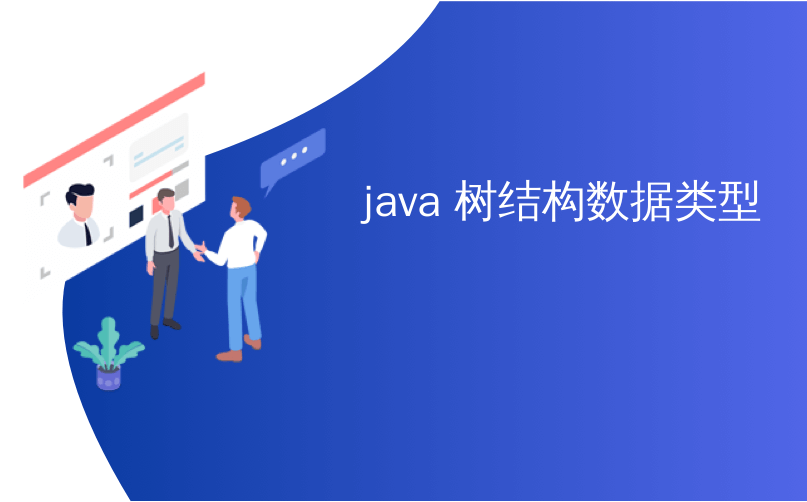
java 树结构数据类型
In this article, we will learn about tree and some of the common types of trees in data structure.
在本文中,我们将学习树以及数据结构中一些常见的树类型。
Tree in computer science is like a tree in the real world, the only difference is that in computer science it is visualized as upside-down with root on the top and branches originating from the root to the leaves of the tree. Tree Data Structure is used for various real-world applications as it can show relation among various nodes using the parent-child hierarchy. Due to this it is also known as hierarchical data structure. It is widely used to simplify and fasten searching and sorting operations. It is considered to be one of the most powerful and advanced data structures.
计算机科学中的树就像现实世界中的一棵树,唯一的区别是计算机科学中的树是倒置的,其根在顶部,而分支从树的根部到叶部。 树数据结构可用于各种实际应用程序,因为它可以使用父子层次结构显示各种节点之间的关系。 因此,它也被称为分层数据结构。 它被广泛用于简化和加快搜索和排序操作。 它被认为是最强大和最先进的数据结构之一。
Tree is a non-linear data structure. A tree can be represented using various primitive or user defined data types. To implement tree, we can make use of arrays, linked lists, classes or other types of data structures. It is a collection of nodes that are related with each other. To show the relation, nodes are connected with edges.
树是一种非线性数据结构。 可以使用各种原始或用户定义的数据类型来表示树。 为了实现树,我们可以利用数组,链表,类或其他类型的数据结构。 它是彼此相关的节点的集合。 为了显示这种关系,节点与边连接。
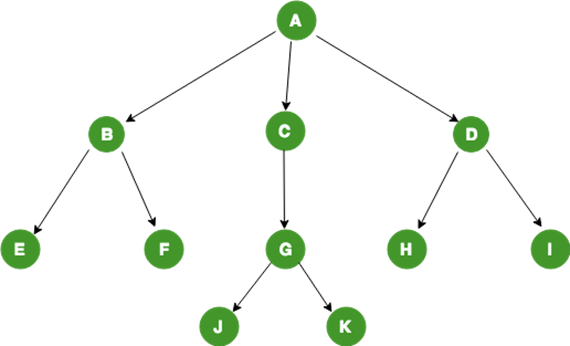
Fig 1: General Tree structure (Source)
图1:常规树结构( 源 )
Relations in a Tree:
树中的关系:
- A is the root of the tree A是树的根
- A is Parent of B, C and D A是B,C和D的父母
- B is child of A B是A的孩子
- B, C and D are siblings B,C和D是兄弟姐妹
- A is grand-parent of E, F, G, H and I A是E,F,G,H和I的祖父母
Properties of Tree:
树的属性:
- Every tree has a special node called the root node. The root node can be used to traverse every node of the tree. It is called root because the tree originated from root only. 每棵树都有一个称为根节点的特殊节点。 根节点可用于遍历树的每个节点。 之所以称为根,是因为该树仅起源于根。
- If a tree has N vertices(nodes) than the number of edges is always one less than the number of nodes(vertices) i.e N-1. If it has more than N-1 edges it is called a graph not a tree. 如果一棵树有N个顶点(节点),则边数总是比节点(顶点)的数量少1,即N-1。 如果它有N-1个以上的边,则称为图而不是树。
- Every child has only a single Parent but Parent can have multiple child. 每个孩子只有一个父母,但父母可以有多个孩子。
数据结构中的树类型 (Types of Trees in Data Structure)
普通树 (General Tree)
A tree is called a general tree when there is no constraint imposed on the hierarchy of the tree. In General Tree, each node can have infinite number of children. This tree is the super-set of all other types of trees. The tree shown in Fig 1 is a General Tree.
