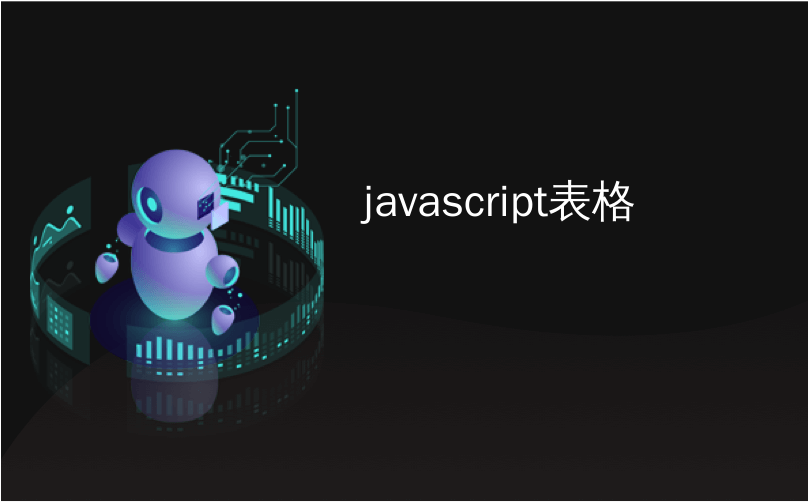
javascript表格
In this tutorial, I will tell you how to make student registration form in html with javascript validation.
在本教程中,我将告诉您如何使用javascript验证来制作html学生注册表格。
Javascript is basically used to validate HTML pages in web application. Validations are basically some rules to follow when inputting values to register on-site. Validation can be anything like:
Javascript基本上用于验证Web应用程序中HTML页面。 输入值进行现场注册时,验证基本上是要遵循的一些规则。 验证可以是:
- Some input fields cannot be empty. 某些输入字段不能为空。
- Some values must be in a particular length range. 一些值必须在特定的长度范围内。
- Some input fields must match (for example, password fields). These are some basic rules that you can make. 某些输入字段必须匹配(例如,密码字段)。 这些是您可以制定的一些基本规则。
- Validations are just simple things like making fields mandatory. 验证只是简单的事情,例如使字段成为必填项。
With the help of validations, we can achieve a lot. We can show the message to the client. You can display this message in the alert box as an error.
借助验证,我们可以取得很多成就。 我们可以向客户显示消息。 您可以在警报框中将此消息显示为错误。
So when all fields are filled accurately the user will able to submit the form means add the data otherwise it will show errors. Purpose of validations is to make sure that data must be correct before submitting the form if the user forgets to add anything.
因此,当正确填写所有字段时,用户将能够提交表格,即添加数据,否则将显示错误。 验证的目的是确保如果用户忘记添加任何内容,则在提交表单之前确保数据必须正确。
Here I will take a simple example of the student registration form which contains different types of buttons like radio, reset, submit. So that you can get full overview of the validity of the form.
在这里,我将举一个简单的学生注册表格示例,其中包含不同类型的按钮,例如单选,重置和提交。 这样您就可以完整了解表单的有效性。
In this, each button has a different role according to it we put some validations. So, we are going to perform form validation on all input fields and buttons.
在此,每个按钮根据其角色都有不同的作用,我们对此进行了一些验证。 因此,我们将对所有输入字段和按钮执行表单验证。
具有JavaScript验证HTML学生注册表格 (Student Registration Form in HTML with JavaScript Validation)
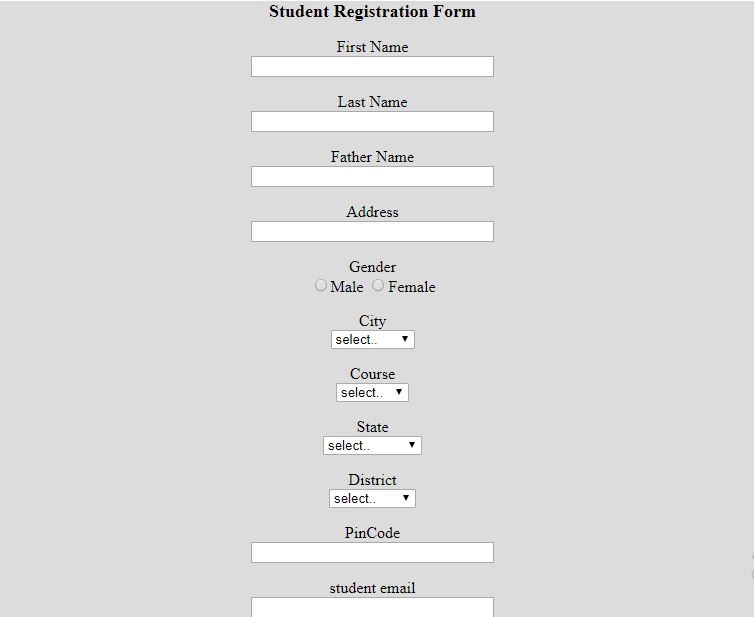
index.html (index.html)
<html>
<head>
<script type="text/javascript" src="validateform.js"></script>
<style>
ul {list-style-type:none;}
form{
background-color: #DCDCDC;
}
</style>
</head>
<body>
<form action="#" name="StudenSignupForm" onsubmit="return(validateHTMlform());">
<div cellpadding="2" width="20%" bgcolor="99FFFF" align="center"
cellspacing="2">
<ul>
<li>
<center><font size=4><b>Student Registration Form</b></font></center>
</li>
</ul>
<ul>
<li>First Name</li>
<li><input type=text name=textnames id="textname" size="30"></li>
</ul>
<ul>
<li>
Last Name</li>
<li><input type=text name=lastnames id="lastname" size="30"></li>
</ul>
<ul>
<li>Father Name</li>
<li><input type="text" name="full_father_name" id="fathername"
size="30"></li>
</ul>
<ul>
<li>Address</li>
<li><input type="text" name="personal_address"
id="personaladdress" size="30"></li>
</ul>
<ul>
<li>Gender</li>
<li><input type="radio" name="sex" value="male" size="10">Male
<input type="radio" name="sex" value="Female" size="10">Female</li>
</ul>
<ul>
<li>City</li>
<li><select name="City">
<option value="-1" selected>select..</option>
<option value="KOLKATA">KOLKATA</option>
<option value="CHENNAI">CHENNAI</option>
<option value="PUNE">PUNE</option>
<option value="JAIPUR">JAIPUR</option>
</select></li>
</ul>
<ul>
<li>Course</li>
<li><select name="Course">
