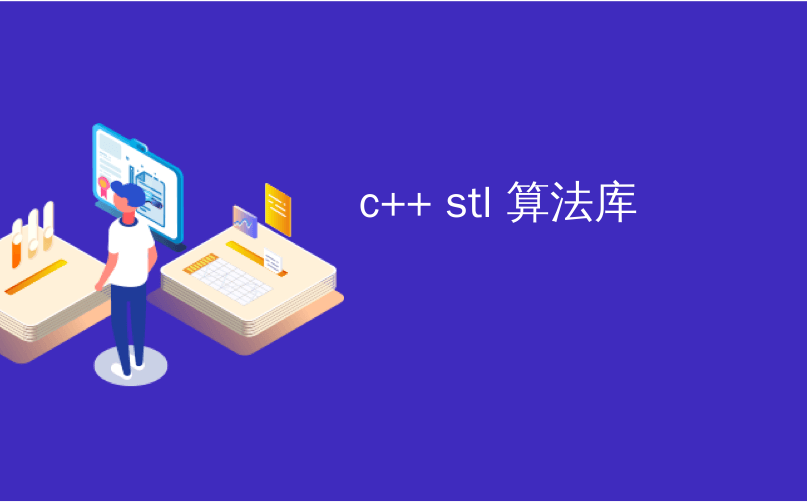
c++ stl 算法库
In this article you will learn about stl algorithm library in c++.
在本文中,您将了解stl算法 C ++中的库。
Are you a competitive programmer or very passionate programmer then you must know about STL algorithm library. It contains very rich set of algorithms. If you practice this well you can nail any programming interview. All inbuilt algorithms implemented in this library were implemented in the best complexity way. So using those is better than writing own code. It also saves time and code readability.
您是一个有竞争力的程序员还是一个非常热情的程序员,那么您必须了解STL算法库。 它包含非常丰富的算法集。 如果练习得好,您可以参加任何编程面试。 该库中实现的所有内置算法均以最佳复杂性方式实现。 因此,使用它们比编写自己的代码更好。 它还节省了时间和代码可读性。
C ++ STL算法库 (C++ STL Algorithm Library)
There are many of those algorithms. We will see some of them which mostly used.
这些算法有很多。 我们将看到其中一些最常用的。
To work with these we first need to include algorithm library. That is #include<algorithm>
要使用它们,我们首先需要包括算法库。 那是#include <algorithm>
In all previous articles we learned about containers, now we can apply these algorithms on those containers.
在之前的所有文章中,我们都了解了容器,现在我们可以将这些算法应用于这些容器。
Since main intention of algorithm is to retrieve information or to know some of properties, these will not do any modifications on container sizes or container storage. They just use iterators to apply on containers.
由于算法的主要目的是检索信息或了解某些属性,因此这些操作不会对容器大小或容器存储进行任何修改。 他们只是使用迭代器将其应用于容器。
min (a, b): This gives minimum value of a and b. Here conditions are both a and b must be same data type. In case if a and b are equal it gives same value.
分钟(a,b): 这给出了a和b的最小值。 这里的条件是a和b都必须是相同的数据类型。 如果a和b相等,则给出相同的值。
max (a,b) : This gives maximum value of a and b. Both a and b should be same data type.
max(a,b) :这给出a和b的最大值。 a和b都应该是相同的数据类型。
sort (first_iterator , last_iterator): Sorts the elements of a container between given vectors.
