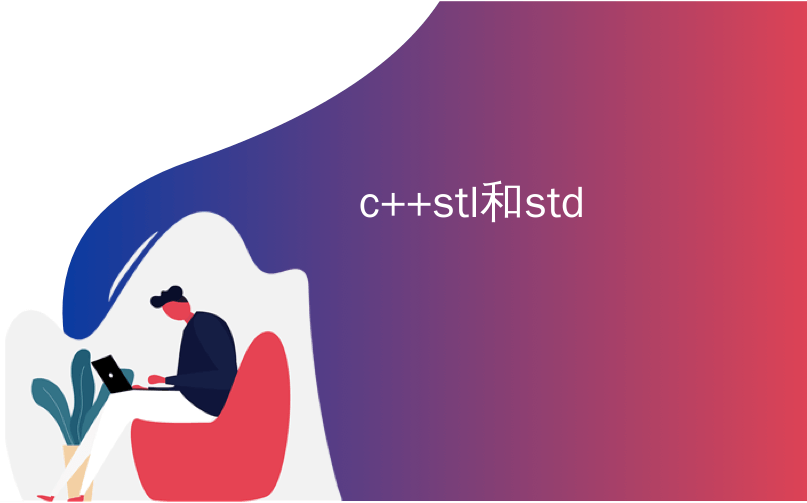
c++stl和std
In this tutorial you will learn about C++ STL list container i.e. std::list and methods which can be applicable on it.
在本教程中,您将了解C ++ STL列表容器,即std :: list及其适用的方法。
List comes under sequence containers. List stores elements in non-contiguous memory locations. List works same as double linked list. It can traverse in both directions. This is the reason list is slow in traversing when compared to vector. But it supports constant time insertion and removal of elements from anywhere in the container. If we want to implement single linked list then we should use forward list.
列表位于序列容器下。 列表将元素存储在不连续的内存位置。 列表的工作原理与双链表相同。 它可以在两个方向上移动。 这是与矢量列表相比,遍历慢的原因。 但是它支持恒定时间地从容器中的任何位置插入和删除元素。 如果要实现单个链接列表,则应使用转发列表。
The main disadvantage by this list is, unlike other sequence containers elements of this container can’t be accessed by its index position.
此列表的主要缺点是,与其他序列容器不同,该容器的元素无法通过其索引位置进行访问。
C ++ STL清单 (C++ STL List)
申报清单 (Declaring List)
list<data_type> listName;
list <数据类型> listName;
适用于清单容器的操作 (Operations Applicable on List Container)
pus_front(x): It adds the new element ‘x’ at front of the list.
pus_front(x):它将新元素“ x”添加到列表的前面。
push_back(x): It adds the new element ‘x’ at the end of the list.
push_back(x):它将新元素“ x”添加到列表的末尾。
insert(): This function inserts the new elements to the list before the element at a specific position.
insert():此函数将新元素插入列表中元素之前的特定位置。
assign(): This erases the current elements of the list and adds new elements. Due to this replacement list size will change.
Assign():这将删除列表中的当前元素并添加新元素。 由于此替换列表的大小将发生变化。
begin(): It returns the iterator pointing to the beginning of the list.
begin():它返回指向列表开头的迭代器。
end(): This returns the iterator pointing to the last element of the list.
end():这将返回指向列表最后一个元素的迭代器。
Example program to show ways to insert elements into list:
示例程序展示了将元素插入列表的方法:
#include<iostream>
#include<list>
#include<iterator>
using namespace std;
int main(){
list<int> lst1;
list <int> :: iterator it;
// inserting elements using push_front
for(int i=0; i<3; i++)
lst1.push_front(i);
// inserting elements using push_back
for(int i=1; i<=3; i++)
lst1.push_back(i+10);
// adding elements using insert() function
it = lst1.begin();
it++;
lst1.insert(it, 34); // this inserts element 34 in front of iterator points
// ohter way of adding elements using insert() method
lst1.insert(it, 2, 44); // this inserts two elements of value 44 at where iterator points
// displaying list
for(it = lst1.begin(); it != lst1.end(); ++it)
cout << *it << " ";
cout << endl;
// this is adding elements using assign method
lst1.assign(5,50);
// this adds 5 elements of each value 50 by erasing all previous elements of the list.
