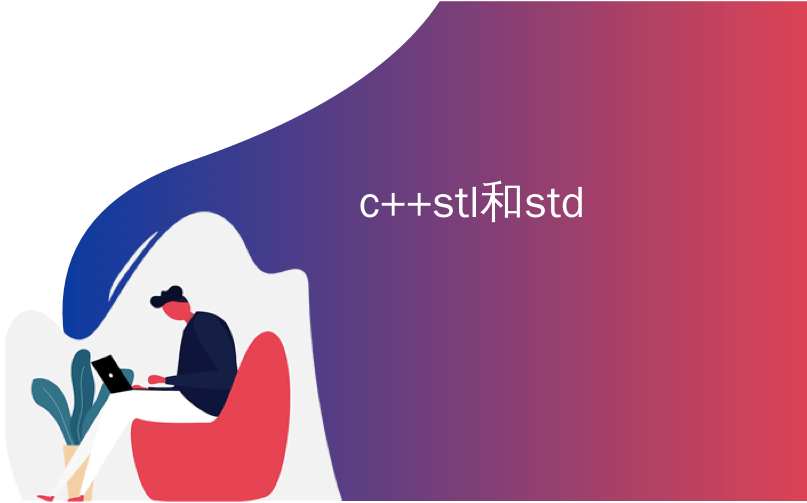
c++stl和std
In this tutorial you will learn about STL Multiset container in C++ i.e. std::multiset and all functions applicable on it.
在本教程中,您将学习C ++中的STL Multiset容器,即std :: multiset及其上适用的所有功能。
Multiset is an associative container. Same like set this also follows some specific order to store elements. But only difference is these multisets allow duplicate values. And some more similarity to sets to multisets are both has the property that, value stored and corresponding key both are same. Elements in multiset are constant. We unable to modify after insertion of the element. If we want to update element then we should delete that element and again insert with updated element. The elements in the multiset are always sorted.
Multiset是一个关联容器。 同样的设置也遵循一些特定的顺序来存储元素。 但是唯一的区别是这些多集允许重复值。 与多集的集合更相似的是它们都具有属性,即存储的值和对应的键都相同。 多重集中的元素是恒定的。 插入元素后,我们无法进行修改。 如果要更新元素,则应删除该元素,然后再次插入更新的元素。 多重集中的元素始终会排序。
C ++ STL多集容器– std :: multiset (C++ STL Multiset Container – std::multiset)
Iterators work on multiset:
迭代器适用于多集:
begin(): returns iterator to the beginning.
begin():将迭代器返回到开头。
end(): returns iterator to the end of the list.
end():将迭代器返回到列表的末尾。
rbegin(): returns reverse iterator to reverse beginning.
rbegin():将反向迭代器返回为反向开始。
rend(): returns reverse iterator to reverse end.
rend():将反向迭代器返回到反向端点。
cbegin(): Returns constant iterator to beginning.
cbegin():将常量迭代器返回到开头。
cend(): Returns constant iterator to end.
cend():返回常量迭代器以结束。
To work with multiset we need to include set library.
要使用多集,我们需要包括集库。
#include<set>
#include <set>
Let’s see some operations on multiset:
让我们看看多集上的一些操作:
1) insert(element): This operation inserts new element in the multiset. Here whatever the order we insert, those elements always inserted in a sorted order to multiset.
1)insert(element):此操作将新元素插入多集。 在这里,无论我们插入的顺序如何,这些元素总是按排序顺序插入以进行多集设置。
2) size(): This returns the number which represents current number of elements in the multiset.
2)size():此方法返回一个数字,该数字表示多重集中的当前元素数。
3) max_size(): This function returns how many number of elements in maximum we can insert into multiset.
3)max_size():此函数返回可插入多集的最大元素数。
4) empty(): This is a Boolean operation. Returns 1 is multiset is empty. Otherwise returns 0.
4)empty():这是一个布尔运算。 返回1是multiset为空。 否则返回0。
5) multiset <dataType> newMultiset (oldMultiset.beginIterator(), oldMultiset.EndIterator()): This function copies all elements of existing multiset from given iterator position to given iterator end position, to a new multiset
5)multiset <dataType> newMultiset(oldMultiset.beginIterator(),oldMultiset.EndIterator()):此函数将现有多集的所有元素从给定的迭代器位置复制到给定的迭代器结束位置,复制到新的多集
For more clearly understanding of above functions/operations, see the below program.
为了更清楚地了解上述功能/操作,请参见以下程序。
#include <iostream>
#include <set>
#include <iterator>
using namespace std;
int main()
{
multiset <int> mset; // declaring a multiset
multiset <int> ::iterator it;
for (int i=0; i<5; i++) {
mset.insert (i+1);
}
mset.insert (5); // here 5 is inserting again in the multiset. This is allowed here unlike set
// showing elements in multiset
cout << "The elements in the multiset are" << endl;
for(it= mset.begin(); it!= mset.end(); it++) {
cout << *it << " ";
}
cout << endl;
// size of multiset
cout << "Size of the multiset is " << mset.size() << endl;
// maximum size
cout << "Maximum size of the multiset is " << mset.max_size() << endl;
bool chk = mset.empty();
if (chk==1) {
cout << "Multiset is empty" << endl;
}
else{
cout << "Multiset is not empty" << endl;
}
// assigning elements of the mset to other multiset
multiset <int> mset2(mset.begin(), mset.end());
// showing elements of the copied multiset
cout << "Elements of new multiset after copying from mset" << endl;
for (it = mset2.begin(); it != mset2.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
Output
输出量
The elements in the multiset are 1 2 3 4 5 5 Size of the multiset is 6 Maximum size of the multiset is 461168601842738790 Multiset is not empty Elements of new multiset after copying from mset 1 2 3 4 5 5
多重集中的元素为 1 2 3 4 5 5 多重集的 大小 为6多重集的 最大大小为461168601842842738790 多重集不为空 从mset复制后的新多重集的元素 1 2 3 4 5 5
Some other modifying operations on multiset are:
对多集进行的其他一些修改操作是:
1) erase(element): It removes the specified element from multiset .We can also delete a range of elements by using iterators.
