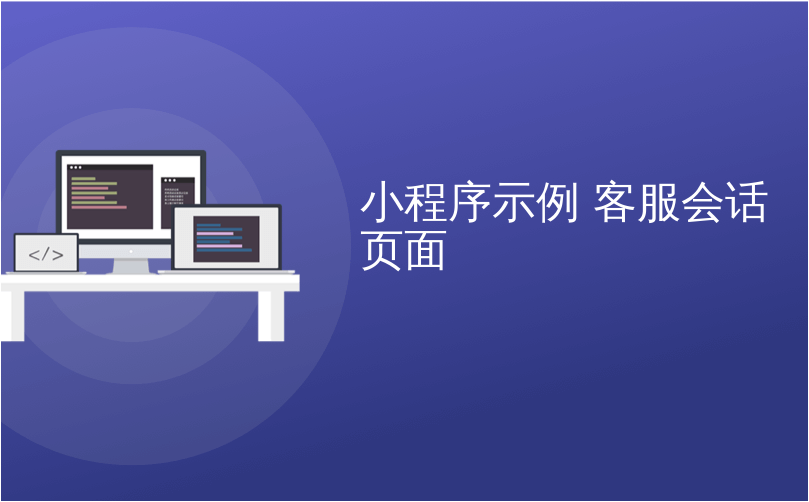
小程序示例 客服会话页面
In this tutorial you will learn how to make JSP login and logout system using session. I have used MySQL as a database in this example.
在本教程中,您将学习如何使用session进行JSP登录和注销系统。 在此示例中,我已将MySQL用作数据库。
This system has following files.
该系统具有以下文件。
index.jsp: It contains a login form which is displayed to user.
index.jsp:它包含一个显示给用户的登录表单。
loginRequestHandler.jsp: When login form is submitted, this page handles the login request.
loginRequestHandler.jsp:提交登录表单后,此页面将处理登录请求。
home.jsp: If the login details are correct then the user will be redirect to home page. It contain welcome message with a logout link.
home.jsp:如果登录详细信息正确,则用户将被重定向到主页。 它包含带有注销链接的欢迎消息。
logout.jsp: It invalidates the session and logout the user from system.
logout.jsp:它将使会话无效并从系统中注销用户。
DBConnection.java: It handles connectivity with the database.
DBConnection.java:它处理与数据库的连接。
LoginBean.java: It is a bean class that has getter and setter methods.
LoginBean.java:这是一个具有getter和setter方法的bean类。
LoginDAO.java: This class verifies the email and password from the database.
LoginDAO.java:此类验证来自数据库的电子邮件和密码。
The database table that I have used has following structure.
我使用的数据库表具有以下结构。
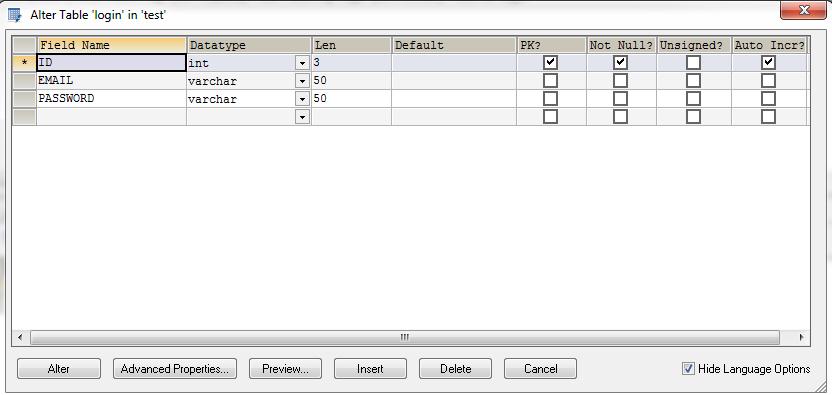
I have done proper session tracking in this example. If the user is not logged in and tries to open home.jsp page then he/she will be redirected to index.jsp page for login. If he/she is already logged in and tries to open index.jsp then he/she will be directly redirected to home.jsp.
在此示例中,我已经进行了正确的会话跟踪。 如果用户未登录并尝试打开home.jsp页面,则他/她将被重定向到index.jsp页面进行登录。 如果他/她已经登录并尝试打开index.jsp,那么他/她将被直接重定向到home.jsp 。
Below I have shared the code for each of these files.
