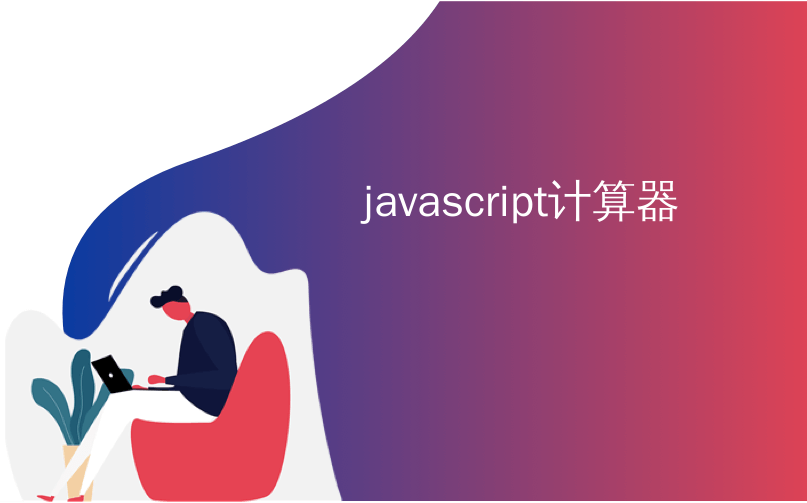
javascript计算器
In this tutorial, I will tell you two ways to make a calculator using basic HTML, CSS and JavaScript. In first way we make functions for all operations and in the second we use some inbuilt global function to make a calculator. In both cases we are going to add some simple math operations like addition, subtraction, multiplication, division in our basic calculator. This project will help you to improve your logical ability and coding skills.
在本教程中,我将告诉您两种使用基本HTML,CSS和JavaScript制作计算器的方法。 在第一种方式中,我们为所有操作创建函数,在第二种方式中,我们使用一些内置的全局函数来生成计算器。 在这两种情况下,我们都将在基本计算器中添加一些简单的数学运算,如加,减,乘,除。 该项目将帮助您提高逻辑能力和编码能力。
JavaScript计算器示例 (JavaScript Calculator Example)
方法1: (Method 1:)
Mainly the functions that we need to work for calculator are handling the keypresses, inputting the digits, inputting the decimal point/key, handling operators, resisting the calculators, updating the display, equal key and clear key.
我们需要为计算器工作的主要功能是处理按键,输入数字,输入小数点/键,处理运算符,抵制计算器,更新显示,等键和清除键。
As we know calculator consists of keys and display. So, in the UI perspective we have to work on:
众所周知,计算器由按键和显示组成。 因此,在UI角度,我们必须致力于:
calculator tile – where we show the title
计算器图块–显示标题的位置
input buttons – where we display all keys
输入按钮–我们在其中显示所有键
output screen – This is the screen where we can check what input we are entering and showing all the values that we entered.
输出屏幕–这是我们可以检查输入的内容并显示所有输入值的屏幕。
Before starting this Project you must have knowledge or idea of these things:
在开始本项目之前,您必须具有以下知识或想法:
- if-else statements if-else语句
- javascript functions javascript函数
- arrow function 箭头功能
- some operators like && and || 一些运算符,如&&和||
- change the text with the value attribute 使用value属性更改文本
- add event listeners 添加事件监听器
- querySelector querySelector
- string methods like includes, contains etc. 字符串方法(包括,包含等)
- eval function 评估功能
So, coding part begins here for the first case. Initially, we have to make a div container in which we are going to add all buttons with proper styling. To make this UI we use CSS Grid box which is the best practice of writing proper responsive code. After this, we start work on functionalities.
因此,对于第一种情况,编码部分从这里开始。 最初,我们必须制作一个div容器,在其中添加所有具有正确样式的按钮。 为了创建此UI,我们使用CSS Grid box,这是编写适当的响应代码的最佳实践。 之后,我们开始进行功能性工作。
Firstly we have to initialise the value of each variable and constant. Then we check what key is pressed by the user like operators, operands, decimal or clear according to this key we store it in different variables that we declared. So, when the user hits number keys then we store these values in operands. We define the separate function for each operation like inputting the digit, updating the display, decimal enter etc.
