In this tutorial you will learn about null pointer in C with examples.
在本教程中,您将通过示例学习C语言中的空指针。
When we declare a pointer, by default it points to some random memory location. If you will access the pointer then it may give some undesired value or the program may crash.
当我们声明一个指针时,默认情况下它指向某个随机的内存位置。 如果您将访问该指针,则它可能会提供一些不必要的值,否则程序可能会崩溃。
什么是C中的空指针? (What is Null Pointer in C?)
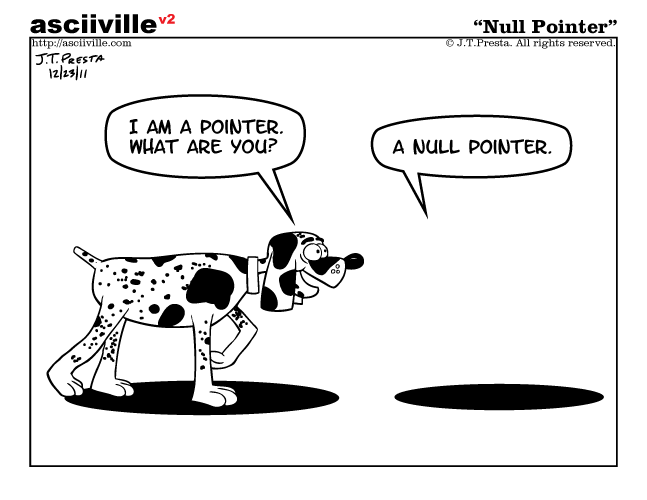
A pointer pointing to nothing or no memory location is called null pointer. For doing this we simply assign NULL to the pointer.
指向无内容或无存储位置的指针称为空指针。 为此,我们只需将NULL分配给指针。
So while declaring a pointer we can simply assign NULL to it in following way.
因此,在声明指针时,我们可以简单地通过以下方式为其分配NULL。
int *p = NULL;
NULL is a constant which is already defined in C and its value is 0. So instead of assigning NULL to pointer while declaring it we can also assign 0 to it.
NULL是已经在C中定义的常数,其值为0。因此,除了在声明指针时将NULL分配给指针,我们还可以为其分配0。
空指针的用法 (Usage of Null Pointer)
1. We can simply check the pointer is NULL or not before accessing it. This will prevent crashing of program or undesired output.
1.我们可以在访问指针之前简单地检查指针是否为NULL。 这样可以防止程序崩溃或不希望的输出。
int *p = NULL;
if(p != NULL) {
//here you can access the pointer
}
2. A pointer pointing to a memory location even after its deallocation is called dangling pointer. When we try to access dangling pointer it crashes the program. So to solve this problem we can simply assign NULL to it.
2.即使在释放后仍指向内存位置的指针也称为悬空指针 。 当我们尝试访问悬空指针时,它会使程序崩溃。 因此,要解决此问题,我们只需为其分配NULL。
void function(){
int *ptr = (int *)malloc(SIZE);
. . . . . .
. . . . . .
free(ptr); //ptr now becomes dangling pointer which is pointing to dangling reference
ptr=NULL; //now ptr is not dangling pointer
}
3. We can simply pass NULL to a function if we don’t want to pass a valid memory location.
3.如果我们不想传递有效的内存位置,则可以简单地将NULL传递给函数。
void fun(int *p) {
//some code
}
int main() {
fun(NULL);
return 0;
}
4. Null pointer is also used to represent the end of a linked list.
4.空指针还用于表示链表的结尾。
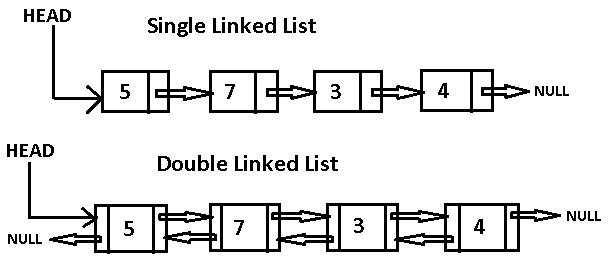
Comment below if you found anything incorrect or have queries regarding above tutorial for null pointer in C.
如果发现任何错误或对以上教程的C语言中的空指针有疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/05/null-pointer-in-c.html