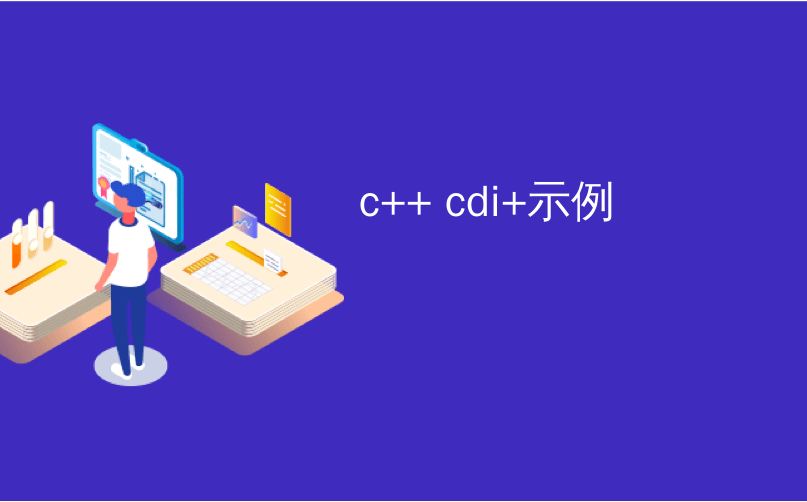
c++ cdi+示例
In this tutorial, we are going to see how object slicing in C++. Before diving into the topic let us revise two simple concepts of object oriented programming.
在本教程中,我们将了解如何在C ++中进行对象切片。 在深入探讨该主题之前,让我们修改一下面向对象编程的两个简单概念 。
- Inheritance 遗产
- Polymorphism 多态性
Inheritance is said to be an IS-A relationship. For e.g.
继承被称为IS-A关系。 例如
class Animal {
// properties common to all animals
}
class Tiger: public Animal {
// properties specific to the tiger.
}
Here Tiger class will inherit all the properties of the animal class and will add some more properties which are specific to Tiger to it. Here, therefore, we can say that:
在这里,Tiger类将继承动物类的所有属性,并将为其添加更多特定于Tiger的属性。 因此,在这里,我们可以这样说:
Every Tiger IS-A Animal
每只老虎都是动物
So while writing code an animal class reference can be used to point to tiger class. This same phenomenon can be used in code which is known as polymorphism when one object can take different forms.
因此,在编写代码时,可以使用动物类引用来指向老虎类。 当一个对象可以采用不同的形式时,可以在称为多态性的代码中使用相同的现象。
C ++对象切片 (C++ Object Slicing)
Object slicing refers to the property of object losing some of its properties when it is pointed by the reference of its parent class. The additional attributes of the derived class are sliced off of the object. In our above example if we write:
对象切片指的是对象的属性在其父类的引用指向它时失去其某些属性。 派生类的其他属性从对象中切出。 在上面的示例中,如果我们写:
Tiger t;
Animal a = t;
Then the object will not have any properties of tiger, the properties specific to the tiger will be sliced off and a will now only contain the properties common to all animals.
这样,该对象将不具有老虎的任何属性,该老虎特有的属性将被切掉,而a将仅包含所有动物共有的属性。
Let’s take a simple example:
让我们举一个简单的例子:
#include <iostream>
using namespace std;
class Base {
public :
int x,y;
int return10() {
return 10;
}
Base() {
x = 1;
y = 2;
}
};
class Derived : public Base {
public :
int z;
int return10() {
return 100;
}
Derived() {
z = 3;
}
};
int main() {
Derived d;
cout<<d.return10()<<" "<<d.x<<" "<<d.z<<endl;
d.x = 5;
Base b = d,a;
cout<<"Size of original Derived object : "<<sizeof(d)<<endl;
cout<<"Size of original Base object : "<<" "<<sizeof(a)<<endl;
cout<<"Size of original Sliced object : "<<sizeof(b)<<endl;
cout<<d.return10()<<" "<<d.x<<" "<<d.z<<endl;
cout<<b.return10()<<" "<<b.x<<endl;
return 0;
}
Output:
输出:
100 1 3 Size of original Derived object : 12 Size of original Base object : 8 Size of original Sliced object : 8 100 5 3 10 5
100 1 3 原始派生对象的 大小 :12 原始基础对象的 大小 :8 原始切片对象的大小:8 100 5 3 10 5
If we change the line:
如果我们更改行:
cout<<b.return10()<<" "<<b.x<<endl;
to
至
cout<<b.return10()<<" "<<b.z<<endl;
we will get an error saying:
我们会收到一条错误消息:
error: ‘class Base’ has no member named ‘z’
错误:“基本类”没有名为“ z”的成员
Here the object b is sliced copy of object d. The same is evident from the size of the objects.
在这里,对象b是对象d的切片副本。 从物体的大小可以看出相同的结果。
Object slicing can be prevented if use pointers or references the example below depicts the same.
如果使用指针或引用的示例在下面的示例中进行了描述,则可以防止对象切片。
Keeping all the other code same if we change the main function to:
如果我们将main函数更改为:,则使所有其他代码保持相同:
int main() {
Derived *pointerd = new Derived();
Base *pointerb = new Base();
Base *inheritedb = new Derived();
cout<<pointerd->x<<" "<<pointerd->z<<endl;
cout<<inheritedb->x<<endl;
return 0;
}
Output:
输出:
1 3 1
1 3 1
Here also we cant directly use:
这里我们也不能直接使用:
cout<<inheritedb->z<<endl;
Which will give us the same error instead what we can do is first cast the pointer of Base class to a Derived class pointer and then access the z variable which will look as below:
这将给我们带来相同的错误,而不是我们可以做的是首先将基类的指针转换为派生类的指针,然后访问z变量,该变量如下所示:
int main() {
Derived *pointerd = new Derived();
Base *pointerb = new Base();
Base *inheritedb = new Derived();
cout<<pointerd->x<<" "<<pointerd->z<<endl;
cout<<((Derived *)inheritedb)->x<<endl;
return 0;
}
Output:
输出:
1 3 1
1 3 1
Note that if we try to print the size of pointerd or any other reference we will get the same size as pointers to any type or class will take the same size. Here, therefore, the objects are not sliced but the properties are hidden from the user which can be accessed after doing a simple cast.
请注意,如果我们尝试打印指针或任何其他引用的大小,我们将得到与指向任何类型或类的指针相同的大小。 因此,此处未对对象进行切片,但对用户隐藏了属性,可以在执行简单的转换后访问这些属性。
Comment down below if you have any queries related to object slicing in C++.
如果您对C ++中的对象切片有任何疑问,请在下面注释掉。
翻译自: https://www.thecrazyprogrammer.com/2020/04/c-object-slicing.html
c++ cdi+示例