了尚未声明的函数,或者可以说我们隐式使用了函数。
Implicit declaration of the function is not allowed in C programming. Every function must be explicitly declared before it can be called.
C编程中不允许隐式声明函数。 必须先明确声明每个函数,然后才能调用它。
In C90, if a function is called without an explicit declaration, the compiler is going to complain about the implicit declaration.
在C90中,如果在没有显式声明的情况下调用函数,则编译器将抱怨隐式声明。
Here is a small code that will give us an Implicit declaration of function error.
这是一个小代码,它将为我们提供函数错误的隐式声明。
#include <stdio.h>
int main(void) {
int a = 10;
int b = 20;
printf("The value of %d + %d is %d",a, b, addTwo(10, 20));
return 0;
}
Now the above code will give you an error of Implicit declaration.
现在,上面的代码将给您一个隐式声明错误。
clang-7 -pthread -lm -o main main.c
main.c:6:45: warning: implicit declaration of function
'addTwo' is invalid in C99
[-Wimplicit-function-declaration]
printf("The value of %d + %d is %d",a, b, addTwo(10...
^
1 warning generated.
/tmp/main-c6e933.o: In function `main':
main.c:(.text+0x38): undefined reference to `addTwo'
clang-7compiler exit status 1
: error: linker command failed with exit code 1 (use -v to see invocation)
Here are some of the reasons why this is giving error.
这是造成错误的一些原因。
- Using a function that is pre-defined but you forget to include the header file for that function. 使用预定义的功能,但是您忘记包含该功能的头文件。
- If you are using a function that you have created but you failed to declare it in the code. It’s better to declare the function before the main. 如果使用的是已创建的函数,但未能在代码中声明它。 最好在main之前声明该函数。
In C90, this error can be removed just by declaring your function before the main function.
在C90中,只需在主函数之前声明您的函数即可消除此错误。
For example:
例如:
#include <stdio.h>
int yourFunctionName(int firstArg, int secondArg);
int main() {
// your code here
// your function call
}
int yourFunctionName(int firstArg, int secondArg) {
// body of the function
}
In the case of C99, you can skip the declaration but it will give us a small warning and it can be ignored but the definition of the function is important.
在C99的情况下,可以跳过该声明,但是会给我们一个小的警告,可以忽略它,但是函数的定义很重要。
#include <stdio.h>
// optional declaration
int main(void) {
int a = 10;
int b = 20;
printf("The value of %d + %d is %d",a, b, addTwo(10, 20));
return 0;
}
int addTwo(int a, int b) {
return a + b;
}
This gives us the output:
这给了我们输出:
clang-7 -pthread -lm -o main main.c
main.c:6:45: warning: implicit declaration of function
'addTwo' is invalid in C99
[-Wimplicit-function-declaration]
printf("The value of %d + %d is %d",a, b, addTwo(10...
^
1 warning generated.
./main
The value of 10 + 20 is 30
Here you can see that our code is working fine and a warning is generated but we are good to go but this is not recommended.
在这里,您可以看到我们的代码运行正常,并生成了警告,但是我们很高兴这样做,但是不建议这样做 。
Well, this was all about the Implicit declaration of the function in C and the error related to it. If you stuck into any kind of error, don’t forget to google it and try to debug it on your own. This will teach you how to debug on your own as someone might have faced a similar problem earlier.
好吧,这全都与C中函数的隐式声明以及与之相关的错误有关。 如果您遇到任何类型的错误,请别忘了用Google搜索并尝试自行调试。 这将教您如何自行调试,因为之前可能有人遇到过类似的问题。
If you still don’t find any solution for your problem, you can ask your doubt in the comment’s section below and we’ll get back to you🤓.
如果您仍然找不到解决问题的方法,可以在下面的评论部分中提出疑问,我们会尽快与您联系🤓。
Thanks for your visit and if you are new here, consider subscribing to our newsletter. See you in my next post. Bye!
感谢您的访问,如果您是这里的新手,请考虑订阅我们的时事通讯。 在下一篇文章中见。 再见!
翻译自: https://www.thecrazyprogrammer.com/2020/06/implicit-declaration-of-function-in-c.html
函数在c99中隐式声明无效