排序(first_iterator,last_iterator):在给定向量之间排序容器的元素。
Above sort sorting function used to sort containers only. Because they only have iterators. But for normal arrays. We have to use sort (array_begin, array_end):
上面的排序排序功能仅用于对容器进行排序。 因为它们只有迭代器。 但是对于普通数组。 我们必须使用sort(array_begin,array_end):
Example program to show above algorithms:
显示上述算法的示例程序:
//Standard library algorithms
#include <iostream>
#include <algorithm>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
int main(){
cout << "min(10,3) gives " << min(10,3) << "\n";
cout << "min(5,5) gives " << min(5,5) << "\n";
cout << "min ('A', 'B') gives" << min ('A' , 'B') << "\n";
cout << endl << "max(10,3) gives " << max(10,3) << "\n";
cout << "max(5,5) gives " << max(5,5) << "\n";
cout << "max ('A', 'B') gives" << max ('A' , 'B') << "\n";
vector <int> v;
vector <int> :: iterator it;
for(int i=0; i<5;i++) v.push_back(10-i);
cout << endl << "Elements of vector before sort " << endl;
for(it= v.begin(); it!=v.end();it++) cout << *it << " ";
cout << endl;
cout << "Performing sort(v.begin(), v.end()) " << endl;
sort(v.begin(), v.end());
cout << "After above function vector elements are " << endl;
for(it= v.begin(); it!=v.end(); it++) cout << *it << " " ;
cout << endl;
int ar[5];
for(int i=0; i<5 ;i++) ar[i]= 15-i;
cout << endl << "Array elements are " << endl;
for(int i=0; i<5; i++) cout << ar[i] << " " ;
cout << endl;
cout << "Performing sort(ar+0, ar+5) " << endl;
sort(ar+0, ar+5);
cout << "After above operation array elements are " << endl;
for(int i=0;i<5; i++) cout << ar[i] << " ";
cout << endl;
return 0;
}
Output
输出量
min(10,3) gives 3 min(5,5) gives 5 min (‘A’, ‘B’) givesA
min(10,3)给出3 min(5,5)给出5 分钟('A','B')得到A
max(10,3) gives 10 max(5,5) gives 5 max (‘A’, ‘B’) givesB
max(10,3)给10 max(5,5)给5 max('A','B')给B
Elements of vector before sort 10 9 8 7 6 Performing sort(v.begin(), v.end()) After above function vector elements are 6 7 8 9 10
排序之前的vector元素 10 9 8 7 6 执行sort(v.begin(),v.end()) 在上述函数之后,vector元素是 6 7 8 9 10
Array elements are 15 14 13 12 11 Performing sort(ar+0, ar+5) After above operation array elements are 11 12 13 14 15
数组元素为 15 14 13 12 11 执行sort(ar + 0,ar + 5) 在上述操作之后,数组元素为 11 12 13 14 15
find(): This will find given element present in container or not. Input parameters for this are range and element. Range contains two values, beginning of the range and ending of the range.
find():这将查找容器中是否存在给定的元素。 为此的输入参数是范围和元素。 范围包含两个值,范围的开始和范围的结束。
find() on arrays will be find(ar_base_address, ar_end, element);
数组上的find()将是find(ar_base_address,ar_end,element);
Similarly find() on other stl containers will be find(iterator_to_1st, iterator_to_last, element);
类似地,在其他stl容器上的find()将是find(iterator_to_1st,iterator_to_last,element);
One more thing we need to know about this find() is, if given element is present it points that element position, else it points to end of the container. This we can use for checking purpose.
关于find()我们需要了解的另一件事是,如果存在给定的元素,它将指向该元素的位置,否则它将指向容器的末端。 我们可以将其用于检查目的。
count (): This is same way of find() algorithm. But this returns number of times given element present in the given range of elements.
count():这与find()算法相同。 但这会返回给定元素在给定范围内的出现次数。
Same like find(), for arrays we need to give base address as range and for other STL containers like vector, dequeue, list, etc. give iterator positions as range.
与find()一样,对于数组,我们需要将基地址指定为范围,对于其他STL容器(例如向量,出队,列表等),则将迭代器位置指定为范围。
equal(): This is used to compare range of sequences. We will give some range as parameter to compare other range. It gives true if all elements equal in the range else returns false.
equal():用于比较序列范围。 我们将给出一个范围作为参数来比较其他范围。 如果范围内所有元素相等,则为true,否则返回false。
For example if give a vector and an array ranges, equal gives true if and only if ith element of vector and array are same. Where i is between given range.
