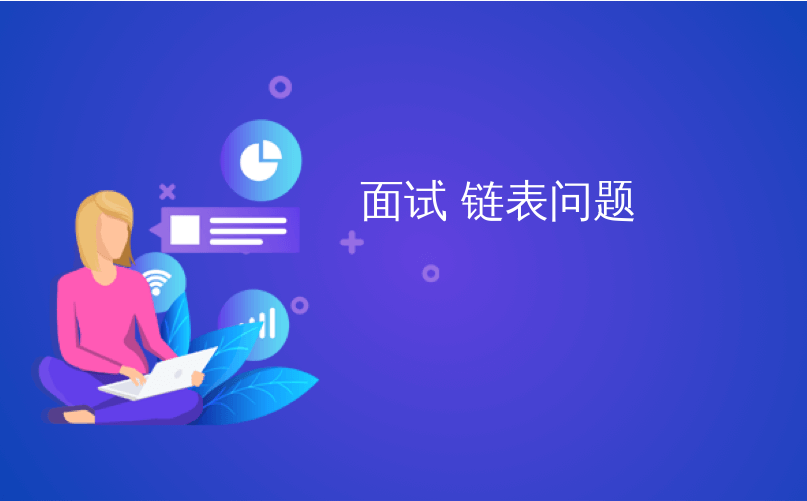
面试 链表问题
Here you will get some basic and advance linked list interview questions and answers.
在这里,您将获得一些基本和高级的链表面试问题和答案。
Linked lists happen to have very complex interview questions. They are generally small, powerful but if you fully don’t understand the principles they become complicated. Your code solutions that include linked listings are speedy and smaller.
链接列表碰巧有非常复杂的面试问题。 它们通常很小,功能强大,但是如果您完全不了解其原理,它们将变得很复杂。 您的包含链接列表的代码解决方案快速而又小巧。
1. What is a Linked list?
1.什么是链接列表?
Linked list is an ordered set of data elements, each containing a link to its successor (and typically its predecessor).
链表是一组有序的数据元素,每个元素都包含一个指向其后继(通常是其前任)的链接。
2. Can you represent a Linked list graphically?
2.您可以用图形表示链接列表吗?
The fundamental data structure for the linked record involves 3 segments: the data itself and also the link to another element. Together (data + link) this particular structure is normally called the Node.
链接记录的基本数据结构包括3个部分:数据本身以及与另一个元素的链接。 一起(数据+链接),此特定结构通常称为节点。
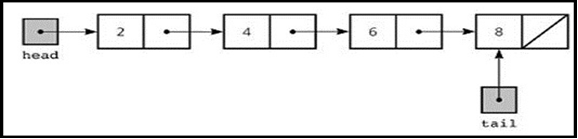
3. How many pointers are required to implement a simple Linked list?
3.实现一个简单的链表需要多少个指针?
You can find generally 3 pointers engaged:
您通常可以找到3个参与的指针:
A head pointer, pointing to the start of the record.
头指针,指向记录的开始。
A tail pointer, pointing on the last node of the list. The key property in the last node is that its subsequent pointer points to nothing at all (NULL).
尾指针,指向列表的最后一个节点。 最后一个节点中的键属性是其后续指针完全不指向任何内容(NULL)。
A pointer in every node, pointing to the next node element.
每个节点中的指针,指向下一个节点元素。
4. How many types of Linked lists are there?
4.有多少种链接列表?
Singly linked list, doubly linked list, multiply linked list, Circular Linked list.
单链表, 双链表 ,多链表,循环链表。
5. How to represent a linked list node?
5.如何表示一个链表节点?
The simplest representation of a linked list node is wrapping the data and the link using a typedef structure and giving the structure as a Node pointer that points to the next node. An example representation in C is
链接列表节点的最简单表示是使用typedef结构包装数据和链接,并将该结构作为指向下一个节点的Node指针给出。 C中的示例表示为
/*ll stands for linked list*/
typedef struct ll
{
int data;
struct ll *next;
} Node;
6. Describe the steps to insert data at the starting of a singly linked list.
6.描述在单链表的开头插入数据的步骤。
Inserting data at the beginning of the linked list involves creation of a new node, inserting the new node by assigning the head pointer to the new node next pointer and updating the head pointer to the point the new node. Consider inserting a temp node to the first of list
在链表的开头插入数据涉及创建新节点,通过将头指针分配给新节点的下一个指针以及将头指针更新到新节点的点来插入新节点。 考虑将临时节点插入列表的第一个
Node *head;
void InsertNodeAtFront(int data)
{
/* 1. create the new node*/
Node *temp = new Node;
temp->data = data;
/* 2. insert it at the first position*/
temp->next = head;
/* 3. update the head to point to this new node*/
head = temp;
}
7. How to insert a node at the end of Linked list?
7.如何在链接列表的末尾插入节点?
