In this tutorial you will learn to pass data from one activity to another in android with and without using intent.
在本教程中,您将学习使用和不使用意图将数据从一个活动传递到android中的另一个活动。
Basically we can send data between activities in two ways.
基本上,我们可以通过两种方式在活动之间发送数据。
- Using Intent 使用意图
- Using Global Variables 使用全局变量
Below I have discussed both the methods in detail.
下面,我详细讨论了这两种方法。
Note: There are some other ways like shared preferences and database (sqlite) but here I have shared only those ways that don’t involve saving of data.
注意:还有其他一些方式,例如共享首选项和数据库( sqlite ),但是在这里,我仅共享了那些不涉及数据保存的方式。
如何在Android中将数据从一个活动传递到另一个活动 (How to Pass Data from One Activity to Another in Android)
方法1:使用意图 (Method 1: Using Intent)
We can send data while calling one activity from another activity using intent. All we have to do is add the data to Intent object using putExtra() method. The data is passed in key value pair. The value can be of types like int, float, long, string, etc.
我们可以在使用意图从另一个活动中调用一个活动的同时发送数据。 我们要做的就是使用putExtra()方法将数据添加到Intent对象。 数据以键值对的形式传递。 该值可以是int,float,long,string等类型。
Sending Data
传送资料
Intent intent = new Intent(context, DestinationActivityName.class);
intent.putExtra(Key, Value);
startActivity(intent);
Here I am sending just one value, in the same way you can attach more values by using putExtra() method.
在这里,我只发送一个值,以相同的方式,您可以使用putExtra()方法附加更多值。
Retrieving Data
检索数据
If the data sent is of type string then it can be fetched in following way.
如果发送的数据为字符串类型,则可以按以下方式获取。
Intent intent = getIntent();
String str = intent.getStringExtra(Key);
There are several other methods like getIntExtra(), getFloatExtra(), etc to fetch other types of data.
还有其他几种方法,例如getIntExtra() , getFloatExtra()等,用于获取其他类型的数据。
Below is the example of passing data between activities using intents.
以下是使用意图在活动之间传递数据的示例。
例 (Example)
In this example a message is passed from first activity to second when a button is pressed.
在此示例中,当按下按钮时,消息从第一活动传递到第二活动。
activity_first.xml
activity_first.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="15dp"
tools:context="com.androidexample.First"
android:orientation="vertical">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textBox"
android:hint="Enter Your Message"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/passButton"
android:text="Pass"/>
</LinearLayout>
activity_second.xml
activity_second.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="15dp"
tools:context="com.androidexample.Second">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text=""
android:id="@+id/text"/>
</LinearLayout>
First.java
第一.java
package com.androidexample;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class First extends AppCompatActivity {
EditText textBox;
Button passButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_first);
textBox = (EditText)findViewById(R.id.textBox);
passButton = (Button)findViewById(R.id.passButton);
passButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String str = textBox.getText().toString();
Intent intent = new Intent(getApplicationContext(), Second.class);
intent.putExtra("message", str);
startActivity(intent);
}
});
}
}
Second.java
第二.java
package com.androidexample;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextView;
public class Second extends AppCompatActivity {
TextView text;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
text = (TextView)findViewById(R.id.text);
Intent intent = getIntent();
String str = intent.getStringExtra("message");
text.setText(str);
}
}
Screenshots
屏幕截图
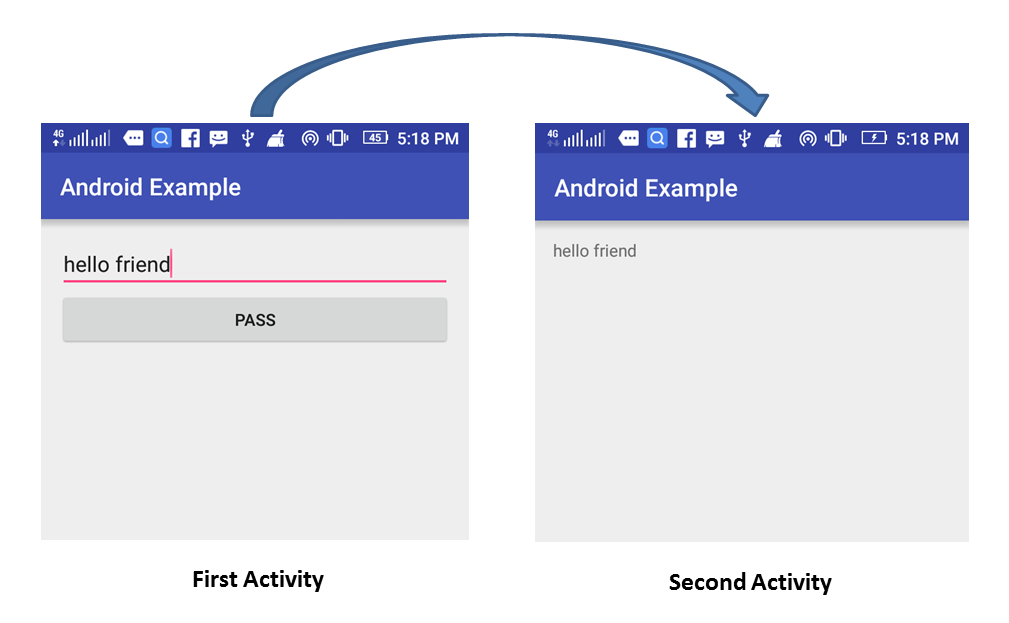
方法2:使用全局变量 (Method 2: Using Global Variables)
We can declare a global variable in a separate class. To make it global just declare it as public static. This will allow us to directly access the variable anywhere in our application using its class name.
我们可以在一个单独的类中声明一个全局变量。 要使其全局,只需将其声明为public static。 这将允许我们使用其类名直接在应用程序中的任何位置访问变量。
Just assign the value that you want to pass in global variable inside source activity and fetch it in destination activity by accessing the global variable.
只需在源活动中分配要传递的全局变量值,然后通过访问全局变量在目标活动中获取它即可。
Below example shows how to do this.
以下示例显示了如何执行此操作。
例 (Example)
The code for activity_first.xml and activity_second.xml will be same as above example.
activity_first.xml和activity_second.xml的代码与上面的示例相同。
DemoClass.java
DemoClass.java
package com.androidexample;
public class DemoClass {
public static String message; //global variable
}
First.java
第一.java
package com.androidexample;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class First extends AppCompatActivity {
EditText textBox;
Button passButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_first);
textBox = (EditText)findViewById(R.id.textBox);
passButton = (Button)findViewById(R.id.passButton);
passButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
DemoClass.message = textBox.getText().toString();
Intent intent = new Intent(getApplicationContext(), Second.class);
startActivity(intent);
}
});
}
}
Second.java
第二.java
package com.androidexample;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextView;
public class Second extends AppCompatActivity {
TextView text;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
text = (TextView)findViewById(R.id.text);
text.setText(DemoClass.message);
}
}
Comment below if you have doubts or know any other way to pass data from one activity to another in android.
如果您有疑问或其他任何方法将数据从一个活动传递到android中的另一个活动,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2016/12/pass-data-one-activity-another-in-android.html