首先,我们必须初始化每个变量和常量的值。 然后,我们根据该键检查用户按下的键,例如运算符,操作数,十进制或清除键,然后将其存储在声明的不同变量中。 因此,当用户按下数字键时,我们会将这些值存储在操作数中。 我们为每个操作定义了单独的功能,例如输入数字,更新显示,输入小数等。
You can check the whole code below.
您可以在下面查看整个代码。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<style>
html {
font-size: 62.5%;
box-sizing: border-box;
}
*, *::before, *::after {
margin: 0;
padding: 0;
box-sizing: inherit;
}
.calculator {
border: 1px solid #ccc;
border-radius: 5px;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 400px;
}
.output-screen {
width: 100%;
font-size: 5rem;
height: 80px;
border: none;
background-color: gray;
color: #fff;
text-align: right;
padding-right: 20px;
padding-left: 10px;
}
button {
height: 60px;
background-color: #fff;
border-radius: 3px;
border: 1px solid #c4c4c4;
background-color: transparent;
font-size: 2rem;
color: #333;
background-image: linear-gradient(to bottom,transparent,transparent 50%,rgba(0,0,0,.04));
box-shadow: inset 0 0 0 1px rgba(255,255,255,.05), inset 0 1px 0 0 rgba(255,255,255,.45), inset 0 -1px 0 0 rgba(255,255,255,.15), 0 1px 0 0 rgba(255,255,255,.15);
text-shadow: 0 1px rgba(255,255,255,.4);
}
button:hover {
background-color: #eaeaea;
}
.operator {
color: #337cac;
}
.clear-value {
background-color: green;
border-color: #b0353a;
color: #fff;
}
.clear-value:hover {
background-color: #f17377;
}
.calculate-value {
background-color: blue;
border-color: #337cac;
color: #fff;
height: 100%;
grid-area: 2 / 4 / 6 / 5;
}
.calculate-value:hover {
background-color: #4e9ed4;
}
.display-keys {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-gap: 20px;
padding: 20px;
}
</style>
<body>
<div class="calculator">
<h1 style="text-align: center;">Simple Javascript calculator</h1>
<input type="text" class="output-screen" value="" disabled />
<div class="display-keys">
<button class="operator" value="+">+</button>
<button class="operator" value="-">-</button>
<button class="operator" value="*">×</button>
<button class="operator" value="/">÷</button>
<button value="7">7</button>
<button value="8">8</button>
<button value="9">9</button>
<button value="4">4</button>
<button value="5">5</button>
<button value="6">6</button>
<button value="1">1</button>
<button value="2">2</button>
<button value="3">3</button>
<button value="0">0</button>
<button class="decimal" value=".">.</button>
<button class="clear-value" value="clear-value">AC</button>
<button class="calculate-value operator" value="=">=</button>
</div>
</div>
</body>
<script>
const calculator = {
outputValue: '0',
firstValue: null,
flagForSecondOperand: false,
operator: null,
};
function inputDigit(digit) {
const { outputValue, flagForSecondOperand } = calculator;
if (flagForSecondOperand === true) {
calculator.outputValue = digit;
calculator.flagForSecondOperand = false;
} else {
calculator.outputValue = outputValue === '0' ? digit : outputValue + digit;
}
}
function inputDecimal(dot) {
if (calculator.flagForSecondOperand === true) return;
// If the `outputValue` does not contain a decimal point
if (!calculator.outputValue.includes(dot)) {
// Append the decimal point
calculator.outputValue += dot;
}
}
function handleOperator(nextOperator) {
const { firstValue, outputValue, operator } = calculator
const inputValue = parseFloat(outputValue);
if (operator && calculator.flagForSecondOperand) {
calculator.operator = nextOperator;
return;
}
if (firstValue == null) {
calculator.firstValue = inputValue;
} else if (operator) {
const currentValue = firstValue || 0;
const result = performCalculation[operator](currentValue, inputValue);
calculator.outputValue = String(result);
calculator.firstValue = result;
}
calculator.flagForSecondOperand = true;
calculator.operator = nextOperator;
}
const performCalculation = {
'/': (firstValue, secondOperand) => (firstValue / secondOperand).toFixed(5),
'*': (firstValue, secondOperand) => firstValue * secondOperand,