例如,如果给定向量和数组范围,则当且仅当向量和数组的第i个元素相同时,equal才返回true。 我在给定范围之间。
Example program to show above algorithms:
显示上述算法的示例程序:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main(){
int ar[5];
for(int i=0;i<5; i++) ar[i]= i*i;
cout << "Array contains 0, 1, 4, 9, 16" << endl;
cout << "Performing find(ar+0, ar+5, 44) " << endl;
int *position= find(ar+0, ar+5, 44);
if(position == ar+5){
cout << "Element not found in the array " << endl;
}
else{
cout << "Element found in the array " << endl;
}
vector <int> vec;
vector <int> :: iterator it;
for(int i=0; i<5; i++) vec.push_back(i*i);
cout << endl << "Vector contains elements 0, 1, 4, 9, 16" << endl;
cout << "Performing find (vec.begin(), vec.end(), 4) " << endl;
it = find (vec.begin(), vec.end(), 4);
if(it == vec.end()){
cout << "Element not found in the vector " << endl;
}
else{
cout << "Element found in the vector " << endl;
}
ar[0]= vec[0]= 2;
ar[1]= vec[1]= 3;
ar[2]= vec[2]= 2;
ar[3]= vec[3]= 2;
ar[4]= vec[4]= 5;
cout << endl << "Array and vector contains elements 2, 3, 2, 2, 3" << endl;
cout << "Performing count (ar+0, ar+5, 2) on array " << endl;
int cnt= count(ar+0, ar+5, 2);
cout << "2 present " << cnt << " times in the array" << endl;
cout << "Performing count (vec.begin(), vec.end(), 3) on vector " << endl;
cnt= count(vec.begin(), vec.end(), 3);
cout << "3 present " << cnt << " times in the vector " << endl;
cout << endl << "elements of array are " << endl;
for(int i=0; i<5; i++) cout << ar[i] << " " ;
cout << endl;
cout << "elements in the vector are " << endl;
for(it=vec.begin(); it!=vec.end(); it++) cout << *it << " ";
cout << endl;
cout << "Now performing equal(vec.begin(), vec.end(), ar) " << endl;
bool chk = equal(vec.begin(), vec.end(), ar);
if(chk)
cout << "Both sequences are equal "<< endl;
else
cout << "Givens sequences are not equal " << endl;
return 0;
}
Output
输出量
Array contains 0, 1, 4, 9, 16 Performing find(ar+0, ar+5, 44) Element not found in the array
数组包含 0、1、4、9、16执行find(ar + 0,ar + 5、44) 在数组中找不到元素
Vector contains elements 0, 1, 4, 9, 16 Performing find (vec.begin(), vec.end(), 4) Element found in the vector
向量包含元素 0、1、4、9、16执行查找(vec.begin(),vec.end(),4) 在向量中找到的元素
Array and vector contains elements 2, 3, 2, 2, 3 Performing count (ar+0, ar+5, 2) on array 2 present 3 times in the array Performing count (vec.begin(), vec.end(), 3) on vector 3 present 1 times in the vector
数组和向量包含元素 2、3、2、2、3的值 数组 2 上的执行计数(ar + 0,ar + 5、2 )在数组中 出现3次 执行计数(vec.begin(),vec.end() ,3)在向量 3上出现1次
elements of array are 2 3 2 2 5 elements in the vector are 2 3 2 2 5 Now performing equal(vec.begin(), vec.end(), ar) Both sequences are equal
数组的元素是 2 3 2 2 5 向量中的元素是 2 3 2 2 5 现在执行equal(vec.begin(),vec.end(),ar) 两个序列都相等
reverse(start_, end_): This algorithm reverse the elements in given range.
reverse(start_,end_):此算法反转给定范围内的元素。
accumulate(start_, end_, initial_sum ): Accumulate is used to sum the all elements in the give range. It adds elements to initial sum which also we specify as a parameter to this accumulate function.
accumulate(start_,end_,initial_sum):累加用于求和给定范围内的所有元素。 它将元素添加到初始总和中,我们也将其指定为该累加函数的参数。
To use this accumulate function we should include numeric library. i.e #include <numeric>
要使用此累加函数,我们应包括数值库。 即#include <数字>
distance( start_, position): This function used to find the how much distance from the given iterator position to given position. Position might be anything specified by user. For example position may be minimum element, of maximum element of the vector/container.