下面,我共享了每个文件的代码。
index.jsp (index.jsp)
<html>
<head>
<title>Login System</title>
</head>
<body>
<%
String email=(String)session.getAttribute("email");
//redirect user to home page if already logged in
if(email!=null){
response.sendRedirect("home.jsp");
}
String status=request.getParameter("status");
if(status!=null){
if(status.equals("false")){
out.print("Incorrect login details!");
}
else{
out.print("Some error occurred!");
}
}
%>
<form action="loginRequestHandler.jsp">
<table cellpadding="5">
<tr>
<td><b>Email:</b></td>
<td><input type="text" name="email" required/></td>
</tr>
<tr>
<td><b>Password:</b></td>
<td><input type="password" name="password" required/></td>
</tr>
<tr>
<td colspan="2" align="center"><input type="submit" value="Login"/></td>
</tr>
</table>
</form>
</body>
</html>
loginRequestHandler.jsp (loginRequestHandler.jsp)
<%@page import="com.LoginDAO"%>
<jsp:useBean id="loginBean" class="com.LoginBean" scope="session"/>
<jsp:setProperty name="loginBean" property="*"/>
<%
String result=LoginDAO.loginCheck(loginBean);
if(result.equals("true")){
session.setAttribute("email",loginBean.getEmail());
response.sendRedirect("home.jsp");
}
if(result.equals("false")){
response.sendRedirect("index.jsp?status=false");
}
if(result.equals("error")){
response.sendRedirect("index.jsp?status=error");
}
%>
home.jsp (home.jsp)
<html>
<head>
<title>Login System</title>
</head>
<body>
<%
String email=(String)session.getAttribute("email");
//redirect user to login page if not logged in
if(email==null){
response.sendRedirect("index.jsp");
}
%>
<p>Welcome <%=email%></p>
<a href="logout.jsp">Logout</a>
</body>
</html>
logout.jsp (logout.jsp)
<%
session.invalidate();
response.sendRedirect("index.jsp");
%>
DBConnection.java (DBConnection.java)
package com;
import java.sql.Connection;
import java.sql.DriverManager;
public class DBConnection {
static final String URL="jdbc:mysql://localhost:3306/";
static final String DATABASE_NAME="test";
static final String USERNAME="root";
static final String PASSWORD="root";
public static Connection getConnection(){
Connection con=null;
try{
Class.forName("com.mysql.jdbc.Driver");
con=DriverManager.getConnection(URL+DATABASE_NAME,USERNAME,PASSWORD);
}catch(Exception e){
e.printStackTrace();
}
return con;
}
}
LoginBean.java (LoginBean.java)
package com;
public class LoginBean {
private String email;
private String password;
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
LoginDAO.java (LoginDAO.java)
package com;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class LoginDAO {
public static String loginCheck(LoginBean loginBean){
String query="select * from login where email=? and password=?";
try{
Connection con=DBConnection.getConnection();
PreparedStatement ps=con.prepareStatement(query);
ps.setString(1,loginBean.getEmail());
ps.setString(2,loginBean.getPassword());
ResultSet rs=ps.executeQuery();
if(rs.next()){
return "true";
}
else{
return "false";
}
}catch(Exception e){
e.printStackTrace();
}
return "error";
}
}
Screenshots
屏幕截图
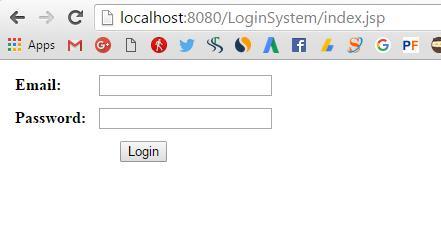
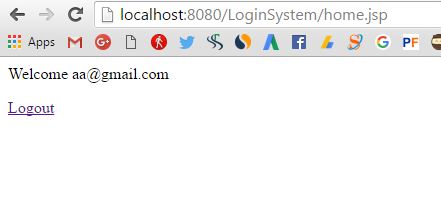
Comment below if you are facing difficulty to understand anything in above JSP login and logout system.
如果您在上面的JSP登录和注销系统中难以理解任何内容,请在下面评论。
Happy Coding!! 🙂 🙂
快乐编码! 🙂
翻译自: https://www.thecrazyprogrammer.com/2016/03/jsp-login-logout-system-example-using-session.html
小程序示例 客服会话页面