<option value="-1" selected>select..</option>
<option value="B.Tech">B.TECH</option>
<option value="MCA">MCA</option>
<option value="MBA">MBA</option>
<option value="BCA">BCA</option>
</select></li>
</ul>
<ul>
<li>State</li>
<li><select Name="State">
<option value="-1" selected>select..</option>
<option value="New Delhi">NEW DELHI</option>
<option value="Mumbai">MUMBAI</option>
<option value="Goa">GOA</option>
<option value="Bihar">BIHAR</option>
</select></li>
</ul>
<ul>
<li>District</li>
<li><select name="Disulict">
<option value="-1" selected>select..</option>
<option value="Nalanda">NALANDA</option>
<option value="UP">UP</option>
<option value="Goa">GOA</option>
<option value="Patna">PATNA</option>
</select></li>
</ul>
<ul>
<li>PinCode</li>
<li><input type="text" name="pin_code" id="pincode" size="30"></li>
</ul>
<ul>
<li>student email</li>
<li><input type="text" name="email_id" id="emailid" size="30"></li>
</ul>
<ul>
<li>Date Of Birth</li>
<li><input type="text" name="date_of_birth" id="dob" size="30"></li>
</ul>
<ul>
<li>Mobile Number</li>
<li><input type="text" name="mobilenumber" id="mobile_no" size="30"></li>
</ul>
<ul>
<li><input type="reset"></li>
<li colspan="2"><input type="submit" value="Submit Form" /></li>
</ul>
</div>
</form>
</body>
</html>
validateform.js (validateform.js)
function validateHTMlform()
{
let form = document.StudenSignupForm;
if( form.textnames.value == "" )
{
alert( "Enter Your First Name!" );
form.textnames.focus() ;
return;
}
if( form.lastnames.value == "" )
{
alert( "Enter Your Last Name!" );
form.textnames.focus() ;
return;
}
if( form.fathername.value == "" )
{
alert( "Enter Your Father Name!" );
form.fathername.focus() ;
return;
}
if( form.paddress.value == "" )
{
alert( "Enter Your Postal Address!" );
form.paddress.focus() ;
return;
}
if( form.personaladdress.value == "" )
{
alert( "Enter Your Personal Address!" );
form.personaladdress.focus() ;
return;
}
if ( ( StudenSignupForm.sex[0].checked == false ) && ( StudenSignupForm.sex[1].checked == false ) )
{
alert ( "Choose Your Gender: Male or Female" );
return false;
}
if( form.City.value == "-1" )
{
alert( "Enter Your City!" );
form.City.focus() ;
return;
}
if( form.Course.value == "-1" )
{
alert( "Enter Your Course!" );
return;
}
if( form.District.value == "-1" )
{
alert( "Select Your District!" );
return;
}
if( form.State.value == "-1" )
{
alert( "Select Your State!" );
return;
}
if( form.pincode.value == "" ||
isNaN( form.pincode.value) ||
form.pincode.value.length != 6 )
{
alert( "Enter your pincode in format ######." );
form.pincode.focus() ;
return;
}
var email = form.emailid.value;
atpos = email.indexOf("@");
dotpos = email.lastIndexOf(".");
if (email == "" || atpos < 1 || ( dotpos - atpos < 2 ))
{
alert("Enter your correct email ID")
form.emailid.focus() ;
return;
}
if( form.dob.value == "" )
{
alert( "Enter your DOB!" );
form.dob.focus() ;
return;
}
if( form.mobileno.value == "" ||
isNaN( form.mobileno.value) ||
form.mobileno.value.length != 10 )
{
alert( "Enter your Mobile No. in the format 123." );
form.mobileno.focus() ;
return;
}
return( true );
}
I hope you got an idea how to make student registration form in html with javascript validation. Comment down below if you have any queries.
希望您对如何使用javascript验证制作html学生注册表格有所了解。 如果您有任何疑问,请在下面注释掉。
javascript表格