In this tutorial you will learn to make an android soap client using ksoap2 library.
在本教程中,您将学习使用ksoap2库制作一个Android soap客户端。
Prerequisites
先决条件
- We need ksop2 library. You can download its jar easily by searching on google. 我们需要ksop2库。 您可以通过在Google上搜索轻松下载其jar。
- A soap web service running on server. 在服务器上运行的Soap Web服务。
Also Read: Android Restful Web Service Client Example
另请参阅: Android Restful Web服务客户端示例
In this example we will create android client that will consume soap web service developed in Java. If you don’t have soap web service and want to know how to develop then follow below link to read the tutorial.
在此示例中,我们将创建将使用Java开发的soap Web服务的android客户端。 如果您没有肥皂网络服务,并且想知道如何开发,请点击以下链接阅读本教程。
Also Read: Java SOAP Web Services Tutorial
另请阅读: Java SOAP Web服务教程
这个怎么运作? (How it works?)
URL: It is the url of WSDL file.
URL:这是WSDL文件的URL。
NAMESPACE: The targetNamespace in WSDL.
命名空间: WSDL中的targetNamespace。
METHOD_NAME: It is the method name in web service. We can have several methods.
METHOD_NAME:这是Web服务中的方法名称。 我们可以有几种方法。
SOAP_ACTION: NAMESPACE + METHOD_NAME.
SOAP_ACTION: NAMESPACE + METHOD_NAME。
You can obtain above details by reading WSDL file of web service.
您可以通过阅读Web服务的WSDL文件来获得上述详细信息。
The soap web service that I have used in this example have only one method that takes a number as parameter and find its square.
我在此示例中使用的soap Web服务只有一种以数字作为参数并找到其平方的方法。
How to call soap web service?
如何调用肥皂网络服务?
First create request object of SoapObject class.
首先创建SoapObject类的请求对象。
Now add information to request object that you want to send to web service. This is done using PropertyInfo class.
现在添加信息以请求要发送到Web服务的对象。 这是使用PropertyInfo类完成的。
Create soap envelope using SoapSerializationEnvelope class.
使用SoapSerializationEnvelope类创建肥皂信封。
Assign request object to envelope using setOutputSoapObject().
使用setOutputSoapObject()将请求对象分配给信封。
Create object of HttpTransportSE() class. Using this object call the soap web service.
创建HttpTransportSE()类的对象。 使用该对象调用soap Web服务。
We can get response from web service by using getResponse() method of SoapSerializationEnvelope class. The response is stored into SoapPrimitive reference variable.
我们可以使用SoapSerializationEnvelope类的getResponse()方法从Web服务获取响应。 响应存储在SoapPrimitive参考变量中。
This is how we use ksop2 library to call soap web service in android.
这就是我们使用ksop2库在android中调用soap Web服务的方式。
Android SOAP客户端示例 (Android SOAP Client Example)
Create an android studio project with package name com.androidsoapclient.
使用包名称com.androidsoapclient创建一个android studio项目。
Import the ksoap2 library in your project. If you don’t know how to import a library then follow below link.
将ksoap2库导入您的项目中。 如果您不知道如何导入库,请点击以下链接。
Read: How to Add Jar as Library in Android Studio
阅读: 如何在Android Studio中将Jar添加为库
Add internet access permission in AndroidManifest.xml file by adding following line.
通过添加以下行,在AndroidManifest.xml文件中添加互联网访问权限。
<uses-permission android:name="android.permission.INTERNET"/>
Add following code in respective files.
在相应的文件中添加以下代码。
activity_main.xml
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
tools:context="com.androidsoapclient.MainActivity">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textBox"
android:layout_marginBottom="5dp"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Find Square"
android:id="@+id/button"
android:layout_below="@+id/textBox"
android:layout_marginBottom="5dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/text"
android:layout_below="@id/button"/>
</RelativeLayout>
MainActivity.java
MainActivity.java
package com.androidsoapclient;
import android.app.ProgressDialog;
import android.os.AsyncTask;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.PropertyInfo;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapPrimitive;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
public class MainActivity extends AppCompatActivity {
EditText textBox;
Button button;
TextView text;
String URL = "http://192.168.1.6:14870/SOAPWebService/services/DemoService?WSDL";
String NAMESPACE = "http://com";
String SOAP_ACTION = "http://com/square";
String METHOD_NAME = "square";
String PARAMETER_NAME = "n";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textBox = (EditText)findViewById(R.id.textBox);
button = (Button)findViewById(R.id.button);
text = (TextView)findViewById(R.id.text);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
new CallWebService().execute(textBox.getText().toString());
}
});
}
class CallWebService extends AsyncTask<String, Void, String> {
@Override
protected void onPostExecute(String s) {
text.setText("Square = " + s);
}
@Override
protected String doInBackground(String... params) {
String result = "";
SoapObject soapObject = new SoapObject(NAMESPACE, METHOD_NAME);
PropertyInfo propertyInfo = new PropertyInfo();
propertyInfo.setName(PARAMETER_NAME);
propertyInfo.setValue(params[0]);
propertyInfo.setType(String.class);
soapObject.addProperty(propertyInfo);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(SoapEnvelope.VER11);
envelope.setOutputSoapObject(soapObject);
HttpTransportSE httpTransportSE = new HttpTransportSE(URL);
try {
httpTransportSE.call(SOAP_ACTION, envelope);
SoapPrimitive soapPrimitive = (SoapPrimitive)envelope.getResponse();
result = soapPrimitive.toString();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
}
AsyncTask class is used to perform some operation in background. As we are doing network related operation so it is good to perform it in background.
AsyncTask类用于在后台执行某些操作。 由于我们正在进行与网络相关的操作,因此最好在后台执行该操作。
屏幕截图 (Screenshot)
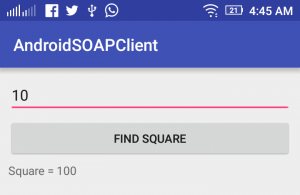
Comment below if you have any queries or found anything incorrect in above android soap client example.
如果您有任何疑问或在上面的android soap客户端示例中发现任何错误,请在下面评论。
翻译自: https://www.thecrazyprogrammer.com/2016/11/android-soap-client-example-using-ksoap2.html