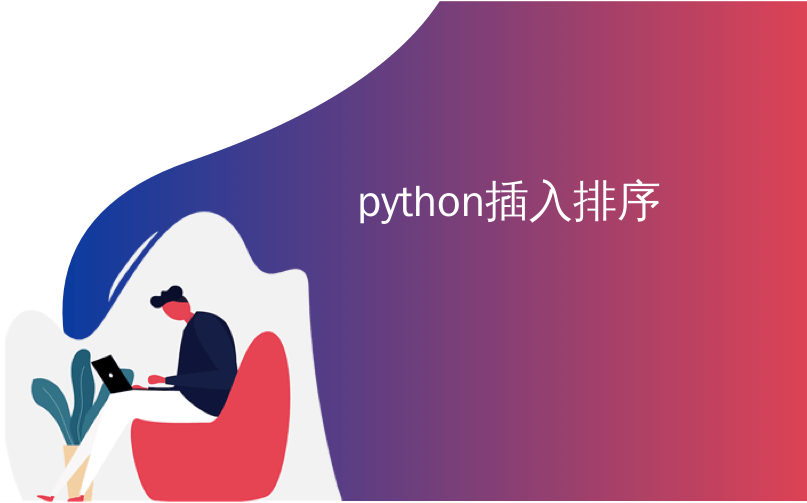
python插入排序
Here you’ll learn about Python insertion sort algorithm.
在这里,您将了解Python插入排序算法。
In terms of performance Insertion sort is not the best sorting algorithm. But it is little bit more efficient then the Selection sort and Bubble sort.
在性能方面,插入排序并不是最佳的排序算法。 但这比“选择”排序和“气泡”排序更有效率。
To understand the Insertion Sort algorithm easily, we’ll start with an example.
为了轻松理解插入排序算法,我们将从一个示例开始。
Also Read: Python Selection Sort
另请参阅: Python选择排序
Python插入排序 (Python Insertion Sort)
例 (Example)
Let’s say we have an array [14,5,18,6,21].
假设我们有一个数组[14,5,18,6,21]。
Assume that we have two parts in this array first one is sorted and another one is unsorted.
假设我们在此数组中有两个部分,第一个部分已排序,而另一部分未排序。
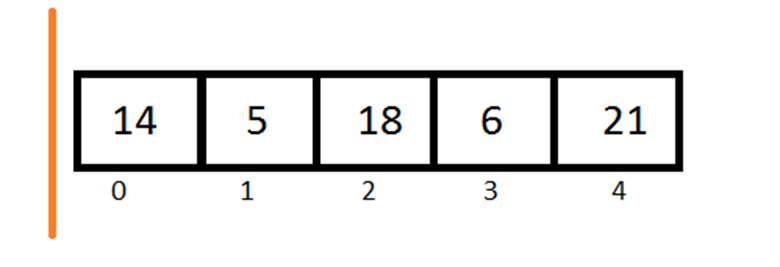
The sorted part of array is in the left of orange line and unsorted part is in the right side.
数组的排序部分在橙色线的左侧,未排序部分在右侧。
Now all we have to do is pick each element one by one from the unsorted part and add it into the sorted array at its appropriate place.
现在,我们要做的就是从未排序的部分中逐一选择每个元素,并将其添加到已排序数组中的适当位置。
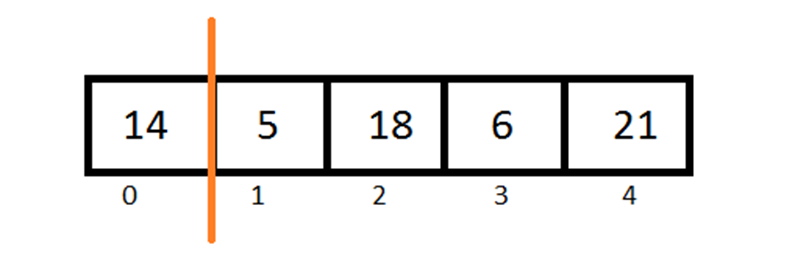
14 is the only element in our sorted part so it is already sorted.
14是我们排序部分中的唯一元素,因此它已经被排序。
Now pick the first element from the unsorted part and add it to the sorted part.