当树的层次结构上没有任何限制时,该树称为普通树。 在“通用树”中,每个节点可以具有无限数量的子代。 该树是所有其他类型树的超集。 图1所示的树是通用树。
二叉树 (Binary Tree)
Binary tree is the type of tree in which each parent can have at most two children. The children are referred to as left child or right child. This is one of the most commonly used trees. When certain constraints and properties are imposed on Binary tree it results in a number of other widely used trees like BST (Binary Search Tree), AVL tree, RBT tree etc. We will see all these types in details as we move ahead.
二叉树是每个父母最多可以有两个孩子的树的类型。 这些孩子被称为左孩子或右孩子。 这是最常用的树之一。 当对Binary树施加某些约束和属性时,它会导致许多其他广泛使用的树,例如BST(二进制搜索树),AVL树,RBT树等。随着我们的前进,我们将详细了解所有这些类型。
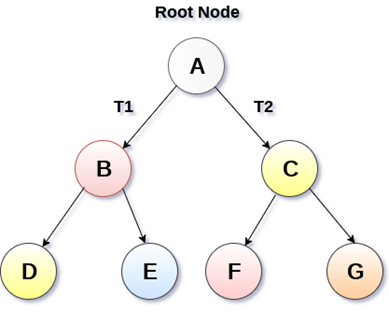
Fig 2: Binary Tree (Source)
无花果2:二叉树( 来源 )
二进制搜索树 (Binary Search Tree)
Binary Search Tree (BST) is an extension of Binary tree with some added constraints. In BST, the value of the left child of a node must be smaller than or equal to the value of its parent and the value of the right child is always larger than or equal to the value of its parent. This property of Binary Search Tree makes it suitable for searching operations as at each node we can decide accurately whether the value will be in left subtree or right subtree. Therefore, it is called a Search Tree.
二进制搜索树(BST)是二进制树的扩展,带有一些附加的约束。 在BST中,节点的左子节点的值必须小于或等于其父节点的值,而右子节点的值始终大于或等于其父节点的值。 二进制搜索树的此属性使其适合于搜索操作,因为在每个节点上,我们都可以准确地确定该值是在左子树中还是在右子树中。 因此,它称为搜索树。
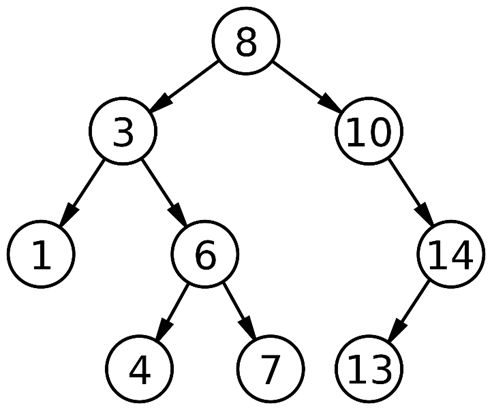
Fig 3: Binary Search Tree (Source)
无花果3:二叉树( Source )
AVL树 (AVL Tree)
AVL tree is a self-balancing binary search tree. The name AVL is given on the name of its inventors Adelson-Velshi and Landis. This was the first dynamically balancing tree. In AVL tree, each node is assigned a balancing factor based on which it is calculated whether the tree is balanced or not. In AVL tree, the heights of children of a node differ by at most 1. The valid balancing factor in AVL tree are 1, 0 and -1. When a new node is added to the AVL tree and tree becomes unbalanced then rotation is done to make sure that the tree remains balanced. The common operations like lookup, insertion and deletion takes O(log n) time in AVL tree. It is widely used for Lookup operations.