// check again
for(it = lst1.begin(); it != lst1.end(); ++it)
cout << *it << " ";
cout << endl;
return 0;
}
Output
输出量
2 34 44 44 1 0 11 12 13 50 50 50 50 50
2 34 44 44 1 0 11 12 13 50 50 50 50 50
Some more functions…
更多功能...
front(): It returns reference to the first element of the list.
front():返回对列表第一个元素的引用。
back(): It returns reference to the current last element of the list.
back():返回对列表当前最后一个元素的引用。
pop_front(): This erases the first element of the list.
pop_front():这将删除列表的第一个元素。
pop_back(): This erases the last element of the list.
pop_back():这将删除列表的最后一个元素。
erase(): It removes a single element or range of elements in from the list.
Erase():从列表中删除单个元素或元素范围。
remove(x): It removes the all elements of the list which has value x .
remove(x):删除列表中所有值为x的元素。
empty(): This is Boolean type method. This returns whether the list is empty or not.
empty():这是布尔型方法。 这将返回列表是否为空。
Example program to show all above functions:
显示上述所有功能的示例程序:
#include<iostream>
#include<list>
#include<iterator>
using namespace std;
int main(){
list <int> lst1;
list <int> :: iterator it;
list <int> :: iterator it1;
// inserting some elements into list.
for(int i=0; i<5; i++)
lst1.push_front(i+10);
for(it =lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
// getting front element
cout << "the first element of the list is ";
cout << lst1.front() << endl;
// getting last element
cout << "the last element of the list is ";
cout << lst1.back() << endl;
// erasing first element of the list
lst1.pop_front();
cout << "the first element after erasing current first elemnt is ";
cout << lst1.front();
cout << endl;
// erasing last element of the list
lst1.pop_back();
cout << "the last element after erasing current last element is ";
cout << lst1.back();
cout << endl;
// deleting elements using erase() function
it = lst1.begin();
lst1.erase(it); // this removes the element where itertaor points
// displaying remaining elements in the list
cout << "remaining elements after doing all above operations " << endl;
for(it =lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
// checking list is empty or not
lst1.empty() ? cout << "List is empty" << endl : cout << "List is not empty" << endl;
// applying remove() method
lst1.remove(11);
// displaying remaining elements in the list
cout << "remaining elements after removing 11 are" << endl;
for(it =lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
return 0;
}
Output
输出量
14 13 12 11 10 the first element of the list is 14 the last element of the list is 10 the first element after erasing current first elemnt is 13 the last element after erasing current last element is 11 remaining elements after doing all above operations 12 11 List is not empty remaining elements after removing 11 are 12
14 13 12 11 10 列表的第一个元素是14列表 的最后一个元素是10 删除当前第一个元素后的第一个元素是13 删除当前的最后一个元素后的最后一个元素是 执行上述所有操作之后的 11个 剩余元素 12 11 列表不为空 删除11后剩余元素为 12
reverse(): This reverse all the elements of the list.
reverse():这将反转列表的所有元素。
sort(): Sorts the all elements in the list in increasing order.
sort():以递增顺序对列表中的所有元素进行排序。
size(): This returns the number of elements in the list.
size():这将返回列表中的元素数。
Example program to show above functions:
显示上述功能的示例程序:
#include<iostream>
#include<list>
#include<iterator>
using namespace std;
int main(){
list <int> lst1;
list <int> :: iterator it;
for(int i=0; i<6; i++){
if(i%2) lst1.push_back(i);
else lst1.push_front(i);
}
cout << "actual elements of the list are" << endl;
for(it = lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
// using reverse function
lst1.reverse();
cout << "Elements in the list after applying reverse operation " << endl;
for(it = lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
// using sort function
lst1.sort();
cout << "Elements in the list after applying sort operation" << endl;
for(it = lst1.begin(); it!= lst1.end(); it++)
cout << *it << " ";
cout << endl;
// finding size
cout << "size of the lis is ";
cout << lst1.size();
return 0;
}
Output
输出量
actual elements of the list are 4 2 0 1 3 5 Elements in the list after applying reverse operation 5 3 1 0 2 4 Elements in the list after applying sort operation 0 1 2 3 4 5 size of the lis is 6
列表的实际元素是 4 2 0 1 3 5 应用反向操作后列表中的元素 5 3 1 0 2 4 应用排序操作后列表中的元素 0 1 2 3 4 5 lis的大小是6
Comment below if you have queries or found information incorrect in above tutorial for C++ STL List.
如果您在上面的C ++ STL列表教程中有疑问或发现不正确的信息,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/07/stl-list.html
c++stl和std