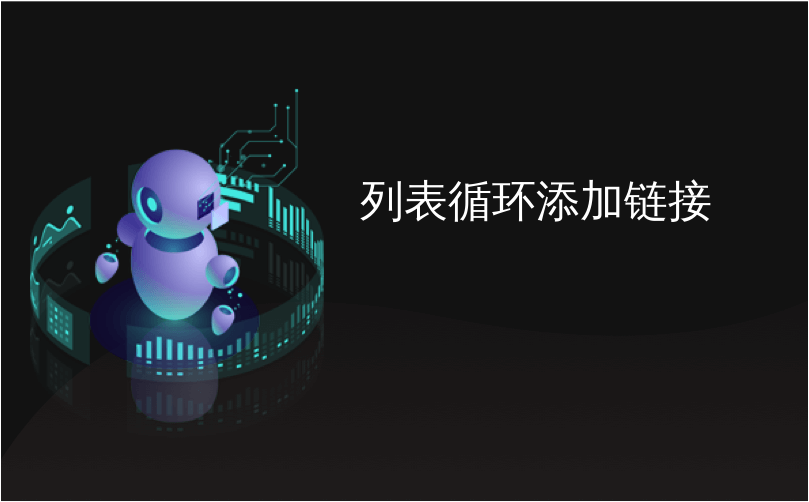
列表循环添加链接
Here you will get program for circular linked list in C.
在这里,您将获得C中循环链表的程序。
What is Circular Linked List?
什么是循环链表?
A circular linked list is a linked list in which the last node points to the head or front node making the data structure to look like a circle. A circularly linked list node can be implemented using singly linked or doubly linked list.
循环链表是链表,其中最后一个节点指向头或头节点,从而使数据结构看起来像一个圆。 循环链表节点可以使用单链表或双链表实现 。
The below representation shows how a circular linked list looks like. Unlike the linear linked list, the last node is pointed to the head using a rear pointer.
下图显示了循环链表的外观。 与线性链表不同,最后一个节点使用后指针指向头。
C中的循环链表程序 (
Program for Circular Linked List in C)
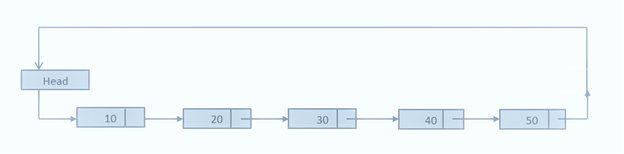
We basically have 3 nodes head, rear and temp to implement a circular linked list. The rear points to the last node of the list, head points to first node. This helps to maintain a track on where is the front and rear nodes of the circle. Let us see the basic operations such as creation, deletion and displaying of the elements of the circular linked list.
我们基本上有3个节点,分别是头,尾和临时节点,以实现循环链表。 后方指向列表的最后一个节点,顶部指向第一个节点。 这有助于保持关于圆的前后节点在哪里的轨迹。 让我们看一下基本操作,例如创建,删除和显示循环链表的元素。
Creation of Node
创建节点
This is much similar to that of creating a node in singly linked list. It involves creating a new node and assigning data and pointing the current node to the head of the circular linked list. The code is as shown below.
这与在单链表中创建节点的过程非常相似。 它涉及创建一个新节点并分配数据,并将当前节点指向循环链表的开头。 代码如下所示。
void create()
{
node *newnode;
newnode=(node*)malloc(sizeof(node));
printf("\nEnter the node value : ");
scanf("%d",&newnode->info);
newnode->next=NULL;
if(rear==NULL)
front=rear=newnode;
else
{
rear->next=newnode;
rear=newnode;
}
rear->next=front;
}
Deletion of Node
删除节点
We conventionally delete the front node from the list in this program. To delete a node, we need to check if the list is empty. If it is not empty then point the rear node to the front->next and rear->next to front. This removes the first node.
