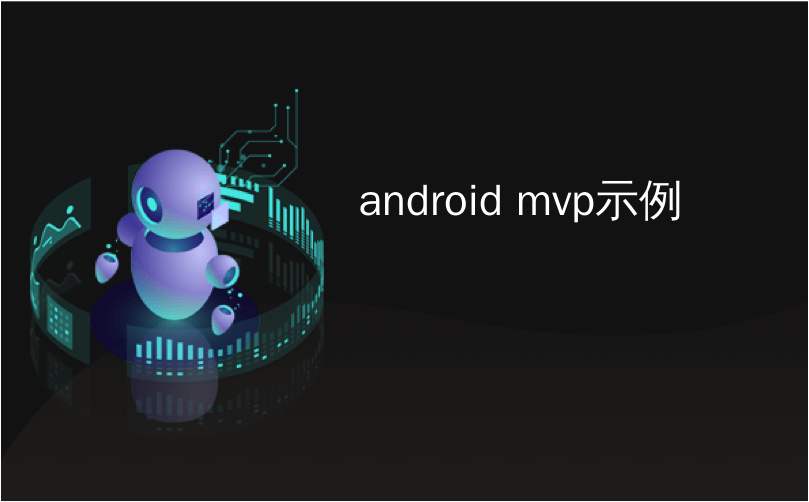
android mvp示例
Here you will learn about android pull or swipe down to refresh using SwipeRefreshLayout example.
在这里,您将使用SwipeRefreshLayout示例了解有关Android拉动或向下滑动以刷新的信息。
You may have seen the pull to refresh feature in apps like Facebook, Twitter, Gmail, etc. We can implement that in android using SwipeRefreshLayout widget.
您可能已经在Facebook,Twitter,Gmail等应用中看到了“刷新刷新”功能。我们可以使用SwipeRefreshLayout小部件在android中实现该功能 。
How to Implement?
如何实施?
Add SwipeRefreshLayout widget in your layout xml file with only one child. The child can be listview, gridview, recyclerview, etc.
仅在一个孩子的情况下,将SwipeRefreshLayout小部件添加到布局xml文件中。 子级可以是listview, gridview ,recyclerview等。
Implement SwipeRefreshLayout.OnRefreshListener and override onRefresh() method. Whenever a pull or swipe down gesture is done the onRefresh() method is called. You have to do any update operation in this method. After completing update operation just call setRefreshing(false) method to remove the progress indicator.
实现SwipeRefreshLayout.OnRefreshListener并重写onRefresh()方法。 每当完成拉动或向下滑动手势时, 都会调用onRefresh()方法。 您必须使用此方法执行任何更新操作。 完成更新操作后,只需调用setRefreshing(false)方法即可删除进度指示器。
Below I have shared one example in which I am updating the data of listview on swipe down gesture.
下面我分享了一个示例,其中我通过向下滑动手势来更新listview的数据。
Android使用SwipeRefreshLayout示例向下拉或向下滑动以刷新 (Android Pull or Swipe Down to Refresh Using SwipeRefreshLayout Example)
Create an android studio project with package name com.swipetorefresh
使用包名称com.swipetorefresh创建一个Android Studio项目
Note: Make sure your project have dependency for android support library in build.gradle file.
注意:请确保您的项目对build.gradle文件中的android支持库具有依赖性。
Now add following code in respective files.
现在,在相应文件中添加以下代码。
activity_main.xml
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.SwipeRefreshLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.swipetorefresh.MainActivity"
android:id="@+id/str">
<ListView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/list"/>
</android.support.v4.widget.SwipeRefreshLayout>
MainActivity.java
MainActivity.java
package com.swipetorefresh;
import android.support.v4.widget.SwipeRefreshLayout;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import java.util.ArrayList;
import java.util.Random;
public class MainActivity extends AppCompatActivity {
SwipeRefreshLayout str;
ListView list;
ArrayAdapter adapter;
ArrayList al;
Random random;
String fruits[] = {"Apple",
"Apricot",
"Avocado",
"Banana",
"Bilberry",
"Blackberry",
"Blackcurrant",
"Blueberry",
"Boysenberry",
"Currant"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
str = (SwipeRefreshLayout)findViewById(R.id.str);
list = (ListView)findViewById(R.id.list);
al = new ArrayList();
random = new Random();
//initially setting 5 items in listview
for(int i = 0; i < 5; ++i){
al.add(fruits[i]);
}
adapter = new ArrayAdapter(this, android.R.layout.simple_list_item_1, al);
list.setAdapter(adapter);
str.setOnRefreshListener(new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
//calling updateData() method to do update operation
updateData();
}
});
}
void updateData(){
int index;
al.clear();
//picking 5 random fruits name from the list of fruits
for(int i= 0; i < 5; ++i){
index = random.nextInt(10);
al.add(fruits[index]);
}
//notifying the adapter to update the listview data
adapter.notifyDataSetChanged();
//removing the progress indicator
str.setRefreshing(false);
}
}
Save and run your project.
保存并运行您的项目。
屏幕截图 (Screenshot)
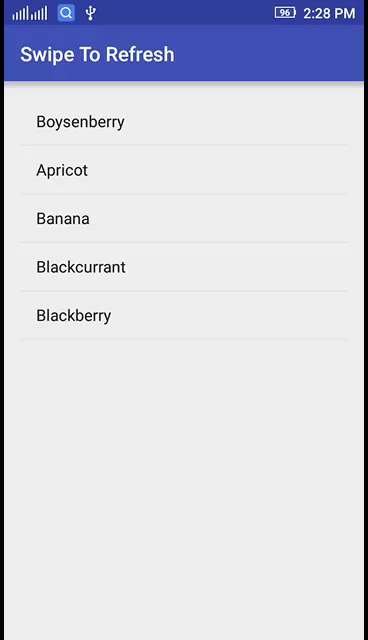
Comment below if you have any difficulty or found anything incorrect in above android pull to refresh tutorial.
如果您在上面的android pull刷新教程中有任何困难或发现任何错误,请在下面评论。
android mvp示例