1)擦除(元素):它从多集中删除指定的元素。我们也可以使用迭代器删除一系列元素。
2) swap(): If there are two multisets m1 and m2. Both need not be equal size. Swap operation swaps all elements of m2 to m1. And also from m1 to m2.
2) swap():如果有两个多重集m1和m2。 两者不必大小相等。 交换操作将m2的所有元素交换为m1。 而且从m1到m2。
3) clear(): This removes all elements from multiset. So results multiset size 0.
3) clear():这将从多集合中删除所有元素。 因此结果多集大小为0。
Example program to understand above functions:
理解以上功能的示例程序:
#include <iostream>
#include <set>
#include <iterator>
using namespace std;
int main()
{
multiset <int> mset1;
multiset <int> mset2;
multiset <int> ::iterator it;
for (int i=0; i<5; i++) {
mset1.insert (i+1);
}
// erase operation
mset1.erase(5);
cout << "Elements after removing 5" << endl;
for (it=mset1.begin(); it!=mset1.end(); it++) {
cout << *it << " ";
}
cout << endl;
// inserting elements to mset2
for (int i=0;i<4;i++) {
mset2.insert(i+100);
}
cout << "elements of multiset2 are" << endl;
for (it=mset2.begin(); it!= mset2.end(); it++) {
cout << *it << " ";
}
cout << endl;
cout << "performing swap operation on entire sets..." << endl;
mset1.swap(mset2);
cout << "Elements of multiset1 after swap operation" << endl;
for (it=mset1.begin(); it!=mset1.end(); it++) {
cout << *it << " ";
}
cout << endl;
cout << "Elements of multiset2 after swap operation" << endl;
for (it=mset2.begin(); it!=mset2.end(); it++) {
cout << *it << " ";
}
cout << endl;
cout << "Applying clear operation on multiset2..." << endl;
mset2.clear();
bool chk= mset2.empty();
if (chk==1) {
cout << "Multiset2 is empty" << endl;
}
else {
cout << "Multiset2 is not empty" << endl;
}
return 0;
}
Output
输出量
Elements after removing 5 1 2 3 4 elements of multiset2 are 100 101 102 103 performing swap operation on entire sets… Elements of multiset1 after swap operation 100 101 102 103 Elements of multiset2 after swap operation 1 2 3 4 Applying clear operation on multiset2… Multiset2 is empty
除去 multiset2的 5 1 2 3 4 元素后的元素是 100 101 102 103 对整个集合执行交换操作… 交换操作后的multiset1元素 100 101 102 103 交换操作后的multiset2元素 1 2 3 4 对multiset2施加清除操作… Multiset2是空的
Some more operations are:
其他一些操作是:
1) find (element): This operation used to find the particular element form multiset. After finding using iterator we can delete that element or we can print.
1)find(element):此操作用于查找特定元素形式的多集。 找到使用迭代器后,我们可以删除该元素或进行打印。
2) count (element): This operation used to count how many number of times element occurs in the multiset.
2)计数(元素):此操作用于计算元素在多组中出现的次数。
3) lower_bound (element): This returns iterator at specified element position.
3)lower_bound(element):这将在指定元素位置返回迭代器。
4) upper_bound (element): This returns iterator at specified element position.
4)upper_bound(element):这将在指定元素位置返回迭代器。
Using upper bound and lower bound we can perform operations on range of elements between them.
使用上限和下限,我们可以对它们之间的元素范围执行操作。
Example program to explain about above operations:
解释上述操作的示例程序:
#include <iostream>
#include <set>
#include <iterator>
using namespace std;
int main()
{
multiset <int> mset1;
multiset <int> ::iterator it;
for (int i=0; i<5; i++) {
mset1.insert (i+1);
}
it= mset1.find(3); // finding element 3
cout << "Element found is " << *it << endl;
// for count operation I am adding some more elements of 2
mset1.insert (2);
mset1.insert (2);
cout << "In multiset element 2 occured " << mset1.count(2) << " times" << endl;
multiset <int> :: iterator lob, upb;
lob= mset1.lower_bound (2);
upb= mset1.upper_bound (4);
cout << "Removing elements form lower bound to upper bound..." << endl;
mset1.erase (lob,upb);
cout << "After removing elements are" << endl;
for (it=mset1.begin(); it!=mset1.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
Output
输出量
Element found is 3 In multiset element 2 occured 3 times Removing elements form lower bound to upper bound… After removing elements are 1 5
找到的元素是3 在多元素集合中发生2次3次 删除元素从下限到上限… 删除元素后为 1 5
Comment below if you have any queries related to above C++ stl multiset or std::multiset tutorial.
如果您对以上C ++ stl multiset或std :: multiset教程有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/10/stl-multiset.html
c++stl和std