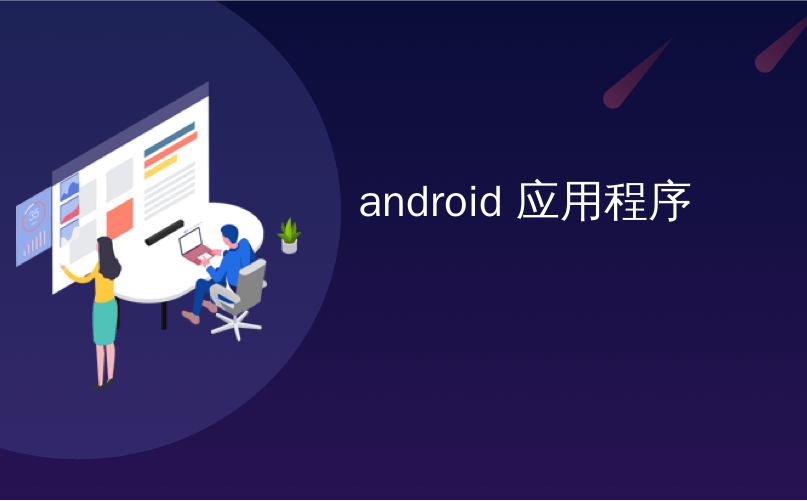
android 应用程序
In this tutorial I will give you a basic overview and explain fundamentals related to android application.
在本教程中,我将为您提供基本概述并解释与android应用程序相关的基础知识。
Activity
活动
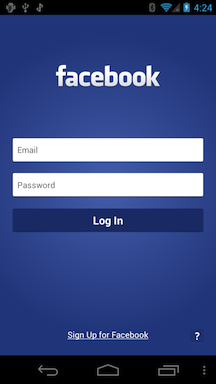
An Activity is a screen or window that interacts with the user. Activity is responsible for holding various UI components like label, button, textbox, etc. An app can have number of activities. Below I have given the login screen of Facebook app. It is an example of Activity. Basically there are two files associated with a single activity. One is a Java file that contains working related code and another is an XML file that contains design related code.
活动是与用户交互的屏幕或窗口。 活动负责保存各种UI组件,例如标签,按钮,文本框等。一个应用程序可以具有许多活动。 下面我给出了Facebook应用程序的登录屏幕。 这是一个活动的例子。 基本上有两个文件与一个活动相关联。 一个是包含工作相关代码的Java文件,另一个是包含设计相关代码的XML文件。
Now I will explain about the various files and folders used in an android app.
现在,我将说明Android应用程序中使用的各种文件和文件夹。
The below image shows the project structure of an app in Android Studio.
下图显示了Android Studio中应用程序的项目结构。
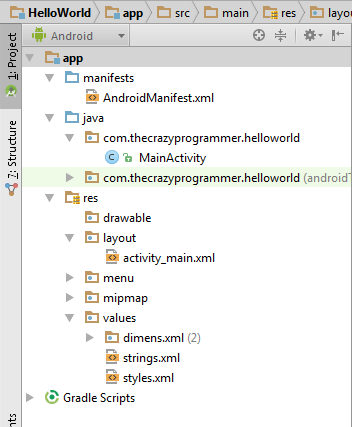
app
应用程式
It is the root folder that contains all other sub-folders.
它是包含所有其他子文件夹的根文件夹。
表现 (manifests)
It contains AndroidManifest.xml file that gives information about the app to the android os.
它包含AndroidManifest.xml文件,该文件将有关应用程序的信息提供给android操作系统。
Java (java)
This folder contains packages and Java source files. By default it contains MainActivity.java, later on we can create more source files according to our need.
该文件夹包含软件包和Java源文件。 默认情况下,它包含MainActivity.java,稍后我们可以根据需要创建更多源文件。
资源 (res)
This folder contains all the extra resources required in our app.
此文件夹包含我们应用程序中所需的所有其他资源。
分辨率/可绘制 (res/drawable)
All the images of different resolutions are placed in this folder.
所有不同分辨率的图像都放置在此文件夹中。
分辨率/布局 (res/layout)
It contains layout xml files that define design or user interface. By default it contains activity_main.xml, later on we can create more layout files according to our need.
