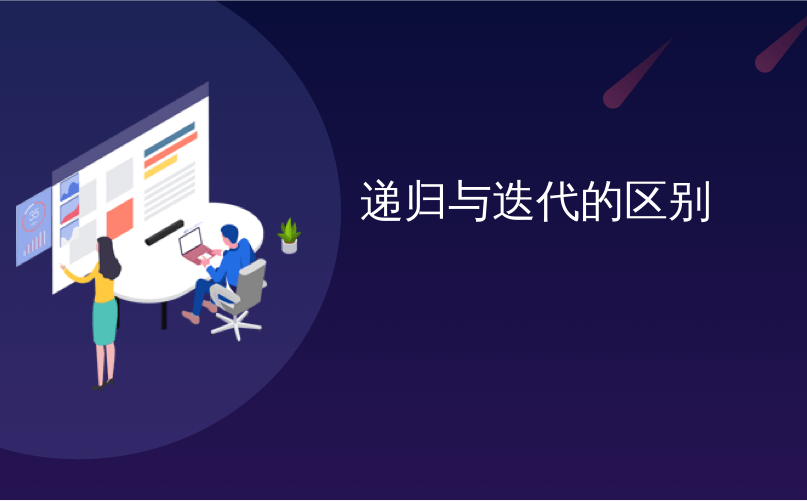
递归与迭代的区别
In this tutorial you will learn about difference between recursion and iteration with example.
在本教程中,您将通过示例了解递归和迭代之间的区别。
Both recursion and iteration are used for executing some instructions repeatedly until some condition is true. A same problem can be solved with recursion as well as iteration but still there are several differences in their working and performance that I have mentioned below.
递归和迭代都用于重复执行某些指令,直到满足某些条件为止。 递归和迭代都可以解决相同的问题,但是我下面已经提到它们在工作和性能上仍然存在一些差异。
递归与迭代之间的区别 (Difference between Recursion and Iteration)
Recursion | Iteration | |
Definition | Recursion refers to a situation where a function calls itself again and again until some base condition is not reached. | Iteration refers to a situation where some statements are executed again and again using loops until some condition is true. |
Performance | It is comparatively slower because before each function call the current state of function is stored in stack. After the return statement the previous function state is again restored from stack. | Its execution is faster because it doesn’t use stack. |
Memory | Memory usage is more as stack is used to store the current function state. | Memory usage is less as it doesn’t use stack. |
Code Size | Size of code is comparatively smaller in recursion. | Iteration makes the code size bigger. |
递归 | 迭代 | |
定义 | 递归是指函数一次又一次地调用自身直到未达到某个基本条件的情况。 | 迭代是指一种情况,其中某些语句使用循环反复执行,直到满足某些条件为止。 |
性能 | 它相对较慢,因为在每个函数调用之前,函数的当前状态都存储在堆栈中。 在return语句之后,先前的功能状态再次从堆栈中恢复。 | 它的执行速度更快,因为它不使用堆栈。 |
记忆 | 内存使用更多,因为使用堆栈来存储当前功能状态。 | 内存使用量较少,因为它不使用堆栈。 |
代码大小 | 递归的代码大小相对较小。 | 迭代使代码更大。 |
Lets write the implementation of finding factorial of number using recursion as well as iteration.
让我们编写使用递归和迭代来查找数字阶乘的实现。
递归示例 (Recursion Example)
Below is the C program to find factorial of a number using recursion.
下面是使用递归查找数字阶乘的C程序。
#include <stdio.h>
int factorial(int n){
if(n == 0)
return 1;
else
return n * factorial(n-1);
}
int main() {
printf("Factorial for 5 is %d", factorial(5));
return 0;
}
迭代示例 (Iteration Example)
Below is the C program to find factorial of a number using iteration.
下面是使用迭代查找数字阶乘的C程序。
#include <stdio.h>
int main() {
int i, n = 5, fac = 1;
for(i = 1; i <= n; ++i)
fac = fac * i;
printf("Factorial for 5 is %d", fac);
return 0;
}
Comment below if you have any queries regarding above tutorial for recursion vs iteration.
如果您对以上教程的递归与迭代有任何疑问,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2017/05/difference-between-recursion-and-iteration.html
递归与迭代的区别