现在,从未排序部分中选择第一个元素,并将其添加到已排序部分中。
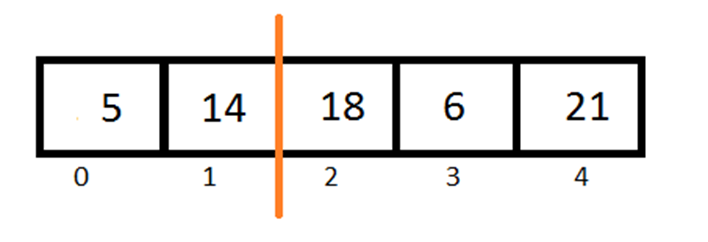
As 5 is less than 14 so 14 have to right shift by one place.
由于5小于14,因此14必须右移一位。
Again pick first element from unsorted part and add it into in sorted part.
再次从未排序的部分中选择第一个元素,并将其添加到已排序的部分中。
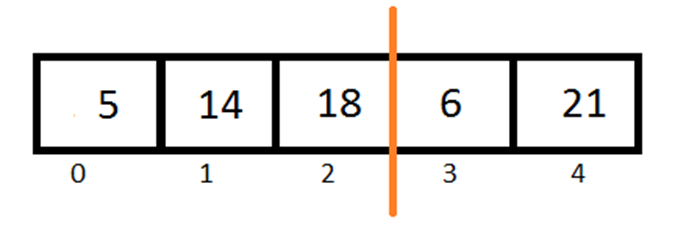
18 is greater than 14 and there is no need to shifting.
18大于14,因此无需移动。
Repeating same
重复相同
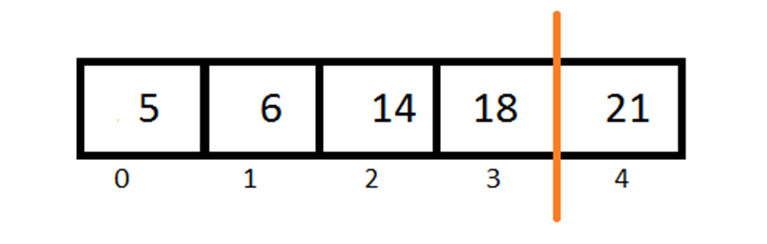
6 is greater than 5 and less than 14.
6大于5且小于14。
So we have to right shift 14 and 18 by one place.
因此,我们必须将14和18右移一位。
At last
最后
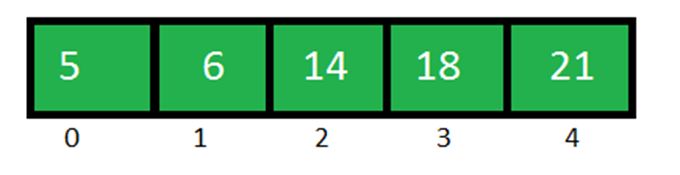
21 is greater than 18 doesn’t need to shift. Done.
21大于18不需要移动。 做完了
算法 (Algorithm)
We have an array ‘Arr[]’ with n elements
我们有一个包含n个元素的数组'Arr []'
Step 1: set i = 1
步骤1:设定i = 1
Step 2: set value = Arr[i]
步骤2:设定值= Arr [i]
Set position = i
设定位置= i
Step3 : if position > 0 and Arr[position-1]>value
步骤3:如果position> 0且Arr [position-1]> value
Arr[position] = Arr[position-1]
Arr [position] = Arr [position-1]
Position = position -1
位置=位置-1
[Repeat this step until condition become false]
[重复此步骤,直到条件变为假]
Step 4: Arr[position] = value
步骤4: Arr [position] =值
Step 5: goto step 2 for (n-1) times.
步骤5:转到步骤2(n-1)次。
Python插入排序程序 (Program for Insertion Sort in Python)
Arr = [5,14,18,6,21]
n= len(Arr)
i = 1
while (i<=n-1):
value = Arr[i]
position = i
while (position>0 and Arr[position-1]>value):
Arr[position] = Arr[position-1]
position = position -1
i = i+1
Arr[position] = value
for i in Arr:
print i,
Output
输出量
5 6 14 18 21
5 6 14 18 21
Comment below if you have any queries related to above python insertion sort algorithm.
如果您对以上python插入排序算法有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/11/python-insertion-sort.html
python插入排序