AVL树是一种自平衡二进制搜索树。 名称为AVL的是其发明者Adelson-Velshi和Landis的名字。 这是第一棵动态平衡树。 在AVL树中,为每个节点分配一个平衡因子,基于此平衡因子,可以计算树是否平衡。 在AVL树中,节点的子代的高度最多相差1。在AVL树中,有效的平衡因子为1、0和-1。 将新节点添加到AVL树后,树变得不平衡,然后进行旋转以确保树保持平衡。 查找,插入和删除等常见操作在AVL树中花费O(log n)时间。 它广泛用于查找操作。
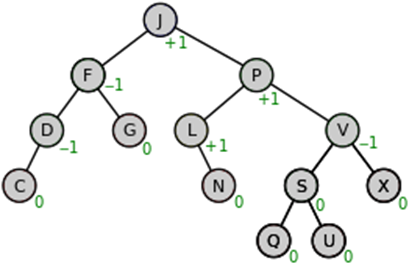
Fig 4: Source
图4: 来源
红黑树 (Red-Black Tree)
Red-Black is another type of self-balancing tree. The name Red-Black is given to it because each node in a Red-Black tree is either painted Red or Black according to the properties of the Red- Black Tree. This make sure that the tree remains balanced. Although the Red-Black tree is not a perfectly balanced tree but its properties ensure that the searching operation takes only O(log n) time. Whenever a new node is added to the Red-Black Tree, the nodes are rotated and painted again if needed to maintain the properties of the Red-Black Tree .
红黑是另一种自平衡树。 之所以使用名称Red-Black,是因为根据Red-Black树的属性,将Red-Black树中的每个节点涂成红色或黑色。 这样可以确保树保持平衡。 尽管红黑树不是完全平衡的树,但是它的属性可确保搜索操作仅花费O(log n)时间。 每当将新节点添加到Red-Black Tree时,如果需要维护Red-Black Tree的属性,就会旋转并再次绘制节点。
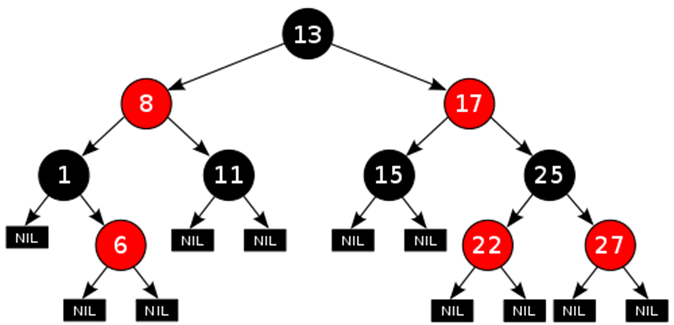
Fig 5: Red-Black Tree (Source)
图5:红黑树( 来源 )
一元树 (N-ary Tree)
In an N-ary tree, the maximum number of children that a node can have is limited to N. A binary tree is 2-ary tree as each node in binary tree has at most 2 children. Trie data structure is one of the most commonly used implementation of N-ary tree. A full N-ary tree is a tree in which children of a node is either 0 or N. A complete N-ary tree is the tree in which all the leaf nodes are at the same level.
在N元树中,一个节点可以具有的最大子节点数限制为N。由于二叉树中的每个节点最多具有2个子节点,因此二叉树是2元树。 Trie数据结构是N元树的最常用实现之一。 完整的N元树是其中节点的子节点为0或N的树。完整的N元树是其中所有叶节点处于同一级别的树。
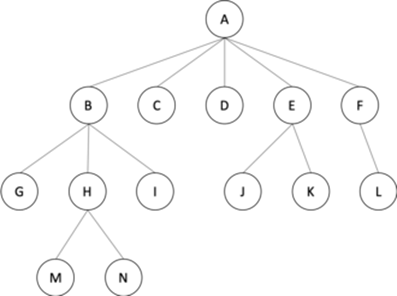
Fig 6: N-ary tree (5-ary)
图6:N元树(5元)
I hope you got the idea about some of the common types of trees in data structure. If you have any queries then feel free to ask in the comment section.
希望您对数据结构中一些常见的树类型有所了解。 如果您有任何疑问,请随时在评论部分提问。
翻译自: https://www.thecrazyprogrammer.com/2019/09/types-of-trees-in-data-structure.html
java 树结构数据类型