我们通常按此程序从列表中删除前端节点。 要删除节点,我们需要检查列表是否为空。 如果它不为空,则将后节点指向front-> next,然后将tail-> next指向front。 这将删除第一个节点。
void del()
{
temp=front;
if(front==NULL)
printf("\nUnderflow :");
else
{
if(front==rear)
{
printf("\n%d",front->info);
front=rear=NULL;
}
else
{
printf("\n%d",front->info);
front=front->next;
rear->next=front;
}
temp->next=NULL;
free(temp);
}
}
Traversing Circular Linked List
遍历循环链接列表
Traversing the circular list starts from front node and iteratively continues until the rear node. The following function is used for this purpose.
遍历循环列表从前节点开始,然后反复进行直到后节点。 为此使用以下功能。
void display()
{
temp=front;
if(front==NULL)
printf("\nEmpty");
else
{
printf("\n");
for(;temp!=rear;temp=temp->next)
printf("\n%d address=%u next=%u\t",temp->info,temp,temp->next);
printf("\n%d address=%u next=%u\t",temp->info,temp,temp->next);
}
}
完成程序 ( Complete Program)
#include<stdio.h>
#include<stdlib.h>
typedef struct Node
{
int info;
struct Node *next;
}node;
node *front=NULL,*rear=NULL,*temp;
void create();
void del();
void display();
int main()
{
int chc;
do
{
printf("\nMenu\n\t 1 to create the element : ");
printf("\n\t 2 to delete the element : ");
printf("\n\t 3 to display the queue : ");
printf("\n\t 4 to exit from main : ");
printf("\nEnter your choice : ");
scanf("%d",&chc);
switch(chc)
{
case 1:
create();
break;
case 2:
del();
break;
case 3:
display();
break;
case 4:
return 1;
default:
printf("\nInvalid choice :");
}
}while(1);
return 0;
}
void create()
{
node *newnode;
newnode=(node*)malloc(sizeof(node));
printf("\nEnter the node value : ");
scanf("%d",&newnode->info);
newnode->next=NULL;
if(rear==NULL)
front=rear=newnode;
else
{
rear->next=newnode;
rear=newnode;
}
rear->next=front;
}
void del()
{
temp=front;
if(front==NULL)
printf("\nUnderflow :");
else
{
if(front==rear)
{
printf("\n%d",front->info);
front=rear=NULL;
}
else
{
printf("\n%d",front->info);
front=front->next;
rear->next=front;
}
temp->next=NULL;
free(temp);
}
}
void display()
{
temp=front;
if(front==NULL)
printf("\nEmpty");
else
{
printf("\n");
for(;temp!=rear;temp=temp->next)
printf("\n%d address=%u next=%u\t",temp->info,temp,temp->next);
printf("\n%d address=%u next=%u\t",temp->info,temp,temp->next);
}
}
Output
输出量
The above program results in the following output. You can see that the last node points to the first node address that is the main theme of circular linked list in C.
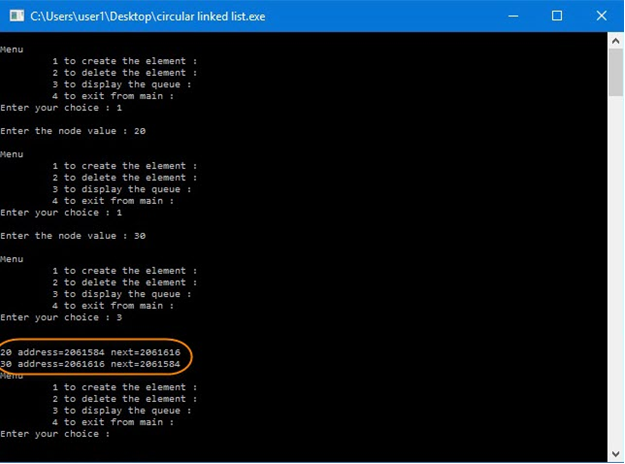
上面的程序产生以下输出。 您可以看到最后一个节点指向第一个节点地址,这是C中循环链接列表的主要主题。
翻译自: https://www.thecrazyprogrammer.com/2015/12/circular-linked-list-in-c.html
列表循环添加链接