This case is a little bit more complicated. It depends on your implementation. If you have a tail pointer, it’s simple. In case you do not have a tail pointer, you will have to traverse the list till you reach the end (i.e. the next pointer is NULL), then create a new node and make that last node’s next pointer point to the new node.
这种情况有点复杂。 这取决于您的实现。 如果您有尾巴指针,这很简单。 如果没有尾指针,则必须遍历列表直到到达末尾(即下一个指针为NULL),然后创建一个新节点,并使最后一个节点的下一个指针指向新节点。
void InsertNodeAtEnd(int data)
{
/* 1. create the new node*/
Node *temp = new Node;
temp->data = data;
temp->next = NULL;
/* check if the list is empty*/
if (head == NULL)
{
head = temp;
return;
}
else
{
/* 2. traverse the list till the end */
Node *traveller = head;
while (traveler->next != NULL)
traveler = traveler->next;
/* 3. update the last node to point to this new node */
traveler->next = temp;
}
}
8. How to insert a node in random location in the list?
8.如何在列表中的随机位置插入节点?
As above, you’d initial produce the new node. Currently if the position is one or the list is empty, you’d insert it initially position. Otherwise, you’d traverse the list until either you reach the specified position or the list ends. Then you’d insert this new node. Inserting within the middle is that the difficult case as you have got to make sure you do the pointer assignment within the correct order. First, you’d set the new nodes next pointer to the node before that the new node is being inserted. Then you’d set the node to the position to purpose to the new node. Review the code below to get an idea.
如上所述,您将首先生成新节点。 当前,如果位置为一个或列表为空,则将其插入到初始位置。 否则,您将遍历列表,直到到达指定位置或列表结束为止。 然后,您将插入这个新节点。 插入中间是困难的情况,因为必须确保以正确的顺序进行指针分配。 首先,在插入新节点之前,将新节点的下一个指针设置为该节点。 然后,将节点设置为新节点的目标位置。 查看下面的代码以了解一个想法。
void InsertNode(int data, int position)
{
/* 1. create the new node */
Node *temp = new Node;
temp->data = data;
temp->next = NULL;
/* check if the position to insert is first or the list is empty */
if ((position == 1) || (head == NULL))
{
// set the new node to point to head
// as the list may not be empty
temp->next = head;
// point head to the first node now
head = temp;
return;
}
else
{
/* 2. traverse to the desired position */
Node *t = head;
int currPos = 2;
while ((currPos < position) && (t->next != NULL))
{
t = t->next;
currPos++;
}
/* 3. now we are at the desired location */
/* 4 first set the pointer for the new node */
temp->next = t->next;
/* 5 now set the previous node pointer */
t->next = temp;
}
}
9. How to delete a node from linked list?
9.如何从链表中删除节点?
The following are the steps to delete node from the list at the specified position.
以下是从列表中指定位置删除节点的步骤。
Set the head to point to the node that head is pointing to.
将头设置为指向头所指向的节点。
Traverse to the desired position or till the list ends; whichever comes first
移至所需位置或直到列表结束; 以先到者为准
You have to point the previous node to the next node.
您必须将上一个节点指向下一个节点。
10. How to reverse a singly linked list?
10.如何反向一个单链表 ?
First, set a pointer (*current) to point to the first node i.e. current=head.
首先,设置一个指针(* current)以指向第一个节点,即current = head。
Move ahead until current!=null (till the end)
向前移动直到当前!= null(直到最后)
set another pointer (*next) to point to the next node i.e. next=current->next
设置另一个指针(* next)指向下一个节点,即next = current-> next
store reference of *next in a temporary variable (*result) i.e. current->next=result
将* next的引用存储在一个临时变量(* result)中,即current-> next = result
swap the result value with current i.e. result=current
将结果值与当前交换,即result = current
And now swap the current value with next. i.e. current=next
现在,将当前值与下一个交换。 即当前=下一个
return result and repeat from step 2
返回结果并从步骤2开始重复
A linked list can also be reversed using recursion which eliminates the use of a temporary variable.
也可以使用递归来反向链接列表,这消除了使用临时变量的麻烦。
11. Compare Linked lists and Dynamic Arrays
11.比较链接列表和动态数组
A dynamic array is a data structure that allocates all elements contiguously in memory, and keeps a count of the present number of elements. If the area reserved for the dynamic array is exceeded, it’s reallocated and traced, a costly operation.