'+': (firstValue, secondOperand) => firstValue + secondOperand,
'-': (firstValue, secondOperand) => firstValue - secondOperand,
'=': (firstValue, secondOperand) => secondOperand
};
function resetCalculator() {
calculator.outputValue = '0';
calculator.firstValue = null;
calculator.flagForSecondOperand = false;
calculator.operator = null;
}
function updateDisplay() {
const display = document.querySelector('.output-screen');
display.value = calculator.outputValue;
}
updateDisplay();
const keys = document.querySelector('.display-keys');
keys.addEventListener('click', (event) => {
const { target } = event;
if (!target.matches('button')) {
return;
}
if (target.classList.contains('operator')) {
handleOperator(target.value);
updateDisplay();
return;
}
if (target.classList.contains('decimal')) {
inputDecimal(target.value);
updateDisplay();
return;
}
if (target.classList.contains('clear-value')) {
resetCalculator();
updateDisplay();
return;
}
inputDigit(target.value);
updateDisplay();
});
</script>
</html>
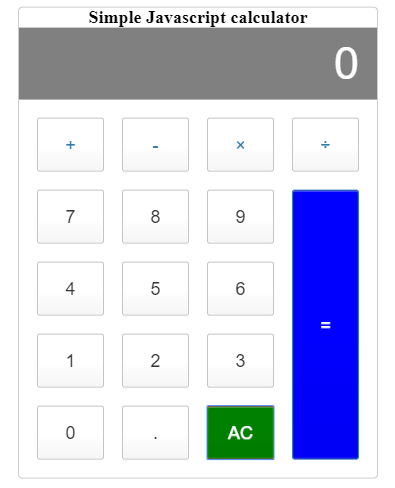
方法2: (Method 2:)
We can also make a JavaScript calculator using the eval function. This function is used to evaluate any string. This is a built-in function of JS.
我们还可以使用eval函数制作一个JavaScript计算器。 此函数用于评估任何字符串。 这是JS的内置功能。
Here we used the table tag to make UI and for adding additional functions we use form HTML element. On each keypress, we put on click method to add that value to the output screen. And after this eval function evaluate all values when you click on any operator.
在这里,我们使用table标签制作UI,并使用HTML元素添加其他功能。 在每次按键时,我们都采用click方法将其值添加到输出屏幕。 在此eval函数之后,当您单击任何运算符时,将评估所有值。
<form name="calculator">
<table>
<tr>
<td colspan="4">
<input type="text" name="display" id="display" disabled>
</td>
</tr>
<tr>
<td><input type="button" name="one" value="1" onclick="calculator.display.value += '1'"></td>
<td><input type="button" name="two" value="2" onclick="calculator.display.value += '2'"></td>
<td><input type="button" name="three" value="3" onclick="calculator.display.value += '3'"></td>
<td><input type="button" class="operator" name="plus" value="+" onclick="calculator.display.value += '+'"></td>
</tr>
<tr>
<td><input type="button" name="four" value="4" onclick="calculator.display.value += '4'"></td>
<td><input type="button" name="five" value="5" onclick="calculator.display.value += '5'"></td>
<td><input type="button" name="six" value="6" onclick="calculator.display.value += '6'"></td>
<td><input type="button" class="operator" name="minus" value="-" onclick="calculator.display.value += '-'"></td>
</tr>
<tr>
<td><input type="button" name="seven" value="7" onclick="calculator.display.value += '7'"></td>
<td><input type="button" name="eight" value="8" onclick="calculator.display.value += '8'"></td>
<td><input type="button" name="nine" value="9" onclick="calculator.display.value += '9'"></td>
<td><input type="button" class="operator" name="times" value="x" onclick="calculator.display.value += '*'"></td>
</tr>
<tr>
<td><input type="button" name="zero" value="0" onclick="calculator.display.value += '0'"></td>
<td><input type="button" name="doit" value="=" onclick="calculator.display.value = eval(calculator.display.value)"></td>
<td><input type="button" class="operator" name="div" value="." onclick="calculator.display.value += '.'"></td>
<td><input type="button" class="operator" name="div" value="/" onclick="calculator.display.value += '/'"></td>
</tr>
</table>
<input type="button" id="clear" name="clear" value="clear" style="width:12%" onclick="calculator.display.value = ''">
</form>
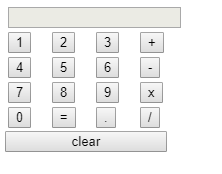
Comment down below if you have any queries related to javascript calculator.
如果您有任何与javascript计算器相关的查询,请在下面注释掉。
翻译自: https://www.thecrazyprogrammer.com/2019/10/javascript-calculator-example.html
javascript计算器