distance(start_,position):此函数用于查找从给定的迭代器位置到给定位置的距离。 位置可以是用户指定的任何内容。 例如,位置可以是矢量/容器的最大元素中的最小元素。
Example program to show above functions:
显示上述功能的示例程序:
#include <iostream>
#include <algorithm>
#include <vector>
#include <numeric> // This is for accumulate function
using namespace std;
int main(){
vector <int> vec;
vector <int> :: iterator it;
for(int i=0; i<5; i++) vec.push_back(i+5);
cout << "Vector contains elements " ;
for(it=vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
cout << "Performing reverse(vec.begin(), vec.end()) " << endl;
reverse(vec.begin(), vec.end());
cout << "Now vector elements are " ;
for(it=vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
cout << endl << "Performing accumulate (vec.begin(), vec.end(), 0) " << endl;
int total= accumulate(vec.begin(), vec.end(), 0);
cout << "Total of vector elements using accumulate function is " << total << endl;
cout << endl << "Finding distance from starting of vector to maximum element of the vector " << endl;
cout << "For that performing distance(vec.begin(), max(vec.begin(), vec.end()) " << endl;
int dist= distance (vec.begin(), max(vec.begin(), vec.end() ));
cout << "The distance to maximum element from starting of the iterator is " << dist << endl;
return 0;
}
Output
输出量
Vector contains elements 5 6 7 8 9 Performing reverse(vec.begin(), vec.end()) Now vector elements are 9 8 7 6 5
向量包含元素5 6 7 8 9 执行反向(vec.begin(),vec.end()) 现在向量元素为9 8 7 6 5
Performing accumulate (vec.begin(), vec.end(), 0) Total of vector elements using accumulate function is 35
执行累加(vec.begin(),vec.end(),0) 使用累加功能的矢量元素总数为35
Finding distance from starting of vector to maximum element of the vector For that performing distance(vec.begin(), max(vec.begin(), vec.end()) The distance to maximum element from starting of the iterator is 5
查找从向量的起始点到向量的最大元素的 距离对于执行该距离(vec.begin(),max(vec.begin(),vec.end()) 的操作,从迭代器开始到最大元素的距离为5
next_permuatation(start_ , end_ ): As I said starting of this article, STL is a friend to competitive programmer, generating permutations is one of the main task in some questions. By using this we can generate next permutation of given sequence between the elements given specified range.
next_permuatation(start_,end_):正如我在本文开头所说,STL是有竞争力的程序员的朋友,生成置换是某些问题中的主要任务之一。 通过使用此方法,我们可以在给定指定范围的元素之间生成给定序列的下一个置换。
prev_permutation (start_, end_ ): This gives previous permutation of the sequence.
prev_permutation(start_,end_):这给出了序列的先前排列。
Example program to show above functions:
显示上述功能的示例程序:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main(){
vector <int> vec;
vector <int> :: iterator it;
for(int i=1; i<=5; i++) vec.push_back(i);
cout << "Elements in the vector are " ;
for(it = vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
cout << "next_permutation (vec.begin(), vec.end()) gives " << endl;
next_permutation(vec.begin(), vec.end());
for(it = vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
cout << "prev_permutation (vec.begin(), vec.end()) gives " << endl;
prev_permutation(vec.begin(), vec.end());
for(it = vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
return 0;
}
Output
输出量
Elements in the vector are 1 2 3 4 5 next_permutation (vec.begin(), vec.end()) gives 1 2 3 5 4 prev_permutation (vec.begin(), vec.end()) gives 1 2 3 4 5
向量中的元素为1 2 3 4 5 next_permutation(vec.begin(),vec.end())给出 1 2 3 5 4 prev_permutation(vec.begin(),vec.end())得到 1 2 3 4 5
lower_bound(start_, end_ , ele ): Returns an iterator pointing to the first element in the range which has element not less than ele.
lower_bound(start_,end_,ele):返回一个迭代器,该迭代器指向范围中第一个元素的元素不少于ele的元素。
upper_bound(start_, end_ ,ele ): Returns an iterator pointing to the first element in the given range which has element greater than ele.
upper_bound(start_,end_,ele):返回一个迭代器,该迭代器指向给定范围内第一个元素大于ele的元素。
These two functions used in performing binary search operation:
用于执行二进制搜索操作的以下两个功能:
Example program to show above functions:
显示上述功能的示例程序:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main(){
int ar[5]= {10, 20, 30, 40, 50};
vector <int> vec (ar, ar+5) ;
vector <int> :: iterator it;
sort(vec.begin(), vec.end()); // Sorting must need for lower_bound, upper_bound and binary search
cout << "Elements in the vector are " ;
for(it = vec.begin(); it!= vec.end(); it++) cout << *it << " ";
cout << endl;
it = lower_bound(vec.begin(), vec.end(), 25);
cout << "Lower bound for 25 is at position " << it- vec.begin() << endl;
it = upper_bound(vec.begin(), vec.end(), 35);
cout << "upper bound for 35 is at position " << it- vec.begin() << endl;
return 0;
}
Output
输出量
Elements in the vector are 10 20 30 40 50 Lower bound for 25 is at position 2 upper bound for 35 is at position 3
向量中的元素是10 20 30 40 50 25的下限在位置2 上35的上限在位置3上
Comment below if you have any queries related to above C++ stl algorithm library.
如果您有任何与以上C ++ stl算法库相关的查询,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/12/stl-algorithm.html
c++ stl 算法库