动态数组是一种数据结构,可在内存中连续分配所有元素,并保留当前元素的数量。 如果超出了为动态数组保留的区域,则会对其进行重新分配和跟踪,这是一项昂贵的操作。
Linked lists have many benefits over dynamic arrays. Insertion or deletion of an element at a specific point of a list, is a constant-time operation, whereas insertion in a dynamic array at random locations would require moving half the elements on the average, and all the elements in the worst case.
链接列表比动态数组有很多好处。 在列表的特定点插入或删除元素是固定时间的操作,而在随机位置的动态数组中插入则需要平均移动一半元素,而在最坏的情况下则需要移动所有元素。
Whereas one can delete an element from an array in constant time by somehow marking its slot as vacant, this causes fragmentation that impedes the performance of iteration.
通过以某种方式将其插槽标记为空,可以在恒定时间内从数组中删除一个元素,但这会导致碎片化,从而阻碍了迭代的性能。
12. What is a Circular Linked list?
12.什么是循环链接列表?
In the last node of a singly linear list, the link field often contains a null reference. A less common convention is to make the last node to point to the first node of the list; in this case the list is said to be ‘circular’ or ‘circularly linked’.
在单个线性列表的最后一个节点中,链接字段通常包含空引用。 较不常见的约定是使最后一个节点指向列表的第一个节点。 在这种情况下,该列表被称为“循环”或“循环链接”。
13. What is the difference between singly and doubly linked lists?
13.单链表和双链表有什么区别?
A doubly linked list whose nodes contain three fields: an integer value and two links to other nodes one to point to the previous node and other to point to the next node. Whereas a singly linked list contains points only to the next node.
一个双向链接列表,其节点包含三个字段:一个整数值和两个指向其他节点的链接,一个指向上一个节点,另一个指向下一个节点。 而单链列表仅包含指向下一个节点的点。
14. What are the applications that use Linked lists?
14.使用链接列表的应用程序是什么?
Both stacks and queues are often implemented using linked lists, other applications are skip list, binary tree, unrolled linked list, hash table, heap, self-organizing list.
堆栈和队列通常都使用链接列表来实现,其他应用程序是跳过列表 ,二叉树, 展开的链接列表 ,哈希表,堆,自组织列表。
15. How to remove loops in a linked list (or) what are fast and slow pointers used for?
15.如何删除链表中的循环(或快速和慢速指针分别用于什么)?
The best solution runs in O(N) time and uses O(1) space. This method uses two pointers (one slow pointer and one fast pointer). The slow pointer traverses one node at a time, while the fast pointer traverses twice as fast as the first one. If the linked list has loop in it, eventually the fast and slow pointer will be at the same node. On the other hand, if the list has no loop, obviously the fast pointer will reach the end of list before the slow pointer does. Hence we detect a loop.
最佳解决方案以O(N)时间运行,并使用O(1)空间。 此方法使用两个指针(一个慢速指针和一个快速指针)。 慢速指针一次遍历一个节点,而快速指针一次遍历第一个节点的两倍。 如果链表中有循环,则最终快和慢指针将位于同一节点。 另一方面,如果列表没有循环,则显然快速指针将在慢指针之前到达列表的末尾。 因此,我们检测到一个循环。
16. What will you prefer to use a singly or a doubly linked lists for traversing through a list of elements?
16.在遍历元素列表时,您更喜欢使用单链表还是双链表?
Double-linked lists require more space per node than singly liked lists, and their elementary operations such as insertion, deletion are more expensive; but they are often easier to manipulate because they allow fast and easy sequential access to the list in both directions. On the other hand, doubly linked lists cannot be used as persistent data structures. So, for traversing through a list of node, doubly linked list would be a better choice.
双重链接列表比单个喜欢的列表每个节点需要更多的空间,并且它们的基本操作(如插入,删除)更昂贵; 但它们通常更易于操作,因为它们允许在两个方向上快速便捷地顺序访问列表。 另一方面,双向链接列表不能用作持久性数据结构。 因此,对于遍历节点列表,双链表将是一个更好的选择。
If you found any mistake in above linked list interview questions and answers then please mention it by commenting below.
如果您在上面的链接列表访谈问题和答案中发现任何错误,请在下面评论中提及。
翻译自: https://www.thecrazyprogrammer.com/2015/12/linked-list-interview-questions-and-answers.html
面试 链表问题