它包含定义设计或用户界面的布局xml文件。 默认情况下,它包含activity_main.xml,稍后我们可以根据需要创建更多布局文件。
RES /值 (res/values)
dimens.xml: It contains dimension files that defines various dimensions like margin, padding, etc.
dimens.xml:它包含定义各种尺寸(如边距,填充等)的尺寸文件。
strings.xml: In this file we can define different texts or strings that we use in app. For example name of activities, buttons, labels, etc.
strings.xml:在此文件中,我们可以定义在应用程序中使用的不同文本或字符串。 例如活动名称,按钮,标签等。
styles.xml: Here we define different styles for the components that we use in our design. For example size and color of buttons, labels, images, etc.
styles.xml:在这里,我们为设计中使用的组件定义了不同的样式。 例如按钮,标签,图像等的大小和颜色。
Below I have shared the code for the Hello World example that you have learnt to create in last tutorial. Now I will explain it in brief.
下面,我分享了您在上一教程中学到的Hello World示例的代码。 现在,我将简要解释它。
MainActivity.java (MainActivity.java)
package com.thecrazyprogrammer.helloworld;
import android.app.Activity;
import android.os.Bundle;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
It is the java source file associated with the activity that we have created.
这是与我们创建的活动关联的Java源文件。
The onCreate() method is the first method called when the activity is created.
onCreate()方法是创建活动时调用的第一个方法。
setContentView() method gives the information about which layout resource file to use in the activity. In this example I have used activity_main.xml.
setContentView()方法提供有关在活动中使用哪个布局资源文件的信息。 在此示例中,我使用了activity_main.xml。
activity_main.xml (activity_main.xml)
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<TextView android:text="@string/hello_world" android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
It is the xml file associated with the activity. It contains the code related to design. You can see in above code that <RelativeLayout> is the outermost tag. It is the layout tag that defines how UI components like buttons, labels, etc are arranged on activity. We will discuss about all layouts in detail in upcoming tutorials.
这是与活动关联的xml文件。 它包含与设计有关的代码。 您可以在上面的代码中看到<RelativeLayout>是最外面的标记。 它是布局标签,用于定义如何在活动中安排UI组件(如按钮,标签等)。 我们将在以后的教程中详细讨论所有布局。
RelativeLayout contains <TextView> tag. TextView is a UI component used to add a label or text on the activity.
RelativeLayout包含<TextView>标记。 TextView是一个UI组件,用于在活动上添加标签或文本。
Each tag has various attributes like android:layout_width and android:layout_height to give width and height.
每个标记都有各种属性,例如android:layout_width和android:layout_height来指定宽度和高度。
@string/hello_world refers to the Hello World string defined in strings.xml file.
@ string / hello_world表示在strings.xml文件中定义的Hello World字符串。
dimens.xml (dimens.xml)
<resources>
<!-- Default screen margins, per the Android Design guidelines. -->
<dimen name="activity_horizontal_margin">16dp</dimen>
<dimen name="activity_vertical_margin">16dp</dimen>
</resources>
Various dimensions are defined in dimens.xml file.
在dimens.xml文件中定义了各种尺寸。
strings.xml (strings.xml)
<resources>
<string name="app_name">Hello World</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
</resources>
Here we define different texts or strings that we use in app.
在这里,我们定义了我们在应用程序中使用的不同文本或字符串。
AndroidManifest.xml (AndroidManifest.xml)
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.thecrazyprogrammer.helloworld" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Every application must have an AndroidManifest.xml file. It gives essential information about app to the android os.
每个应用程序必须具有一个AndroidManifest.xml文件。 它为Android操作系统提供了有关应用程序的基本信息。
R.java (R.java)
It is an auto generated file and we should not modify its content. It contains the ids of all the resources present in res folder. Whenever we add new component in our layout xml file, its id is automatically generated in R.java file. We use these ids in our Java source file to perform any action on the components.
这是一个自动生成的文件,我们不应修改其内容。 它包含res文件夹中存在的所有资源的ID。 每当我们在布局xml文件中添加新组件时,其ID都会在R.java文件中自动生成。 我们在Java源文件中使用这些ID对组件执行任何操作。
I have tried my best to explain everything. If you have any doubts you can ask it by commenting below.
我已经尽力解释了一切。 如果您有任何疑问,可以通过以下评论来提出。
翻译自: https://www.thecrazyprogrammer.com/2015/11/basic-overview-of-android-application.html
android 应用程序